Code is copied!
How To Create a Fingerprint Authentication App Using Flutter
In this project, we're excited to present our "Fingerprint authentication" app, developed using Flutter. Our project will guide you through the creation of this engaging Otp authentication app using Flutter. You'll learn how to build an intuitive and responsive user interface. Let's start...
Source Code for the main.dart file
Add the following Code inside your main.dart file :
import 'package:flutter/material.dart';
import 'home_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
import 'package:flutter/material.dart';
import 'home_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
Source Code for the home_page.dart file
Add the following Code inside your home_page.dart file :
import 'package:flutter/material.dart';
import 'package:local_auth/local_auth.dart';
import 'done_page.dart';
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final LocalAuthentication auth = LocalAuthentication();
checkAuth() async{
bool isAvailable;
isAvailable = await auth.canCheckBiometrics;
print(isAvailable);
if(isAvailable)
{
bool result = await auth.authenticate(
localizedReason: 'Scan your fingerprint to proceed',
options: AuthenticationOptions(
biometricOnly: true
)
);
if(result)
{
Navigator.push(
context, MaterialPageRoute(builder: (context) => DonePage()));
}
else
{
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('Error Occured'),
content: Text('Permission Denied'), // Format total price with two decimal places
actions: [
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('Close'),
),
],
);
},
);
}
}
else
{
print("No biometric detected");
}
}
@override
Widget build(BuildContext context) {
return
Scaffold(
// backgroundColor: Color(0xFF0B0B45),
backgroundColor: Colors.indigo[900],
body: Center(
child: Column(
children: [
SizedBox(
height: 150,
),
Text('Login',
style: TextStyle(
color: Colors.white,
fontSize: 45,
fontWeight: FontWeight.w700
),),
SizedBox(
height: 60,
),
Padding(
padding: EdgeInsets.fromLTRB(30, 0, 10, 0),
child:
Text('Use your fingerprint to authenticate yourself ',
style: TextStyle(
color: Colors.white,
fontSize: 16,
),),
),
Text('before using the app ',
style: TextStyle(
color: Colors.white,
fontSize: 16,
),),
SizedBox(
height: 100,
),
GestureDetector(
onTap: checkAuth,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(60),
border: Border.all(
color: Colors.green,
width: 3,
)
),
child: Icon(
Icons.fingerprint_rounded,
size: 120,
color:Colors.green
),
),
),
],
),
),
);
}
}
import 'package:flutter/material.dart';
import 'package:local_auth/local_auth.dart';
import 'done_page.dart';
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final LocalAuthentication auth = LocalAuthentication();
checkAuth() async{
bool isAvailable;
isAvailable = await auth.canCheckBiometrics;
print(isAvailable);
if(isAvailable)
{
bool result = await auth.authenticate(
localizedReason: 'Scan your fingerprint to proceed',
options: AuthenticationOptions(
biometricOnly: true
)
);
if(result)
{
Navigator.push(
context, MaterialPageRoute(builder: (context) => DonePage()));
}
else
{
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('Error Occured'),
content: Text('Permission Denied'), // Format total price with two decimal places
actions: [
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('Close'),
),
],
);
},
);
}
}
else
{
print("No biometric detected");
}
}
@override
Widget build(BuildContext context) {
return
Scaffold(
// backgroundColor: Color(0xFF0B0B45),
backgroundColor: Colors.indigo[900],
body: Center(
child: Column(
children: [
SizedBox(
height: 150,
),
Text('Login',
style: TextStyle(
color: Colors.white,
fontSize: 45,
fontWeight: FontWeight.w700
),),
SizedBox(
height: 60,
),
Padding(
padding: EdgeInsets.fromLTRB(30, 0, 10, 0),
child:
Text('Use your fingerprint to authenticate yourself ',
style: TextStyle(
color: Colors.white,
fontSize: 16,
),),
),
Text('before using the app ',
style: TextStyle(
color: Colors.white,
fontSize: 16,
),),
SizedBox(
height: 100,
),
GestureDetector(
onTap: checkAuth,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(60),
border: Border.all(
color: Colors.green,
width: 3,
)
),
child: Icon(
Icons.fingerprint_rounded,
size: 120,
color:Colors.green
),
),
),
],
),
),
);
}
}
Source Code for the done_page.dart file
Add the following Code inside your done_page.dart file :
import 'package:flutter/material.dart';
class DonePage extends StatefulWidget {
const DonePage({super.key});
@override
State<DonePage> createState() => _DonePageState();
}
class _DonePageState extends State<DonePage> {
@override
Widget build(BuildContext context) {
return
Scaffold(
backgroundColor: Colors.indigo[900],
appBar: AppBar(
automaticallyImplyLeading: false,
backgroundColor: Colors.indigo[900],
elevation: 0,),
body: Center(
child: SingleChildScrollView(
scrollDirection: Axis.vertical,
child: Column(
children: [
Container(
child: Image.asset('images/verify.webp',
height: 300,),
),
SizedBox(
height: 40,
),
Text('Successfully Verified',
style: TextStyle(
fontSize: 30,
fontWeight: FontWeight.bold,
color: Colors.green
),)
],
),
),
),
);
}
}
import 'package:flutter/material.dart';
class DonePage extends StatefulWidget {
const DonePage({super.key});
@override
State<DonePage> createState() => _DonePageState();
}
class _DonePageState extends State<DonePage> {
@override
Widget build(BuildContext context) {
return
Scaffold(
backgroundColor: Colors.indigo[900],
appBar: AppBar(
automaticallyImplyLeading: false,
backgroundColor: Colors.indigo[900],
elevation: 0,),
body: Center(
child: SingleChildScrollView(
scrollDirection: Axis.vertical,
child: Column(
children: [
Container(
child: Image.asset('images/verify.webp',
height: 300,),
),
SizedBox(
height: 40,
),
Text('Successfully Verified',
style: TextStyle(
fontSize: 30,
fontWeight: FontWeight.bold,
color: Colors.green
),)
],
),
),
),
);
}
}
The Output is shown below :
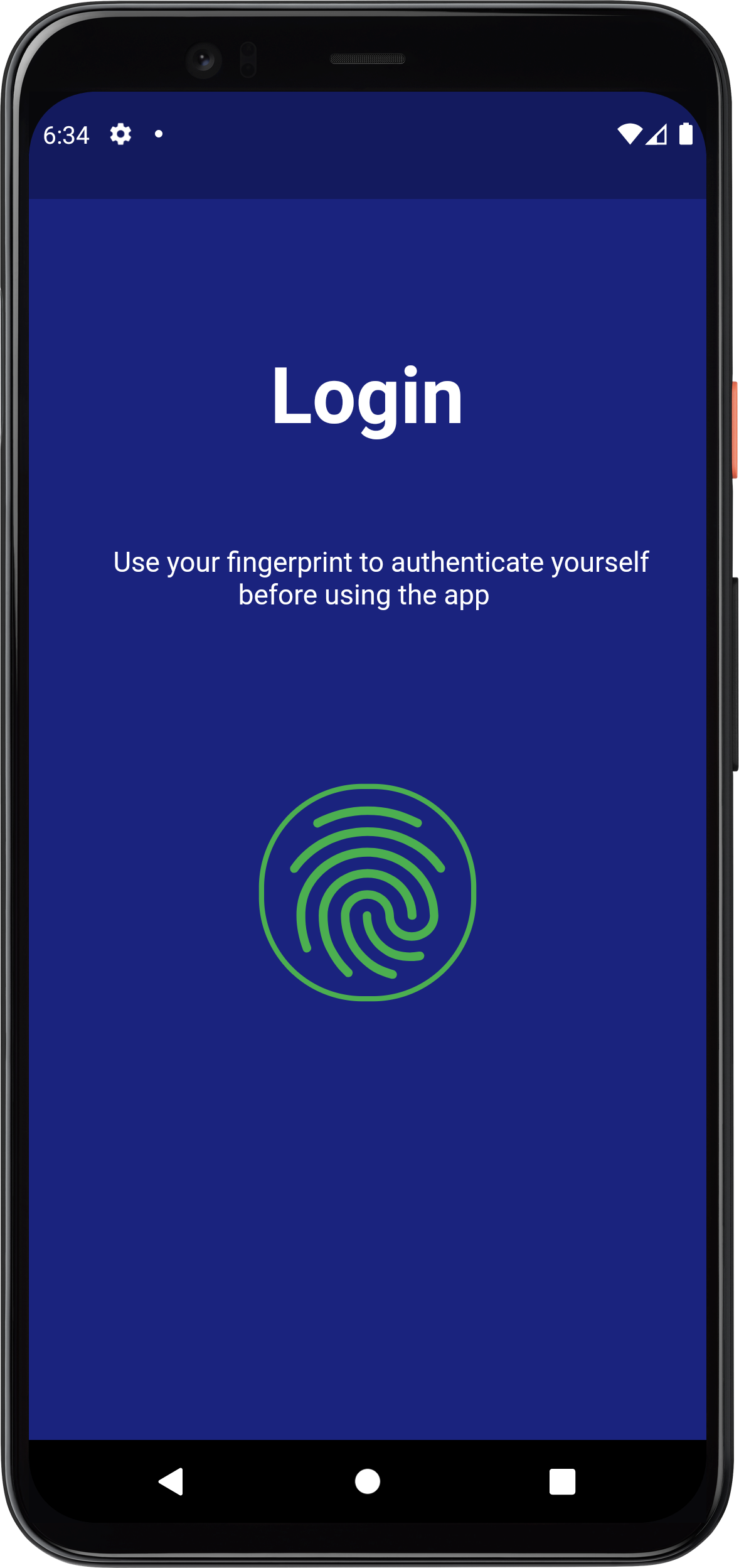
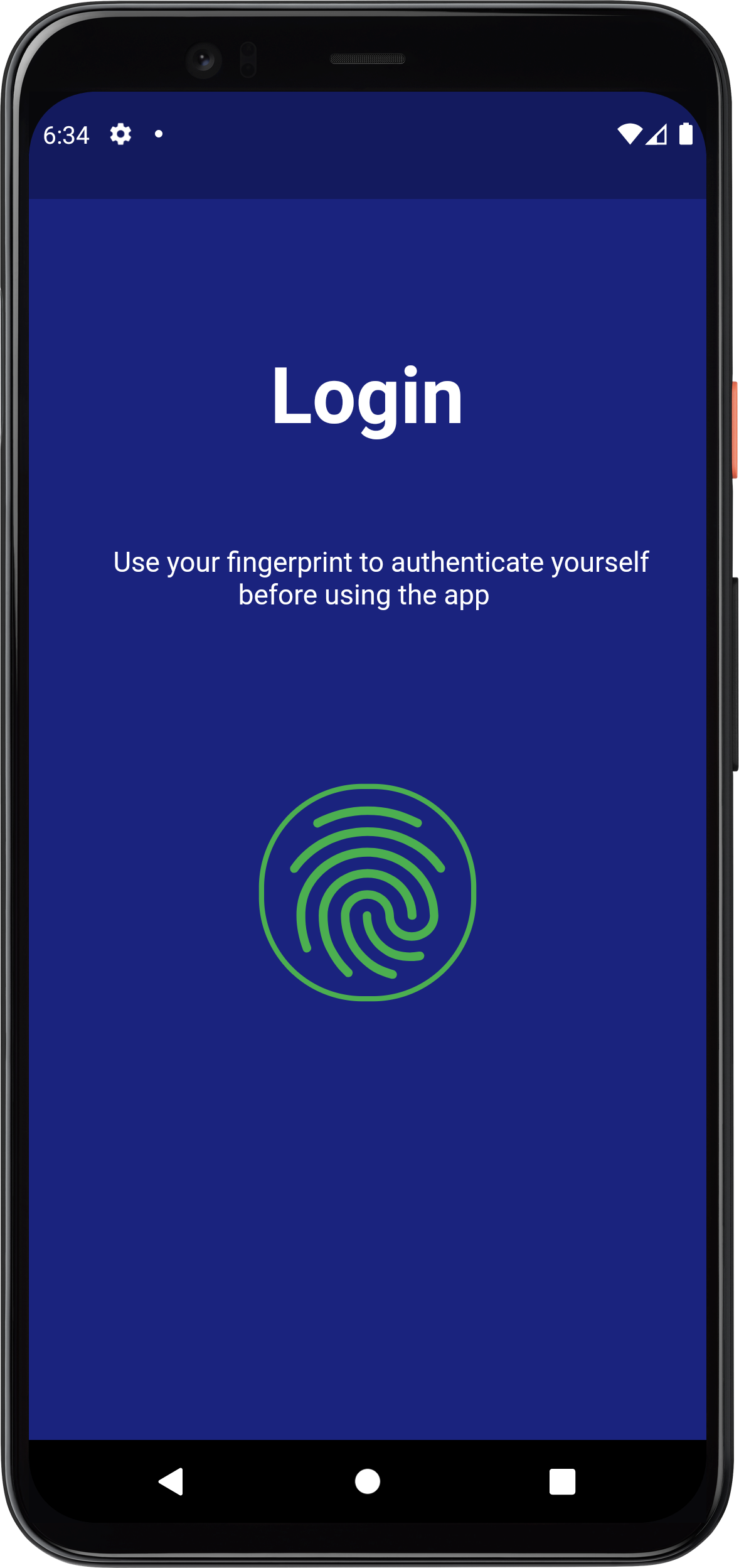