Class 12 ISC- Java
Class 12th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 12th java topics includes revision of class 11th, constructors, user-defined methods, objects and classes, library classes , etc.
Single & Multi-Dimensional Arrays
Single-Dimensional Array
- An array is a collection of similar types of data having contiguous memory allocation.
- The indexing of the array starts from 0., i.e 1st element will be stored at the 0th index, 2nd
element at 1st index, 3rd at 2nd index, and so on.
- The size of the array can not be increased at run time therefore we can store only a fixed size of
elements in array.
-
Example of storing marks of 5 students :
Accessing array elements :
So , this is how array works:
int [] marks; ;⇛ Declaration!
marks = new int[5]; ⇛ Memory allocation!
int [] marks = new int[5]; ⇛ Declaration + Memory
allocation!
int [] marks = {100, 70, 80, 71, 98} ⇛ Declare +
Initialize!
Note : Array indices start from 0 and go till (n-1) where n is the size of the
array.
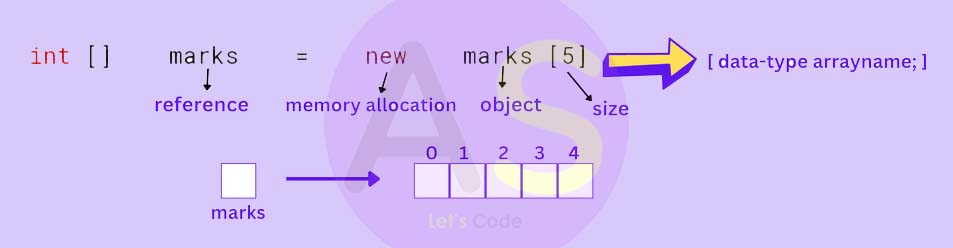
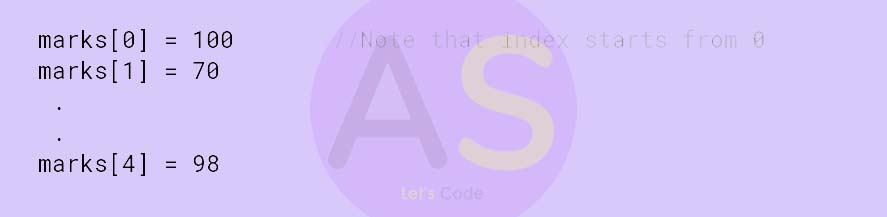
marks = new int[5]; ⇛ Memory allocation!
int [] marks = new int[5]; ⇛ Declaration + Memory allocation!
int [] marks = {100, 70, 80, 71, 98} ⇛ Declare + Initialize!
Note : Array indices start from 0 and go till (n-1) where n is the size of the
array.
Creating & Displaying Array
⇛ Creation of Array :
There are three main ways to create an array :
(i) Declaration and memory allocation
// int[] marks = new int[5];
(ii) Declaration and then memory allocation
int [] marks;
marks = new int[5];
Initialization
marks[0] = 100;
marks[1] = 60;
marks[2] = 70;
marks[3] = 90;
marks[4] = 86;
(iii) Declaration, memory allocation and initialization together
int[] marks = {98, 45, 79, 99, 80};
⇛ Displaying array :
An array can be displayed using a for loop:
marks = new int[5];
Initialization
marks[0] = 100;
marks[1] = 60;
marks[2] = 70;
marks[3] = 90;
marks[4] = 86;

Array Length
The array length is the number of elements that an array can hold. There is no predefined method to obtain
the length of an array. We can find the array length by using the array "length" property. The "length"
property determines the length of an array.
The length property can be invoked by using the dot (.) operator followed by the array name.
For Example :
int[] arr=new int[5];
int Length = arr.length;
In the above example, arr is an array of type int that can hold 5 elements. The Length is a variable that
stores the length of an array. To find the length of the array, we have used array name (arr) followed by
the dot operator (.) and length property, respectively. It determines the size of the array.
Example : 1 Write a program to store 10 numbers in an array and display their sum and average.
Output :
Example : 2 Write a program to enter elememts in an array and display them in reverse order.
Output :
Example : 3 Write a program to store the marks in three subject and print the marks of three students .
Output :
int Length = arr.length;
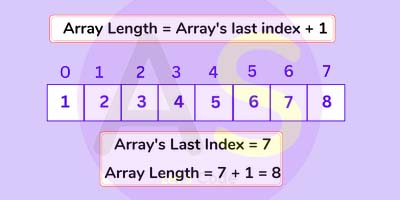
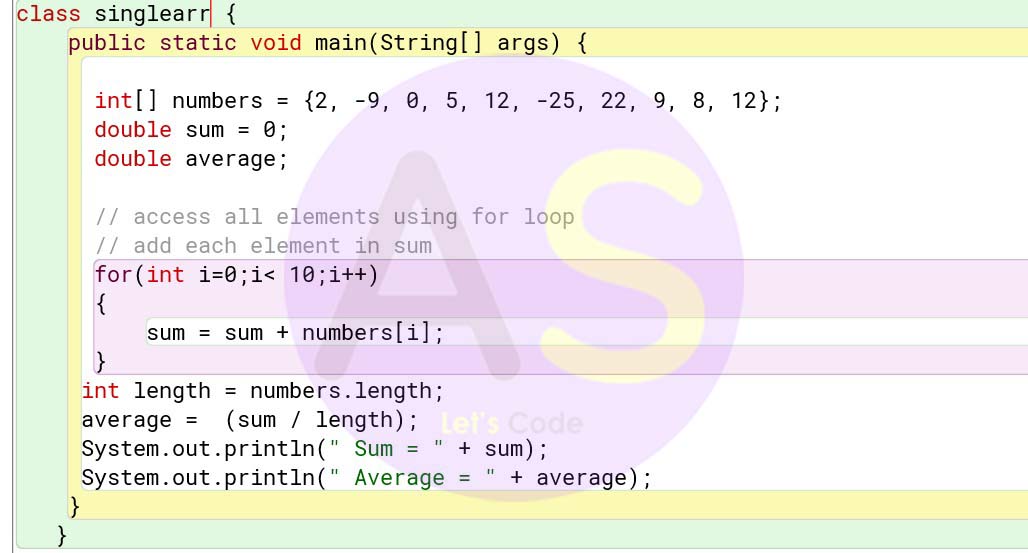
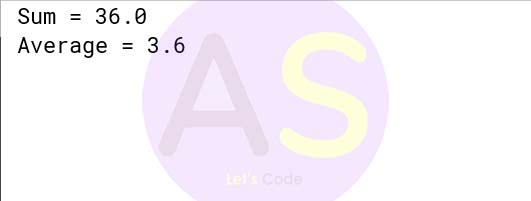
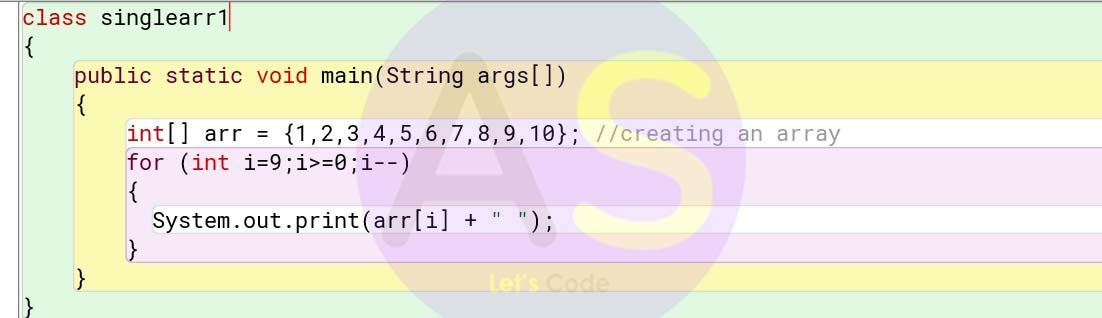
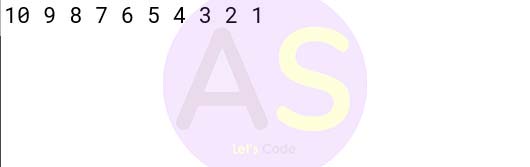
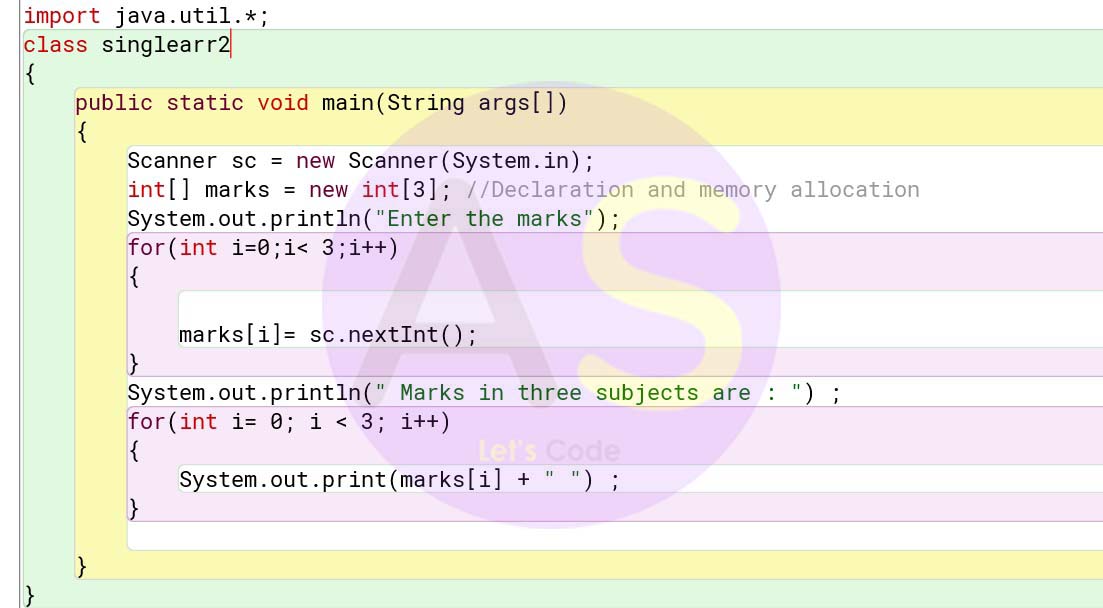
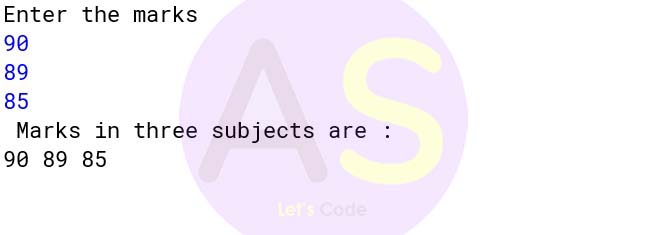
Double-Dimensional Array
A two-dimensional array is an array of arrays. Each element of a 2D array is an array itself. Data in 2D arrays are stored in tabular form (i.e. row and columns).
Accessing array elements :
So , this is how 2D array works:
int [][] flats; ;⇛ Declaration!
flats = new int[2][2]; ⇛ Memory allocation!
int [][] flats = new int[2][2]; ⇛ Declaration + Memory
allocation!
int flats [][] flats = {{1,2}, {3,4}} ⇛ Declare +
Initialize!
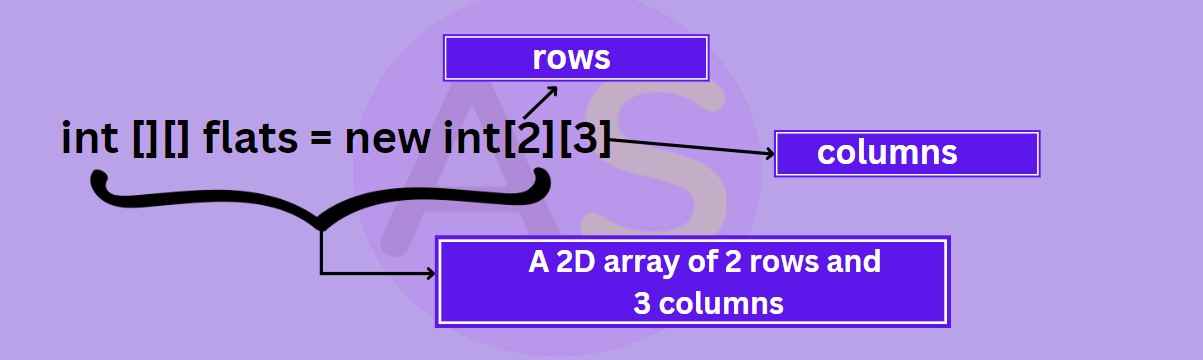
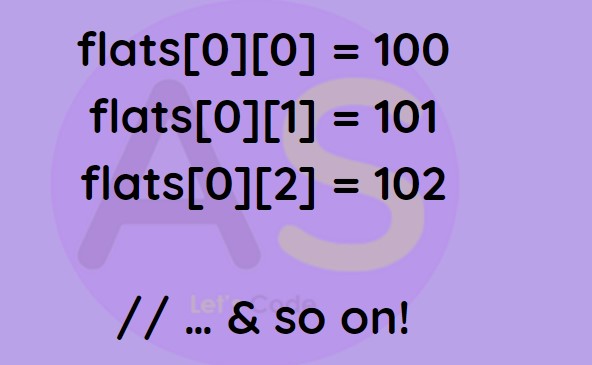
flats = new int[2][2]; ⇛ Memory allocation!
int [][] flats = new int[2][2]; ⇛ Declaration + Memory allocation!
int flats [][] flats = {{1,2}, {3,4}} ⇛ Declare + Initialize!
Creating & Displaying 2D Array
⇛ Creation of Array :
There are three main ways to create a 2D array :
(i) Declaration and memory allocation
// int[][] flats = new int[2][2];
(ii) Declaration and then memory allocation
int [][] flats;
flats = new int[2][2];
Initialization
flats[0][0] = 1;
flats[0][1] = 2;
flats[1][0] = 3;
flats[1][1] = 4;
(iii) Declaration, memory allocation and initialization together
int [][] flats = new int[2][2];
⇛ Displaying array :
A 2D array can be displayed using a nested for loop:
Example 1 (By Object Passing)
Design a class MatRev to reverse each element of a matrix.
Example:
Some of the members of the class are given below:
Class name: MatRev
Data members/instance variables:
arr[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
MatRev(int mm, int nn): parameterized constructor to initialise the data members m = mm and n = nn
void fillarray(): to enter elements in the array
int reverse(int x): returns the reverse of the number x
void revMat(MatRev P): reverses each element of the array of the parameterized object and stores it in the array of the current object
void show(): displays the array elements in matrix form
Define the class MatRev giving details of the constructor ( ), void fillarray (), int reverse(int), void revMat(MatRev) and void show().
Define the main () function to create objects and call the functions accordingly to enable the task
import java.util.*;
class MatRev
{
int arr[][];
int m;
int n;
public MatRev(int mm, int nn)
{
m=mm;
n = nn;
arr = new int[m][n];
}
public void fillArray( )
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter matrix elements:");
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
arr[i][j] = sc.nextInt();
}
}
}
public int reverse(int x)
{
int rev = 0;
for(int i = x; i != 0; i /= 10)
rev = rev * 10 + i % 10;
return rev;
}
public void revMat(MatRev p)
{
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
arr[i][j] = reverse(p.arr[i][j]);
}
}
}
public void show()
{
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.print("Enter number of rows::");
int x = sc.nextInt();
System.out.print("Enter number of columns::");
int y = sc.nextInt();
MatRev obj1 = new MatRev(x, y); //parametrised object
MatRev obj2 = new MatRev(x, y); // current calling object
obj1.fillArray();
obj2.revMat(obj1);
System.out.println("Original Matrix is::");
obj1.show();
System.out.println("Matrix with reversed elements");
obj2.show();
}
}
Output :
Enter number of rows::3
Enter number of columns::3
Enter matrix elements:
12
34
56
89
67
87
35
65
49
Original Matrix is::
12 34 56
89 67 87
35 65 49
Matrix with reversed elements
21 43 65
98 76 78
53 56 94
Example 2 (Without Object Passing)
Design a class MatRev to reverse each element of a matrix.
Example:
Some of the members of the class are given below:
Class name: MatRev
Data members/instance variables:
arr[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
MatRev(int mm, int nn): parameterized constructor to initialise the data members m = mm and n = nn
void fillarray(): to enter elements in the array
int reverse(int x): returns the reverse of the number x
void revMat(): reverses each element of the array and stores in it.
void show(): displays the array elements in matrix form
Define the class MatRev giving details of the constructor ( ), void fillarray (), int reverse(int), void revMat() and void show().
Define the main () function to create objects and call the functions accordingly to enable the task
import java.util.*;
class MatRev
{
int arr[][];
int m;
int n;
public MatRev(int mm, int nn)
{
m=mm;
n = nn;
arr = new int[m][n];
}
public void fillArray( )
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter matrix elements:");
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
arr[i][j] = sc.nextInt();
}
}
}
public int reverse(int x)
{
int rev = 0;
for(int i = x; i != 0; i /= 10)
rev = rev * 10 + i % 10;
return rev;
}
public void revMat()
{
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
arr[i][j] = reverse(arr[i][j]);
}
}
}
public void show()
{
for(int i = 0; i < m; i++)
{
for(int j = 0; j < n; j++)
{
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.print("Enter number of rows::");
int x = sc.nextInt();
System.out.print("Enter number of columns::");
int y = sc.nextInt();
MatRev obj1 = new MatRev(x, y);
obj1.fillArray();
System.out.println("Original Matrix is::");
obj1.show();
obj1.revMat();
System.out.println("Matrix with reversed elements");
obj1.show();
}
}
Output :
Enter number of rows::2
Enter number of columns::2
Enter matrix elements:
34
89
67
56
Original Matrix is::
34 89
67 56
Matrix with reversed elements
43 98
76 65
flats = new int[2][2];
Initialization
flats[0][0] = 1;
flats[0][1] = 2;
flats[1][0] = 3;
flats[1][1] = 4;
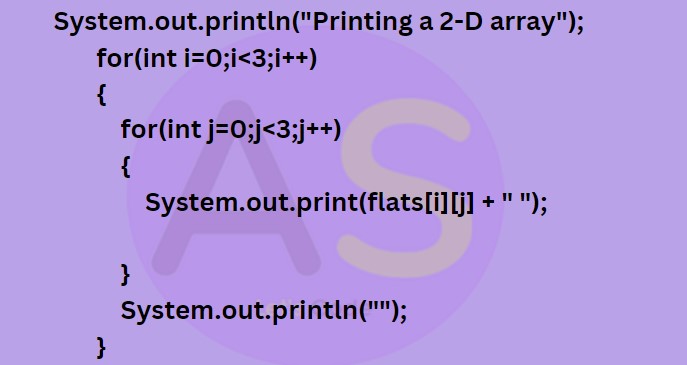
Design a class MatRev to reverse each element of a matrix.
Example:
Some of the members of the class are given below:
Class name: MatRev
Data members/instance variables:
arr[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
MatRev(int mm, int nn): parameterized constructor to initialise the data members m = mm and n = nn
void fillarray(): to enter elements in the array
int reverse(int x): returns the reverse of the number x
void revMat(MatRev P): reverses each element of the array of the parameterized object and stores it in the array of the current object
void show(): displays the array elements in matrix form
Define the class MatRev giving details of the constructor ( ), void fillarray (), int reverse(int), void revMat(MatRev) and void show().
Define the main () function to create objects and call the functions accordingly to enable the task
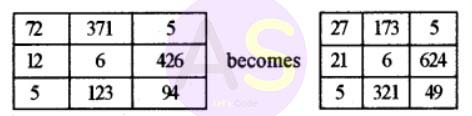
import java.util.*; class MatRev { int arr[][]; int m; int n; public MatRev(int mm, int nn) { m=mm; n = nn; arr = new int[m][n]; } public void fillArray( ) { Scanner sc = new Scanner(System.in); System.out.println("Enter matrix elements:"); for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { arr[i][j] = sc.nextInt(); } } } public int reverse(int x) { int rev = 0; for(int i = x; i != 0; i /= 10) rev = rev * 10 + i % 10; return rev; } public void revMat(MatRev p) { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { arr[i][j] = reverse(p.arr[i][j]); } } } public void show() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(arr[i][j] + "\t"); } System.out.println(); } } public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter number of rows::"); int x = sc.nextInt(); System.out.print("Enter number of columns::"); int y = sc.nextInt(); MatRev obj1 = new MatRev(x, y); //parametrised object MatRev obj2 = new MatRev(x, y); // current calling object obj1.fillArray(); obj2.revMat(obj1); System.out.println("Original Matrix is::"); obj1.show(); System.out.println("Matrix with reversed elements"); obj2.show(); } }
Enter number of rows::3 Enter number of columns::3 Enter matrix elements: 12 34 56 89 67 87 35 65 49 Original Matrix is:: 12 34 56 89 67 87 35 65 49 Matrix with reversed elements 21 43 65 98 76 78 53 56 94
Design a class MatRev to reverse each element of a matrix.
Example:
Some of the members of the class are given below:
Class name: MatRev
Data members/instance variables:
arr[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
MatRev(int mm, int nn): parameterized constructor to initialise the data members m = mm and n = nn
void fillarray(): to enter elements in the array
int reverse(int x): returns the reverse of the number x
void revMat(): reverses each element of the array and stores in it.
void show(): displays the array elements in matrix form
Define the class MatRev giving details of the constructor ( ), void fillarray (), int reverse(int), void revMat() and void show().
Define the main () function to create objects and call the functions accordingly to enable the task
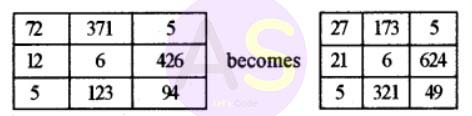
import java.util.*; class MatRev { int arr[][]; int m; int n; public MatRev(int mm, int nn) { m=mm; n = nn; arr = new int[m][n]; } public void fillArray( ) { Scanner sc = new Scanner(System.in); System.out.println("Enter matrix elements:"); for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { arr[i][j] = sc.nextInt(); } } } public int reverse(int x) { int rev = 0; for(int i = x; i != 0; i /= 10) rev = rev * 10 + i % 10; return rev; } public void revMat() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { arr[i][j] = reverse(arr[i][j]); } } } public void show() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(arr[i][j] + "\t"); } System.out.println(); } } public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter number of rows::"); int x = sc.nextInt(); System.out.print("Enter number of columns::"); int y = sc.nextInt(); MatRev obj1 = new MatRev(x, y); obj1.fillArray(); System.out.println("Original Matrix is::"); obj1.show(); obj1.revMat(); System.out.println("Matrix with reversed elements"); obj1.show(); } }