Class 12 ISC - Java Array Programs
Class 12th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 12th java topics includes revision of class 11th, constructors, user-defined methods, objects and classes, library classes , etc.
Program 1:
Design a class MatRev to reverse each element of a matrix.
Example:
Some of the members of the class are given below:
Class name: MatRev
Data members/instance variables:
arr[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
MatRev(int mm, int nn): parameterized constructor to initialise the data members m = mm and n = nn
void fillarray(): to enter elements in the array
int reverse(int x): returns the reverse of the number x
void revMat(MatRev P): reverses each element of the array of the parameterized object and stores it in the array of the current object
void show(): displays the array elements in matrix form
Define the class MatRev giving details of the constructor ( ), void fillarray (), int reverse(int), void revMat(MatRev) and void show().
Define the main () function to create objects and call the functions accordingly to enable the task
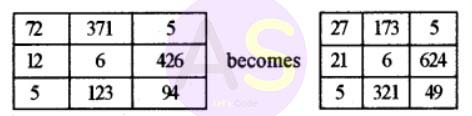
Solution:
import java.util.*; class MatRev { int arr[][]; int m; int n; public MatRev(int mm, int nn) { m=mm; n = nn; arr = new int[m][n]; } public void fillArray( ) { Scanner sc = new Scanner(System.in); System.out.println("Enter matrix elements:"); for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { arr[i][j] = sc.nextInt(); } } } public int reverse(int x) { int rev = 0; for(int i = x; i != 0; i /= 10) rev = rev * 10 + i % 10; return rev; } public void revMat(MatRev p) { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { arr[i][j] = reverse(p.arr[i][j]); } } } public void show() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(arr[i][j] + "\t"); } System.out.println(); } } public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter number of rows::"); int x = sc.nextInt(); System.out.print("Enter number of columns::"); int y = sc.nextInt(); MatRev obj1 = new MatRev(x, y); //parametrised object MatRev obj2 = new MatRev(x, y); // current calling object obj1.fillArray(); obj2.revMat(obj1); System.out.println("Original Matrix is::"); obj1.show(); System.out.println("Matrix with reversed elements"); obj2.show(); } }
Output :
Enter number of rows::3
Enter number of columns::3
Enter matrix elements:
12
34
56
89
67
87
35
65
49
Original Matrix is::
12 34 56
89 67 87
35 65 49
Matrix with reversed elements
21 43 65
98 76 78
53 56 94
Program 2:
Two matrices are said to be equal if they have the same dimension and their corresponding elements are equal.
For example, the two matrices A and B is given below are equal:
Design a class EqMat to check if two matrices are equal or not. Assume that the two matrices have the same dimension.
Some of the members of the class are given below :
Class name: EqMat
Data members/instance variables:
a[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
EqMat: parameterized constructor to initialise the data members m = mm and n = nn
void readArray(): to enter elements in the array
int check(EqMat P, EqMat Q): checks if the parameterized objects P and Q are equal and returns 1 if true, otherwise returns 0
void print(): displays the array elements
Define the class EqMat giving details of the constructor (), void readarray( ), int check(EqMat, EqMat) and void print( ).
Define the main( ) function to create objects and call the functions accordingly to enable the task.
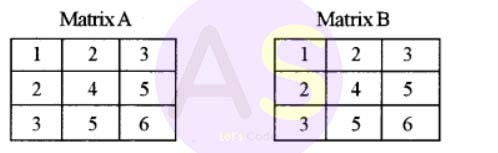
Solution:
import java.util.*; class EqMat{ int a[][]; int m; int n; public EqMat(int mm, int nn) { m = mm; n = nn; a = new int[m][n]; } public void readArray() { Scanner sc = new Scanner(System.in); for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { a[i][j] = sc.nextInt(); } } } public int check(EqMat p, EqMat q) { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { if(p.a[i][j] !=q.a[i][j]) { return 0; } } } return 1; } public void print() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(a[i][j] + "\t"); } System.out.println(); } } public static void main(String args[ ]) { Scanner sc = new Scanner(System.in); System.out.print("Enter no. of rows"); int r = sc.nextInt(); System.out.print("Enter no. of columns"); int c = sc.nextInt(); EqMat obj1 = new EqMat(r, c); EqMat obj2 = new EqMat(r, c); EqMat obj3 = new EqMat(r, c); System.out.println("Enter element for first matrix"); obj1.readArray(); System.out.println("Enter element for second matrix"); obj2.readArray(); System. out.println("First Matrix:"); obj1.print(); System.out.println("Second Matrix:"); obj2.print(); int res = obj3.check(obj1, obj2); if(res==1) System.out.println("Both matrices are equal"); else System.out.println("Both matrices are not equal"); } }
Enter no. of rows3
Enter no. of columns3
Enter element for first matrix
1
2
3
4
5
6
7
8
9
Enter element for second matrix
1
2
3
4
5
6
7
8
9
First Matrix:
1 2 3
4 5 6
7 8 9
Second Matrix:
1 2 3
4 5 6
7 8 9
Both matrices are equal
Program 3:
A class Adder has been defined to add any two accepted time.
Example:
Time A – 6 hours 35 minutes
Time B – 7 hours 45 minutes
Their sum is – 14 hours 20 minutes (where 60 minutes = 1 hour)
The details of the members of the class are given below:
Class name: Adder
Data member/instance variable:
a[]: integer array to hold two elements (hours and minutes)
Member functions/methods:
Adder (): constructor to assign 0 to the array elements
void readtime (): to enter the elements of the array
void addtime (Adder X, Adder Y): adds the time of the two parameterized objects X and Y and stores the sum in the current calling object
void disptime(): displays the array elements with an appropriate message (i.e., hours= and minutes=)
Specify the class Adder giving details of the constructor( ), void readtime( ), void addtime(Adder, Adder) and void disptime().
Define the main() function to create objects and call the functions accordingly to enable the task.
Solution:
import java.util.*; class Adder { int a[]; Adder() { a = new int[2]; } void readtime() { Scanner sc = new Scanner(System.in); System.out.println("Time:"); System.out.println("Enter hour:"); a[0] = sc.nextInt(); System.out.println("Enter minute:"); a[1] = sc.nextInt(); } void addtime(Adder X, Adder Y) { int hour1 = X.a[0]; int min1 = X.a[1]; int hour2 = Y.a[0]; int min2 = Y.a[1]; int hourSum = hour1 + hour2; int minSum = min1 + min2; if(minSum > 59) { a[0] = hourSum + 1; a[1] = minSum= minSum-60; } } void disptime() { System.out.println("Their sum is-"); System.out.println("hours =" + a[0] +" minutes =" + a[1]); } public static void main(String args[]){ Adder obj1 = new Adder(); Adder obj2 = new Adder(); Adder sumObj = new Adder(); obj1.readtime(); obj2.readtime(); sumObj.addtime(obj1, obj2); sumObj.disptime(); } }
Output :
Time:
Enter hour:
6
Enter minute:
35
Time:
Enter hour:
7
Enter minute:
45
Their sum is-
hours =14 minutes =20
Program 4:
A class Shift contains a two-dimensional integer array of order (m×n) where the maximum values of both m and n are 5.
Design the class Shift to shuffle the matrix (i.e. the first row becomes the last, the second row becomes the first and so on).
The details of the members of the class are given below:
Class name: Shift
Data member/instance variable:
mat[][]: stores the array element
m: integer to store the number of rows
n: integer to store the number of columns
Member functions/methods:
Shift(int mm, int nn): parameterized constructor to initialize the data members m=mm and n=nn
void input(): enters the elements of the array
void cyclic(Shift p): enables the matrix of the object (p) to shift each row upwards in a cyclic manner and store the resultant matrix in the current object
void display(): displays the matrix elements
Specify the class Shift giving details of the constructor(), void input(), void cyclic(Shift) and void display().
Define the main() function to create an object and call the methods accordingly to enable the task of shifting the array elements
Solution:
import java.util.*; class Shift { int mat[][]; int m; int n; public Shift(int mm, int nn){ m = mm; n = nn; mat = new int[m][n]; } public void input() { Scanner sc = new Scanner(System.in); for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(+i+", "+j+"th Element of the matrix :" ); mat[i][j] = sc.nextInt(); } } } public void cyclic(Shift p){ for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { if(i == 0) { mat[m-1][j] = p.mat[i][j]; } else { mat[i-1][j] = p.mat[i][j]; } } } } public void display() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(mat[i][j]+" \t"); } System.out.println(); } } public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("m = "); int r=sc.nextInt(); System.out.print("n = "); int c = sc.nextInt(); Shift obj1 = new Shift(r, c); Shift obj2 = new Shift(r, c); obj1.input(); System.out.println("Original Matrix :"); obj1.display(); obj2.cyclic(obj1); System.out.println("New Shifted Matrix is :"); obj2.display(); } }
Output :
m = 3
n = 3
0, 0th Element of the matrix :1
0, 1th Element of the matrix :2
0, 2th Element of the matrix :3
1, 0th Element of the matrix :4
1, 1th Element of the matrix :5
1, 2th Element of the matrix :6
2, 0th Element of the matrix :7
2, 1th Element of the matrix :8
2, 2th Element of the matrix :9
Original Matrix :
1 2 3
4 5 6
7 8 9
New Shifted Matrix is :
4 5 6
7 8 9
1 2 3
Program 5:
Design a class BinSearch to search for a particular value in an array.
Some of the members of the class are given below:
Classname : BinSearch
Data members/instance variables:
arr[] - to store integer elements
n - integer to store the size of the array
Member functions/methods:
BinSearch(int nn)- parametrised constructor to initialise n = nn
void fillarray() - input array elements
void sort() - sorts the array elements in ascending order using any standard technique.
int bin_search(int l,int u,int v) - searches for the value ‘v’ using binary search and recursive technique and returns its location if found otherwise returns -1
Define the class BinSearch giving details of the constructor( ), void fillarray( ), void sort( ) and int bin_search(int,int,int).
Define the main( ) function to create an object and call the functions accordingly to enable the task.
Solution:
import java.util.*; class BinSearch { int arr[]; int n; BinSearch(int nn) { n=nn; arr=new int[n]; } void fillarray() { Scanner sc = new Scanner(System.in); System.out.println("Enter elements"); for(int i =0;iarr[j+1]) { t=arr[j]; arr[j]=arr[j+1]; arr[j+1]=t; } } } } public int bin_search(int l,int u, int v ) { if(l<= u) { int mid =(l+u)/2; if(v==arr[mid]) { return mid; } if(v < arr[mid]) { return bin_search(l, mid-1, v); } else { return bin_search(mid+1, u, v); } } return -1; } public static void main(String args[]) { BinSearch obj = new BinSearch(5); obj.fillarray(); obj.sort(); int index = obj.bin_search(0,4,2); System.out.println(" location: " +index ); } }