Code is copied!
How To Create a Emoji Adder Using Flutter
In this project,we will be building a simple flutter application called "Emoji Adder". The main goal of this project is to create an interactive mobile app where user can add multiple random emojis to the screen by clicking a button The game continues until 12 emojis are reached, at which point it transitions to another screen. This project demonstrates the basics of building a simple mobile application using Flutter and handling user interactions.
Before moving towards our "main.dart" file we first have to set two things :
1.Prepare Your Image Assets:
Ensure you have the image files you want to use in your flutter project.Create a folder named "images" in the root directory of your flutter project and place your images there.
2. We have to make some changes to our "pubspec.yaml" file. Follow the steps given below :
(a) At first you have uncomment the following lines in your "pubspec.yaml" file as shown below :
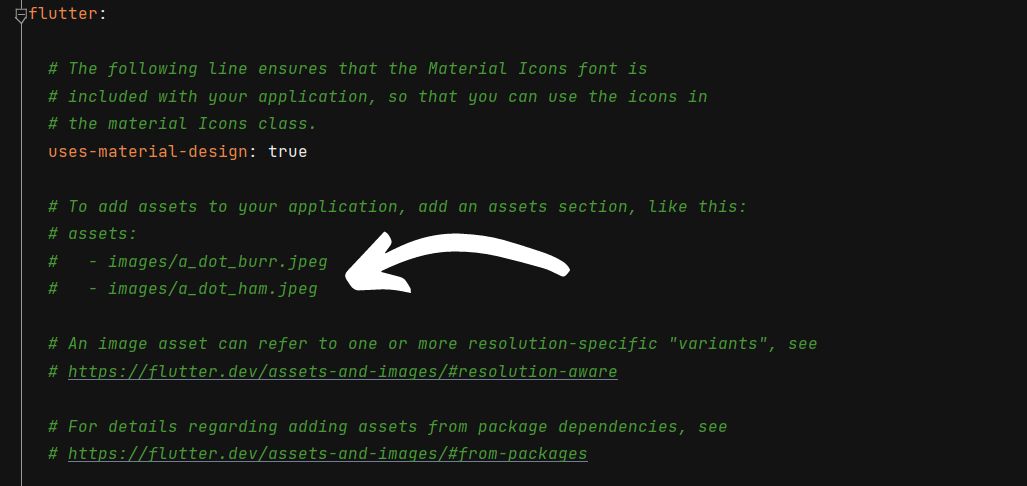
(b) Add your assets path inside your "pubspec.yaml" file as shown below :
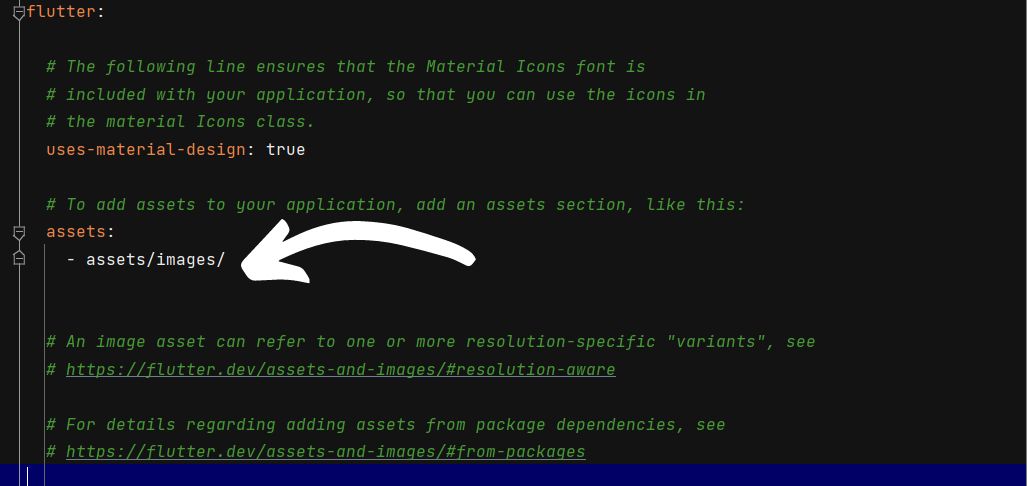
You can create only images folder rather than creating "assets/images" as per your choice. You just have to specify the path correctly.
(c) Then click on "Pub get" at the top right corner of your screen as shown below :
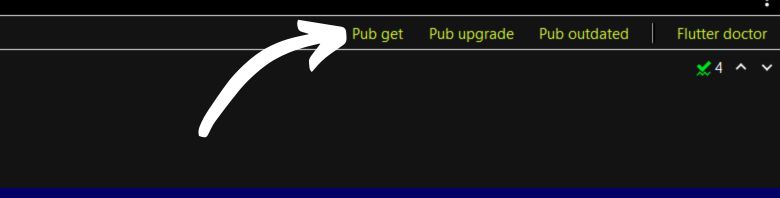
2. We have to make the same folder structure inside our root folder as we have specified in "pubspec.yaml" file and add and your images inside "images" folder (You can add images of your own choice) as shown below :
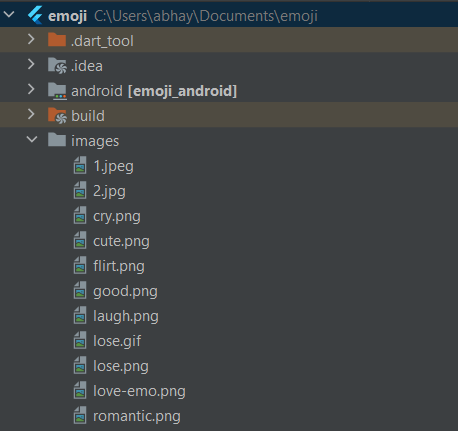
Source Code for the main.dart file
Add the following Code inside your main.dart file :
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:emoji/gameover.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: EmojiGame(),
);
}
}
class EmojiGame extends StatefulWidget {
const EmojiGame({Key? key}) : super(key: key);
@override
State< EmojiGame> createState() => _EmojiGameState();
}
class _EmojiGameState extends State< EmojiGame> {
int count = 0;
int counter = 0;
List< Widget> bodyElements = [];
List< String> emojis = ['love-emo', 'laugh', 'cry', 'cute', 'flirt', 'romantic', 'good'];
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.purple.shade50,
appBar: AppBar(
title: Text(
'EMOJI ADDER',
style: TextStyle(
color: Colors.white,
),
),
backgroundColor: const Color(0xFFBA68C8),
actions: [
Padding(
padding: const EdgeInsets.fromLTRB(0, 0, 60.0, 0),
child: Center(
child: Text(
"Points:${count}",
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.w400,
),
),
),
)
],
),
body: Wrap(
alignment: WrapAlignment.start,
spacing: 53,
runSpacing: 32,
children: bodyElements,
),
floatingActionButton: SizedBox(
height: 130.0,
width: 130.0,
child: FittedBox(
child: FloatingActionButton.extended(
backgroundColor: Colors.amber,
label: Text('Add'),
icon: const Icon(Icons.emoji_emotions_outlined),
onPressed: () {
setState(() {
counter = Random().nextInt(7);
count = count + 1;
if (bodyElements.length == 12) {
bodyElements.clear();
count = 0;
Navigator.push(
context,
MaterialPageRoute(builder: (context) => Over()),
);
}
bodyElements.add(
Image.asset(
'images/${emojis[counter]}.png',
fit: BoxFit.cover,
width: 70,
height: 70,
),
);
});
},
),
),
),
);
}
}
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:emoji/gameover.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: EmojiGame(),
);
}
}
class EmojiGame extends StatefulWidget {
const EmojiGame({Key? key}) : super(key: key);
@override
State< EmojiGame> createState() => _EmojiGameState();
}
class _EmojiGameState extends State< EmojiGame> {
int count = 0;
int counter = 0;
List< Widget> bodyElements = [];
List< String> emojis = ['love-emo', 'laugh', 'cry', 'cute', 'flirt', 'romantic', 'good'];
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.purple.shade50,
appBar: AppBar(
title: Text(
'EMOJI ADDER',
style: TextStyle(
color: Colors.white,
),
),
backgroundColor: const Color(0xFFBA68C8),
actions: [
Padding(
padding: const EdgeInsets.fromLTRB(0, 0, 60.0, 0),
child: Center(
child: Text(
"Points:${count}",
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.w400,
),
),
),
)
],
),
body: Wrap(
alignment: WrapAlignment.start,
spacing: 53,
runSpacing: 32,
children: bodyElements,
),
floatingActionButton: SizedBox(
height: 130.0,
width: 130.0,
child: FittedBox(
child: FloatingActionButton.extended(
backgroundColor: Colors.amber,
label: Text('Add'),
icon: const Icon(Icons.emoji_emotions_outlined),
onPressed: () {
setState(() {
counter = Random().nextInt(7);
count = count + 1;
if (bodyElements.length == 12) {
bodyElements.clear();
count = 0;
Navigator.push(
context,
MaterialPageRoute(builder: (context) => Over()),
);
}
bodyElements.add(
Image.asset(
'images/${emojis[counter]}.png',
fit: BoxFit.cover,
width: 70,
height: 70,
),
);
});
},
),
),
),
);
}
}
The Output is shown below :
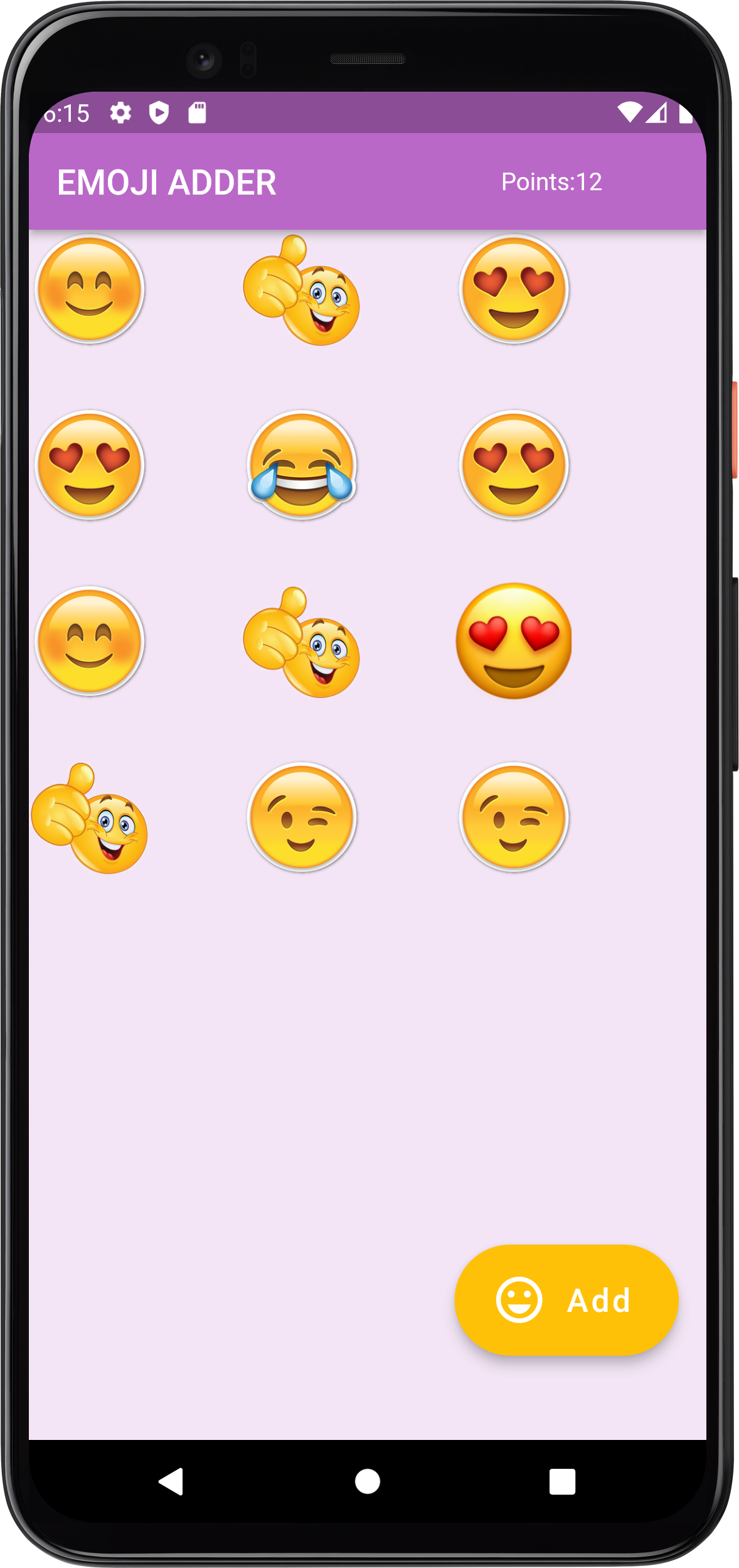
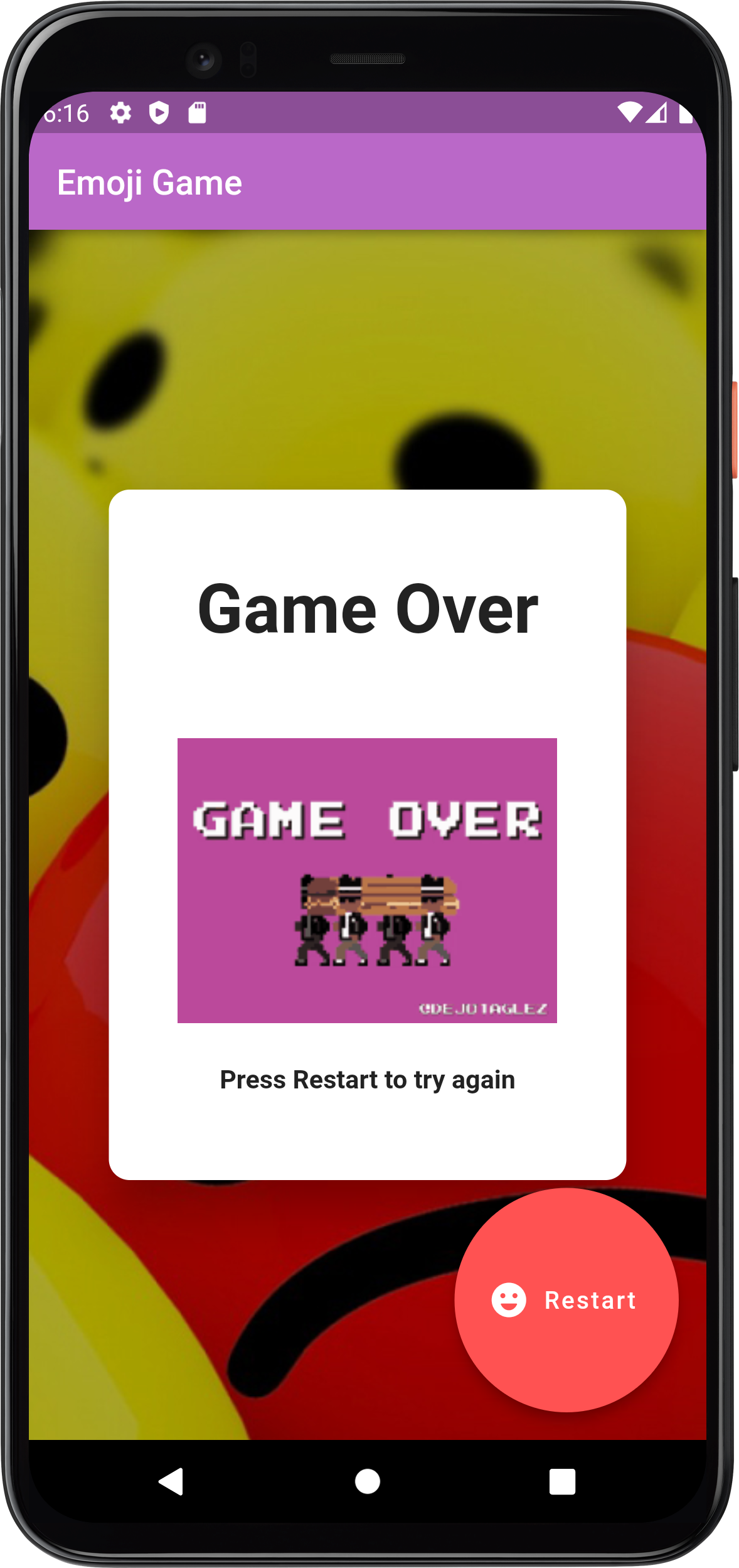
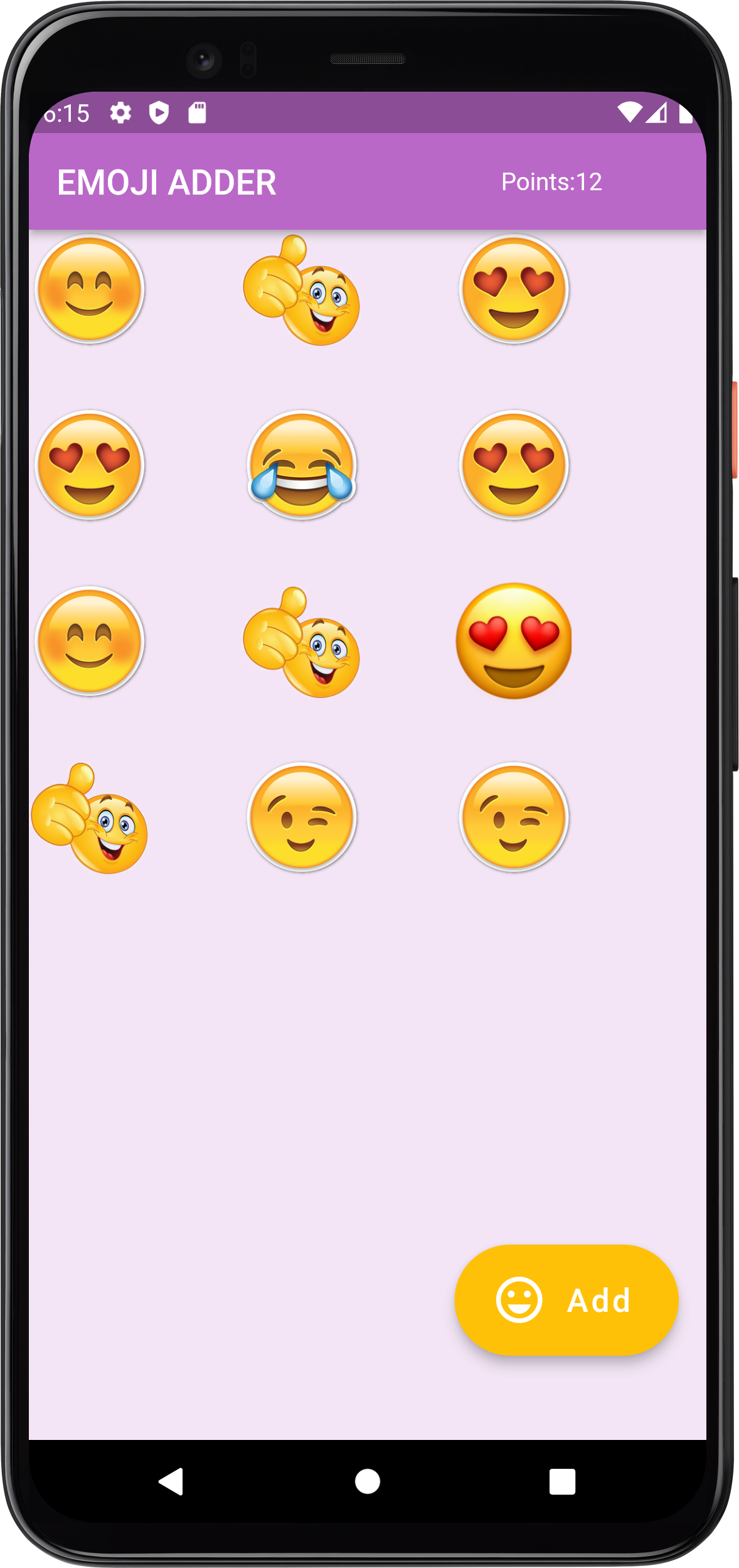
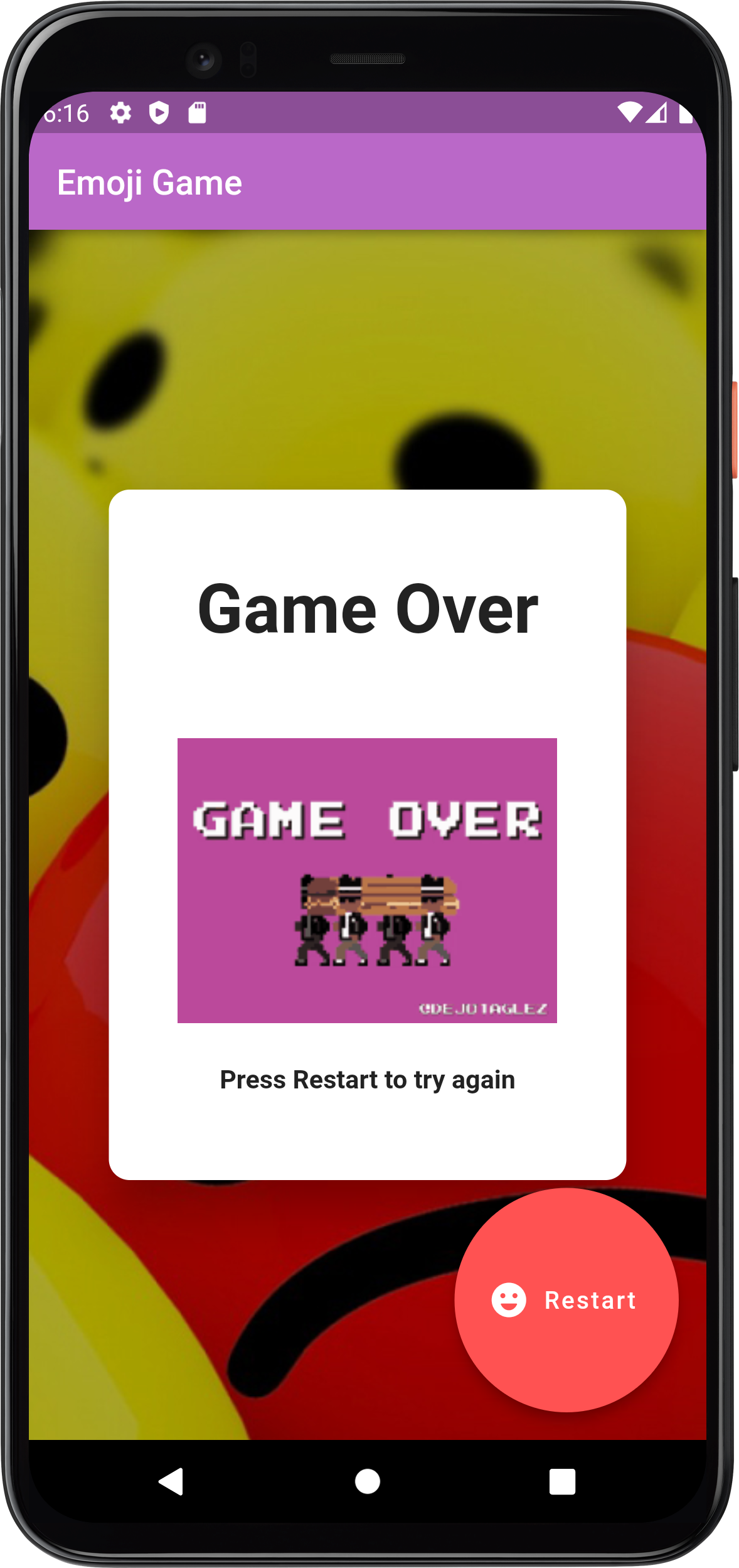