Class 9 ICSE - Java
Class 9th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 9th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Inputs in Java
Packages
A package is a group of similar types of classes, interfaces and sub-packages.Packages can be categorized in two form, built-in package and user-defined package.
Common Packages in Java :
There are many built-in packages such as java, lang, awt, io, util, etc. A package that is contained in another package is called as sub-package.
One of the commonly used sub-packages in Java is the util package. Since util is contained within the Java package it is written as "java.util"
Package
Short Description
java.lang
Provides classes that are fundamental to the design of the Java programming language such as primitive data types, string and math functions.
java.util
Provides classes for printing, scanning etc.
java.io
Provides classes for input and output of data.
java.awt
Provides classes to implement graphical user interfaces.
java.net
Provides classes for networking.
java.applet
Provides classes for implementing applets.
Using and importing Packages :
import statement allows a program to refer to class is defined in other packages without using the fully qualified class name.
Syntax :
import package1[.package2][.package3].classname;
Here package1 is the name of the top level package package2 is the name of the package that is inside package1 and so on you can have any number of packages in package hierarchy. At last specify the classname.
Example :
Input using Parameters :
In this form of input the required data is decided at the time of program execution. The variable whose values are to be input must be used as parameter to the method. Let us understand this with the example below :
Output :
Package | Short Description |
java.lang | Provides classes that are fundamental to the design of the Java programming language such as primitive data types, string and math functions. |
java.util | Provides classes for printing, scanning etc. |
java.io | Provides classes for input and output of data. |
java.awt | Provides classes to implement graphical user interfaces. |
java.net | Provides classes for networking. |
java.applet | Provides classes for implementing applets. |
import package1[.package2][.package3].classname;
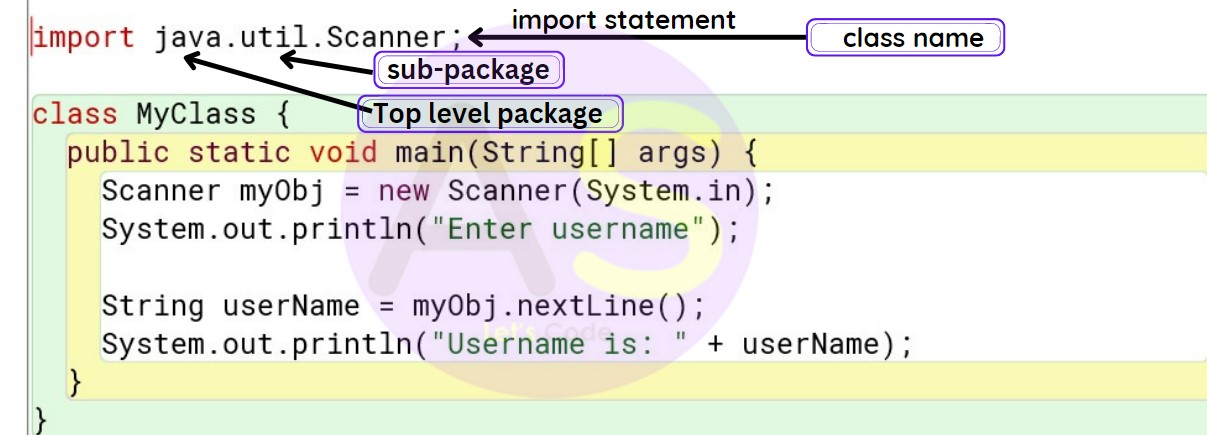

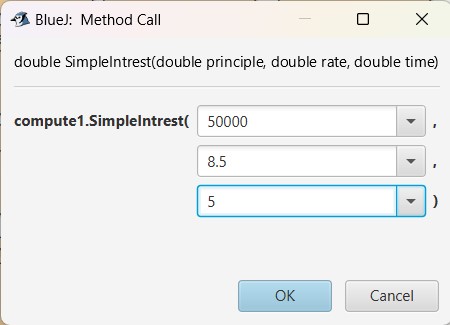
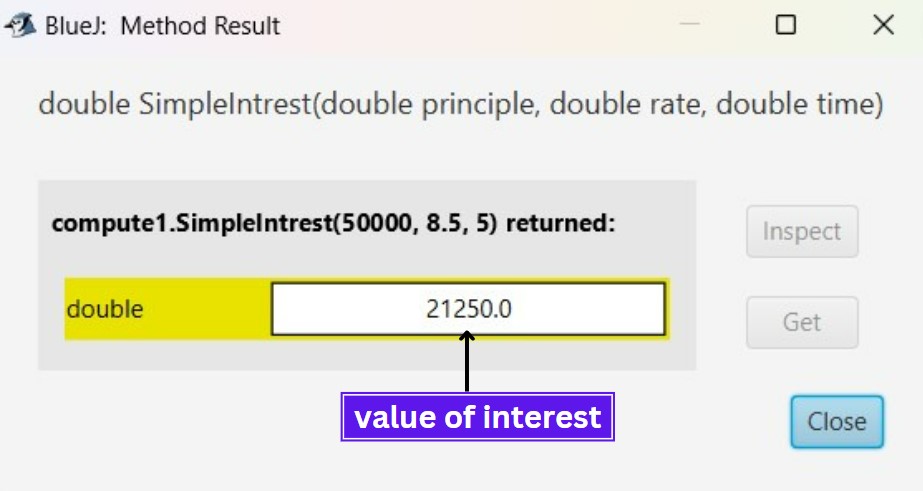
Using Scanner Class
What is Scanner Class :
Scanner Class is a library class which reside in the package "util". To use scanner class function it has to
be included in the program using :
⇛ import java.util.Scanner;
Need for Scanner Class :
You know that class contains the functions a group of function are associated with the class so if you want
to use those function you have to use the concern class. Similarly, Scanner class is used in the program to
take user input, so that we can use the associated function of the scanner class in our program.
Functions of Scanner Class :
(i) nextShort() ⇛ short variable = Scanner obj.nextShort();
(ii) nextInt() ⇛ int variable = Scanner obj.nextInt();
(iii) nextLong() ⇛ long variable = Scanner obj.nextLong();
(iv) nextDouble() ⇛ double variable = Scanner obj.nextDouble();
(v) nextFloat() ⇛ float variable = Scanner obj.nextFloat();
(vi) next() ⇛ String variable = Scanner obj.next();
(vii) nextLine() ⇛ String variable = Scanner obj.nextLine();
(viii) next.charAt(0) ⇛ char variable = Scanner obj.next().charAt(0);
How to use Scanner Class :
Program :
Output :
(ii) nextInt() ⇛ int variable = Scanner obj.nextInt();
(iii) nextLong() ⇛ long variable = Scanner obj.nextLong();
(iv) nextDouble() ⇛ double variable = Scanner obj.nextDouble();
(v) nextFloat() ⇛ float variable = Scanner obj.nextFloat();
(vi) next() ⇛ String variable = Scanner obj.next();
(vii) nextLine() ⇛ String variable = Scanner obj.nextLine();
(viii) next.charAt(0) ⇛ char variable = Scanner obj.next().charAt(0);
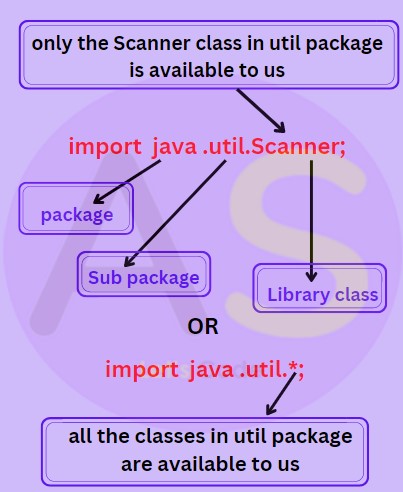
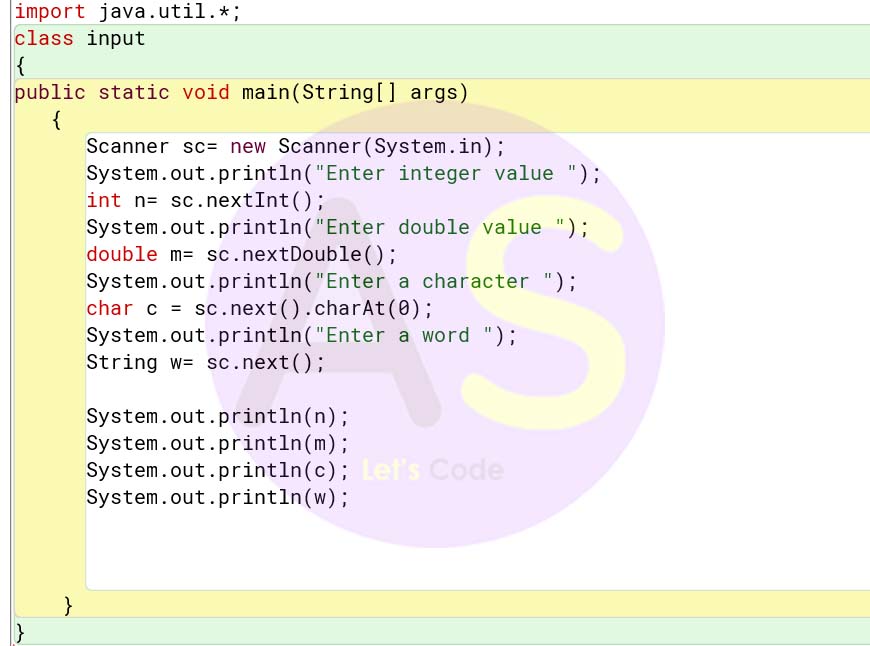
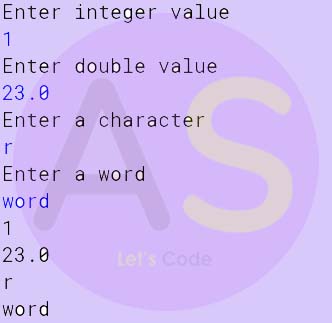
Errors
Errors are mistakes in a program that prevented from its normal working. In programming terms errors are often referred to as bugs.
Errors are broadly classified into three categories as follows :
(i) Runtime Errors
(ii) Syntax Errors
(iii) Logical Errors
Runtime Errors :
Run Time errors are those errors that occurs during the execution of the program when the user enters an invalid data or data which is irrelevant. To handle the error during the run time we can put our code inside the try and catch block and the error inside the catch block.
Some common runtime errors you may encounter are as follows :
- Divide by zero
int p=13, q=5, r=10;
int result;
result = p/(q*2 - r); //division by zero error
- Passing an invalid string into numeric value
class Testing
{
public static void method()
{
String x='765a';
System.out.println(" Result :" + Integer.parseInt(x)); ---->"765a" cannot converted to an integer
}
}
- Entering a string value where numeric values expected for the scanner object.
Scanner sc = new Scanner(System.in);
System.out.println("Enter Roll Number : ");
int rollNumber = sc.nextInt();
Entering a non integer value are 'R1234' the "Roll Number" will cause and runtime error.
- Running out of memory during program execution
- Trying to access the data file which doesn't exist.
Syntax Errors :
Syntax Errors are those errors which prevent the code from running because of an incorrect syntax such as a missing semicolon at the end of a statement or a missing bracket, etc. These errors are detected by the java compiler and an error message is displayed on the screen while compiling. Syntax Errors are also referred to as Compile Time errors.
Some common syntax errors are as follows :
- Missing semicolon after a statement
int sum
sum++
- Missing or unmatched brackets.
public void DisplayMethod
{
System.out.print( "Java");
- Incorrect spelling of keywords or identifiers.
In this example, the keyword static is misspelled, and the identifier counter is spelled with zero (0) instead of o.
public statc void Hello()
{
int counter;
cOunter++;
}
- Using variables that have not been defined but are being used in the program.
In this example the compiler will report syntax error because the variable sum has not been declared and initialised
public void TestMethod()
{
sum--;
}
- Incompatible assignment for example assigning a string value "322a" to integer variable.
Logical Errors :
logical errors are those when your program compiles and executes, but does the wrong thing or returns an incorrect result or no output. These errors are not detected by the compiler. Logical errors are also called Semantic Errors. These errors are caused due to an incorrect concept used by a programmer while coding. Syntax errors are grammatical errors whereas, logical errors are errors arising out of an incorrect meaning. For example, if a programmer accidentally adds two variables when he or she meant to divide them, the program will give no error and will execute successfully but with an incorrect result.
Some common logical errors are as follows :
- Using wrong variable name
public int FindMax()
{
int x=17, int y=23;
int max;
max=(x>y) ? x : y;
return x; // Returning wrong variable, max should be returned
}
- Using integer division by mistake instead of the floating point division. For example given x, y and z are all int using
(x + y + z)/3;
instead of :
(x + y + z)/3.0;
to compute average
- Using operators without understanding their precedence and associativity for example using :
x + y / z;
instead of :
(x + y) / z;
-
Displaying the wrong message
int p=13, q=5, r=10; int result; result = p/(q*2 - r); //division by zero error
class Testing { public static void method() { String x='765a'; System.out.println(" Result :" + Integer.parseInt(x)); ---->"765a" cannot converted to an integer } }
Scanner sc = new Scanner(System.in); System.out.println("Enter Roll Number : "); int rollNumber = sc.nextInt(); Entering a non integer value are 'R1234' the "Roll Number" will cause and runtime error.
int sum sum++
public void DisplayMethod { System.out.print( "Java");
In this example, the keyword static is misspelled, and the identifier counter is spelled with zero (0) instead of o.
public statc void Hello() { int counter; cOunter++; }
In this example the compiler will report syntax error because the variable sum has not been declared and initialised
public void TestMethod() { sum--; }
public int FindMax() { int x=17, int y=23; int max; max=(x>y) ? x : y; return x; // Returning wrong variable, max should be returned }
(x + y + z)/3;
instead of :
(x + y + z)/3.0;
to compute average
x + y / z;
instead of :
(x + y) / z;