Class 9 ICSE - Java
Class 9th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 9th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Conditional Construct
if statement
The if statement in java tests the condition. It executes the statement inside if block if condition is
true.
Syntax :
if(condition){
//statements
}
Example :
Output :
if(condition){ //statements }
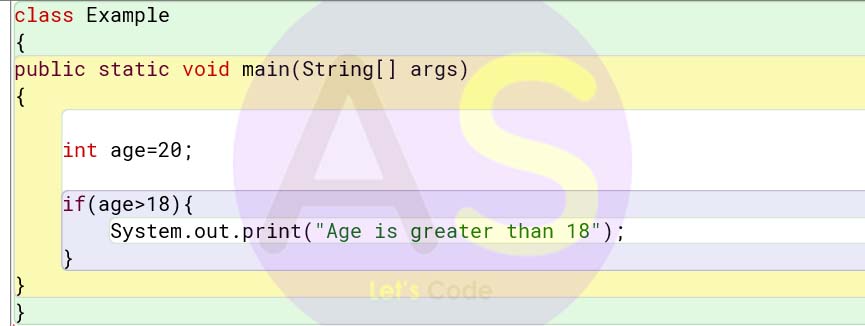

if-else statement
The if-else statement in java also tests the condition. if condition is true it executes the statement
inside if block otherwise the statement inside else block is executed .
Syntax :
if(condition){
//statements
}
else
{
//statements
}
Example :
Output :
if(condition){ //statements } else { //statements }
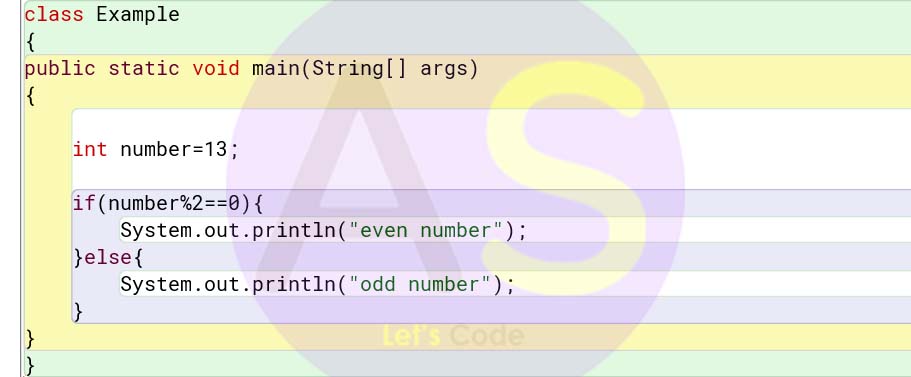

nested-if statement
The nested if statement contains if block within another if block. The inner if block executes only when
outer if block condition is true.
Syntax :
if(condition)
{
//statements
if(condition)
{
//statements
}
}
Example :
Output :
if(condition) { //statements if(condition) { //statements } }
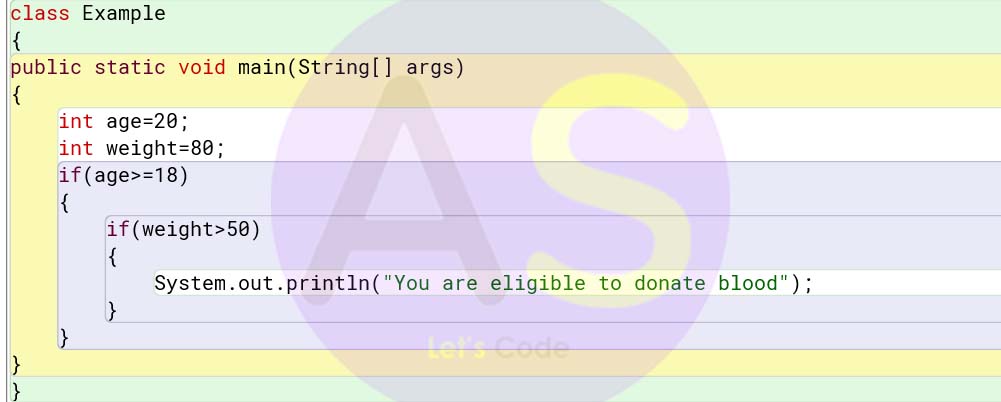

if-else-if ladder statement
It is used when multiple decisions are involved and in this along with if statement each else if construct
is used.
Syntax :
if(condition1)
{
//statements to be executed if condition1 is true
}
else if(condition2)
{
//statements to be executed if condition2 is true
}
else if(condition3)
{
//statements to be executed if condition3 is true
}
...
...
else
{
//statements to be executed if all the conditions are false
}
Example :
Output :
if(condition1) { //statements to be executed if condition1 is true } else if(condition2) { //statements to be executed if condition2 is true } else if(condition3) { //statements to be executed if condition3 is true } ... ... else { //statements to be executed if all the conditions are false }
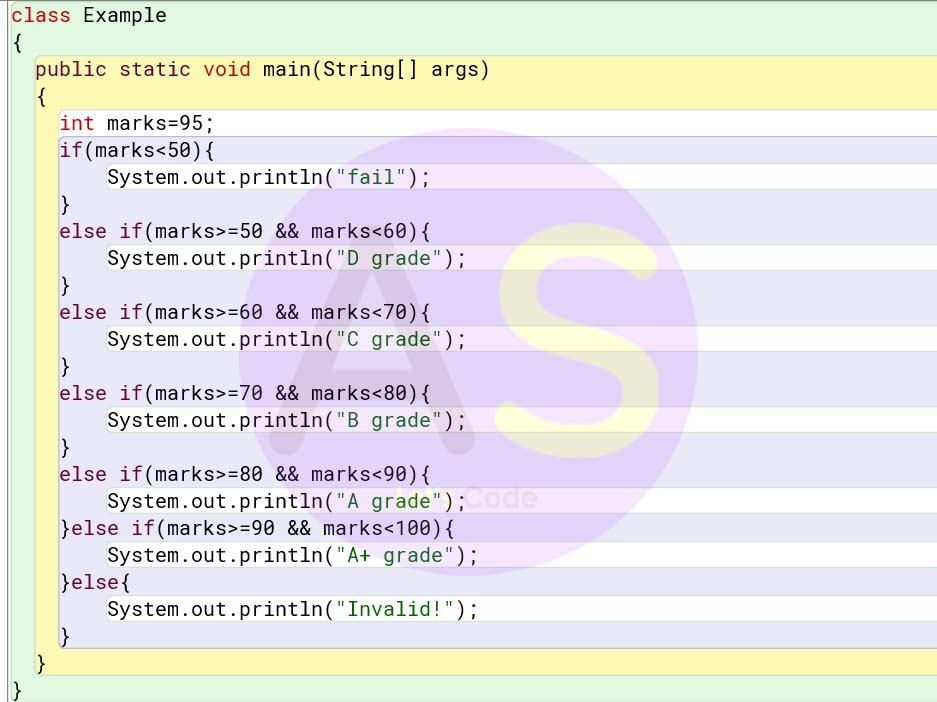
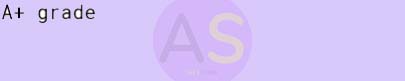
Switch-Case
Switch case is one of the conditional statement which is found in most of the programming languages
In this if we have more than one choices,and we have to select one of the choice then we use switch case.The
choice is selected based on the value of expression.The expression can be a byte,short,char and int
primitive datatype
It does not support the conditional operators(which are >,<,>=,<=,etc), like the if-else statement
can,nor
it
can handle
multiple variables
Syntax:
In the above syntax,when any of the case will be true,then the statement inside it will be executed and
break
statement will be applied.After that this process won't be executed further,it will move out of the
switch
case.
When condition does not match with any of the case,it will run default case.
Example:
Here "num" is the variable to be compared and if we input num as 3,then the output will be
Enter the number
3
Three
this means that case3 will be executed,after that this switch statment will be terminated.
3
Three
Fall-through in Switch case
In switch case,when there is no break commands mentioned in the particular case and cause execution of the
cases until it finds any break statement,then such statments is called fall through statement.
If there is no break statement till the end then default statements will be executed.
Let us understand this by an example:
In the above program ,if we input n as 1 then all the cases till default case will be executed.So the output
will be:
This is
the
fallthrough
Statement
the
fallthrough
Statement
Difference in switch and if-else statement
Switch case:
-
In switch case,it check only double assignment(==) operator,no other relational or logical operator
is used.
-
Here the datatypes that we can use in conditions are int and char only
If else:
-
In if-else statement,all the logical,relational and assignment operator is used
-
Here the datatype which can be used in condition are int,char,string,double,etc.
Menu-driven Programs
Example : 1 Write a menu driven program to print the area of different geometrical shapes as given below :
Choice
Shape
1
Right Triangle
2
Equilateral triangle
3
Square
4
Rectangle
5
Circle
import java.util.*;
class Menu
{
public static void main(String[] args)
{
int choice;
double area;
int l,b;
int a;
int r;
Scanner sc = new Scanner(System.in);
System.out.println("1- Area of Right Triangle");
System.out.println("2- Area of Equilateral Triangle");
System.out.println("3- Area of Square");
System.out.println("4- Area of Rectangle");
System.out.println("5- Area of Circle");
System.out.println("------------------------------");
System.out.print("Select your choice=");
choice = sc.nextInt();
switch (choice)
{
case 1:
System.out.print("Enter l=");
l=sc.nextInt();
System.out.print("Enter b=");
b=sc.nextInt();
area=(l*b)/2;
System.out.println("Area of Right Triangle="+area);
break;
case 2:
System.out.print("Enter a=");
a=sc.nextInt();
area=(a*a*Math.sqrt(3))/4;
System.out.println("Area of Equilateral Triangle="+area);
break;
case 3:
System.out.print("Enter a=");
a=sc.nextInt();
area=a*a;
System.out.println("Area of Square="+area);
break;
case 4:
System.out.print("Enter l=");
l=sc.nextInt();
System.out.print("Enter b=");
b=sc.nextInt();
area=l*b;
System.out.println("Area of Rectangle="+area);
break;
case 5:
System.out.print("Enter r=");
r=sc.nextInt();
area=r*r*22/7;
System.out.println("Area of Circle="+area);
break;
default:
System.out.print("Enter valid choice.");
}
}
}
Output :
1- Area of Right Triangle
2- Area of Equilateral Triangle
3- Area of Square
4- Area of Rectangle
5- Area of Circle
------------------------------
Select your choice=1
Enter l=12
Enter b=12
Area of Right Triangle=72.0
Example : 2 Write a menu driven program to print the corresponding week day as per the input number as given below :
No.
Day
1
Monday
2
Tuesday
3
Wednesday
4
Thursday
5
Friday
6
Saturday
7
Sunday
import java.util.*;
public class NameOfDay
{
public static void main(String[] args)
{
int day;
Scanner sc=new Scanner(System.in);
System.out.print("Enter day=");
day=sc.nextInt();
switch(day)
{
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Enter valid day.");
}
}
}
Output :
Enter day=5
Friday
Example : 3 Write a menu driven program to perform basic arithmetic operations as per the input number given below :
No.
Operation
1
Addition
2
Subtraction
3
Multiplication
4
Division
5
Quit
import java.util.*;
//Creating Class
class menu2 {
//Creating main method
public static void main(String[] args){
int a,b,c;
int choice;
Scanner scanner = new Scanner(System.in);
//Creating menu
System.out.println("Press 1 for Addition");
System.out.println("Press 2 for Subtraction");
System.out.println("Press 3 for Multiplication");
System.out.println("Press 4 for Division");
System.out.println("Press 5 to Quit\n \n ");
//Asking user to make choice
System.out.println("Make your choice");
choice = scanner.nextInt();
switch (choice) {
//First case for finding the addition
case 1:
System.out.println("Enter the first number ");
a = scanner.nextInt();
System.out.println("Enter the second number");
b = scanner.nextInt();
c = a + b;
System.out.println("The sum of the numbers is = " + c +"\n");
break;
//Second case for finding the difference
case 2:
System.out.println("Enter the first number ");
a = scanner.nextInt();
System.out.println("Enter the second number");
b = scanner.nextInt();
c = a - b;
System.out.println("The difference of the numbers is = " + c +"\n");
break;
//Third case for finding the product
case 3:
System.out.println("Enter the first number");
a = scanner.nextInt();
System.out.println("Enter the second number");
b = scanner.nextInt();
c = a * b;
System.out.println("The product of the numbers is = " + c + "\n");
break;
//Fourth case for finding the quotient
case 4:
System.out.println("Enter the first number");
a = scanner.nextInt();
System.out.println("Enter the second number");
b = scanner.nextInt();
c = a / b;
System.out.println("The quotient is = " + c +"\n");
break;
//Fifth case to quit the program
case 5:
System.exit(0);
//default case to display the message invalid choice made by the user
default:
System.out.println("Invalid choice!!! Please make a valid choice. \\n\\n");
}
}
}
Output :
Press 1 for Addition
Press 2 for Subtraction
Press 3 for Multiplication
Press 4 for Division
Press 5 to Quit
Make your choice
1
Enter the first number
12
Enter the second number
12
The sum of the numbers is = 24
Choice | Shape |
1 | Right Triangle |
2 | Equilateral triangle |
3 | Square |
4 | Rectangle |
5 | Circle |
import java.util.*; class Menu { public static void main(String[] args) { int choice; double area; int l,b; int a; int r; Scanner sc = new Scanner(System.in); System.out.println("1- Area of Right Triangle"); System.out.println("2- Area of Equilateral Triangle"); System.out.println("3- Area of Square"); System.out.println("4- Area of Rectangle"); System.out.println("5- Area of Circle"); System.out.println("------------------------------"); System.out.print("Select your choice="); choice = sc.nextInt(); switch (choice) { case 1: System.out.print("Enter l="); l=sc.nextInt(); System.out.print("Enter b="); b=sc.nextInt(); area=(l*b)/2; System.out.println("Area of Right Triangle="+area); break; case 2: System.out.print("Enter a="); a=sc.nextInt(); area=(a*a*Math.sqrt(3))/4; System.out.println("Area of Equilateral Triangle="+area); break; case 3: System.out.print("Enter a="); a=sc.nextInt(); area=a*a; System.out.println("Area of Square="+area); break; case 4: System.out.print("Enter l="); l=sc.nextInt(); System.out.print("Enter b="); b=sc.nextInt(); area=l*b; System.out.println("Area of Rectangle="+area); break; case 5: System.out.print("Enter r="); r=sc.nextInt(); area=r*r*22/7; System.out.println("Area of Circle="+area); break; default: System.out.print("Enter valid choice."); } } }
1- Area of Right Triangle 2- Area of Equilateral Triangle 3- Area of Square 4- Area of Rectangle 5- Area of Circle ------------------------------ Select your choice=1 Enter l=12 Enter b=12 Area of Right Triangle=72.0
No. | Day |
1 | Monday |
2 | Tuesday |
3 | Wednesday |
4 | Thursday |
5 | Friday |
6 | Saturday |
7 | Sunday |
import java.util.*; public class NameOfDay { public static void main(String[] args) { int day; Scanner sc=new Scanner(System.in); System.out.print("Enter day="); day=sc.nextInt(); switch(day) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; case 4: System.out.println("Thursday"); break; case 5: System.out.println("Friday"); break; case 6: System.out.println("Saturday"); break; case 7: System.out.println("Sunday"); break; default: System.out.println("Enter valid day."); } } }
Enter day=5 Friday
No. | Operation |
1 | Addition |
2 | Subtraction |
3 | Multiplication |
4 | Division |
5 | Quit |
import java.util.*; //Creating Class class menu2 { //Creating main method public static void main(String[] args){ int a,b,c; int choice; Scanner scanner = new Scanner(System.in); //Creating menu System.out.println("Press 1 for Addition"); System.out.println("Press 2 for Subtraction"); System.out.println("Press 3 for Multiplication"); System.out.println("Press 4 for Division"); System.out.println("Press 5 to Quit\n \n "); //Asking user to make choice System.out.println("Make your choice"); choice = scanner.nextInt(); switch (choice) { //First case for finding the addition case 1: System.out.println("Enter the first number "); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a + b; System.out.println("The sum of the numbers is = " + c +"\n"); break; //Second case for finding the difference case 2: System.out.println("Enter the first number "); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a - b; System.out.println("The difference of the numbers is = " + c +"\n"); break; //Third case for finding the product case 3: System.out.println("Enter the first number"); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a * b; System.out.println("The product of the numbers is = " + c + "\n"); break; //Fourth case for finding the quotient case 4: System.out.println("Enter the first number"); a = scanner.nextInt(); System.out.println("Enter the second number"); b = scanner.nextInt(); c = a / b; System.out.println("The quotient is = " + c +"\n"); break; //Fifth case to quit the program case 5: System.exit(0); //default case to display the message invalid choice made by the user default: System.out.println("Invalid choice!!! Please make a valid choice. \\n\\n"); } } }
Press 1 for Addition Press 2 for Subtraction Press 3 for Multiplication Press 4 for Division Press 5 to Quit Make your choice 1 Enter the first number 12 Enter the second number 12 The sum of the numbers is = 24