Code is copied!
Class 12 ISC - Java Recursion Questions
Class 12th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 12th java topics includes revision of class 11th, constructors, user-defined methods, objects and classes, library classes , etc.
Recursion Output Questions
Question 1:
The following function Mystery( ) is a part of some class.
What will the function Mystery( ) return when the value of
num = 43629, x = 3 and y = 4 respectively?
Show the dry run/working.
int Mystery (int num, int x, int y)
{
if(num < 10)
return num;
else
{
int z = num % 10;
if(z%2 == 0)
return z*x + Mystery (num/10, x, y);
else
return z*y + Mystery(num/10, x, y);
}
}
Solution:
Given n = 43629, x = 3, y = 4
Step 5 returns 4
Step 4 returns 12 + 4 = 16
Step 3 returns 18 + 16 = 34
Step 2 returns 6 + 34 = 40
Step 1 returns 36 + 40 = 76
Question 2:
The following function magicfun() is a part of some class.
What will the function magicfun() return,
when the value of n=7 and n=10, respectively?
Show the dry run/working:
int magicfun (int n)
{
if(n = = 0)
return 0;
else
return magicfun(n/2) * 10 + (n%2);
}
Solution:
At n = 7 ⇒ 111
At n = 10 ⇒ 1010
Binary equivalent of n
Question 3:
The following function Check( ) is a part of some class.
What will the function Check( )
return when the values of both ‘m’ and ‘n’ are equal to 5?
Show the dry run / working.
int Check (int m, int n)
{
if (n = = 1)
return − m − −;
else
return + + m + Check (m, − −n);
}
Solution:
Check(5,5)
(Step-1) 6 + Check (6,4)
(Step-2) 7 + Check(7,3)
(Step-3) 8 + Check(8,2)
(Step-4) 9 + Check (9,1)
(Step-5) -9
Hence the output = -9 + 9 + 8 + 7 + 6 = 21
Question 4:
The following function check( ) is a part of some class.
What will the function check( ) return when the value of :
(i) n = 25 and (ii) n = 10. Show the dry run/ working.
int check(int n)
{ if(n<=1)
return 1;
if( n%2==0)
return 1 + check(n/2);
else
return 1 + check(n/2 + 1);
}
Solution:
(i) check( 25 )
1+ check(12+1)
1+ check(6+1)
1+ check(3+1)
1+ check(2)
1+ check(1)
1
Final output: 6
(ii) check( 10 )
1+ check( 5 )
1+ check(2+1)
1+ check(1+1)
1+ check(1)
1
Final output: 5
Question 5:
What is the output of the program code given below,
when the value of x = 294?
int simple(int x)
{
return (x<=0)? 0: x + simple(x/10);
}
Solution:
The statement :
return ((x<)?0:x+simple(x/10));
calls itself recursively with x/10.
Hence the processing of x + simple(x/10) is calculated as
294 + 29 + 2 =325
Recursive Function Questions
Program 1:
A class CalSeries has been defined to calculate the sum of the series:
sum =1+ x+ x2 + x3 ....... + xn
Some of the members of the class are given below:
Class name: CalSeries
Data members/instance variables:
x : integer to store the value of x
n : integer to store the value of n
sum : integer to store the sum of the series
Member functions/methods:
CalSeries( ) : default constructor
void input( ) : to accept the values of x andn
int power(int p, int q) : return the power of p raised to q (p%) using recursive
technique
void cal( ) : calculates the sum of the series by invoking the method power() and displays the result with an appropriate message
Specify the class CalSeries, giving details of the constructor, void input( ), int power(int,int) and void cal( ).Define the main() function to create an object and call the member function accordingly to enable the task.
Solution:
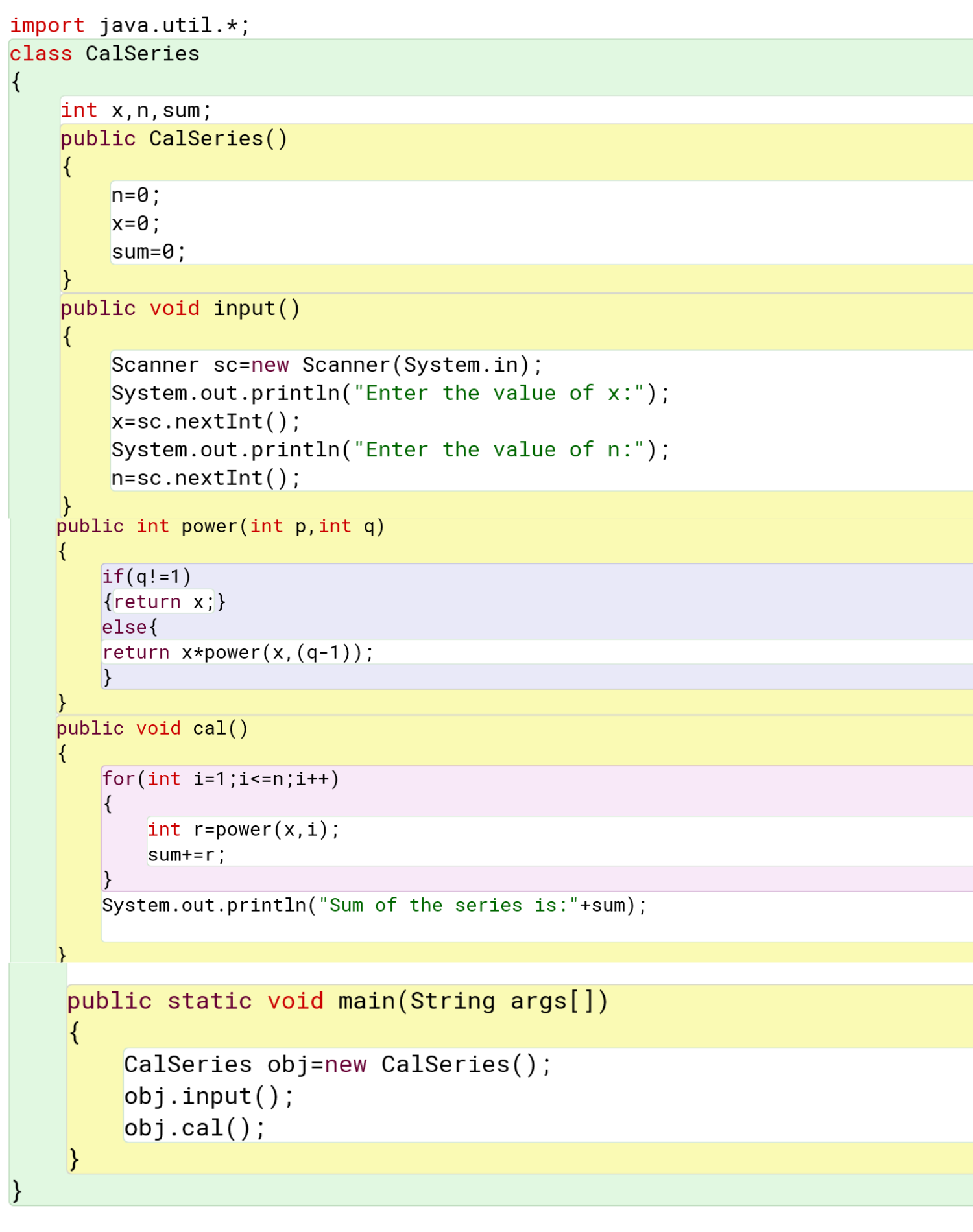
import java.util.*; class CalSeries { int x,n,sum; public CalSeries() { n=0; x=0; sum=0; } public void input() { Scanner sc=new Scanner(System.in); System.out.println("Enter the value of x:"); x=sc.nextInt(); System.out.println("Enter the value of n:"); n=sc.nextInt(); } public int power(int p,int q) { if(q!=1) {return x;} else{ return x*power(x,(q-1)); } } public void cal() { for(int i=1;i<=n;i++) { int r=power(x,i); sum+=r; } System.out.println("Sum of the series is:"+sum); } public static void main(String args[]) { CalSeries obj=new CalSeries(); obj.input(); obj.cal(); } }
Program 2:
Design a class Revno which reverse an integer number.
Example: 94765 becomes 56749 on reversing the digits of the number.
Some of the members of the class are given below:
Class name : Revno
Data member/instance variable:
num : to store the integer number
Member functions/methods:
Revno(): default constructor
void inputnum(): to accept the number
int reverse(int nn): returns the reverse of a number by using recursive technique
void display():displays the original number along with its reverse by invoking the method reverse()
Specify the class Revno, giving details of the constructor, void inputnum( ), int reverse(int) and void display().Define the main() function to create an object and call the functions accordingly to enable the task.
Solution:
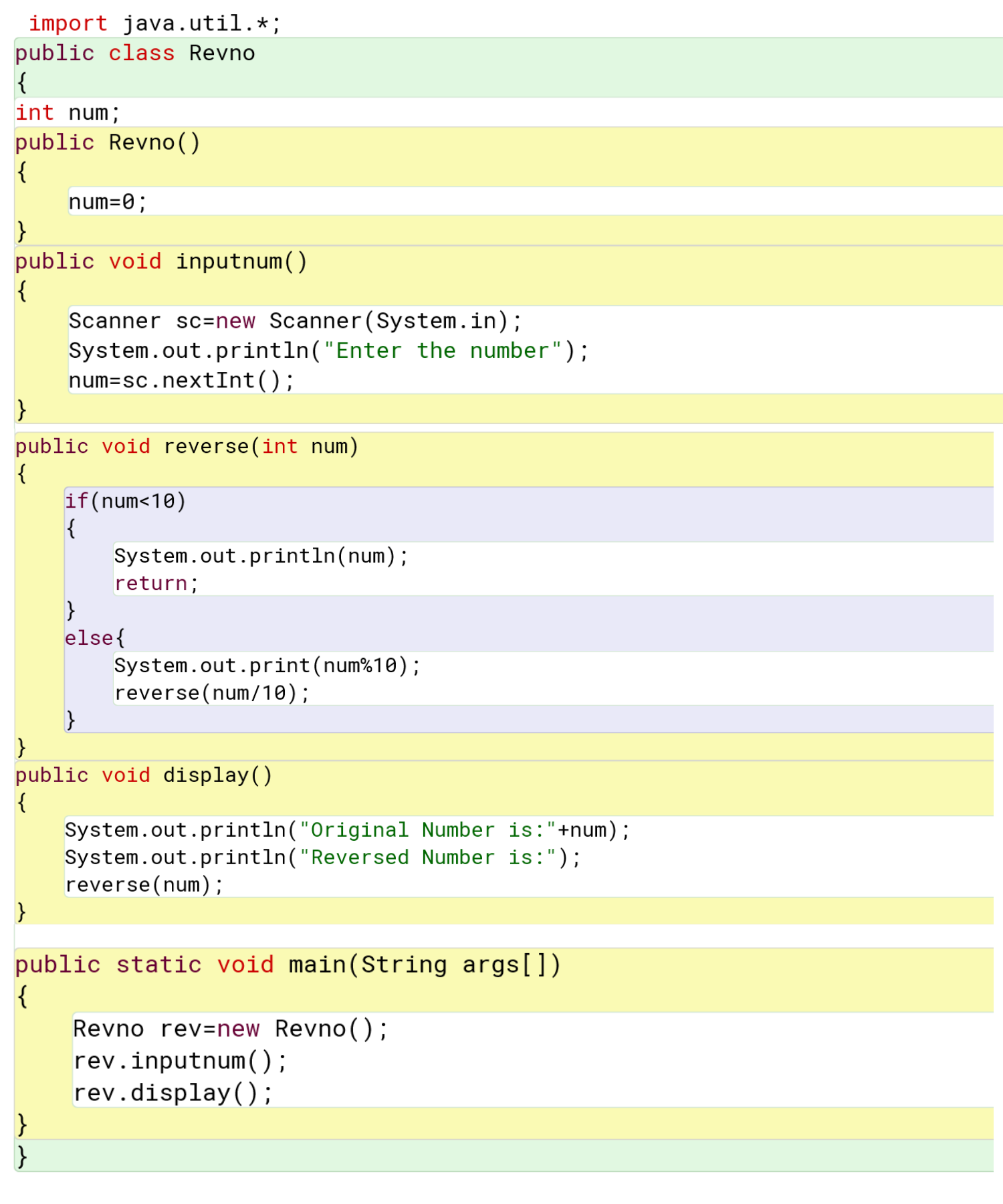
import java.util.*; public class Revno { int num; public Revno() { num=0; } public void inputnum() { Scanner sc=new Scanner(System.in); System.out.println("Enter the number"); num=sc.nextInt(); } public void reverse(int num) { if(num< 10) { System.out.println(num); return; } else{ System.out.print(num%10); reverse(num/10); } } public void display() { System.out.println("Original Number is:"+num); System.out.println("Reversed Number is:"); reverse(num); } public static void main(String args[]) { Revno rev=new Revno(); rev.inputnum(); rev.display(); } }
Program 3:
Design a class ArmNum to check if a given number is an Armstrong number or not. [A number is said to be Armstrong if sum of its digits raised to the power of length of the number is equal to the number]
Example : 371 = 33 + 73 + 13
1634 = 14 + 64 + 34 + 44
54748 = 55 + 45 + 75 + 45 + 85
Thus 371, 1634 and 54748 are all examples of Armstrong numbers.
Some of the members of the class are given below:
Class name : ArmNum
Data members/instance Variables:
n : to store the number
l : to store the length of the number
Methods/Member functions:
ArmNum (int nn): parameterized constructor to initialize the data member n=nn
int sum_pow(int i) : returns the sum of each digit raised to the power of the length of the number using recursive technique
eg. 34 will return 32 + 42 (as the length of the number is 2)
void isArmstrong( ) : checks whether the given number is an Armstrong number by invoking the function sum_pow() and displays the result with an appropriate message.
Specify the class ArmNum giving details of the constructor( ), int sum_pow(int) and void isArmstrong( ).Define a main( ) function to create an object and call the functions accordingly to enable the task.
Solution:
![Design a class ArmNum to check if a given number is an Armstrong number or not. [A number is said to be Armstrong if sum of its digits raised to the power of length of the number is equal to the number]](class12subchap/recursionprog/img3.png)
import java.util.*; public class Revno { int num; public Revno() { num=0; } public void inputnum() { Scanner sc=new Scanner(System.in); System.out.println("Enter the number"); num=sc.nextInt(); } public void reverse(int num) { if(num< 10) { System.out.println(num); return; } else{ System.out.print(num%10); reverse(num/10); } } public void display() { System.out.println("Original Number is:"+num); System.out.println("Reversed Number is:"); reverse(num); } public static void main(String args[]) { Revno rev=new Revno(); rev.inputnum(); rev.display(); } }
Program 4:
A class Palin has been defined to check whether a positive number is a Palindrome number or not.
The number ‘N’ is palindrome if the original number and its reverse are same. Some of the members of the class are given below :
Class Name : Palin
Data members/instance variables:
num : integer to store the number
revnum : integer to store the reverse of the number
Methods/Member functions :
Palin( ) : constructor to initialize data members with legal initial values
void accept( ): to accept the number
int reverse(int y): reverses the parameterized argument ‘y’ and stores it in ‘revnum’ using recursive technique
void check( ): Check whether the number is a Palindrome by invoking the
function reverse( ) and display the result with an appropriate message.
Specify the class Palin giving the details of the constructor(), void accept(), int reverse(int) and void check() . Define the main() function to create an object and call the functions accordingly to enable the task.
Solution:
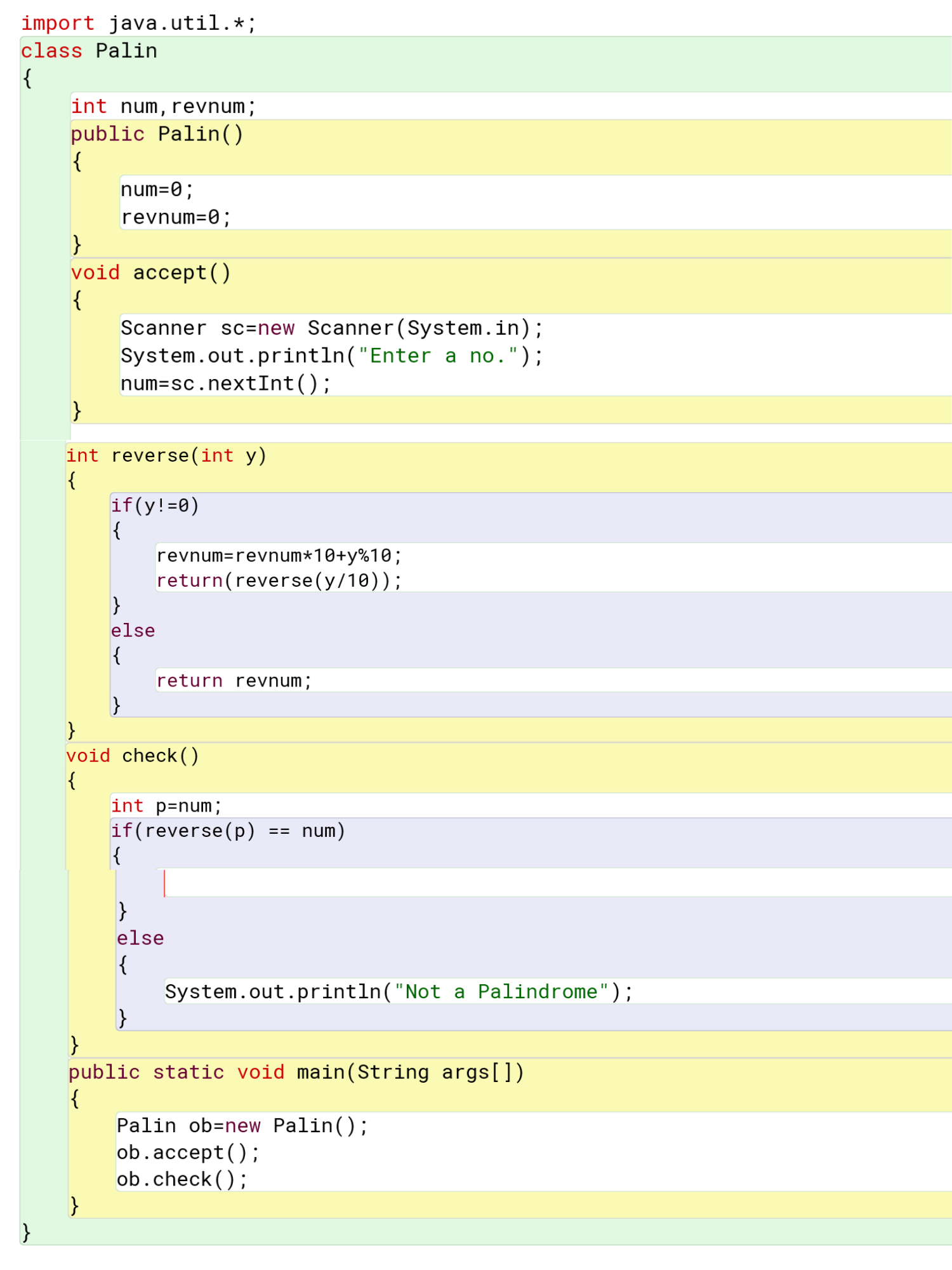
import java.util.*; class Palin { int num,revnum; public Palin() { num=0; revnum=0; } void accept() { Scanner sc=new Scanner(System.in); System.out.println("Enter a no."); num=sc.nextInt(); } int reverse(int y) { if(y!=0) { revnum=revnum*10+y%10; return(reverse(y/10)); } else { return revnum; } } void check() { int p=num; if(reverse(p) == num) { System.out.println("Palindrome"); } else { System.out.println("Not a Palindrome"); } } public static void main(String args[]) { Palin ob=new Palin(); ob.accept(); ob.check(); } }
Program 5:
A disarium number is a number in which the sum of the digits to the power of their respective position is equal to the number itself.
Example: 135 = 11 + 32 + 53
Hence, 135 is a disarium number.
Design a class Disarium to check if a given number is a disarium number or not. Some of the members of the class are given below:
Class name: Disarium
Data members/instance variables:
int num: stores the number
int size: stores the size of the number
Methods/Member functions:
Disarium (int nn): parameterized constructor to initialize the data members n = nn and size = 0
void countDigit(): counts the total number of digits and assigns it to size
int sumofDigits (int n, int p): returns the sum of the digits of the number(n) to the power of their respective positions (p) using recursive technique
void check(): checks whether the number is a disarium number and displays the result with an appropriate message
Specify the class Disarium giving the details of the constructor! ), void countDigit(), int sumofDigits(int, int) and void check(). Define the mainO function to create an object and call the functions accordingly to enable the task.
Solution:
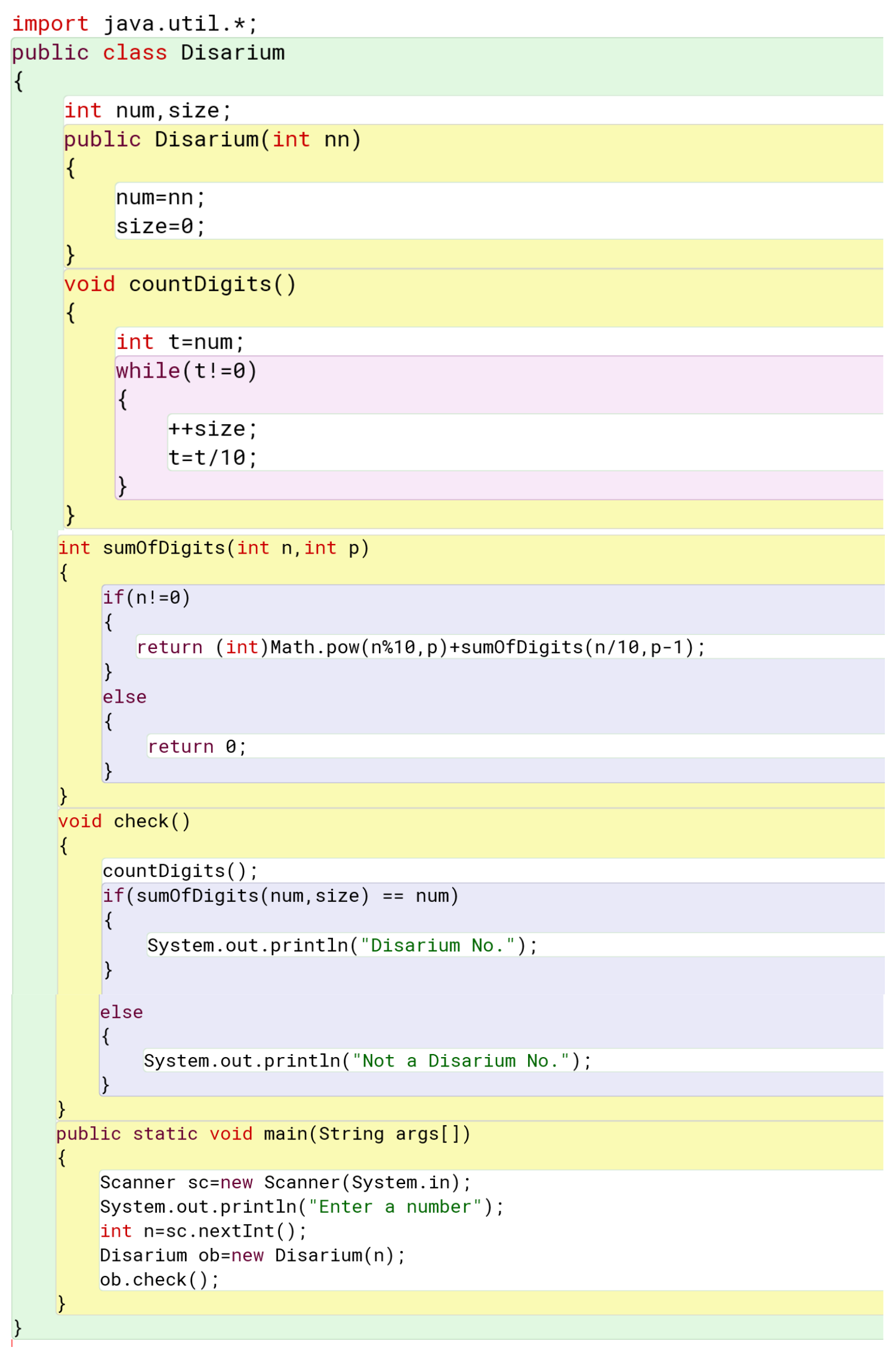
import java.util.*; public class Disarium { int num,size; public Disarium(int nn) { num=nn; size=0; } void countDigits() { int t=num; while(t!=0) { ++size; t=t/10; } } int sumOfDigits(int n,int p) { if(n!=0) { return (int)Math.pow(n%10,p)+sumOfDigits(n/10,p-1); } else { return 0; } } void check() { countDigits(); if(sumOfDigits(num,size) == num) { System.out.println("Disarium No."); } else { System.out.println("Not a Disarium No."); } } public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter a number"); int n=sc.nextInt(); Disarium ob=new Disarium(n); ob.check(); } }