Class 9 ICSE - Java
Class 9th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 9th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Values and Datatype
Character Set
First step in learning any languages knowing its character set
How do we have learn English language?
English language consist of a set of characters set which is comprised of :
(a) Alphabets ⇛ (A-Z) and (a-z)
(b) Digits ⇛ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
(c) Special characters ⇛ !, @, %, *, $, ^, etc.
So, character set is defined as a set of characters used in any language for forming words sentences paragraphs etc.
Similarly,
Java is also programming language it also has a set of characters used in forming tokens, instructions, programs etc.
Character Set in Java :
- Alphabets : (A-Z) and (a-z)
- Digits : 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
- Special Characters : !, @, %, *, $, ^, etc.
- Escape sequences : \n, \t, \b, \a, \v, \", \' etc.
Note : In Java programming language characters are defined using Unicode character set
ASCII Character Set
- ASCII code stand for American standard code for information interchange
- In ASCII format we use alphanumeric numbers
- In this each character represented in 7 bits
- It consists of total 128 characters.
Note : We cannot represent all characters using ASCII code
Let us see the example given below :
Output :
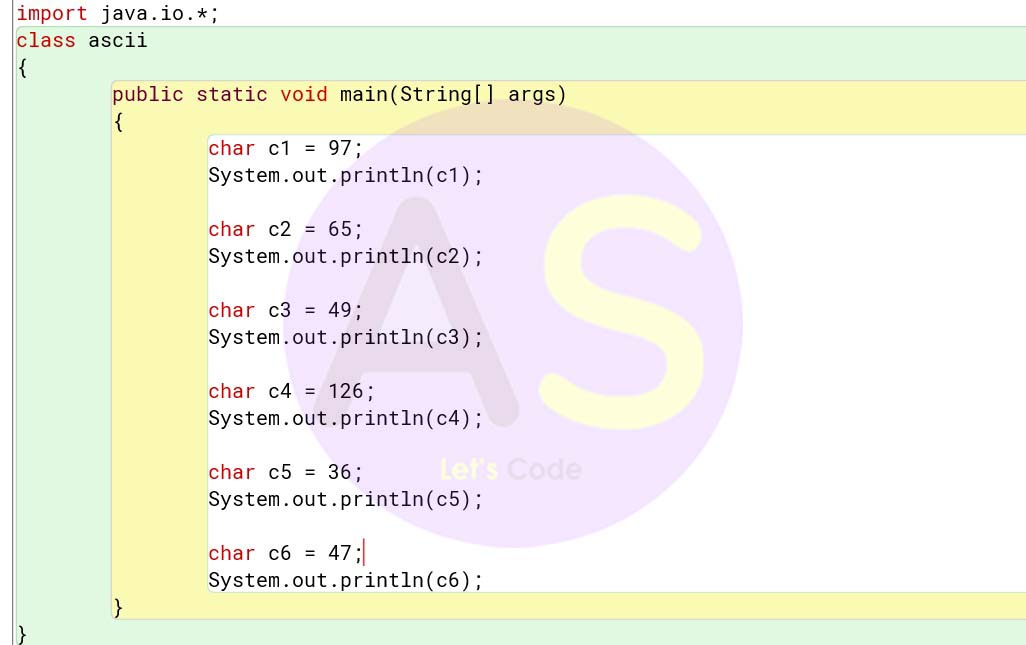
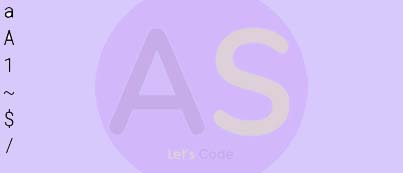
Unicode Character Set
- It was developed by Unicode Consortium
- It can represent all type of languages like Japanese, Hindi, French, English and German.
- In this each character represented in 16 bits
- It supports total of 65,000 characters
- It can represent all types of characters.
Note :
⇛ Unicode is not efficient due to storage and transmission
⇛ Java program mostly uses ASCII code and Unicode for variable names, comments etc.
Let us see the example given below :
Output :
⇛ Unicode is not efficient due to storage and transmission
⇛ Java program mostly uses ASCII code and Unicode for variable names, comments etc.
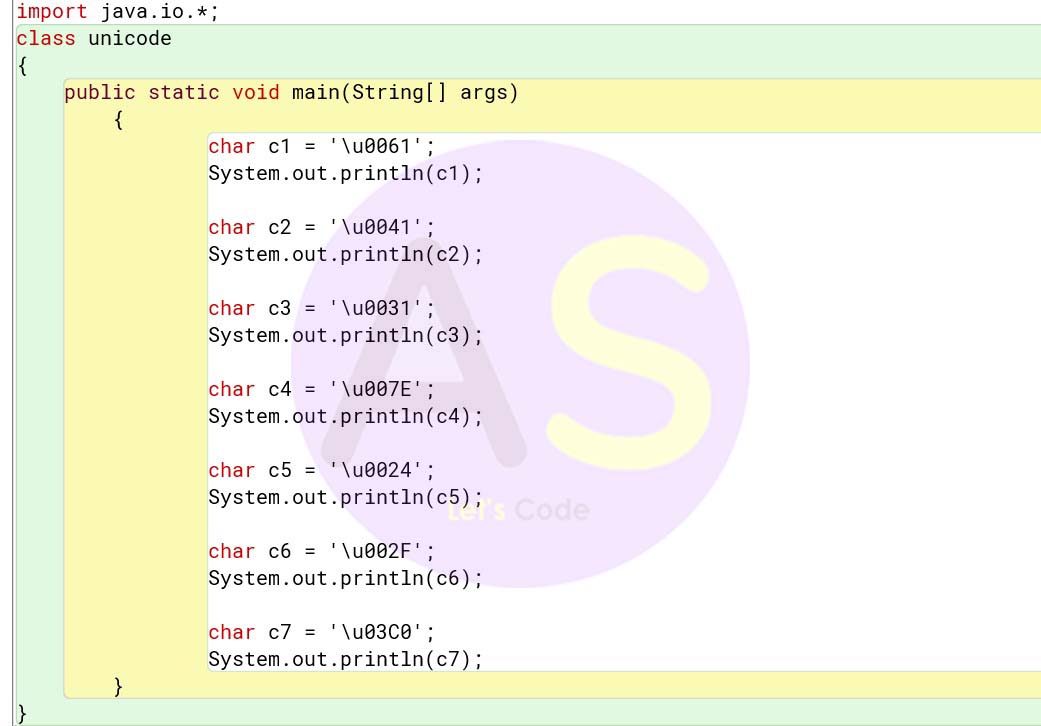
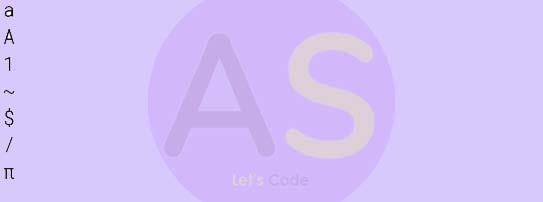
Tokens
A statement is composed of various components. Each smallest individual unit of a programming statement is known as Token.
The various types of tokens available in Java are :
⇛ literals
⇛ Identifiers
⇛ Keywords
⇛ Punctuators
⇛ Seperataors
⇛ Operators
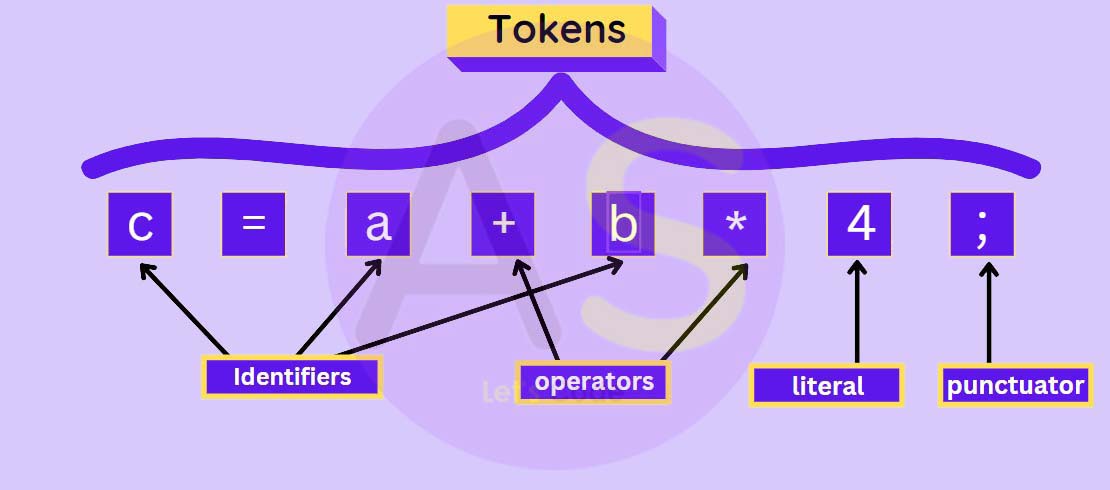
Literals
Literal are the constants in Java program ,which remains fixed (i.e. do not change) during the execution of the program. Such quantities are termed as literals or constants
Java uses the literals classified in the following ways:
Integer Literals :
The numbers which are represented without decimal point are called Integer Literals. These are the whole numbers having positive or negative values.
Ex - 14, 345, 8, 6392, -18, -391 etc.
Real Literals :
These are also called floating-point constants. They represent number with decimal points.
Ex- 24.6. 0.0072. -3.652. 1.0E-03 etc.
Character Literals :
The constants, which are alphanumeric in nature, are called character literals. All alphabets upper or lower case, digits, special characters can be termed as character literals.
Ex - 'A', 'd', '3', '*' etc.
A character literal represents a single character enclosed within single quotes.
String Literals :
String is a set of alphanumeric characters. A group of characters enclosed within a pair of opening and closing double quotes is known as string literal. e.g., "CRISTOPHER ALEXANDER", "Coder", "teacher" etc.
Boolean Literals :
Boolean constants are special literals. They represent true or false and can be applied in a java program to ensure that a given logical condition is satisfied or not. You must note that Boolean constants (i.e. true or false) are never enclosed within quotes. This characteristic makes Boolean constants different than string constants.
Null Literals :
This is another special purpose literal. It is represented as '\0'. It is applied in a java program as a string terminator to mark end of the string.
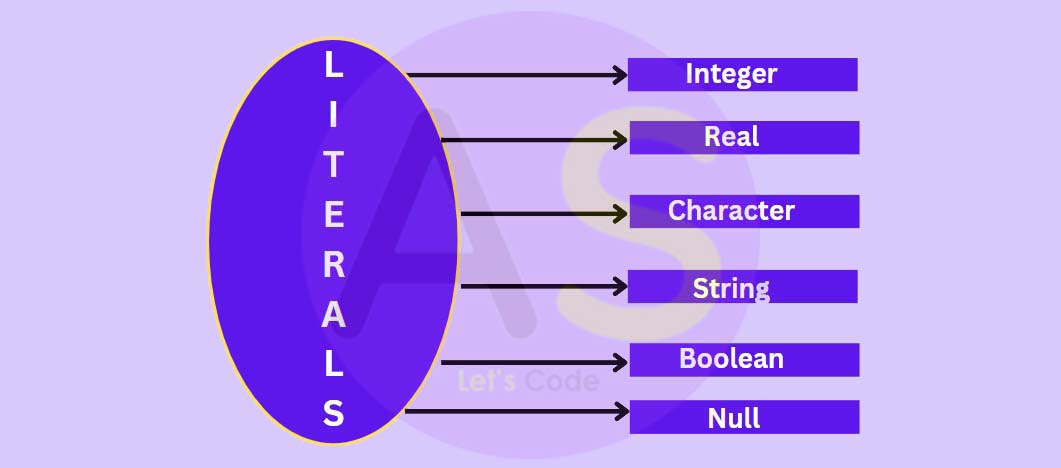
Identifiers
Identifiers are names for a class, a method, a constant, or a variable. Some words in Java are reserved and cannot be used as identifiers. Certain rules must be followed in java while we are defining an identifier otherwise the compiler will throw an error.
It is recommended to follow these rules while defining an identifier :
- An identifier can consist of any combination of letters, digits , the underscore character (_) and the dollar sign ($).
- It cannot begin with a digit.
- It may be of any length.
- Both uppercase and lower case letters can be used in naming an identifier
- Java is a case sensitive language which means that 2 identifier names that differ only in uppercase and lower case characters are considered to be different identifiers. Therefore, total, Total, ToTal and TOTAL are different identifiers
- Can identify and cannot be a keyword or a boolean literal or a null literal
Examples of some valid identifiers :
student1, my_login_details, CheckPalindrome, $10_20, _details, SIMPLE_INTREST, SUM, customerDetails.
student1, my_login_details, CheckPalindrome, $10_20, _details, SIMPLE_INTREST, SUM, customerDetails.
Keywords
Keywords, also known as reserved Words, are the words that convey a special meaning to the Java compiler. Java compiler reserves these keywords for its own use, and hence they cannot be used as names for variables or methods. Some of the Java keywords are listed below:
abstract
assert
boolean
break
byte
case
catch
char
class
const
continue
default
do
double
else
enum
extends
final
finally
float
for
goto
if
implements
import
instanceof
int
interface
long
native
new
package
private
protected
public
return
short
static
strictfp
super
switch
synchronized
this
throw
throws
transient
try
void
volatile
while
abstract | assert | boolean | break | byte |
case | catch | char | class | const |
continue | default | do | double | else |
enum | extends | final | finally | float |
for | goto | if | implements | import |
instanceof | int | interface | long | native |
new | package | private | protected | public |
return | short | static | strictfp | super |
switch | synchronized | this | throw | throws |
transient | try | void | volatile | while |
Punctuators
Punctuators are the punctuation signs used as special characters in Java. Some of the punctuators are ?(Question mark), ;(semi colon), .(Dot) etc.
Dot(.) is used to represent the scope of a function i.e. a function belonging to an object of a class.
Example : System.out.println(), java.io.* etc.
Semi colon(;) is used in a Java program as a statement terminator. It indicates the end of a statement. Any line continued after (;) is treated as the next line.
Example : int a = 5; System.out.println(a); are treated two separate statements in Java.
Seperators
They are the special characters in Java, which are used to separate the variables are the characters.
Example : Brackets (), Curly brackets {}, Square brackets [], etc.
Comma (,) In Java program is applied to separate multiple variables under same declaration.
Example : int a,b,c;
Brackets () are used to enclose any arithmetical or relational expression.
Curly brackets {} are applied to enclose a group of statement under compound statement.
Square brackets [] are used to include subscript or cell number of a dimensional array.
Operators
Operators are basically the symbols are tokens to perform arithmetical or logical operations to yield meaningful results. Basically there are 3 types of operators used in Java :
- Arithmetical Operators : +, -, /, * etc.
- Relational Operators : <, >, = =, !=, <= etc.
- Logical Operators : &&, ||, ! etc.
Let us see some of the operators in the table given below :
Unary Operators
+(unary)
-(unary)
Assignment Operator
=
Arithmetic Operators
+
-
*
/
%
Relational Operators
>
<
>=
<=
==
!=
Increment/Decrement Operators
++
--
Logical Operators
&&
||
!
Bitwise Operators
&
|
^
~
<<
>>
Conditional/Ternary Operator
?:
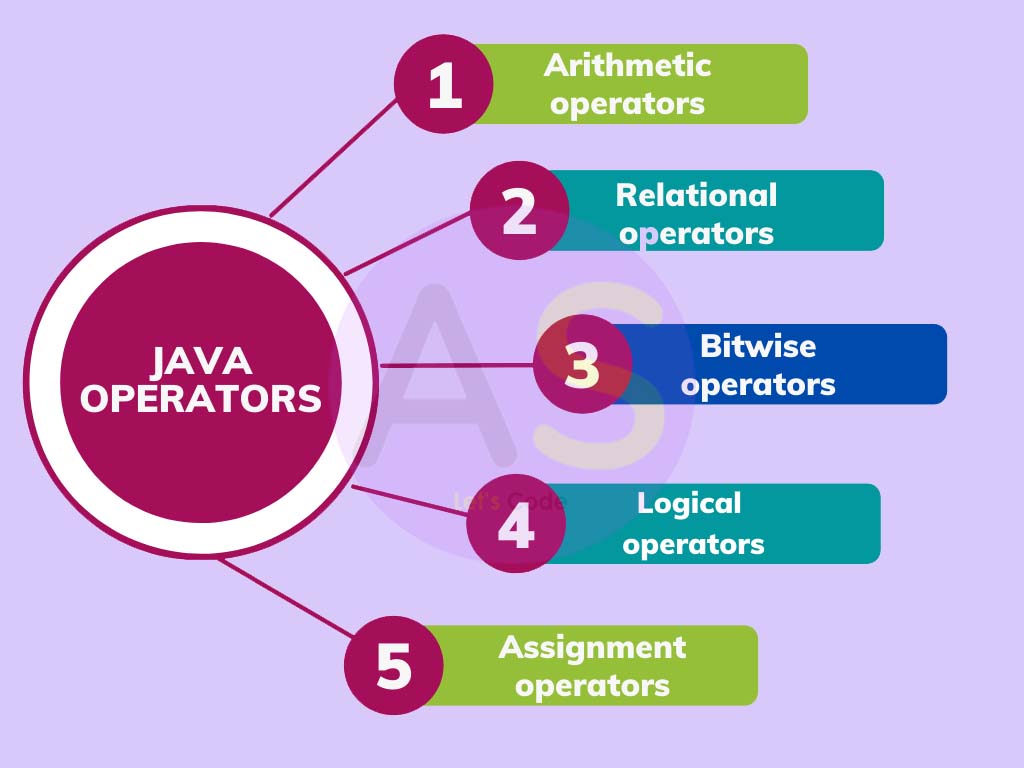
Unary Operators | +(unary) | -(unary) | ||||
Assignment Operator | = | |||||
Arithmetic Operators | + | - | * | / | % | |
Relational Operators | > | < | >= | <= | == | != |
Increment/Decrement Operators | ++ | -- | ||||
Logical Operators | && | || | ! | |||
Bitwise Operators | & | | | ^ | ~ | << | >> |
Conditional/Ternary Operator | ?: |
Escape Sequences
These are some special non graphic characters, which are used as commands to direct the cursor while printing. These characters are frequently used in Java programming called escape sequences. An escape sequence character begins with a backslash (\) and it is followed by one or more character. A table is given below with some escape sequence character
Escape Sequences
Short Description
\t
Horizontal tab
\v
Vertical tab
\\
Backslash
\'
Single quote
\"
Double quote
\n
New line
\b
backspace
\f
Formfeed
\0
Null
\r
Carriage return
Escape Sequences | Short Description |
\t | Horizontal tab |
\v | Vertical tab |
\\ | Backslash |
\' | Single quote |
\" | Double quote |
\n | New line |
\b | backspace |
\f | Formfeed |
\0 | Null |
\r | Carriage return |
Datatype
As the name suggest,java allows programmer to use any type of data needed in the program.Whenever,we
pass any value,we have to tell,what type of value we are using like integer type,decimal type or
alphabets type.So for that purpose datatype is used.
Datatype is used to declare variables and each variable is necesssary to be declared to be used in
the program.
Let us see example of this concept.
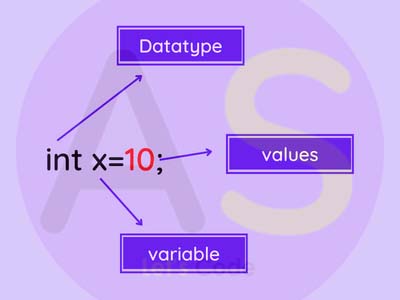
Here "x" is the variable that contains value as 10.And after x variable is int datatype that
allow variable x to store integer value.This int datatype represent the type of data which is stored in x.
The datatype is broadly divided into two types,these are:
-
Primitive Datatype: This datatype include byte,short,int,long,char,float,
double,and boolean
-
Non-Primitive Datatype: This datatype include classes,arrays and interfaces
double,and boolean
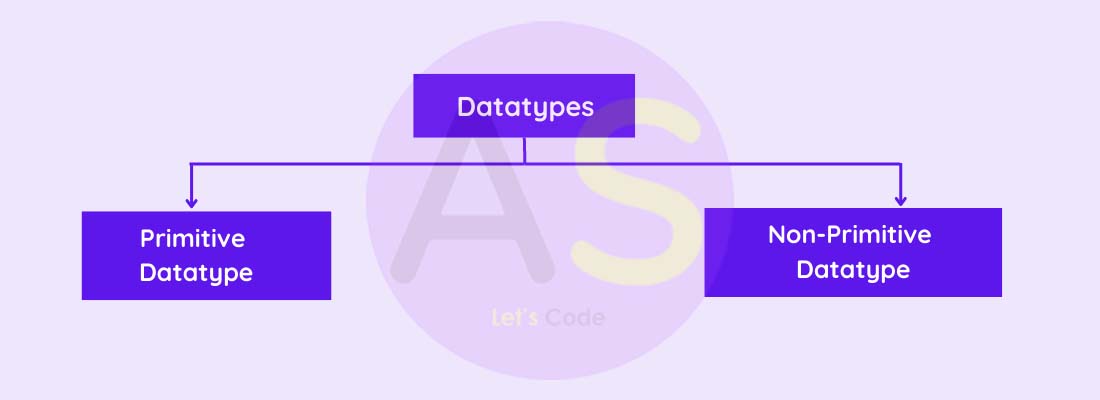
Primitive Datatypes
Primitive datatypes are those datatypes which are predefined in programming languages,without these
datatypes it is not possible to frame programs.They are the most basics datatypes in java languages.
There are 8 primitive datatypes boolean,char, byte, short, int, long, float and double .
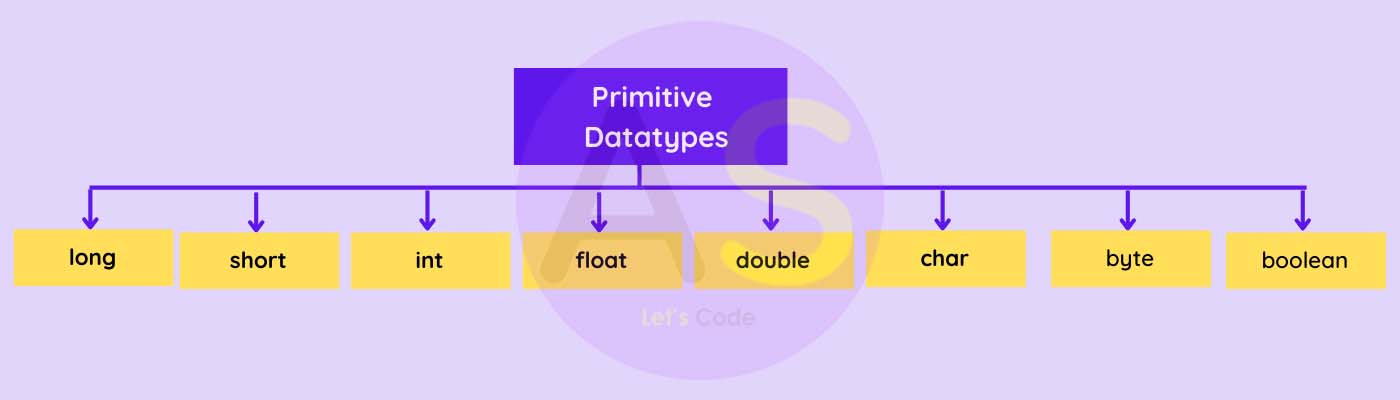
Int Datatype in Java
This datatype is used to store integer type values.Its size is 32bits (4 bytes) and has a default value of
0.
The maximum and minimum value of integer it can store is -2 billon (-2^31) to +2 billion (2^31 -1)
(approx.) .It can store numbers larger or smaller than the limits
Syntax:
int i=40;
The following table show size and range of int datatype.
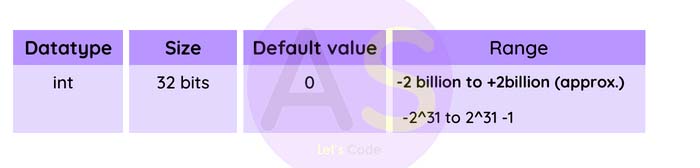
Float Datatype in Java
This datatype is used to store decimal values.It size is 32 bits(4 bytes) and has a default value as 0.0f.
It is always recommended to use float in place of double datatype because it can store low
precision value (upto 7 significant digits) while double store value with high precision values(upto 15
significant digits),so it can
save memory in large arrays of floating-point numbers.
Syntax:
float b=43.4f;
The following table show size and range of float datatype.

Double Datatype in Java
This is similar to float datatype ,that is used to store decimal values.Its size is 64bits(8 bytes) and has
a default value as 0.0d.
It is used to store high precision value,such as scientific readings,location of celestial bodies
Syntax:
double c=97.787d;
The following table show size and range of double datatype.

Char Datatype in Java
This datatype is used for storing single value character.Its size is 16 bits(2 bytes) and has default value
as '\u0000',this is unicode format which java uses in place of ASCII.
These datatypes are enclosed with single quotes,for example 'k','q'.These characters group together and form
a string,example "java","code".
Syntax:
char c='h';
The following table show size and range of char datatype.

Short Datatype in Java
It is similar to int datatype,and is used to save memory in large arrays.its size is 16 bits (2 bytes) and
has a default value as 0.
Syntax:
short x=11;
The following table show size and range of short datatype.

Long Datatype in Java
It is similar to int datatype,and is used to huge sized numeric value .its size is 64 bits (8 bytes) and
has a default value as 0.
It is useful where int type is not large enough to store the desired value
Syntax:
long x=93219898L;
The following table show size and range of long datatype.

Byte Datatype in Java
This datatype is used to store numeric values,it can be used as a replacement for int datatype as it is
useful for
saving memory in large arrays,it doesn't have the size range as the integer datatype.
Its size is 8bits(1 byte) and has default value as 0.
Syntax:
byte x=8;
The following table show size and range of byte datatype.

Boolean Datatype in Java
This datatype have only 2 values which are 'true' and 'false',these represent logical value.Its size is only
8bits(1 byte) that is either true or false.It has a default value as 'false'.
Syntax:
boolean check=true;
The following table show size and range of boolean datatype.

Non-Primitive Datatype
Non-Primitive datatype are also called user-defined datatype.They are created by programmers and can be assigned as null.
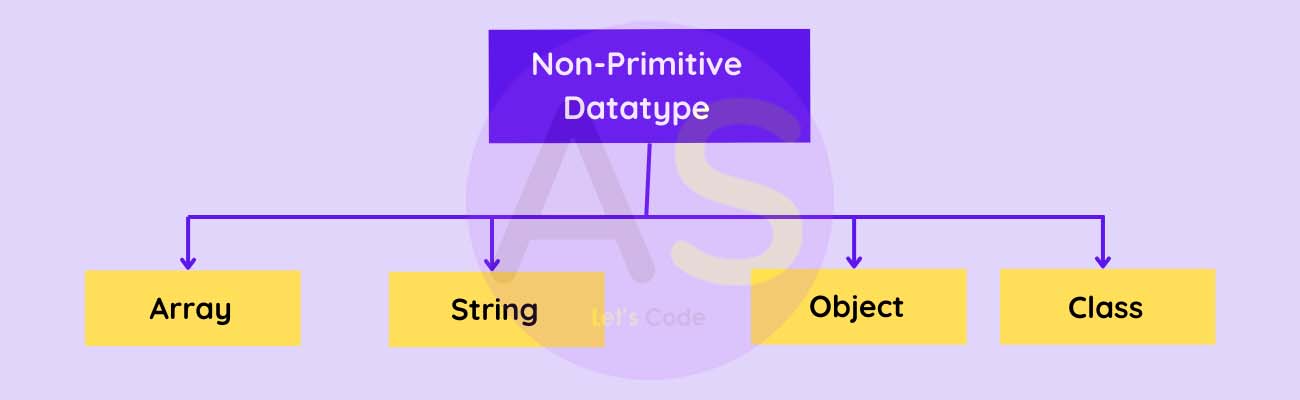
Object
It is defined as a real life entity,whatever we see in real life is termed as an object.These entities have
some properties and task to perform.It is a basic unit of object-Oriented Programming language.
An object is also said to be an instance of class,that is ,it will follow all the prototype defined by the
class.There can be mutliple instance of a class in a program.
An object consists of:-
-
unique Identity:Each object has unique Identity,that enable object to interact with other
objects
-
Behavior: It tells what object does.It can be used a response for one object to other objects
-
State/Properties/Attributes: It tells how the object will look like.
Class
- A Java class is a structure from which an object can be instantiated and it can have various
methods and class attributes. A class determines the data fields and actions of an object.
- In Java class variables are used to define the data fields and methods to define the actions.
- It is also known as blueprint of prototype of an object
-
Classes are divided in two categories :
- Pre-defined Classes : Pre-defined
classes are those classes that already exists in Java, such as Scanner, System, String
etc
- User-defined Classes : User-defined
classes are created by you the programmer / user. These classes take on names that you
assign to them and perform tasks that you create . Such as Dog , Test, Demo etc
- Pre-defined Classes : Pre-defined classes are those classes that already exists in Java, such as Scanner, System, String etc
- User-defined Classes : User-defined classes are created by you the programmer / user. These classes take on names that you assign to them and perform tasks that you create . Such as Dog , Test, Demo etc
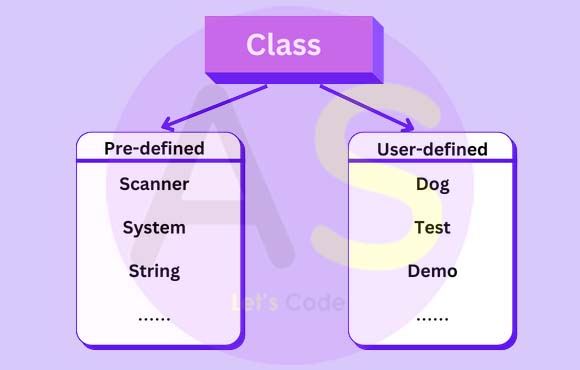
Strings
In java string is a class ,which has lot of different methods performed on string like
valueOf(),replace(),length(),etc.To access these methods we create instance of this class.
This String class is available in java.lang package and to use this package we write
import java.lang.*;
This package is the default package ,so we dont need to explicitly write in the program,it is
imported in the java program by default.
These String objects are immutable(cannot modified on same location).That is when we modify this class
object as:
str="World" .
Then new instance will be created,the more the number of times the user will modify this object ,the more
new instance will be created and consume the heap memory.
It is also a non-primitive datatype,having sequence of characters or we can say array of characters
that is enclosed in a double quotes.
Variables
Variables are defined as the name of memory location that contain a data value.It also said to be
user-defined name ,which is given by the user.
As soon as the variable is declared the memory will be allocated to the data value and can be used in java
program.
Variable can store any type of value,but for that we have to mention datatype before creating the
variable,like:
int x=12;
String a="ch";
char d='a';
double a=19.87;
Let us understand this concept by the example:-
String a="ch";
char d='a';
double a=19.87;
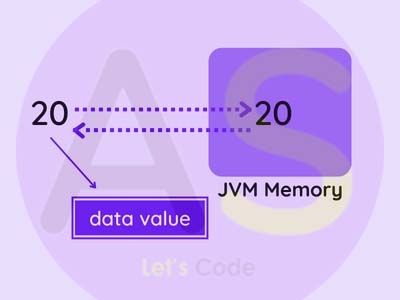
In the above diagram to store or access this data value,we have to add a user-defined name,so that we can call that data value by the name defined,for these purpose we use variables along with datatype.
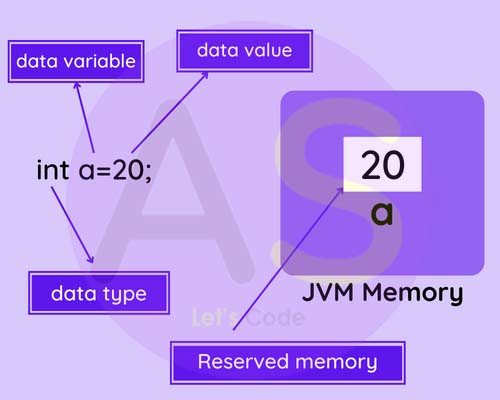
We can change value of variable also during program execution.
a=a+40;
Now the value in memory location for variable a will change to 60(20+40).
Constants
A constant is a variable whose value cannot be changed during the execution of program.
Syntax :
final float pi = 3.14f;
To define a variable as a constant, we just need to add the keyword “final" as shown in above syntax.
Let us understand it with a example given below :
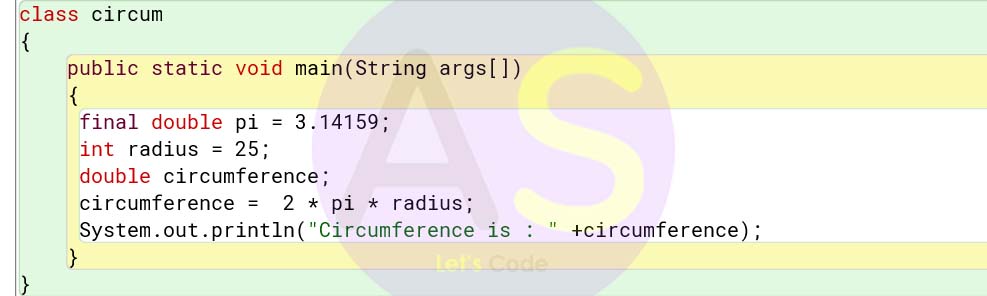
Output :
There are various advantages of using constants in our code :
(i) It increases the readbility of the program and make it easier for the user to understand the code.
(ii) Any unintentional changes to such variables is flagged by compiler.
(iii) If you want to make changes to such variables then we have to modify its value at one place and all other occurrences will be done automatically.
Comments
Comments in Java are non-executable statements that are ignored by the compiler and interpreter. It can be used to increase the readbility of the code by providing information or explanation about the variable, method, class or any statement.
Basically, there are three types of comments in Java. They are as follows:
- Single-line comments
- Multi-line comments
- Documentation comments
Single-line Comment :
A single-line comment starts and ends in the same line. To write a single-line comment, we can use the // symbol.
Syntax :
// This is a single line comment
Example :
class Main {
public static void main(String[] args) {
// prints "Hello, World!"
System.out.println("Hello, World!");
}
}
Multi-line Comment :
When we want to write comments in multiple lines, we can use the multi-line comment. To write multi-line comments, it should start with '/*' and ends with '*/' symbol.
Syntax :
/* This is a
multiline comment
.
.
.
Comment ends*/
Example :
class HelloWorld
{
/* This is an example of multi-line comment.
* The program prints "Hello, World!" to the standard output.
*/
public static void main(String[] args)
{
System.out.println("Hello, World!");
}
}
Documentation Comment :
This type of comments is used generally when you are writing code for a project/ software package. It helps you to generate a documentation page for reference, which can be used for getting information about methods present, its parameters, etc.
Syntax :
/** this is a documentation
comment
comment ends*/
// This is a single line comment
class Main { public static void main(String[] args) { // prints "Hello, World!" System.out.println("Hello, World!"); } }
/* This is a multiline comment . . . Comment ends*/
class HelloWorld { /* This is an example of multi-line comment. * The program prints "Hello, World!" to the standard output. */ public static void main(String[] args) { System.out.println("Hello, World!"); } }
/** this is a documentation comment comment ends*/