Code is copied!
How To Create a Card UI Layout Using Flutter
In this tutorial, we will learn how to create beautiful and customizable card UI in Flutter. We will use the Container widget to create the card layout and other widgets like Image and Text to add images and text to the card. We will also learn how to customize the card's appearance by adding borders and changing its shape. By the end of this tutorial, you will have a good understanding of how to create beautiful and customizable card UI in Flutter.
Before moving towards our "main.dart" file we first have to set two things :
1. We have to make some changes to our "pubspec.yaml" file. Follow the steps given below :
(a) At first you have to uncomment the following lines in your "pubspec.yaml" file as shown below :
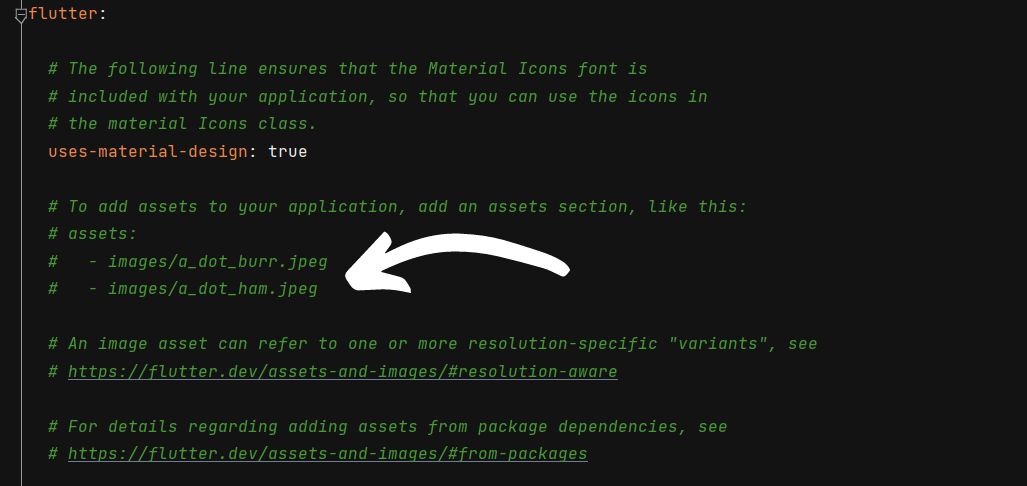
(b) Add your assets path inside your "pubspec.yaml" file as shown below :
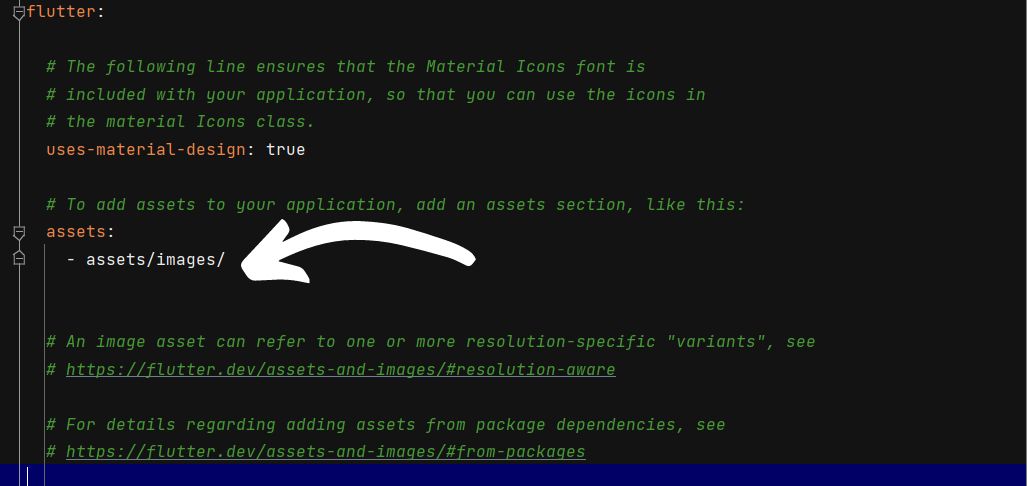
You can create only images folder rather than creating "assets/images" as per your choice. You just have to specify the path correctly.
(c) Then click on "Pub get" at the top right corner of your screen as shown below :
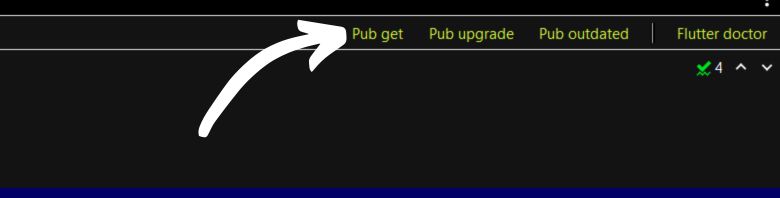
2. We have to make the same folder structure inside our root folder as we have specified in "pubspec.yaml" file and add your images inside "images" folder (You can add images of your own choice) as shown below :
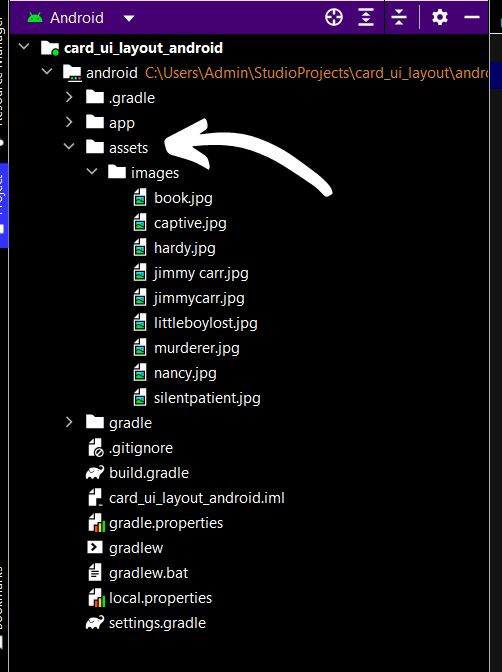
Source Code for the main.dart file
Add the following Code inside your main.dart file :
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.white,
body: SingleChildScrollView(
child: Column(
children: [
Container(
height: 230,
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
bottomRight: Radius.circular(50),
),
color: Colors.deepPurple,
),
child: Stack(
children: [
Positioned(
top:80,
left:0,
child: Container(
height: 100,
width:300,
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
topRight: Radius.circular(50),
bottomRight: Radius.circular(50),
),
color: Colors.white,
),
),
),
Positioned(
top:115,
left:20,
child:Text('Your Books',
style: TextStyle(
color: Colors.deepPurple,
fontSize: 25,
fontWeight: FontWeight.bold
)
)
)
],
),
),
SizedBox(
height: 20,
),
Wrap(
direction: Axis.horizontal,
children: [
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/nancy.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Nancy Drew',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Unseen Mystry',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
SizedBox(
height: 200,
width: 20,
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/hardy.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Hardy Boys',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Thriller',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/jimmycarr.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Jimmy Carr',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Comedy',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
SizedBox(
height: 200,
width: 20,
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/littleboylost.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Little Boy Lost',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Crime',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/silentpatient.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Silent Patient',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Horror',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
SizedBox(
height: 200,
width: 20,
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/murderer.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Murderer',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Horror',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
]
)
]
),
),
),
debugShowCheckedModeBanner: false,
);
}
}
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.white,
body: SingleChildScrollView(
child: Column(
children: [
Container(
height: 230,
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
bottomRight: Radius.circular(50),
),
color: Colors.deepPurple,
),
child: Stack(
children: [
Positioned(
top:80,
left:0,
child: Container(
height: 100,
width:300,
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
topRight: Radius.circular(50),
bottomRight: Radius.circular(50),
),
color: Colors.white,
),
),
),
Positioned(
top:115,
left:20,
child:Text('Your Books',
style: TextStyle(
color: Colors.deepPurple,
fontSize: 25,
fontWeight: FontWeight.bold
)
)
)
],
),
),
SizedBox(
height: 20,
),
Wrap(
direction: Axis.horizontal,
children: [
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/nancy.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Nancy Drew',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Unseen Mystry',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
SizedBox(
height: 200,
width: 20,
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/hardy.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Hardy Boys',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Thriller',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/jimmycarr.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Jimmy Carr',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Comedy',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
SizedBox(
height: 200,
width: 20,
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/littleboylost.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Little Boy Lost',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Crime',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/silentpatient.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Silent Patient',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Horror',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
SizedBox(
height: 200,
width: 20,
),
Container(
width: 170,
height: 180,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow:[
BoxShadow(
color: Colors.grey,
spreadRadius: 3,
blurRadius: 10,
offset: Offset(0,3),
)
]
),
child: Padding(
padding: EdgeInsets.symmetric(
horizontal: 10
),
child: Column(
children: [
Container(
child: Image.asset("assets/images/murderer.jpg",
height: 100,
width: 200,
fit: BoxFit.fitWidth,
),
height: 120,
),
Text(
'Murderer',
style:TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold
)
),
SizedBox(
height: 4,
),
Text(
'Horror',
style:TextStyle(
fontSize: 15,
)
),
],
),
),
),
]
)
]
),
),
),
debugShowCheckedModeBanner: false,
);
}
}