Code is copied!
How To Create a Rock Paper Scissors Game App Using Flutter
In this project, we're excited to present our "Rock Paper Scissors Game" app, developed using Flutter. Rock paper scissors is an intransitive hand game, usually played between two people, in which each player simultaneously forms one of three shapes with an outstretched hand. These shapes are "rock", "paper", and "scissors". Our project will guide you through the creation of this engaging Rock Paper Scissors Game app using Flutter. You'll learn how to build an intuitive and responsive user interface. Let's start...
Source Code for the main.dart file
Add the following Code inside your main.dart file :
import 'package:flutter/material.dart';
import 'game_page.dart';
import 'home_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
initialRoute: '/',
routes: {
'/': (context) => const HomePage(),
'/game': (context) => const GamePage(),
},
);
}
}
import 'package:flutter/material.dart';
import 'game_page.dart';
import 'home_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
initialRoute: '/',
routes: {
'/': (context) => const HomePage(),
'/game': (context) => const GamePage(),
},
);
}
}
Source Code for the home_page.dart file
Add the following Code inside your home_page.dart file :
import 'package:flutter/material.dart';
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
backgroundColor: Colors.deepPurpleAccent,
elevation: 0,
centerTitle: true,
title: Text('Rock Paper Scissors',style: TextStyle(
fontSize: 25,
fontWeight: FontWeight.w600
),),
),
backgroundColor: Colors.deepPurpleAccent,
body: SafeArea(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Expanded(flex: 12, child: Image.asset('images/background.png')),
Padding(
padding: const EdgeInsets.all(15.0),
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.yellow,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0)
)
),
onPressed: (){
Navigator.pushNamed(context, '/game');
},
child: Padding(
padding: const EdgeInsets.all(15.0),
child: Text(
'START',
style: TextStyle(
color: Colors.black87,
fontWeight: FontWeight.bold,
letterSpacing: 2,
fontSize: 18,
),
),
),
),
),
SizedBox(
height: 10,
)
],
),
),
);
}
}
import 'package:flutter/material.dart';
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
backgroundColor: Colors.deepPurpleAccent,
elevation: 0,
centerTitle: true,
title: Text('Rock Paper Scissors',style: TextStyle(
fontSize: 25,
fontWeight: FontWeight.w600
),),
),
backgroundColor: Colors.deepPurpleAccent,
body: SafeArea(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Expanded(flex: 12, child: Image.asset('images/background.png')),
Padding(
padding: const EdgeInsets.all(15.0),
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.yellow,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0)
)
),
onPressed: (){
Navigator.pushNamed(context, '/game');
},
child: Padding(
padding: const EdgeInsets.all(15.0),
child: Text(
'START',
style: TextStyle(
color: Colors.black87,
fontWeight: FontWeight.bold,
letterSpacing: 2,
fontSize: 18,
),
),
),
),
),
SizedBox(
height: 10,
)
],
),
),
);
}
}
Source Code for the game_page.dart file
Add the following Code inside your game_page.dart file :
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:rock/home_page.dart';
class GamePage extends StatefulWidget {
const GamePage({super.key});
@override
State<GamePage> createState() => _GamePageState();
}
class _GamePageState extends State<GamePage> {
List<String> images=[
"images/rock.png",
"images/paper.png",
"images/scissors.png"
];
int compOpt=0;
final Random random = Random();
int decidewin(int index)
{
compOpt = random.nextInt(3);
switch(index)
{
// if User Opt Rock
case 0:
if(compOpt==index)
return -1;
else if(compOpt==1)
return 1;
else
return 0;
// If user opt for Paper
case 1:
if(compOpt==index)
return -1;
else if(compOpt==0)
return 0;
else
return 1;
// If user opt for Scissors
case 2:
if(compOpt==index)
return -1;
else if(compOpt==1)
return 0;
else
return 1;
default:
return 0;
}
}
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
elevation: 0,
automaticallyImplyLeading: false,
centerTitle: true,
backgroundColor: Colors.deepPurpleAccent,
title: Text('YOUR CHOICE',
style: TextStyle(
fontSize: 30,
fontWeight: FontWeight.w900,
letterSpacing: 2,
),),
),
backgroundColor: Colors.deepPurpleAccent,
body: Column(
children: [
SizedBox(
height: 100,
),
Container(
decoration: BoxDecoration(
color: Colors.yellow[200],
borderRadius: BorderRadius.circular(20)
),
child: Padding(
padding: const EdgeInsets.all(10.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Game Rules :".toUpperCase(),
style: TextStyle(
fontSize: 20,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text(
"paper win rock".toUpperCase(),
style: TextStyle(
fontSize: 17,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text(
"rock win scissors ".toUpperCase(),
style: TextStyle(
fontSize: 17,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text(
"scissors win paper".toUpperCase(),
style: TextStyle(
fontSize: 17,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
),
],
),
),
),
SizedBox(
height: 100,
),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
for(int i =0;i< 3;i++)
GestureDetector(
onTap: (){
final winner = decidewin(i);
showDialog(
context: context,
barrierDismissible: false,
builder: (BuildContext context)
{return AlertDialog(
title: Text("Result"),
content: SingleChildScrollView(
child: ListBody(
children: [
Padding(
padding: const EdgeInsets.all(15.0),
child: Row(
children: [
Text("You Chose : "),
Image.asset(images[i],width: 50,)
],
),
),
Padding(
padding: const EdgeInsets.all(15.0),
child: Row(
children: [
Text("Comp Chose : "),
Image.asset(images[compOpt],width: 50,)
],
),
),
SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(15.0),
child: Text(winner==-1?"Urgh, it's a tie"
:winner==0?"Congratulations You Win !"
:"Sorry! You Lose"
),
)
],
),
),
actions: [
TextButton(
onPressed: (){
setState(() {
});
Navigator.of(context).pop();
},
child: Text("Play Again"),
)],
);},
);
},
child: Container(
height: 100,
width: 100,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(50)
),
child: Padding(
padding: const EdgeInsets.all(10.0),
child: Image.asset(images[i],
),
),
),
)
],
),
SizedBox(
height: 100,
),
Padding(
padding: const EdgeInsets.all(15.0),
child: SizedBox(
width: double.infinity,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.yellow,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0)
)
),
onPressed: (){
Navigator.pushNamed(context, '/');
},
child: Padding(
padding: const EdgeInsets.all(15.0),
child: Text(
'HOME',
style: TextStyle(
color: Colors.black87,
fontWeight: FontWeight.bold,
letterSpacing: 2,
fontSize: 18,
),
),
),
),
),
),
SizedBox(
height: 10,
)
],
),
);
}
}
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:rock/home_page.dart';
class GamePage extends StatefulWidget {
const GamePage({super.key});
@override
State<GamePage> createState() => _GamePageState();
}
class _GamePageState extends State<GamePage> {
List<String> images=[
"images/rock.png",
"images/paper.png",
"images/scissors.png"
];
int compOpt=0;
final Random random = Random();
int decidewin(int index)
{
compOpt = random.nextInt(3);
switch(index)
{
// if User Opt Rock
case 0:
if(compOpt==index)
return -1;
else if(compOpt==1)
return 1;
else
return 0;
// If user opt for Paper
case 1:
if(compOpt==index)
return -1;
else if(compOpt==0)
return 0;
else
return 1;
// If user opt for Scissors
case 2:
if(compOpt==index)
return -1;
else if(compOpt==1)
return 0;
else
return 1;
default:
return 0;
}
}
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(
elevation: 0,
automaticallyImplyLeading: false,
centerTitle: true,
backgroundColor: Colors.deepPurpleAccent,
title: Text('YOUR CHOICE',
style: TextStyle(
fontSize: 30,
fontWeight: FontWeight.w900,
letterSpacing: 2,
),),
),
backgroundColor: Colors.deepPurpleAccent,
body: Column(
children: [
SizedBox(
height: 100,
),
Container(
decoration: BoxDecoration(
color: Colors.yellow[200],
borderRadius: BorderRadius.circular(20)
),
child: Padding(
padding: const EdgeInsets.all(10.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
"Game Rules :".toUpperCase(),
style: TextStyle(
fontSize: 20,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text(
"paper win rock".toUpperCase(),
style: TextStyle(
fontSize: 17,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text(
"rock win scissors ".toUpperCase(),
style: TextStyle(
fontSize: 17,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text(
"scissors win paper".toUpperCase(),
style: TextStyle(
fontSize: 17,
color: Colors.black,
fontWeight: FontWeight.w500
),
textAlign: TextAlign.center,
),
),
],
),
),
),
SizedBox(
height: 100,
),
Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
for(int i =0;i< 3;i++)
GestureDetector(
onTap: (){
final winner = decidewin(i);
showDialog(
context: context,
barrierDismissible: false,
builder: (BuildContext context)
{return AlertDialog(
title: Text("Result"),
content: SingleChildScrollView(
child: ListBody(
children: [
Padding(
padding: const EdgeInsets.all(15.0),
child: Row(
children: [
Text("You Chose : "),
Image.asset(images[i],width: 50,)
],
),
),
Padding(
padding: const EdgeInsets.all(15.0),
child: Row(
children: [
Text("Comp Chose : "),
Image.asset(images[compOpt],width: 50,)
],
),
),
SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(15.0),
child: Text(winner==-1?"Urgh, it's a tie"
:winner==0?"Congratulations You Win !"
:"Sorry! You Lose"
),
)
],
),
),
actions: [
TextButton(
onPressed: (){
setState(() {
});
Navigator.of(context).pop();
},
child: Text("Play Again"),
)],
);},
);
},
child: Container(
height: 100,
width: 100,
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(50)
),
child: Padding(
padding: const EdgeInsets.all(10.0),
child: Image.asset(images[i],
),
),
),
)
],
),
SizedBox(
height: 100,
),
Padding(
padding: const EdgeInsets.all(15.0),
child: SizedBox(
width: double.infinity,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.yellow,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0)
)
),
onPressed: (){
Navigator.pushNamed(context, '/');
},
child: Padding(
padding: const EdgeInsets.all(15.0),
child: Text(
'HOME',
style: TextStyle(
color: Colors.black87,
fontWeight: FontWeight.bold,
letterSpacing: 2,
fontSize: 18,
),
),
),
),
),
),
SizedBox(
height: 10,
)
],
),
);
}
}
The Output is shown below :
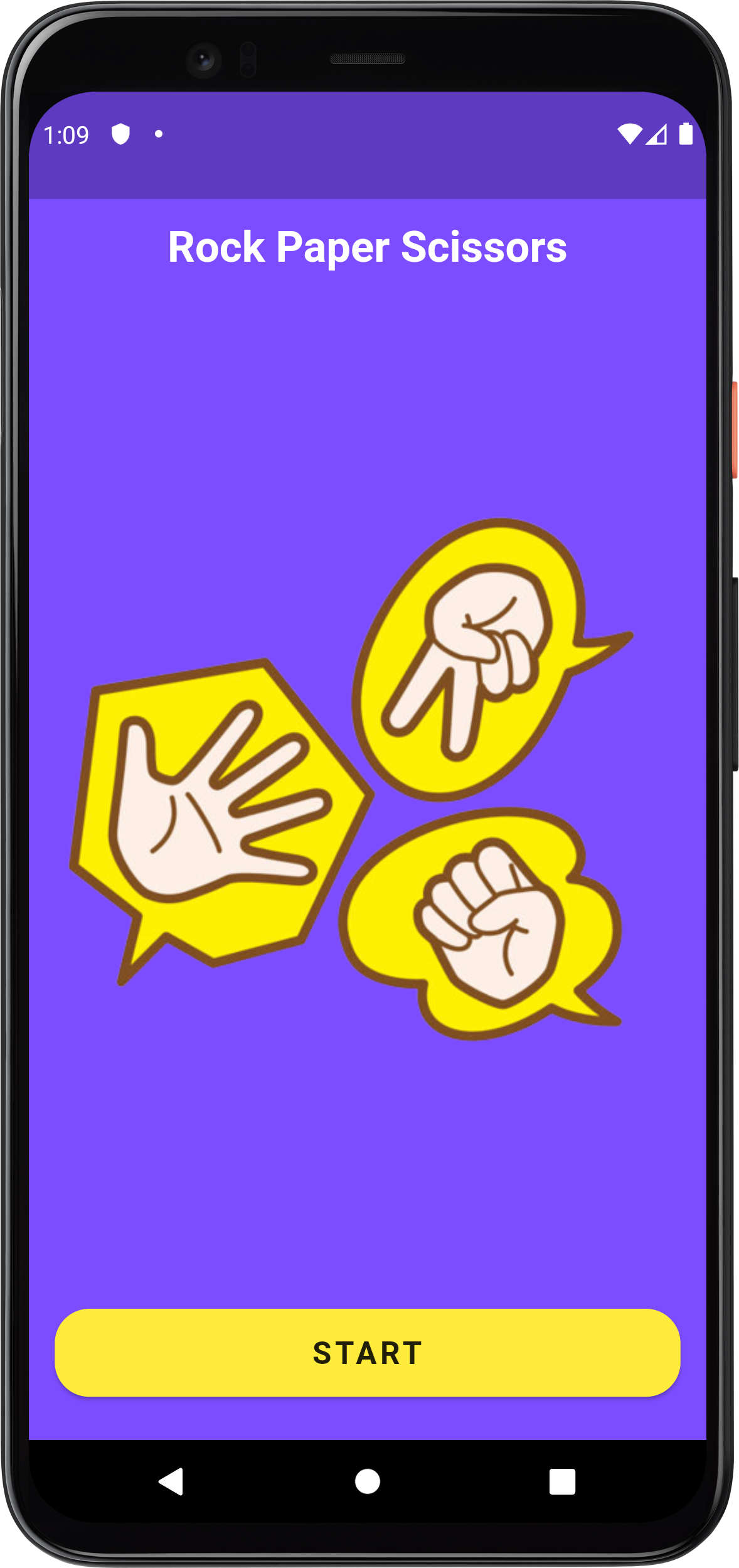
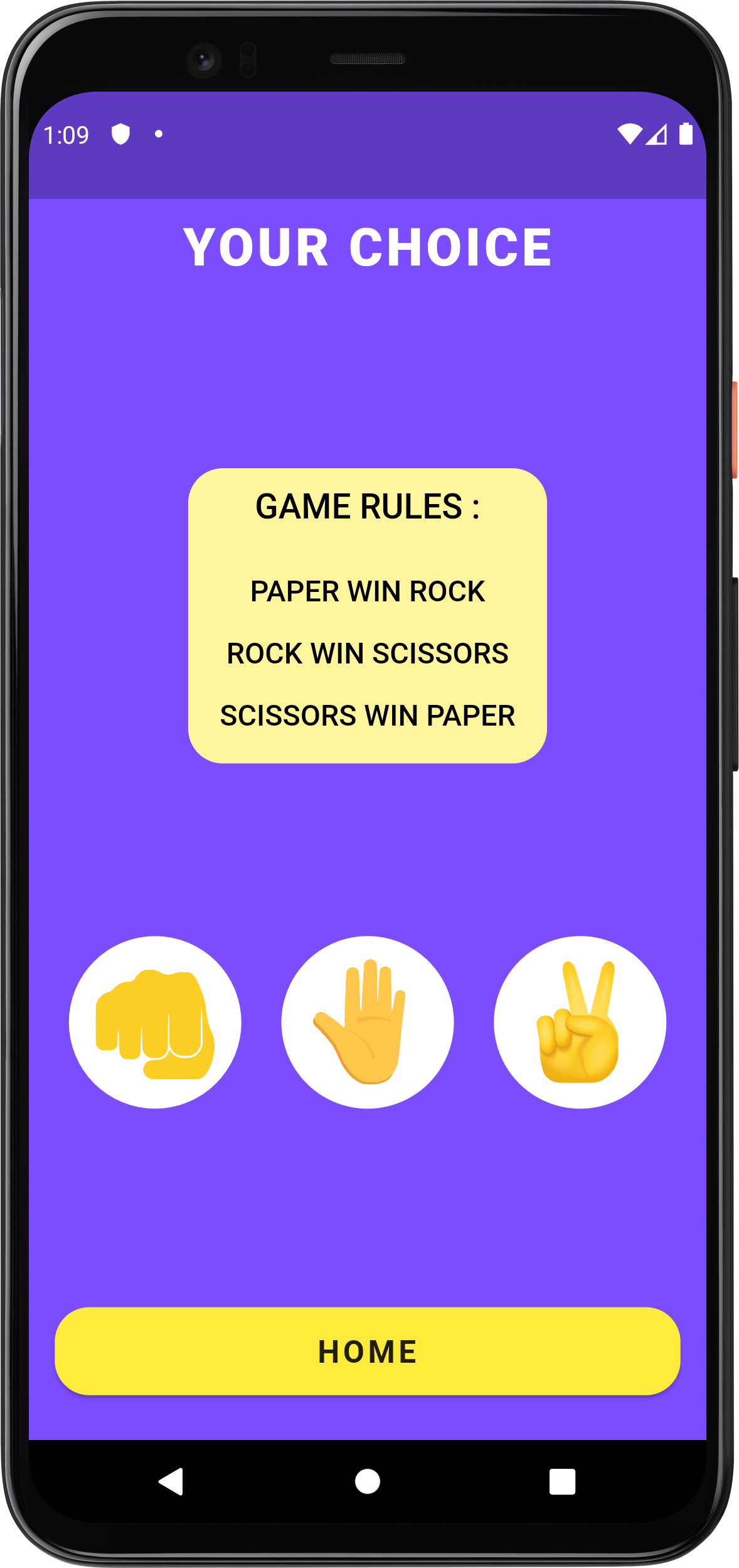
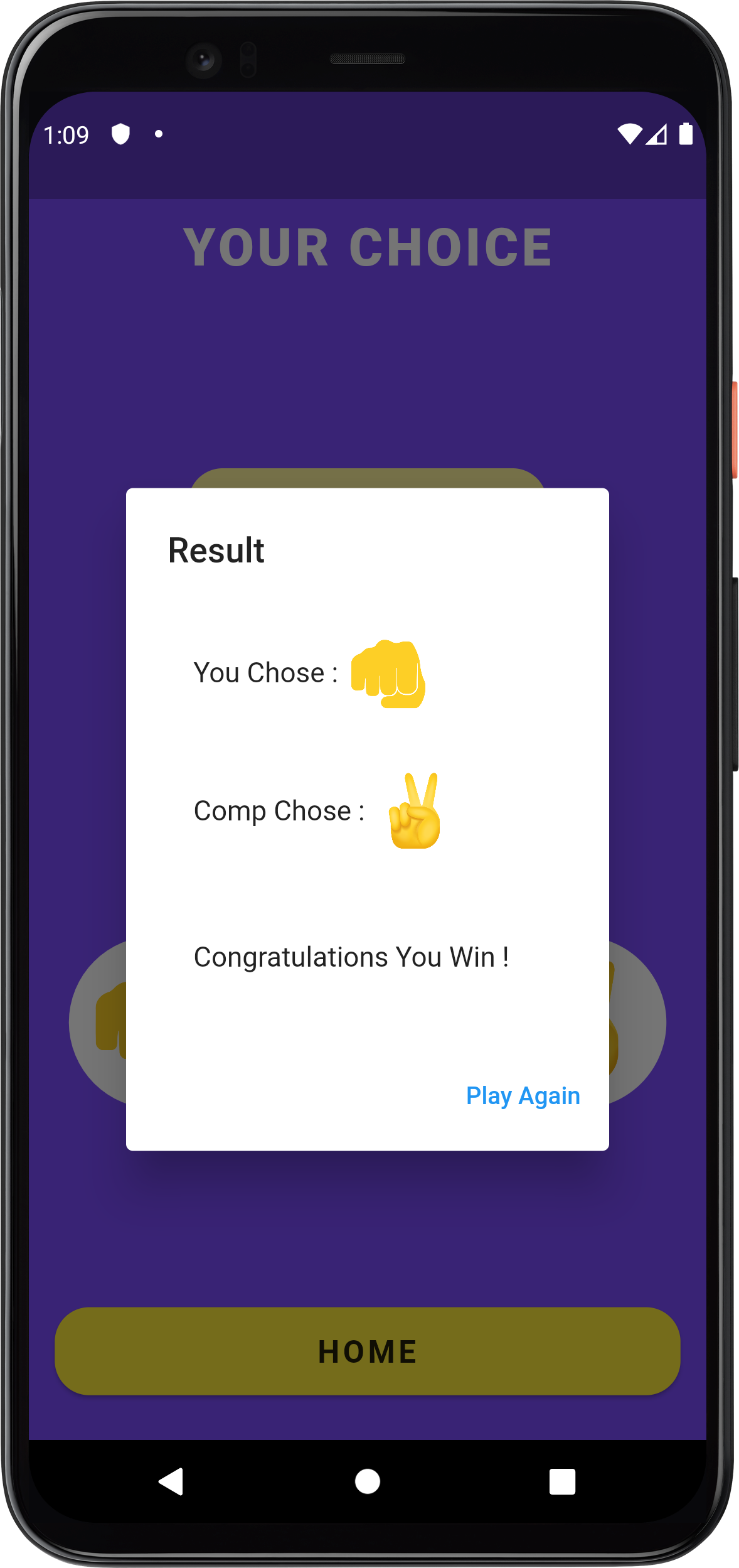
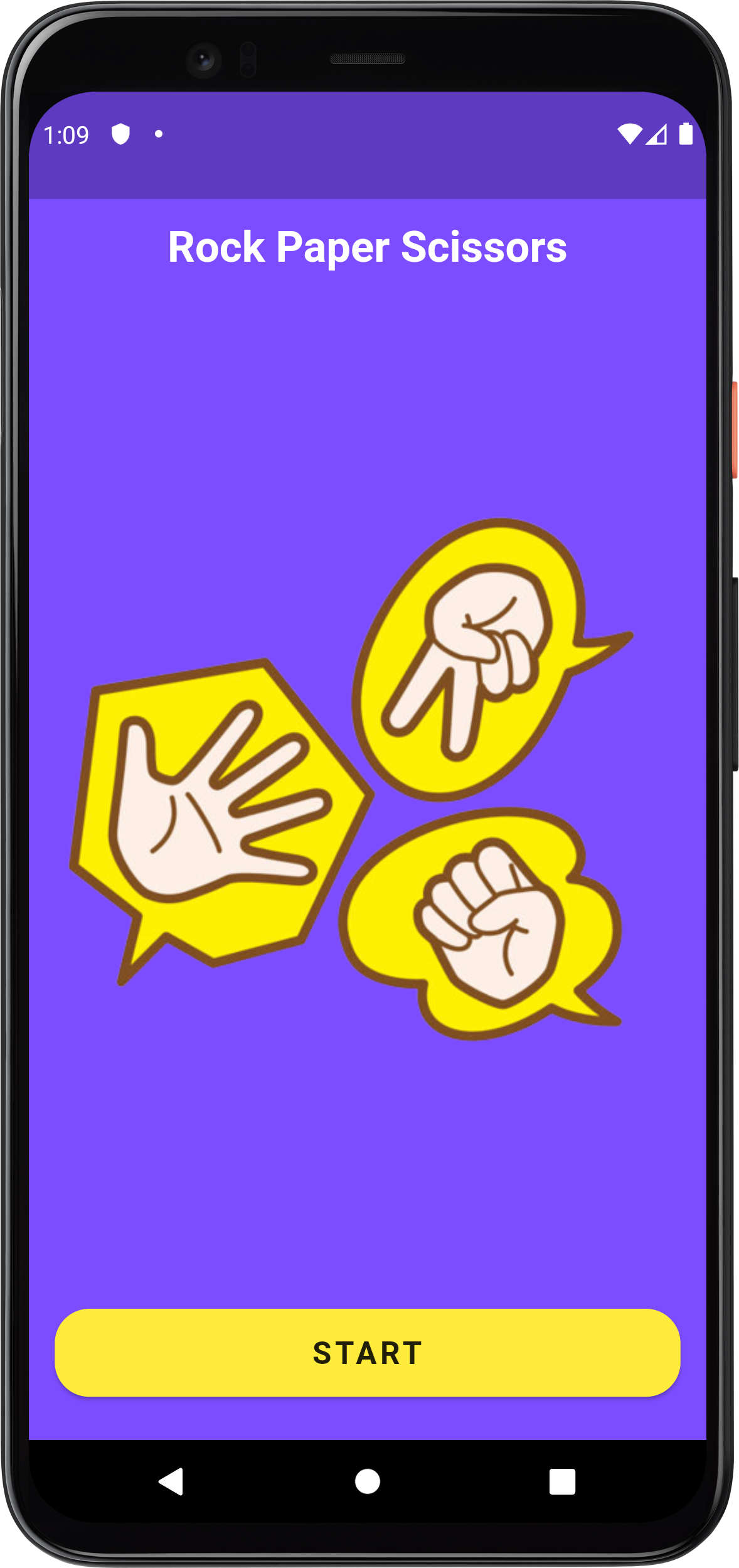
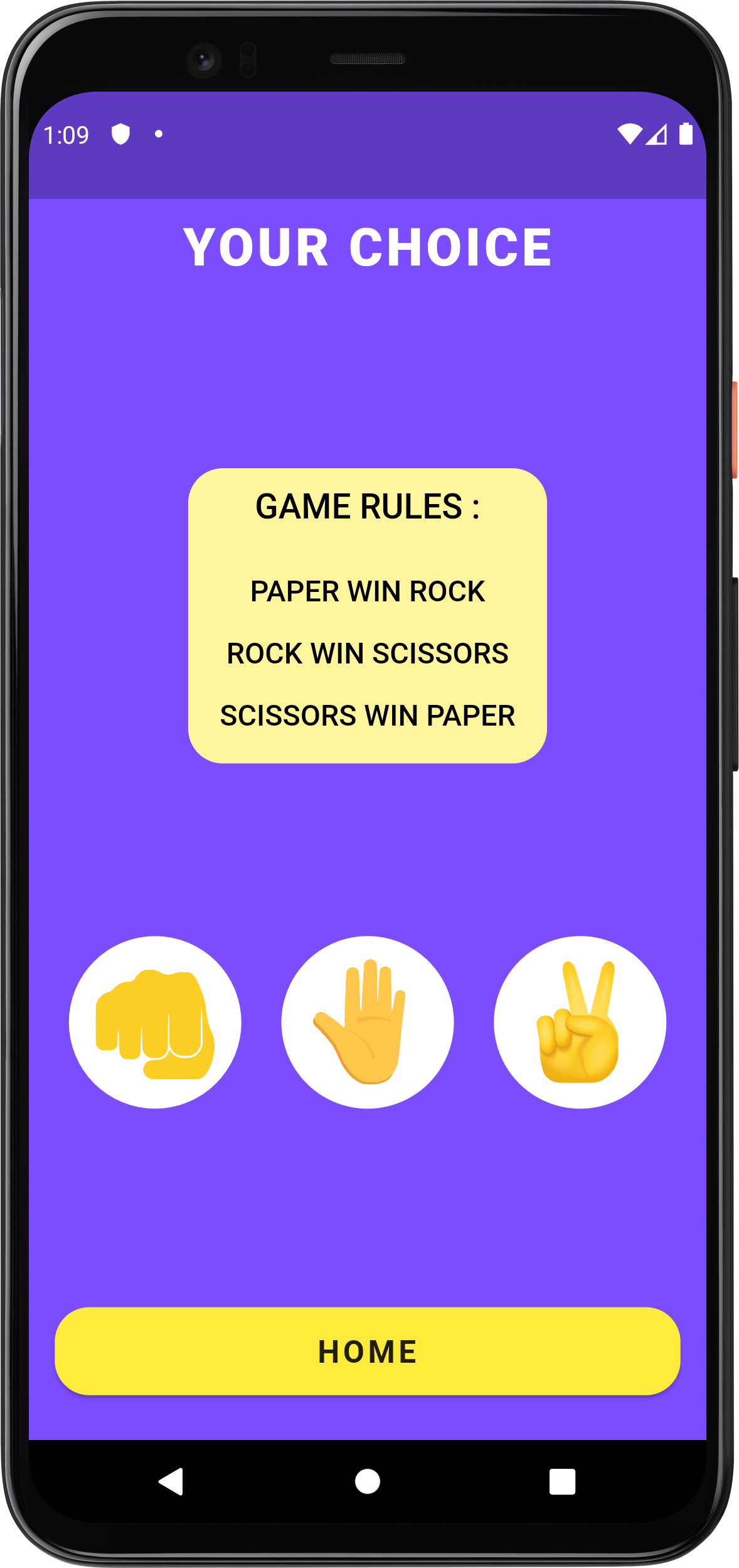
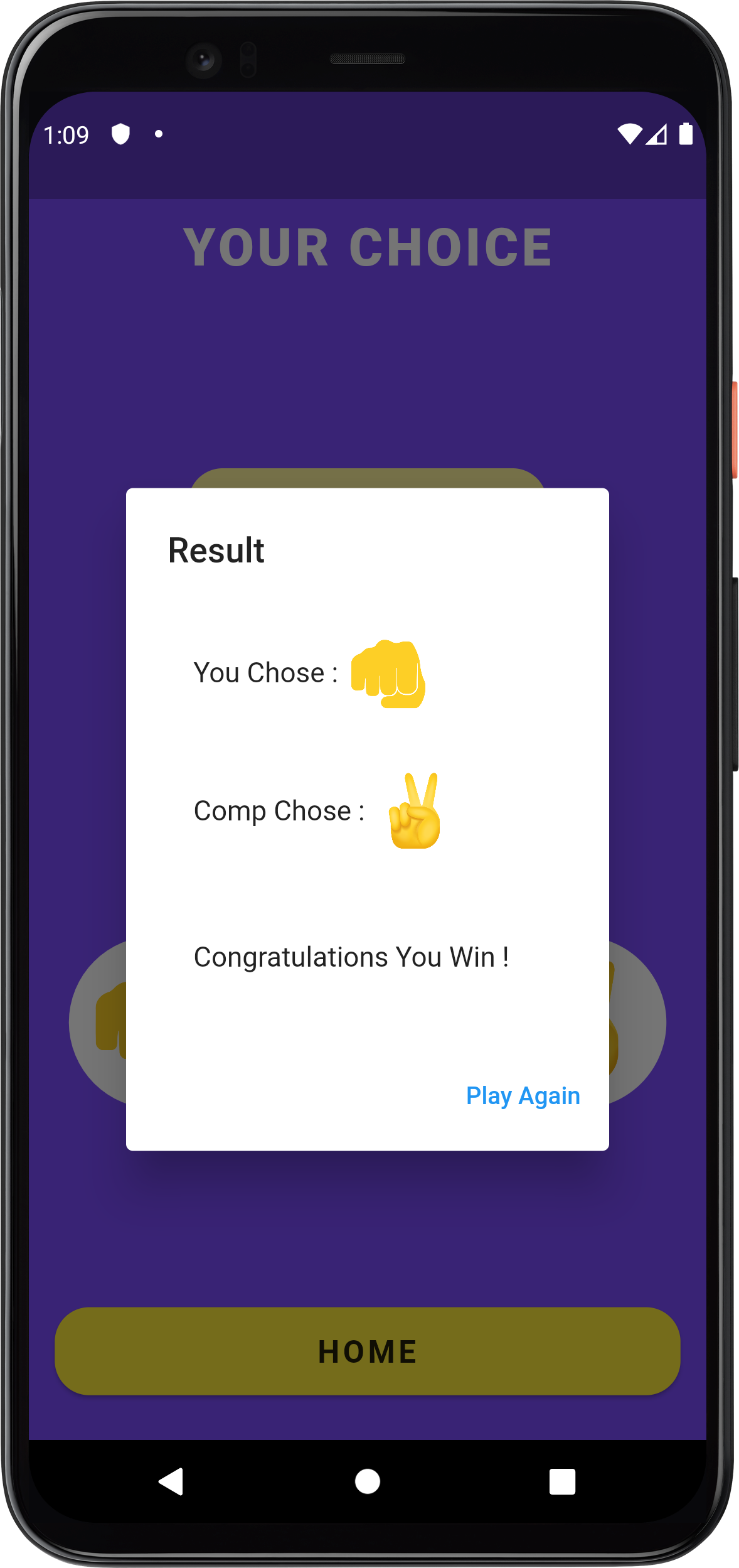