Code is copied!
Contact List App Using Flutter
In this tutorial, we'll be creating a Contact App application using Firebase in Flutter,
Contact app are essential app for keeping Contacts and staying organized.
Our app creates a scrollable contacts list , allowing users to save contact via a text field. Contacts are dynamically
displayed within containers. Users can scroll through the list as needed. Users can edit , delete their contact details.
By the end of this tutorial, you'll have a functional Contact List app that you can run on both Android and iOS
devices.
Source Code for the main.dart file
Add the following Code inside your main.dart file :
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:cloud_firestore/cloud_firestore.dart';
void main() async{
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Firebase Firestore',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State createState() => _HomePageState();
}
class _HomePageState extends State {
final TextEditingController _nameController = TextEditingController();
final TextEditingController _phoneController = TextEditingController();
final TextEditingController _nameController1 = TextEditingController();
final TextEditingController _phoneController1 = TextEditingController();
//add data
Future _create() async {
await showModalBottomSheet(
isScrollControlled: true,
context: context,
builder: (BuildContext ctx) {
return Padding(
padding: EdgeInsets.only(
top: 20,
left: 20,
right: 20,
bottom: MediaQuery.of(ctx).viewInsets.bottom + 20),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
SizedBox(
height: 20,
),
Text('Add Contact',
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 19,
fontWeight: FontWeight.w600
),),
SizedBox(
height: 20,
),
TextField(
controller: _nameController1,
decoration: const InputDecoration(labelText: 'Name'),
),
TextField(
controller: _phoneController1,
decoration: const InputDecoration(labelText: 'Phone'),
),
const SizedBox(
height: 20,
),
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.redAccent
),
child: const Text('Create'),
onPressed: () async
{
final String name = _nameController1.text;
final String phone = _phoneController1.text;
if (phone != null) {
await contact.add({"name": name, "phone": phone});
_nameController1.text = '';
_phoneController1.text = '';
Navigator.of(context).pop();
}
},
)
],
),
);
});
}
// update and delete
Future _update([DocumentSnapshot? documentSnapshot]) async {
if (documentSnapshot != null) {
_nameController.text = documentSnapshot['name'];
_phoneController.text = documentSnapshot['phone'];
}
await showModalBottomSheet(
isScrollControlled: true,
context: context,
builder: (BuildContext ctx) {
return Padding(
padding: EdgeInsets.only(
top: 20,
left: 20,
right: 20,
bottom: MediaQuery.of(ctx).viewInsets.bottom + 20),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
SizedBox(
height: 20,
),
Text('Update Contact',
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 19,
fontWeight: FontWeight.w600
),),
SizedBox(
height: 20,
),
TextField(
controller: _nameController,
decoration: const InputDecoration(labelText: 'Name'),
),
TextField(
controller: _phoneController,
decoration: const InputDecoration(labelText: 'Phone'),
),
const SizedBox(
height: 20,
),
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.redAccent
),
child: const Text( 'Update'),
onPressed: () async {
final String name = _nameController.text;
final String phone = _phoneController.text;
if (phone != null)
{
await contact.doc(documentSnapshot!.id).update({"name": name, "phone": phone});
_nameController.text = '';
_phoneController.text = '';
Navigator.of(context).pop();
}
},
)
],
),
);
});
}
Future _delete(String contactId) async {
await contact.doc(contactId).delete();
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('You have successfully deleted a contact')));
}
final CollectionReference contact =
FirebaseFirestore.instance.collection('contact');
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.redAccent,
title: const Center(child: Text('Contact List')),
),
body:
StreamBuilder(
stream: contact.snapshots(),
builder: (context, AsyncSnapshot streamSnapshot) {
if (streamSnapshot.hasData)
{
return ListView.builder(
itemCount: streamSnapshot.data!.docs.length,
itemBuilder: (context, index) {
final DocumentSnapshot documentSnapshot =
streamSnapshot.data!.docs[index];
return Card(
color: Colors.pink[50],
margin: const EdgeInsets.all(10),
child: ListTile(
title: Text(documentSnapshot['name'],style: TextStyle(
fontWeight: FontWeight.w600,
fontSize: 17
),),
subtitle: Text(documentSnapshot['phone'],style: TextStyle(
fontSize: 15,
fontWeight: FontWeight.w500
),),
trailing: SizedBox(
width: 100,
child: Row(
children: [
IconButton(
icon: const Icon(Icons.edit),
color: Colors.yellow[700],
onPressed: ()
{
_update(documentSnapshot);
}
),
IconButton(
icon: const Icon(Icons.delete),
color: Colors.red,
onPressed: ()
{
_delete(documentSnapshot.id);
}
),
],
),
),
),
);
},
);
}
return const Center(
child: CircularProgressIndicator(),
);
},
),
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.redAccent,
onPressed: (){
_create();
},
child: const Icon(Icons.add),
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat
);
}
}
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:cloud_firestore/cloud_firestore.dart';
void main() async{
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Firebase Firestore',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State createState() => _HomePageState();
}
class _HomePageState extends State {
final TextEditingController _nameController = TextEditingController();
final TextEditingController _phoneController = TextEditingController();
final TextEditingController _nameController1 = TextEditingController();
final TextEditingController _phoneController1 = TextEditingController();
//add data
Future _create() async {
await showModalBottomSheet(
isScrollControlled: true,
context: context,
builder: (BuildContext ctx) {
return Padding(
padding: EdgeInsets.only(
top: 20,
left: 20,
right: 20,
bottom: MediaQuery.of(ctx).viewInsets.bottom + 20),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
SizedBox(
height: 20,
),
Text('Add Contact',
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 19,
fontWeight: FontWeight.w600
),),
SizedBox(
height: 20,
),
TextField(
controller: _nameController1,
decoration: const InputDecoration(labelText: 'Name'),
),
TextField(
controller: _phoneController1,
decoration: const InputDecoration(labelText: 'Phone'),
),
const SizedBox(
height: 20,
),
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.redAccent
),
child: const Text('Create'),
onPressed: () async
{
final String name = _nameController1.text;
final String phone = _phoneController1.text;
if (phone != null) {
await contact.add({"name": name, "phone": phone});
_nameController1.text = '';
_phoneController1.text = '';
Navigator.of(context).pop();
}
},
)
],
),
);
});
}
// update and delete
Future _update([DocumentSnapshot? documentSnapshot]) async {
if (documentSnapshot != null) {
_nameController.text = documentSnapshot['name'];
_phoneController.text = documentSnapshot['phone'];
}
await showModalBottomSheet(
isScrollControlled: true,
context: context,
builder: (BuildContext ctx) {
return Padding(
padding: EdgeInsets.only(
top: 20,
left: 20,
right: 20,
bottom: MediaQuery.of(ctx).viewInsets.bottom + 20),
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
SizedBox(
height: 20,
),
Text('Update Contact',
textAlign: TextAlign.center,
style: TextStyle(
fontSize: 19,
fontWeight: FontWeight.w600
),),
SizedBox(
height: 20,
),
TextField(
controller: _nameController,
decoration: const InputDecoration(labelText: 'Name'),
),
TextField(
controller: _phoneController,
decoration: const InputDecoration(labelText: 'Phone'),
),
const SizedBox(
height: 20,
),
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.redAccent
),
child: const Text( 'Update'),
onPressed: () async {
final String name = _nameController.text;
final String phone = _phoneController.text;
if (phone != null)
{
await contact.doc(documentSnapshot!.id).update({"name": name, "phone": phone});
_nameController.text = '';
_phoneController.text = '';
Navigator.of(context).pop();
}
},
)
],
),
);
});
}
Future _delete(String contactId) async {
await contact.doc(contactId).delete();
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('You have successfully deleted a contact')));
}
final CollectionReference contact =
FirebaseFirestore.instance.collection('contact');
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.redAccent,
title: const Center(child: Text('Contact List')),
),
body:
StreamBuilder(
stream: contact.snapshots(),
builder: (context, AsyncSnapshot streamSnapshot) {
if (streamSnapshot.hasData)
{
return ListView.builder(
itemCount: streamSnapshot.data!.docs.length,
itemBuilder: (context, index) {
final DocumentSnapshot documentSnapshot =
streamSnapshot.data!.docs[index];
return Card(
color: Colors.pink[50],
margin: const EdgeInsets.all(10),
child: ListTile(
title: Text(documentSnapshot['name'],style: TextStyle(
fontWeight: FontWeight.w600,
fontSize: 17
),),
subtitle: Text(documentSnapshot['phone'],style: TextStyle(
fontSize: 15,
fontWeight: FontWeight.w500
),),
trailing: SizedBox(
width: 100,
child: Row(
children: [
IconButton(
icon: const Icon(Icons.edit),
color: Colors.yellow[700],
onPressed: ()
{
_update(documentSnapshot);
}
),
IconButton(
icon: const Icon(Icons.delete),
color: Colors.red,
onPressed: ()
{
_delete(documentSnapshot.id);
}
),
],
),
),
),
);
},
);
}
return const Center(
child: CircularProgressIndicator(),
);
},
),
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.redAccent,
onPressed: (){
_create();
},
child: const Icon(Icons.add),
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat
);
}
}
The Output is shown below :
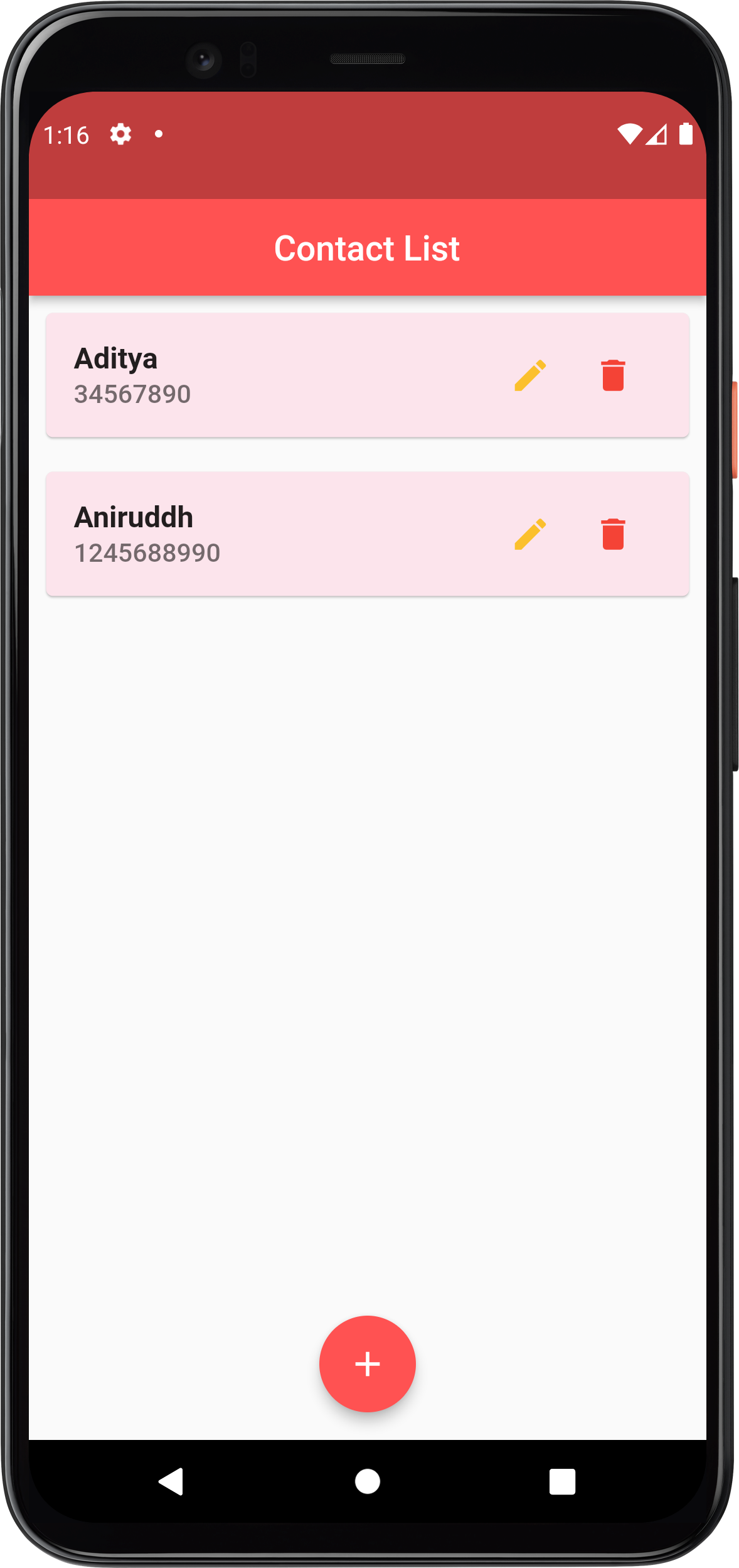
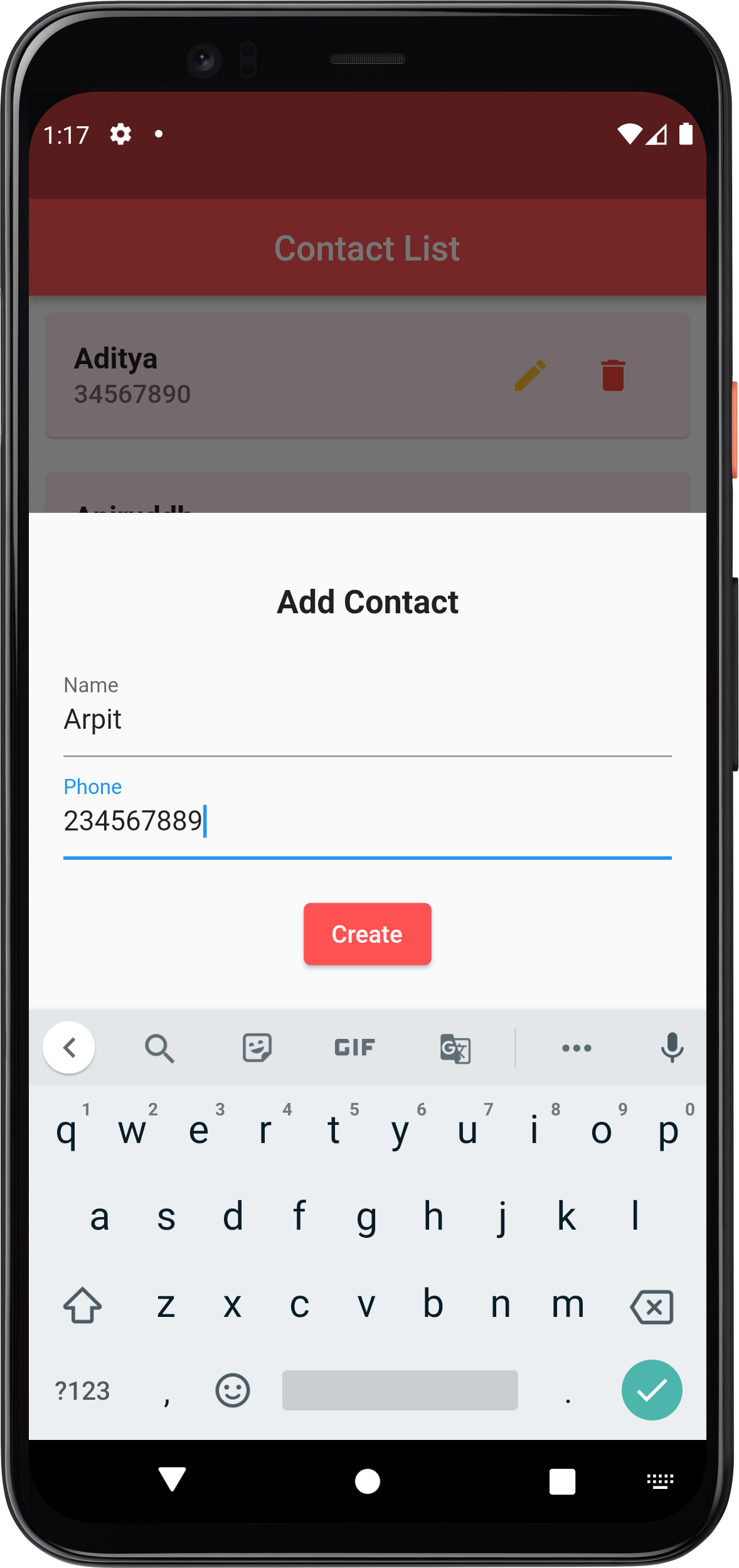
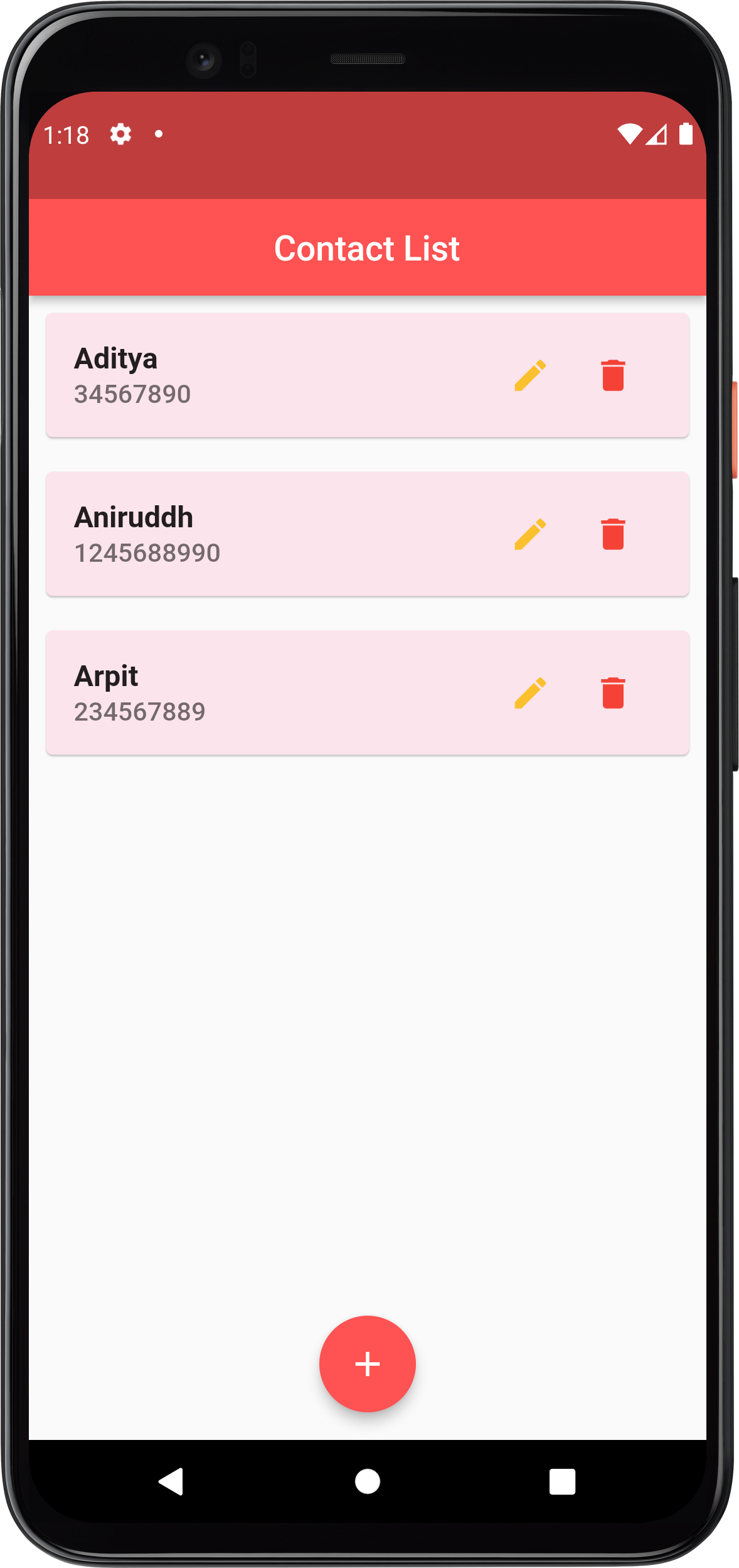
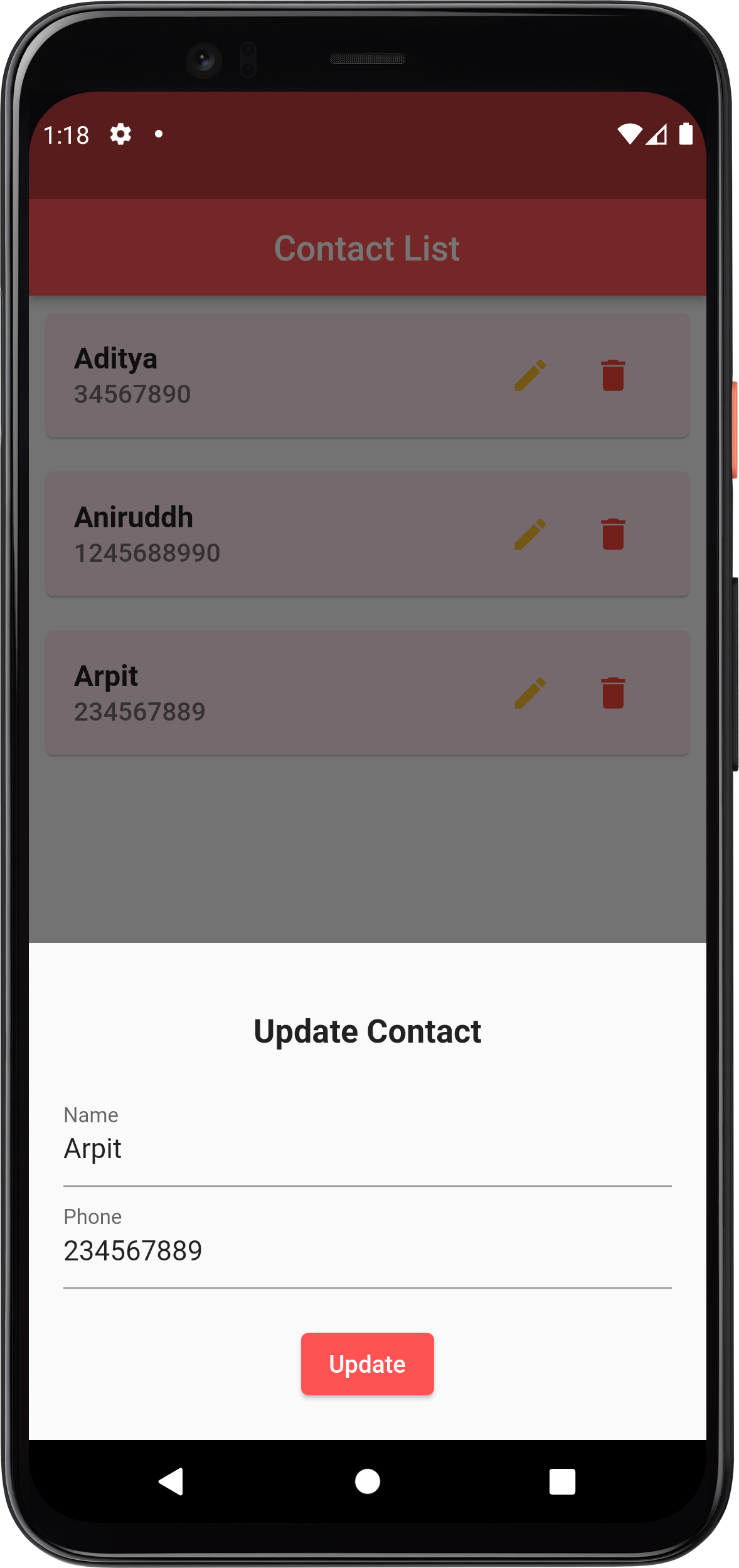
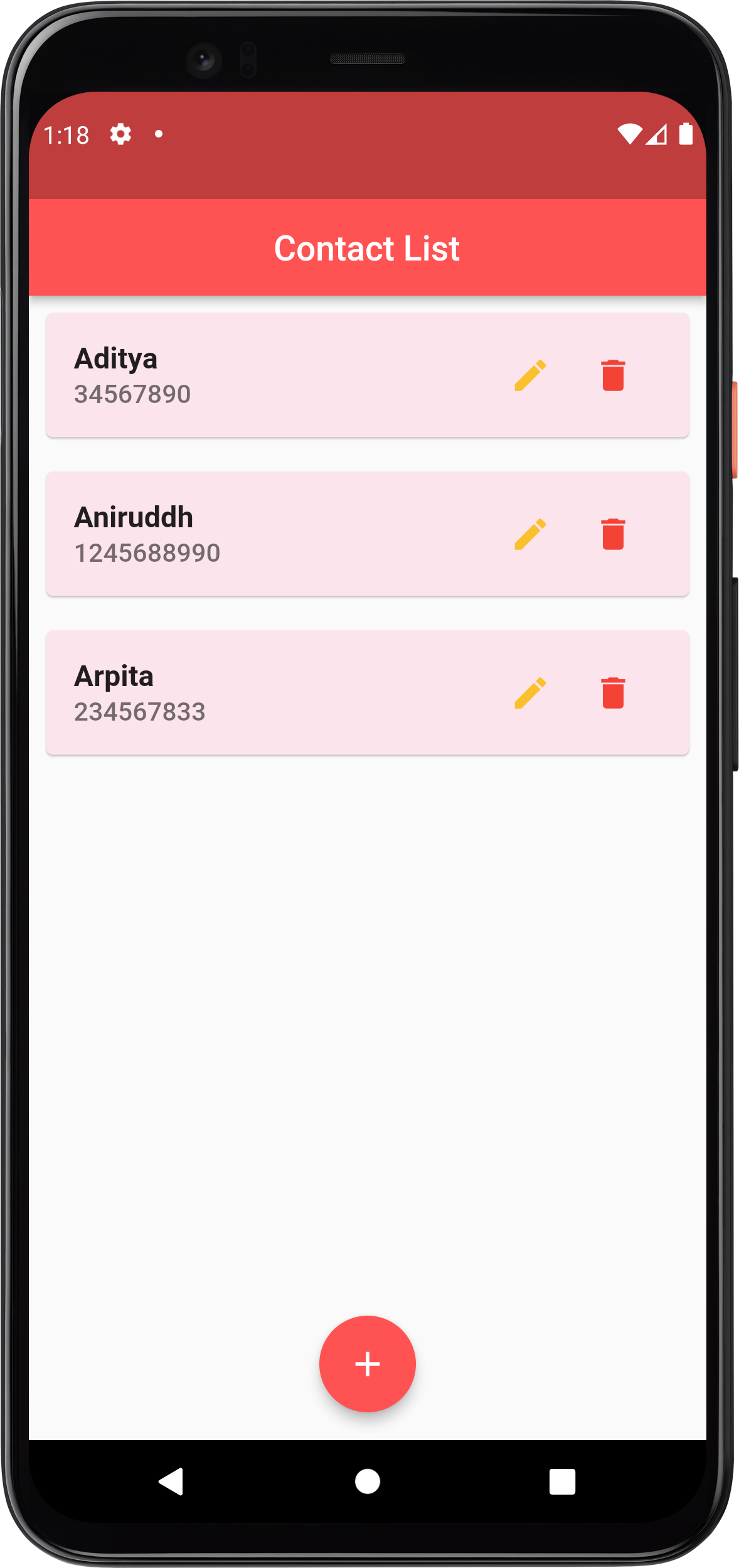
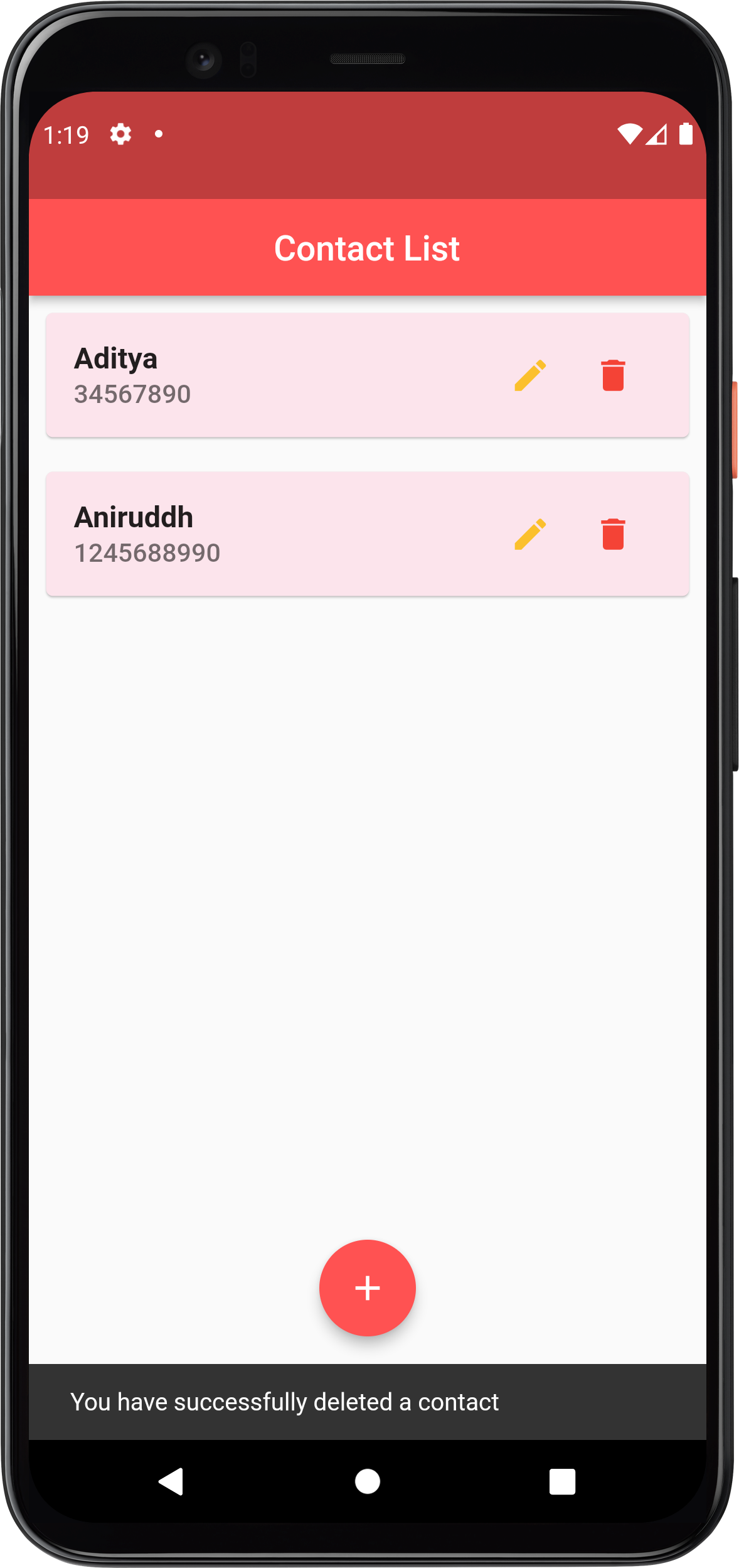
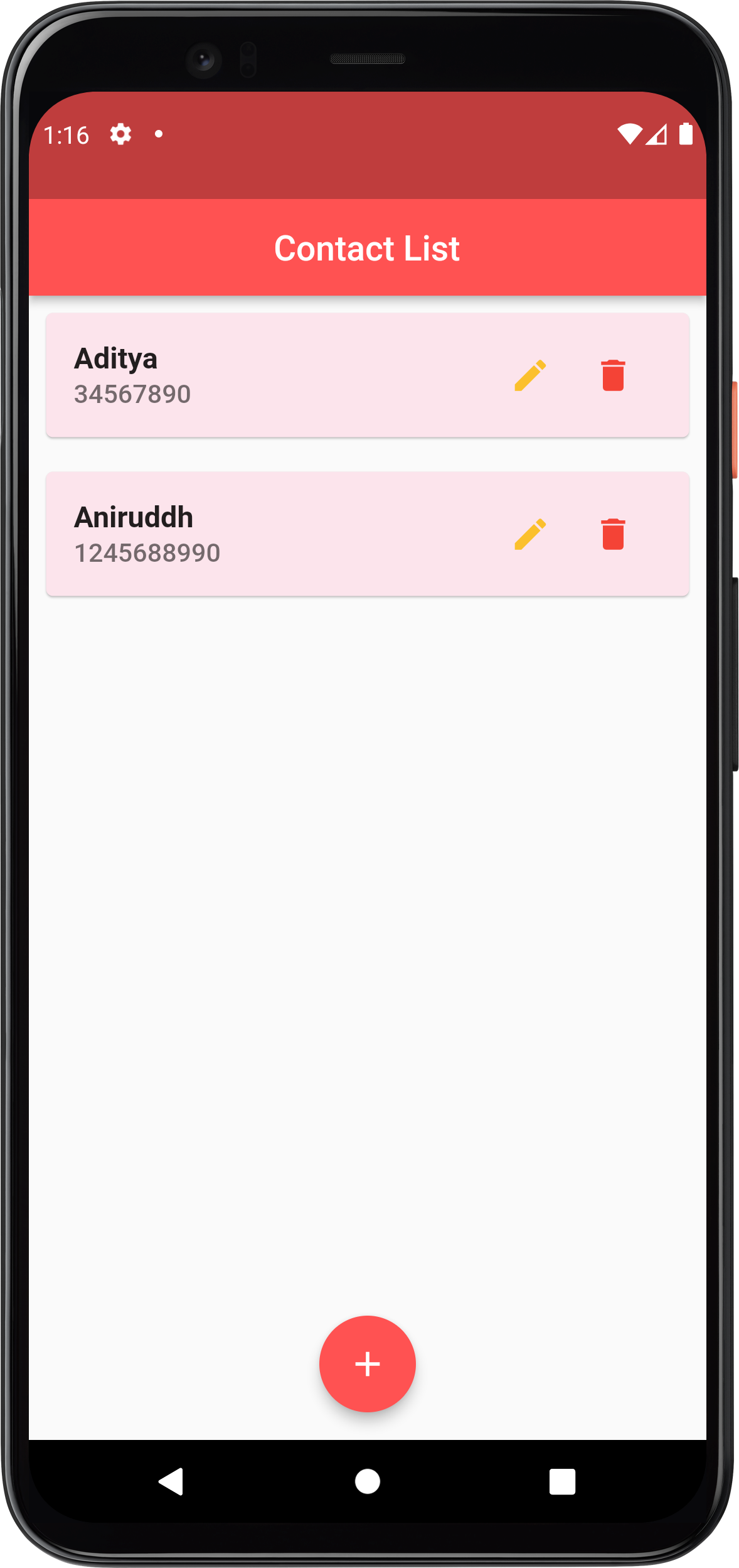
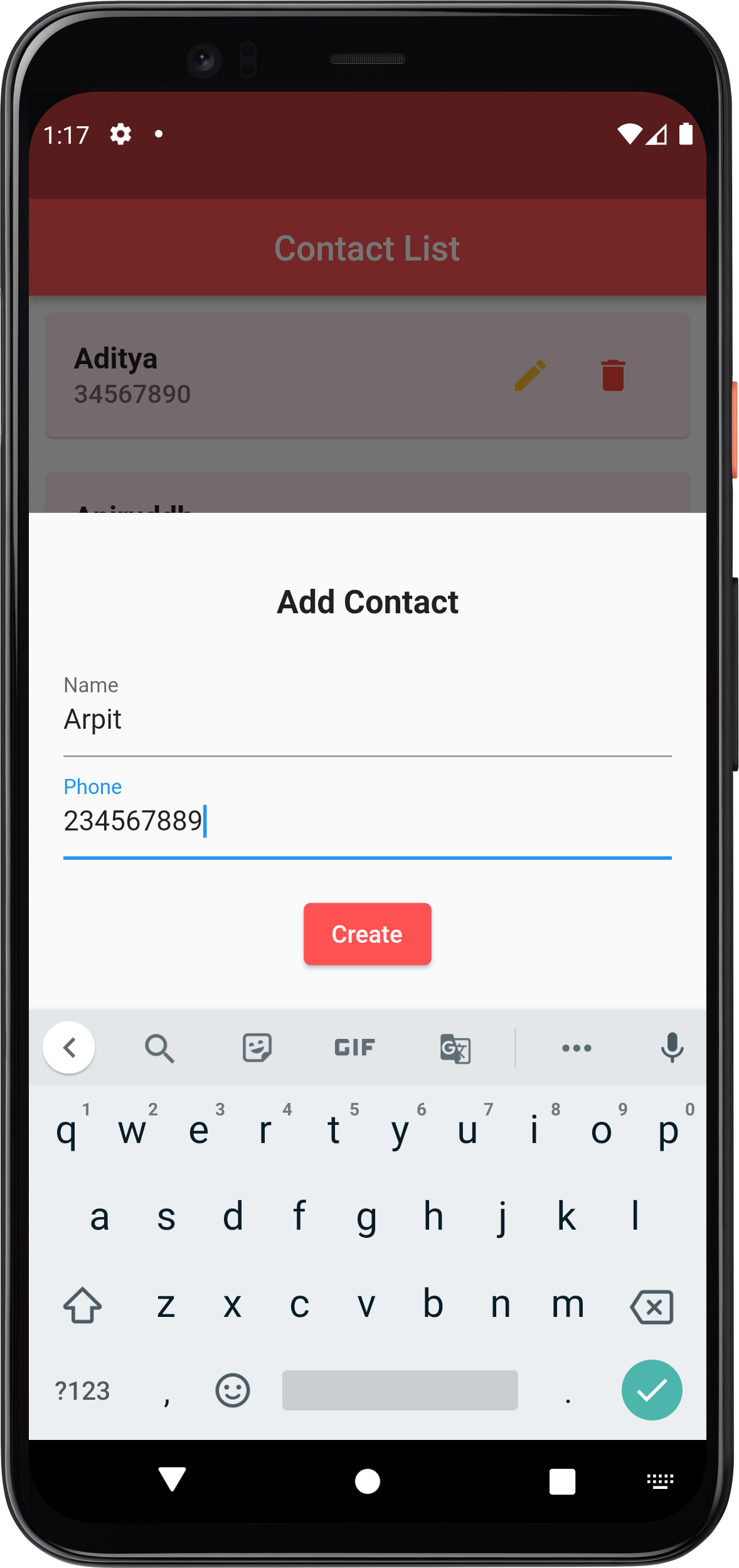
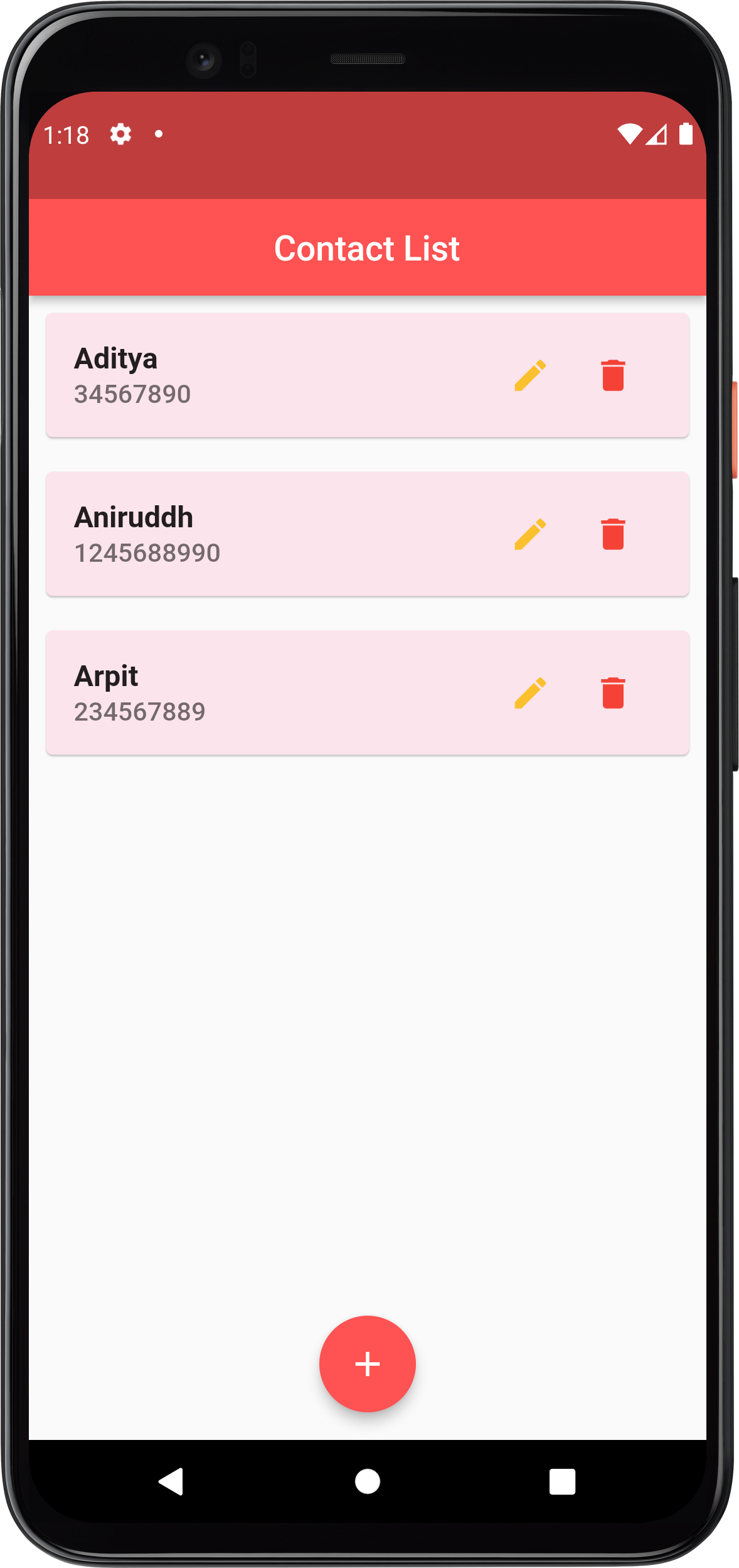
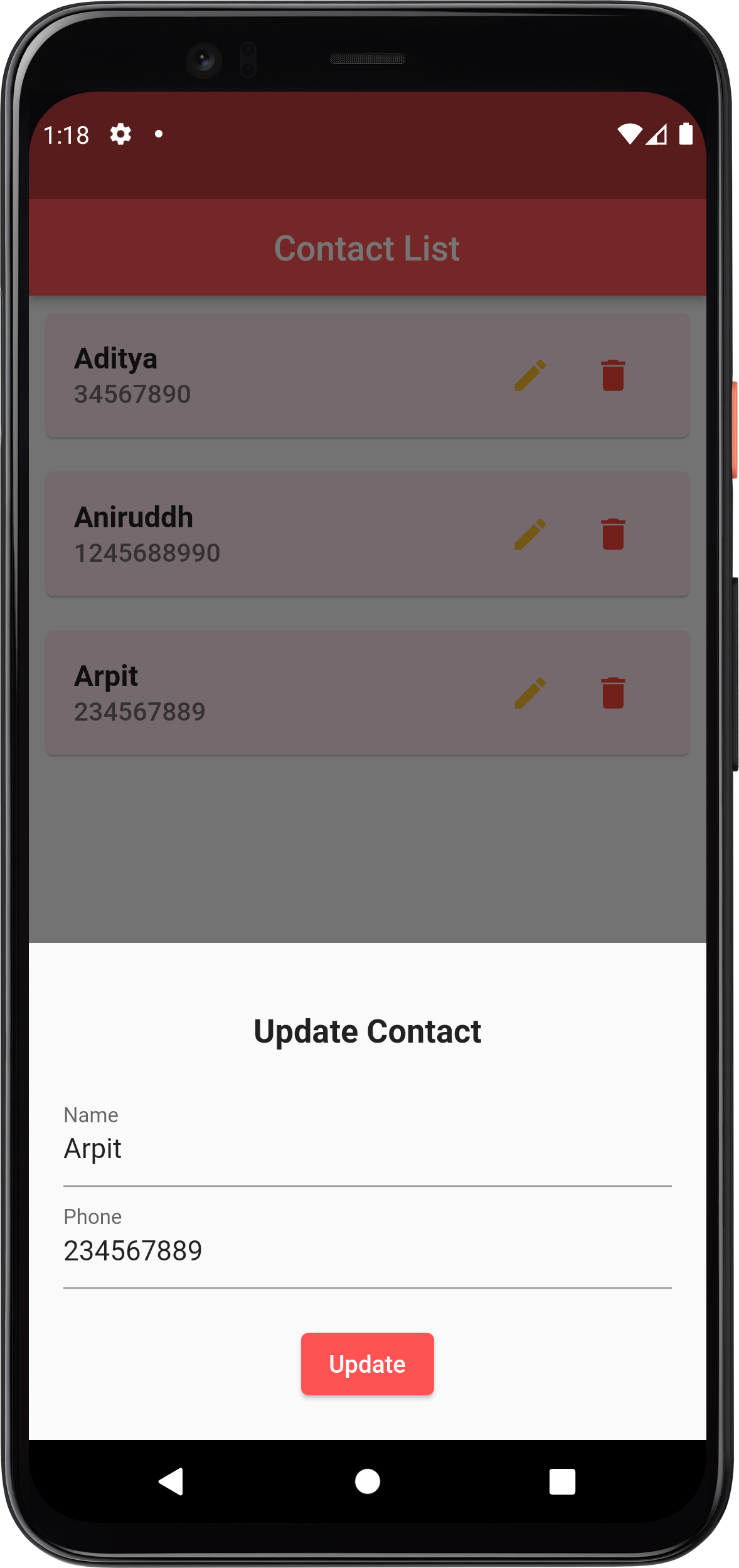
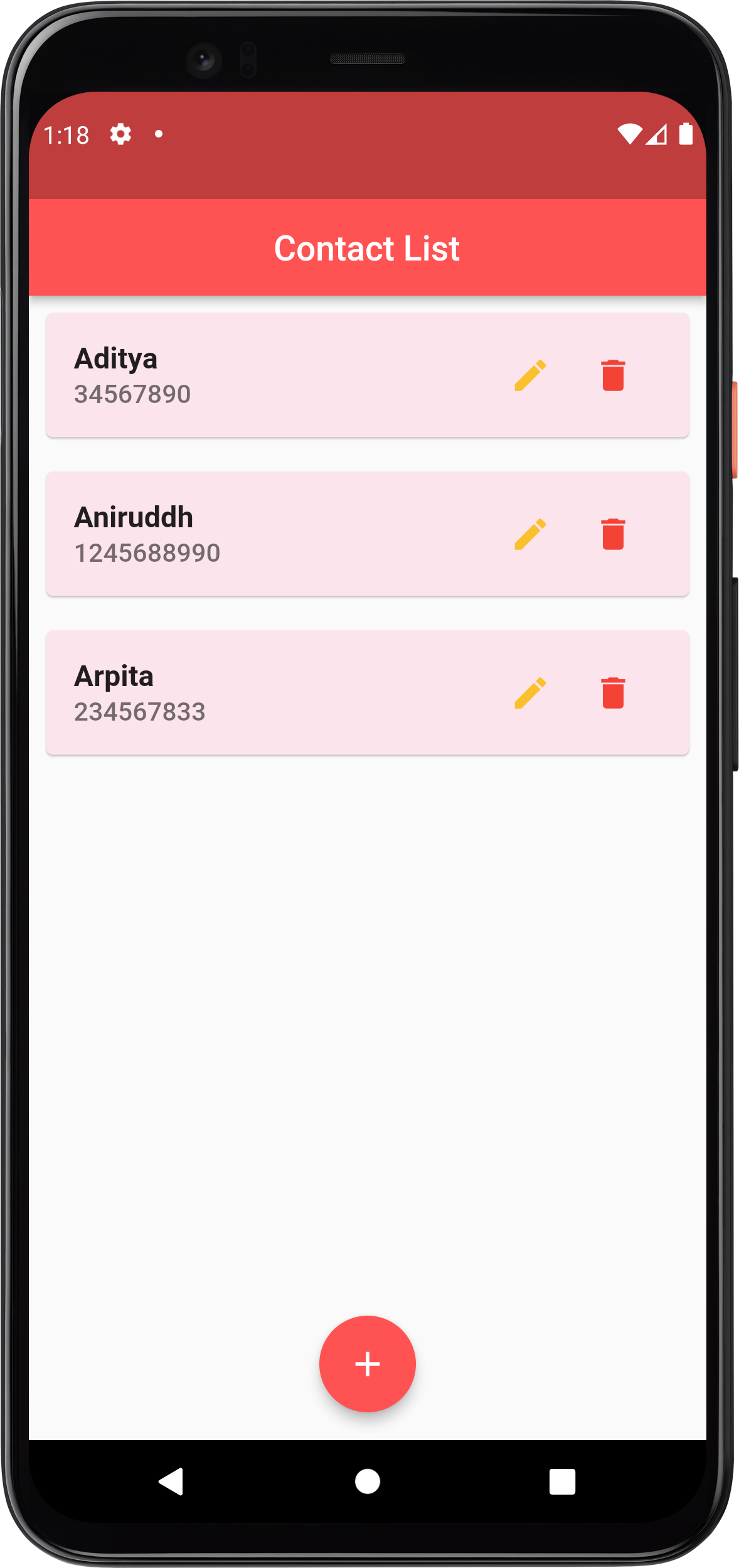
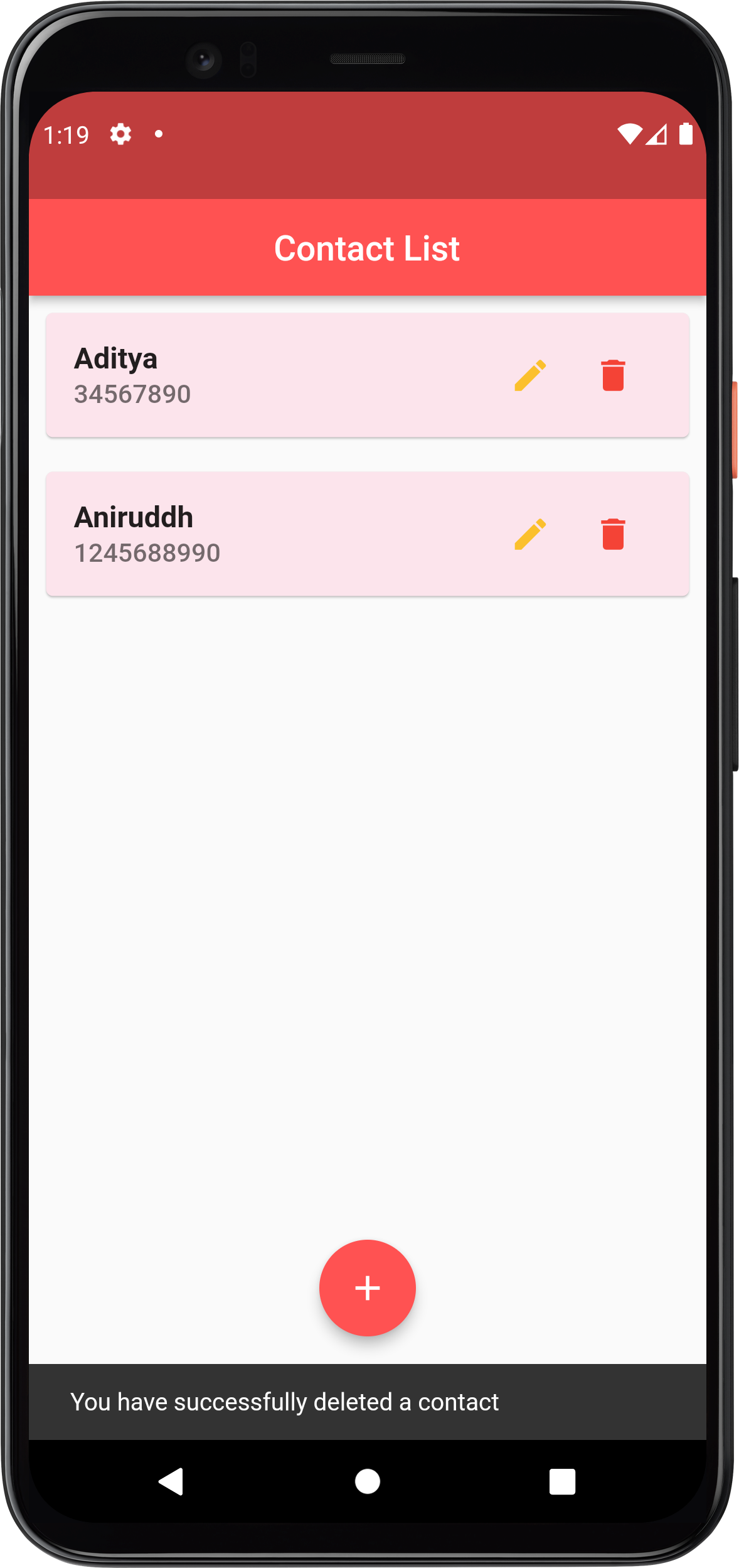