Class 9 ICSE - Java
Class 9th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 9th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Operators in Java
Definition
There are special symbols which are used to perform mathematical calculations like
addition,subtraction,multiplication,less than,greater than and many more opeations,all these symbols
are
called operators
And variables on which these operators are applied are called
operands.
Let us understand this by example:-
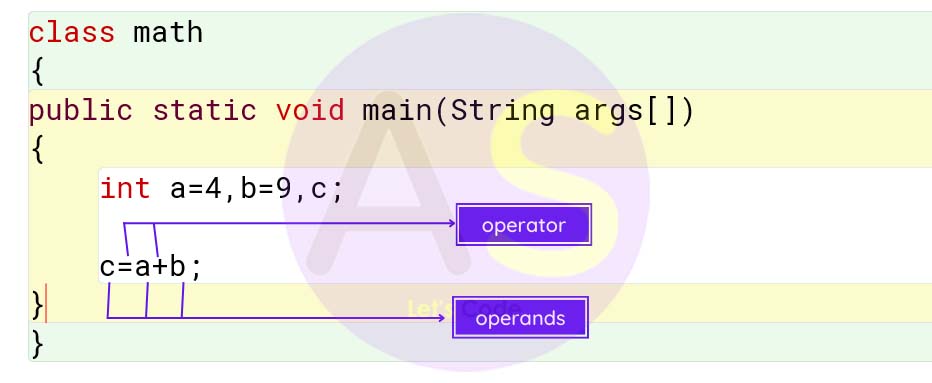
In the above example,"=","+" are operators which are performing different operations and "a","b","c" are operands on which operation is applied.
Forms of Operators
There are 3 forms of operators used in java,these forms depend upon the numbers of operands used to perform an operation.These forms are shown in below figure:
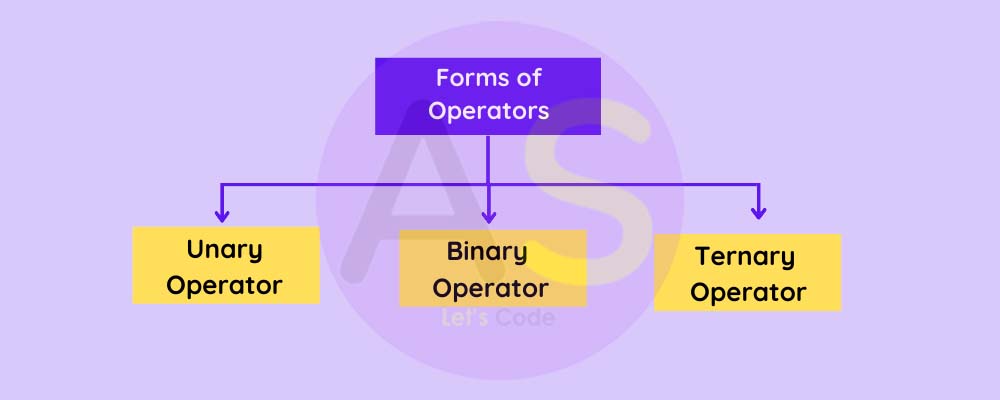
Unary Operators
These operators require only one operand to perform specific operation.They can be used to change the sign
of
operand or increment/decrement an operand.Examples of unary operator are +,-,++,-.
There are different types of unary operators used,which is shown in figure below:
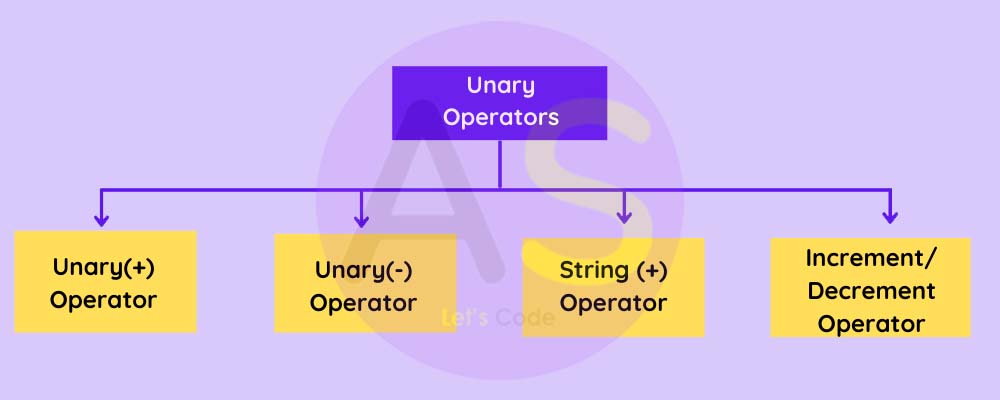
Unary(+) Operators
These operator represent positive operand.It is used before the operand.It will result the same value as the
operand.
let us understand by an example:-
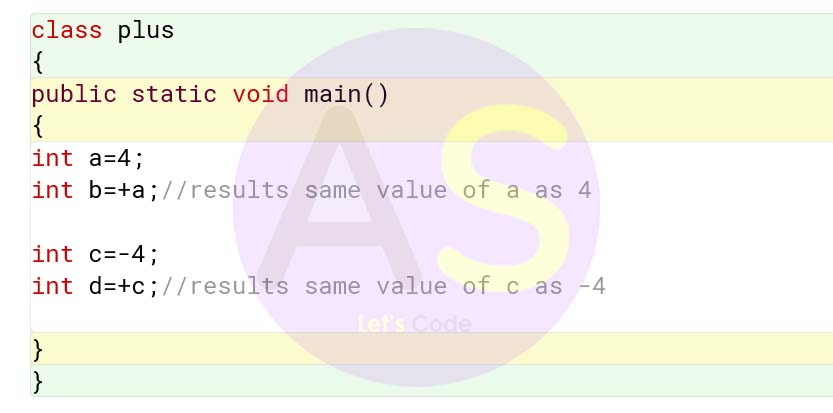
In the above program,this unary plus operator returns same value of the operand which are initially intialized.
Unary(-) Operators
This operator is used to convert the positive value of operand to negative value and vice versa.It negates the value of an operand,if it has positive value. Example of urnary(-) operator is:
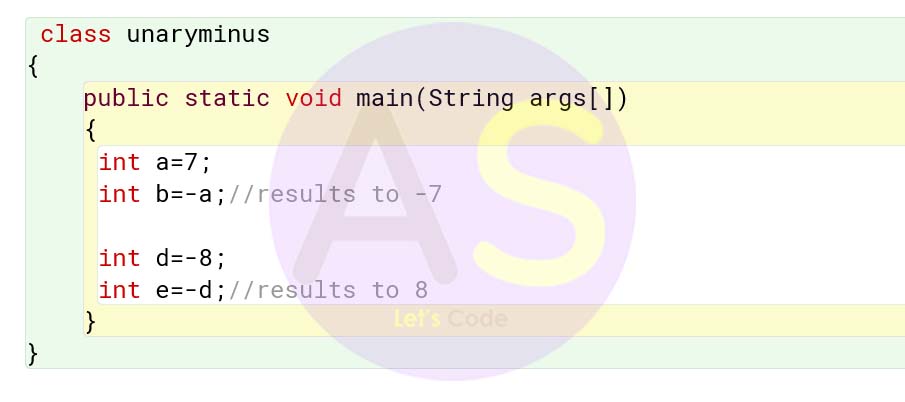
String(+) Operators
This operator looks same as unary plus operator,which has symbol "+",but this is used to concatenate two strings,it combines the two string together into a single string.Let us understand by an example:
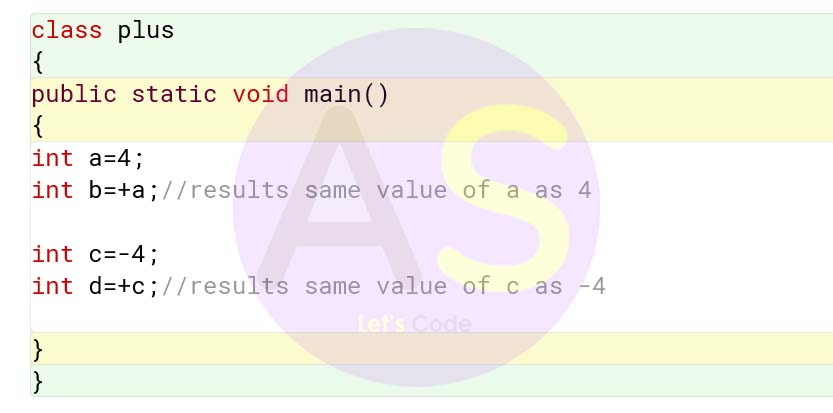
Increment and Decrement Operator
These operators are used to increase or decrease value of operands/variable by 1.It works
only on one
variable
so it is also a unary operator.
Here Increment oprator is represented by ++,which increase the value of operand by 1.
and Decrement operator is represented by --,which decrease the value of operand by
1.
Let us understand by an example:
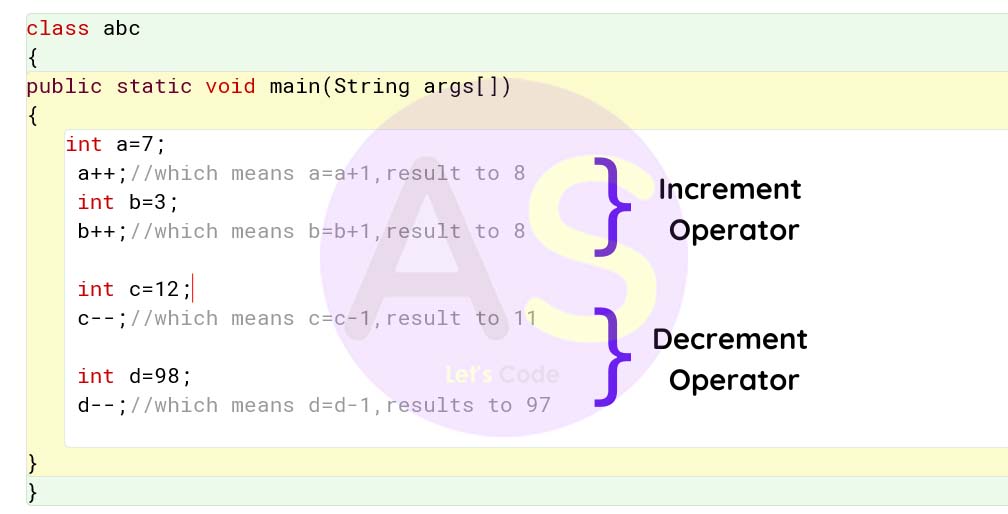
In java,increment/decrement operator is of two types:
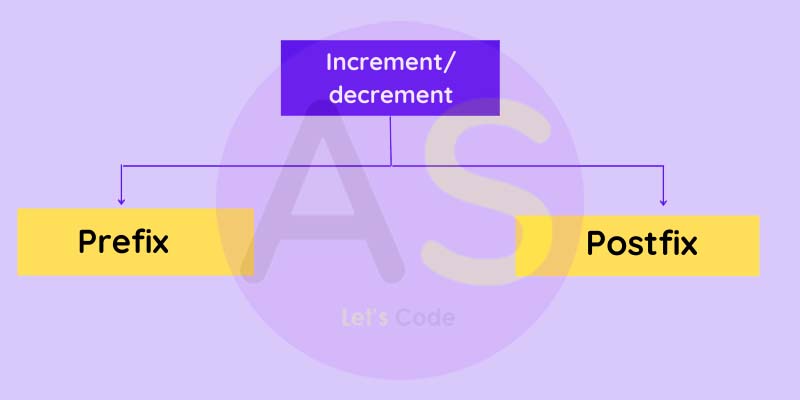
Prefix Operators
As the name suggest pre which means before,so prefrix operators are those operators in which
(++) or
(--)
operators
are applied after the variable.
This is also called change-then-use,this line means first increase or decrease the value
then use
that new
value
in specific operations.Here java perform the increment or decrement operation before using
the value
of
operand.
Let us undestand this by example:
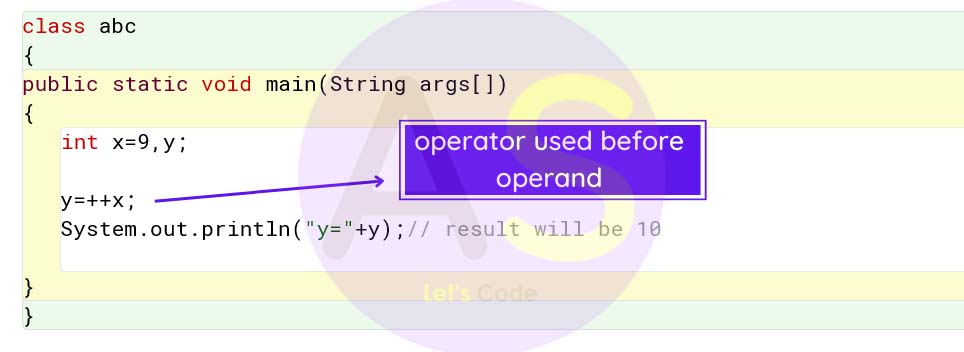
In the above example the value of y is increased by 1.That is in memory the value of x
changes
first from 9 to 10.
After increasing the value by 1,it will be assigned to y by the assignment
operator(=),so
here
both operand value will be x=10 and y=10.
Postfix Operators
As the name suggest post,which means after,so postfix operators are those operators in which
(++) or
(--)
operators are applied before the variable.
This is also called use-then-change,which means first use the value in expression then
change the
value by
1.Here increment or decrement operation are performed after using the value of operand.
let us understand this by example:
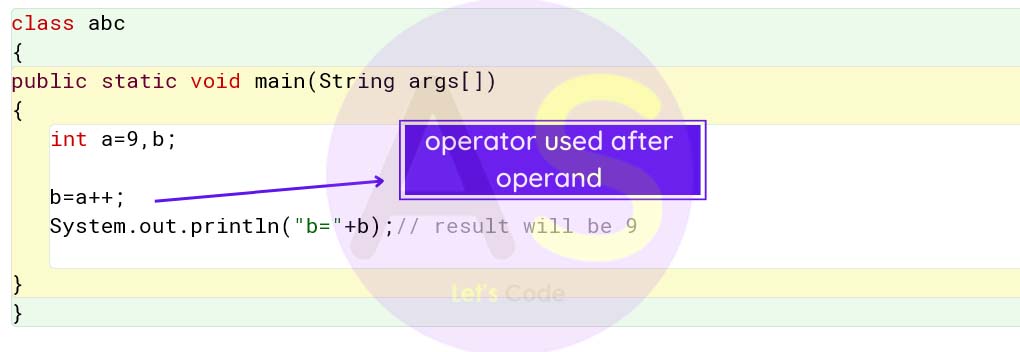
In the above example,first the value of a will be assigned to b by the assignment
operator(=),so now
the value
of b becomes 9 here.After assigning the value to b,now the the variable a will change to
10 ,that is increasing the value of a by 1,yielding
a=10.
Binary Operators
These operators require two operands to perform specific operation.It can be used to compare two values,use
to perform arithmetic operations and can combine more than two expressions and compare them.Examples of
binary
operator are >,<,>=,&&,||,etc.
There are different types of binary operators used in java .Let us discuss each of these operators one
by one
Arithmetic Operators
In java,Arithmetic operators are used to perform simple and advanced operations on primitive datatypes like
addition,subtraction,multiplication and
division,etc and many other arithmetic operations.
Arithmetic operators are of 4 types:
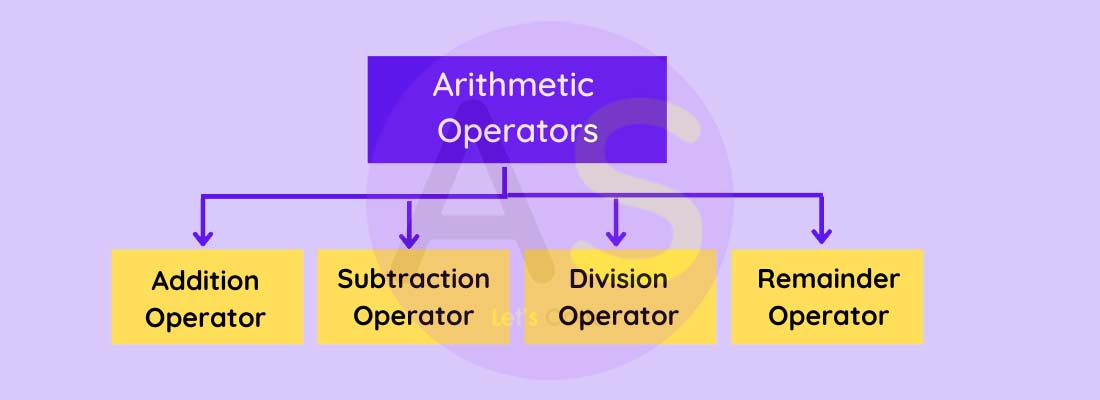
Addition Operator(+):
This operator is used to add the value of two operands and results the sum of its operands.
Here the operands must be of integer type or float type.Let us see the examples of this operator.
Subtraction Operator(-):
This operator is used to subtract the second operand with the first and result the difference of its two
operands.
Here the operands must be of integer type or float type.Let us see the examples of this operator.Let us see
the example of it.
Division Operator(/):
This operator is used divide the first operand by second operand and returns quotient value of the division
between two operands.
Here both the operands must be of integer type.Let us see by the example
Remainder Operator:
This operator is also called modulus operator.It finds the remainder by dividing the first operator by
second operator.And
results the remainder value of the division
between two operands.
Here both the operands must be of integer type.Let us see by the example
Relational operator
These operators are used to compare the value of two variables.It will always result to boolean type,that
is,true or false.
These cannot be used on string datatype value,because in string compareTo() and
equals() methods are used to compare values of string.
In java ,Relational operators are of 6 types:
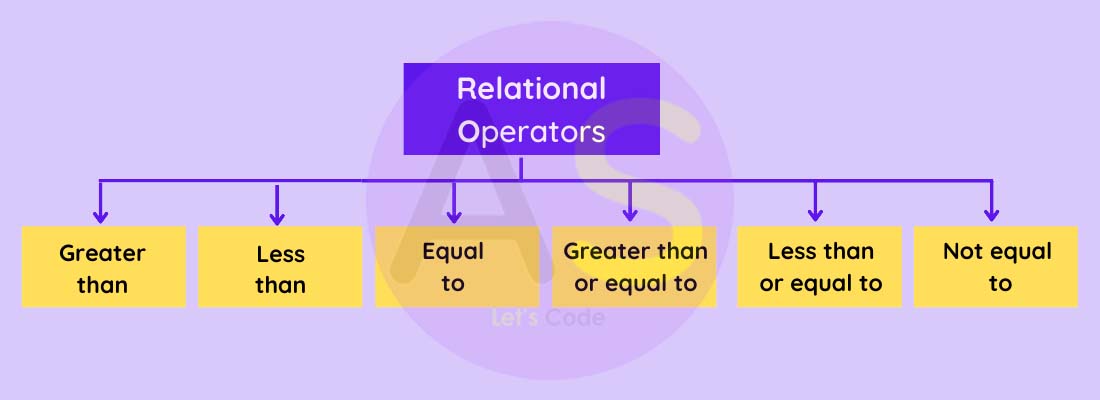
This operator is used to compare two operands,it results to true if first operand is greater than second operand.
Less than(<) :This operator is used to compare two operands,it results to true if first operand is less than second operand.
Greater than or equal to(>=):This operator is used to compare two operands,it results to true if first operand is greater than or equal to second operand.
Less than or equal to(<=):This operator is used to compare two operands,it results to true if first operand is less than or equal to second operand.
Not equal to(!=):This operator is used to compare two operands,it results to true if first operand is not equal to second operand.
Logical Operators
These are those operators which forms compound condition by combining two or more
conditions.It is
used for
complex-decision making purposes.
This logical operator returns either true or false i.e, boolean type value,depending upon
the
condition.
In java,logical operators are of 3 types:
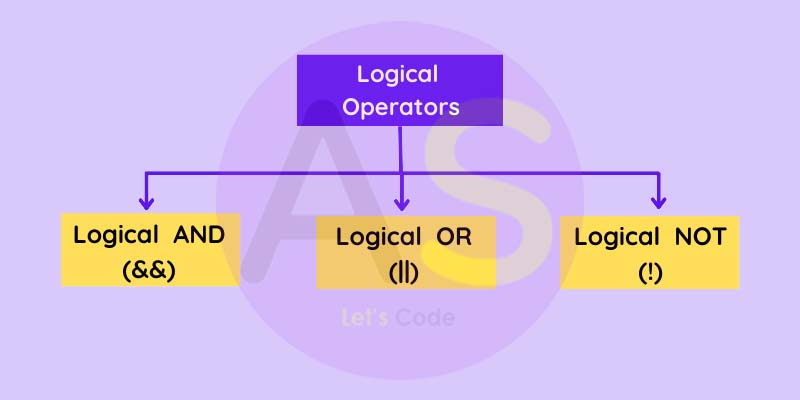
Logical AND(&&):
This operator is used to combine two or more conditions,and it results true when all the
condition
is
true,otherwise it will give false.In this operator two operand is required so it is also one
of
the
binary
operator.
Syntax for logical AND is:
Let us understand by an example:
Logical OR(||):
This operator is used to combine two or more conditions.It will result true when all
the
conditions in the expression
is
true or any one of the condition in the expression is true ,and results false, when all
conditions in expression are false.
In this
operator two
operand is required so it is also one of the binary
operator.
Syntax for logical OR is:
Let us understand by an example:
Logical NOT(!):
This operator negates or reverse the truth value of its operand,that is if expression is
true,then
it
returns false and if expression is false,it results true.
Let us understand by an example:
Assignment Operators
These operators are used to assign values to the variable of the left side of equal sign.The
value
which
will be there on the right will be assigned to the left side.
Syntax of Assignment operator is:
variable=expression;
Let us consider assignment operator by an example
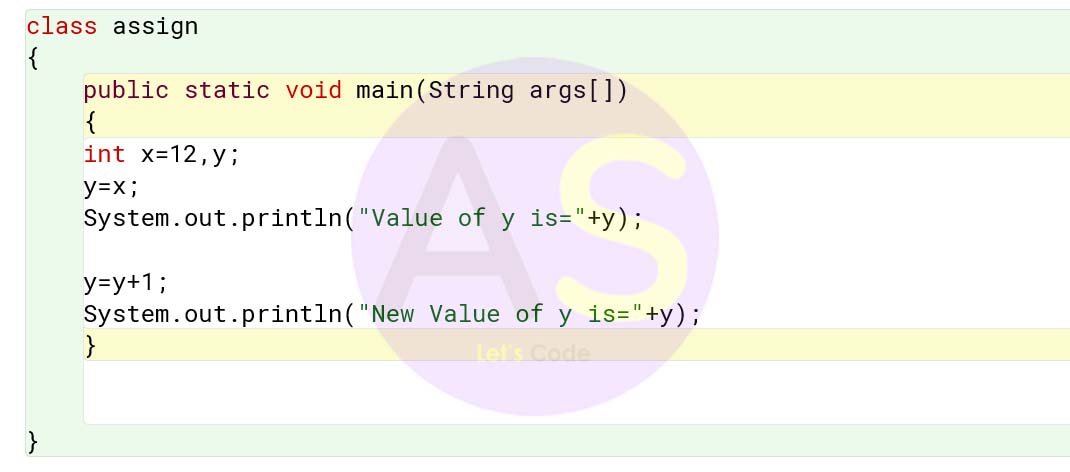
In the above example,variable x is created with value as 12,after that this value of x is assigned to variable y using assignment operator(=).Now the variable y holds the value of x as 10.After that new value of b is assigned by adding the old value of b by 1.Now b holds the new value as 13.
Ternary Operator
Ternary operator require three operands to perform specific operations.Ternary operator is
also called conditional operator.This
operator
decreases
the statements of if-else,and used for any condition.
It consist of a condition followed by a question mark(?).It contains two expressions
seperated by a
colon(:)
If the condition is true,then first expression will be executed,otherwise,the second
expression will
be
executed.
Syntax of this operator is:
(condition)?(expression1):(expression2);
Let us understand by an example:-
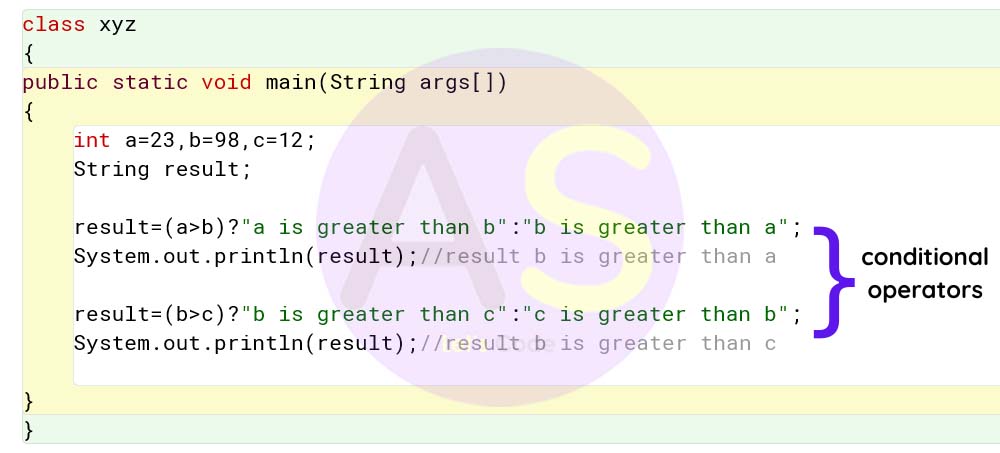
Precedence of Operators
Precedence of operator determines the order in which the operators in an expression are
evaluated.
Operators
Precedence
Associativity
postfix increment and decrement
++
--
left to right
prefix increment and decrement, and unary
++
--
+
-
~
!
right to left
multiplicative
*
/
%
left to right
additive
+
-
left to right
shift
<<
>>
>>>
left to right
relational
<
>
<=
>=
instanceof
left to right
equality
==
!=
left to right
bitwise AND
&
left to right
bitwise exclusive OR
^
left to right
bitwise inclusive OR
|
left to right
logical AND
&&
left to right
logical OR
||
left to right
ternary
? :
right to left
assignment
=
+=
-=
*=
/=
%=
&=
^=
|=
<<=
>>=
>>>=
right to left
Operators | Precedence | Associativity |
---|---|---|
postfix increment and decrement | ++ -- |
left to right |
prefix increment and decrement, and unary | ++ -- + -
~
!
|
right to left |
multiplicative | * / % |
left to right |
additive | + - |
left to right |
shift | << >> >>>
|
left to right |
relational | < > <=
>=
instanceof
|
left to right |
equality | == != |
left to right |
bitwise AND | & |
left to right |
bitwise exclusive OR | ^ |
left to right |
bitwise inclusive OR | | |
left to right |
logical AND | && |
left to right |
logical OR | || |
left to right |
ternary | ? : |
right to left |
assignment | = += -= *=
/=
%= &= ^= |=
<<= >>= >>>=
|
right to left |
New Operator
As we have learned about class concept earlier,that it contains methods and instance
variable as a user-defined types.And to access these variables and methods,we create objects
of respective class.
In java,there are two mehods to create objects.
First method to create object is, to declare a variable of the class type.This variable can
be used as a reference of an object.
Syntax for this object creation is
Student xyz;
Second method to create object is,to use new keyword,this keyword will dynamically
allocates memory for an object,and intializes its data memmbers by using default or
paramterized constructor after it.
Syntax for this object creation is:
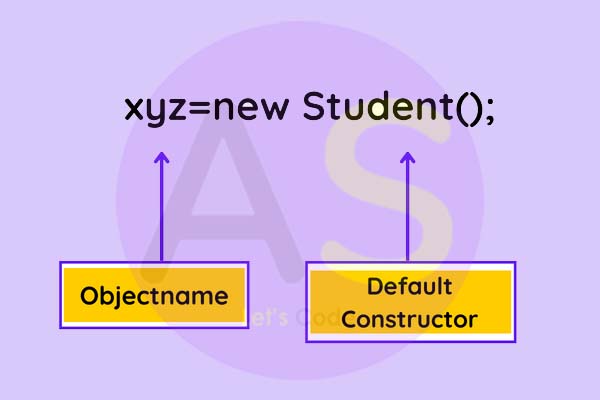
Inside the memory,this new keyword allocates memory for xyz object,and intializes the data members of the student class also,as shown in the given figure below
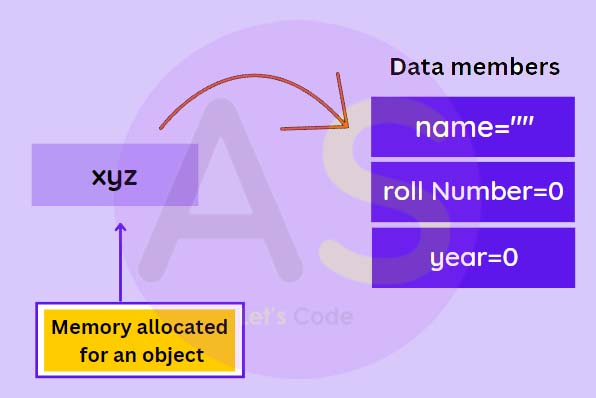
So we have seen above, there are two ways to create an object of a class which are as:
Student xyz;
xyz=new Student();
xyz=new Student();
On combining these two ways,we can use this combined syntax to declare and allocate memory in one statement only ,that is, declaration of class variable and creating instant of class can be performed in one statement.
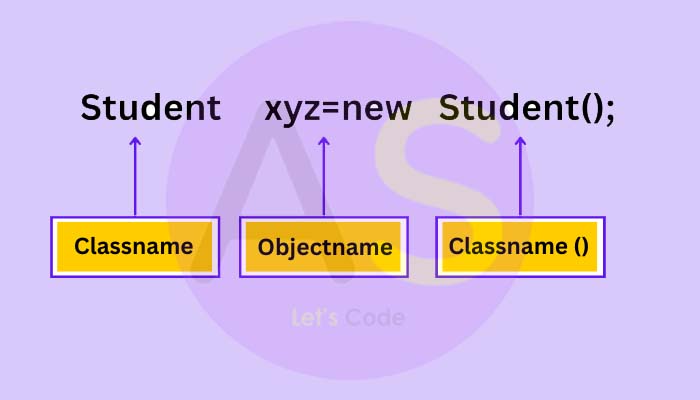
We can also create multiple objects of a class also.Let us see the example of object creation using the new keyword.
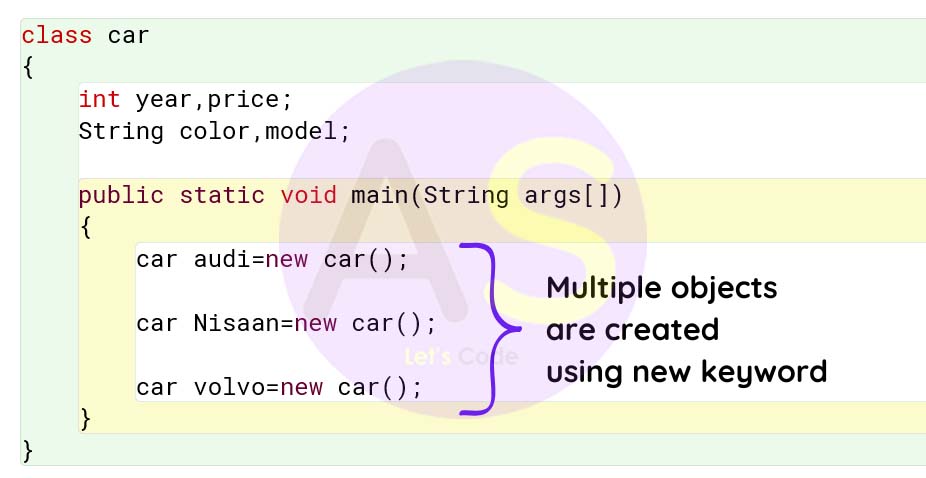
Dot Operator
This operator is used to access or call the member variables and member methods of a class.
Once the object is created we can use that object to access variables and methods of a class
by dot operator.
Let us see the example for this concept:
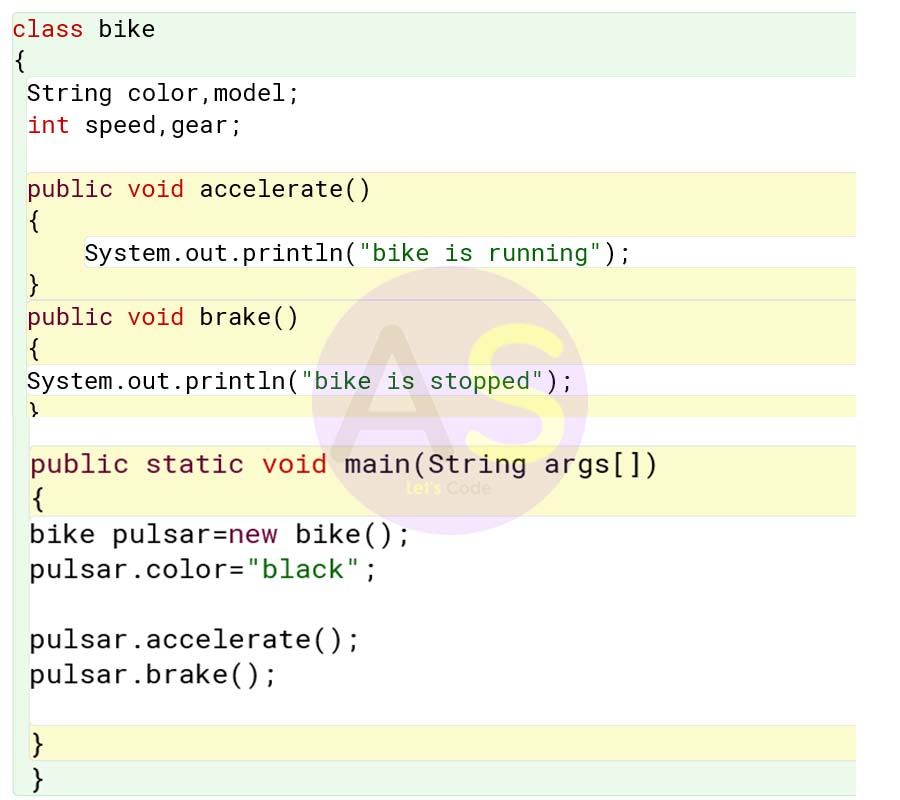
In the above example we created a class named as bike which has various methods and
variables.
Now to access these methods and variables we use object with dot operator,then method or
variable name after that.
pulsar.color=black;
pulsar.accelerate();
pulsar.brake();
pulsar.accelerate();
pulsar.brake();
Expressions
Anything that returns some value,even null value is termed as expression.It consists of
variable,operator,method invocation and constants.
Every expression consist of at least one operator and one operand.The value of one
expression can be combined with another expression to form a complex expressions.
Let us see the example of expressions:
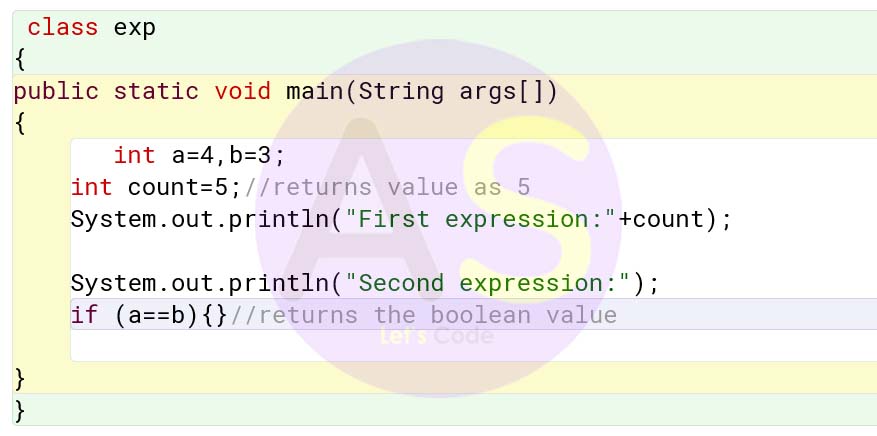
There are different types of expressions used in java,which are:
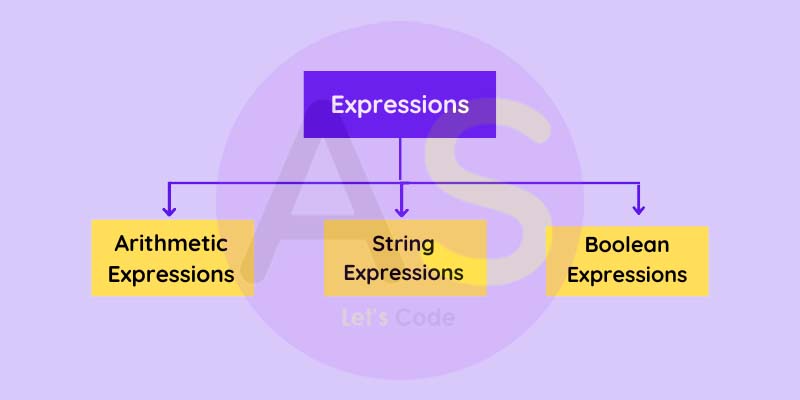
Arithmetic Expressions
Arithmetic expressions consist of numeric value and arithmetic operators.It can be pure integer,real or mixed expressions.Let us discuss each one by one.
Integer Expressions
These expressions are formed by integer constant, variables and integer
arithmetic operator all these are combined together and form an integer expressions.
Let us understand by an example:
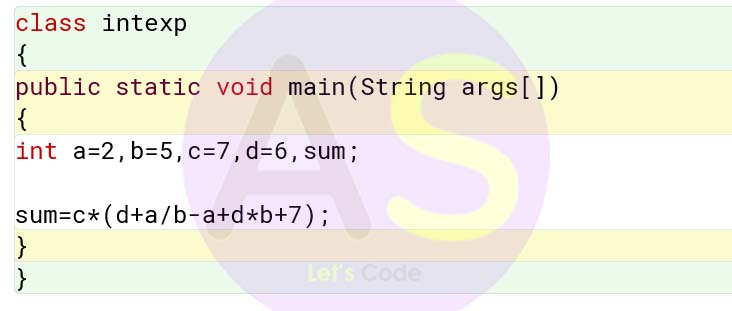
Real Expressions
It is formed by real constants,variables and integer arithmetic operator all these are combined to form real expressions
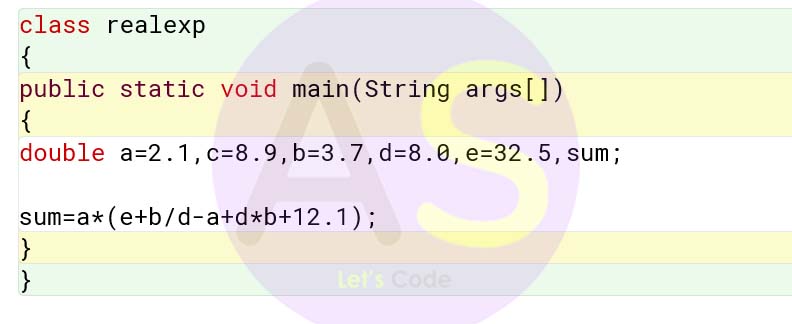
Mixed Expressions
It is formed by the combination of integer and real expressions along with integer arithmetic operators
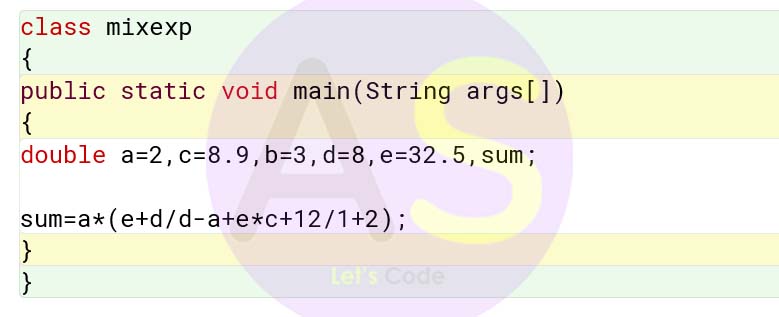
String Expressions
This expressions consist of group of characters and functions of strings.
Here we cannot perform arithmetic operations like addition,subtraction,division,etc,but java
provide other operators like "+" operator by which we can perform concatination of
strings.Let us understand this by example:
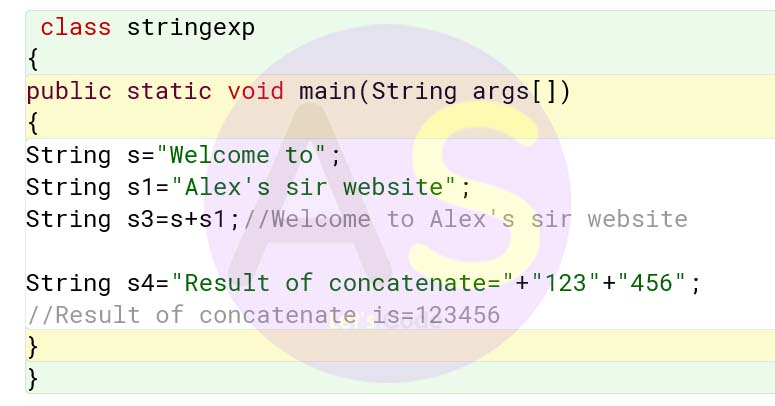
Boolean Expressions
Boolean expression returns either true or false.It contains relational and logical operators along with booolean variables.These expressions are basically used for conditions only.Let us understand by an example
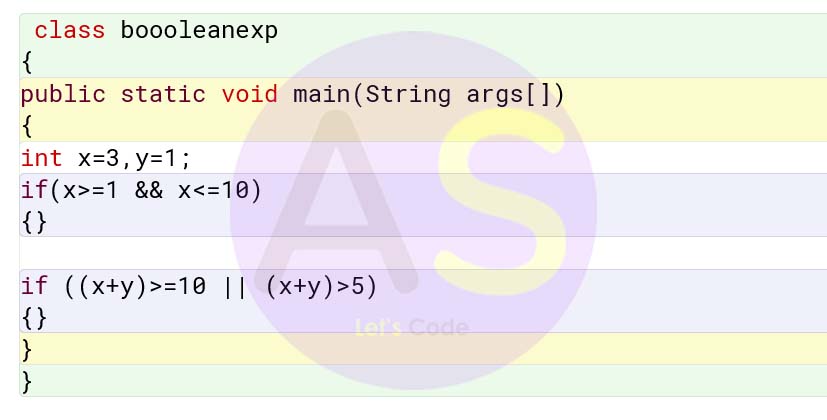
Type Conversion
Java allows programmmers to use different types of data such as
int,float,long,double,char,etc.It also allows to convert these datatypes from one type to
another type,this process of conversion is called type conversion.
In arithmetic expression,if the variables and constants have same datatype,then it will
result to same datatype,no type conversion will be performed.
If there are different types of variables and constants used in arithmetic expression then
type conversion will be performed,to attain result in a specific type
There are two types of conversion performed in java:
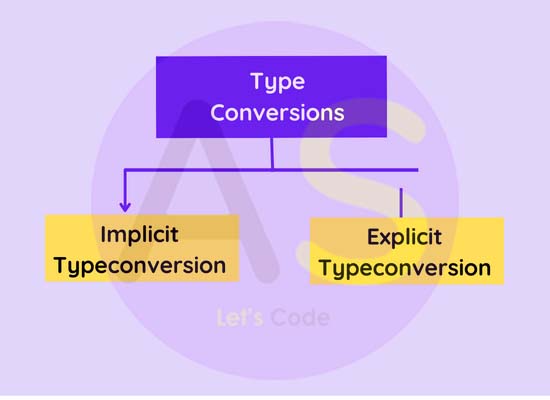
Implicit TypeConversion
This coversion is also called widening or automatic type conversion.This conversion takes
place when two datatypes are automatically converted.It converts lower datatype to higher
datatype.
There is also no loss of data occurs throughout the data conversion.There is also no chance
of throwing exception throught the conversion.
Let us see the example of implicit conversion
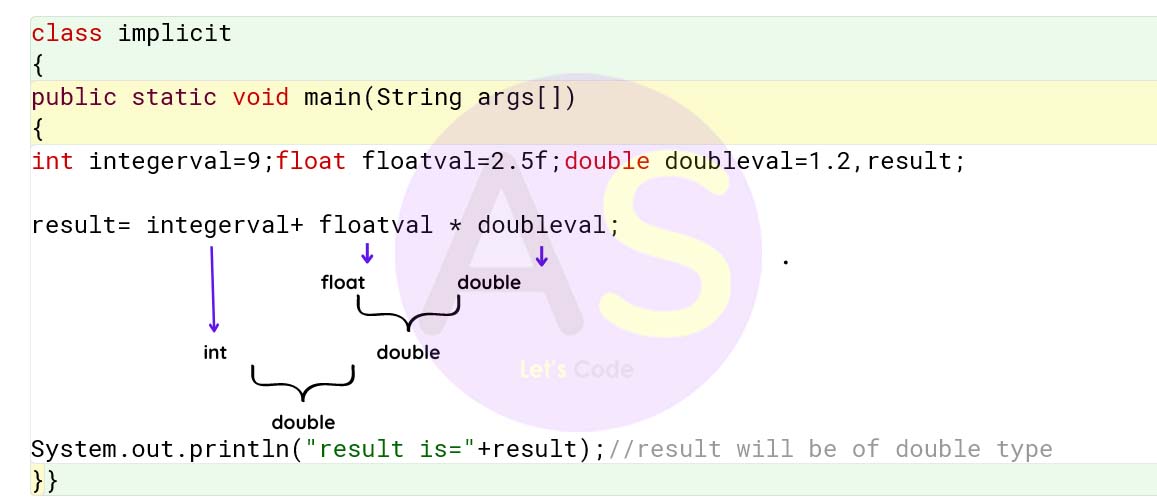
Explicit TypeConversion
Explicit conversion is also called narrow typecasting.Here,programmer forcefully converts a
large datatype to a smaller datatype.It used to convert higher datatype to
lower datatype
Here,Loss of data occurs in data conversion,and it can draw error as "Possible lossy conversion",if we try to perform conversion
without typecasting
Let us understand explicit type casting by an example:
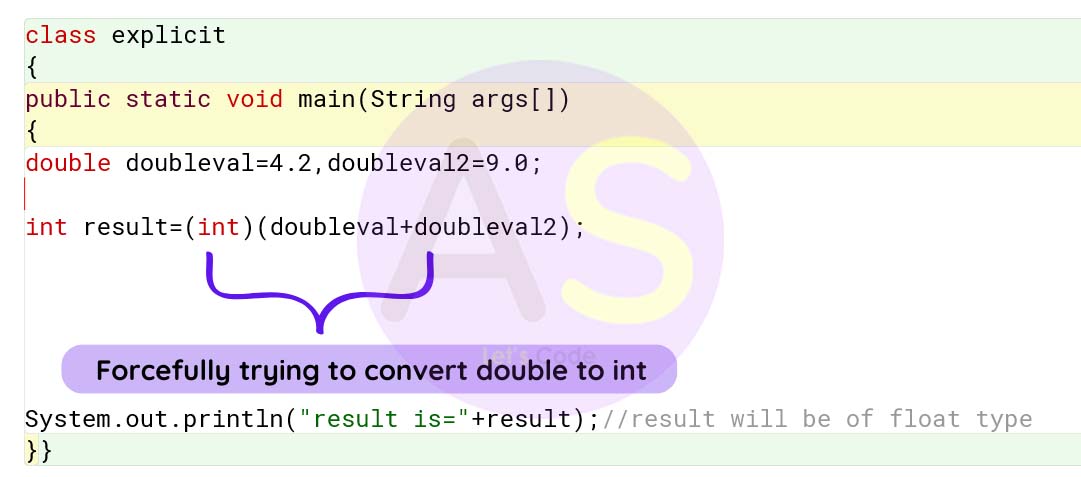