Code is copied!
CLASS 12 ISC - Java Question Paper 2022
Section - A (7 marks)
(Attempt all questions)
Question 1
(i)
The concept in Java to perform method overloading and method overriding is called:
(a) inheritance
(b) polymorphism
(c) data abstraction
(d) encapsulation
Solution
(b) Polymorphism
(ii)
The interface in Java is a mechanism to achieve:
(a) encapsulation
(b) overriding
(c) polymorphism
(d) abstraction
Solution
(d) abstraction
(iii)
System.out.println("Computer Science".
lastIndexOf('e')):
The output of the statement given above will be:
(a) 8
(b) 7
(c) -1
(d) 15
Solution
(c) -1
(iv) Write a statement in Java to extract the last four characters from the word "SIMPLICITY".
Solution
System.out.println("SIMPLICITY".substring(6));
(v) Name a data structure that follows LIFO principle and one that follows FIFO principle.
Solution
LIFO architecture : Stack
FIFO architecture: Queue
(vi)
What is the output of the program code given below,
when the value of x = 294?
int simple(int x)
{ return (x<=0)? 0: x + simple(x/10);}
Solution
325
Explanation:
The statement
return (x<=0)? 0: x + simple(x/10); calls itself recursively with x/10. Hence the processing of x + simple(x/10) is calculated as 294 +29+2-325
(vii) State any one drawback of using recursive technique in a Java program
Solution
Disadvantages of recursion:
1. Recursive functions are generally slower than non-recursive function.
2. It may require a lot of memory space to hold intermediate results on the system stacks.
3. Hard to analyze or understand the code.
4. It is not more efficient in terms of space and time complexity.
Section - B (8 marks)
(Attempt all questions)
Question 2
Differentiate between finite recursion and infinite recursion.
Solution
Finite Recursion:
Finite Recursion occurs when the recursion terminates after a finite number of recursive calls.
A recursion terminates only when a base condition is met.
Infinite Recursion:
Infinite Recursion occurs when the recursion does
not terminate after a finite number of recursive calls. As the base condition is never met, the recursion carries on infinitely
Convert the following infix notation to prefix notation:
P*(Q- R) + S
Solution
+*P-QRS
Explanation:
P*(Q-R)+S
P*(-QR)+S
(*P-QR)+S
+*P-QRS
Answer the following questions from the diagram of a Binary Tree given below:
(i) State the siblings of the nodes C and E. Also, state the
predecessor node(s) and successor node(s) of node B
(ii) Write the in-order traversal of the left sub tree and pre-order traversal of the right sub tree.
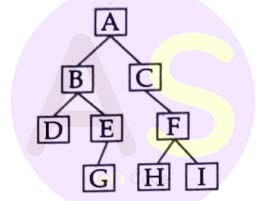
Solution
The above is the tree given
(i) Sibling of node C is B, sibling og node E is D, Predecessor of B is D and successor of B is E
(ii) Inorder traversal of Left subtree gjves:
DBGE
Preorder traversal of right subtree gives: CFHI
Explanation :
Nodes which belong to the same parent are called as siblings. In other words, nodes with the same parent are sibling nodes.
When you do the inorder traversal of a binary tree, the neighbors of given node are called Predecessor(the node lies behind of given node) and Successor (the node lies ahead of given node)
Inorder traversal is Left → Root → Right
Preorder traversal is Root → Left → Right
SECTION B
(Attempt all questions from this Section.)
Question 5
Design a class Unique, which checks whether a word begins and ends with a vowel.
Example: APPLE, ARORA etc.
The details of the members of the class are given below:
Class name :Unique
Data members/instance variables:
word:stores a word
len:to store the length of the word
Methods/Member functions:
Unique():default constructor
void aceptword():to accept the word in UPPERCASE
boolean checkUnique(): checks and returns 'true' if the word starts and ends with a vowel otherwise returns 'false'
void display(): displays the word along with an appropriate message
Specify the class lInique, giving details ofthe constructor,void acceptword(), boolean checkUnique() and void display(). Define the main() function to create an object and call the functions accordingly to enable the task
Solution:
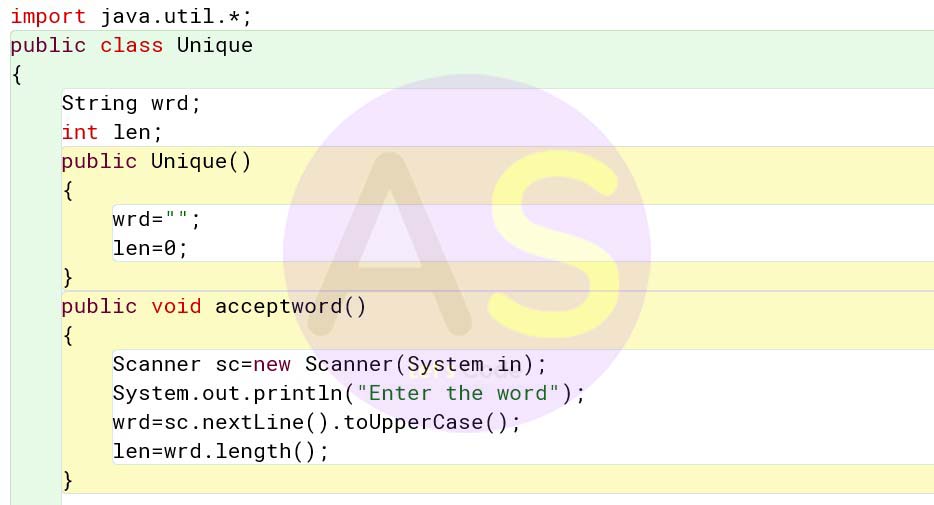
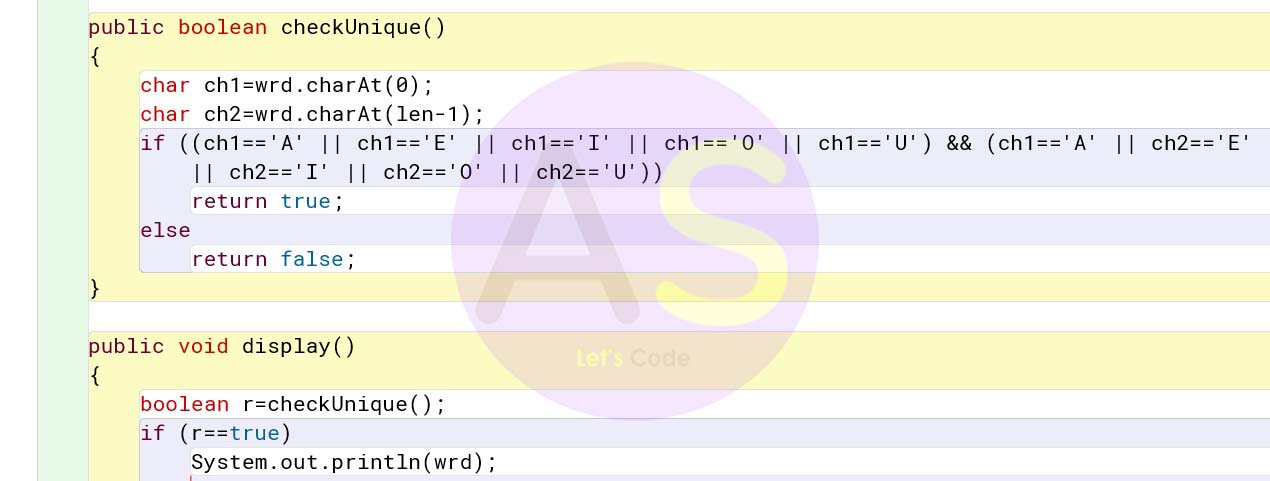
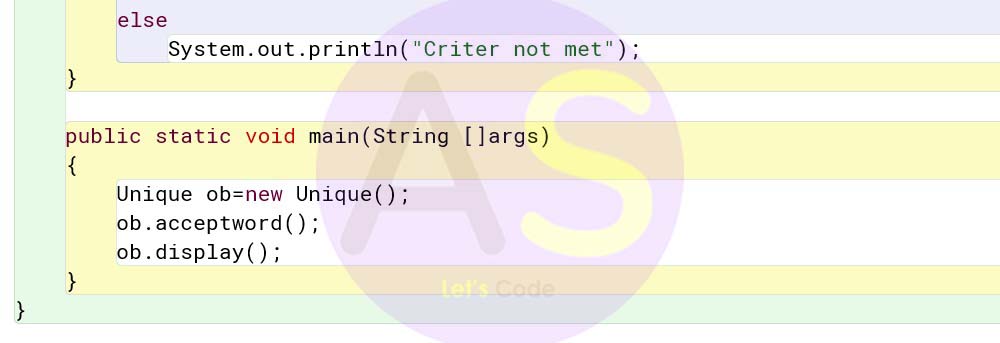
import java.util.*; public class Unique { String wrd; int len; public Unique() { wrd=""; len=0; } public void acceptword() { Scanner sc=new Scanner(System.in); System.out.println("Enter the word"); wrd=sc.nextLine().toUpperCase(); len=wrd.length(); } public boolean checkUnique() { char ch1=wrd.charAt(0); char ch2=wrd.charAt(len-1); if ((ch1=='A' || ch1=='E' || ch1=='I' || ch1=='O' || ch1=='U') && (ch1=='A' || ch2=='E' || ch2=='I' || ch2=='O' || ch2=='U')) return true; else return false; } public void display() { boolean r=checkUnique(); if (r==true) System.out.println(wrd); else System.out.println("Criter not met"); } public static void main(String []args) { Unique ob=new Unique(); ob.acceptword(); ob.display(); } }
Question 6
A class CalSeries has been defined to calculate the sum of the series:
sum =1+ x+ x2 + x3 ....... + xn
Some of the members of the class are given below:
Class name: CalSeries
Data members/instance variables:
x : integer to store the value of x
n : integer to store the value of n
sum : integer to store the sum of the series
Member functions/methods:
CalSeries( ) : default constructor
void input( ) : to accept the values of x and n
int power(int p, int q) : return the power of p raised to q (p%) using recursive
technique
void cal( ) : calculates the sum of the series by invoking the method power() and displays the result with an appropriate message
Specify the class CalSeries, giving details of the constructor, void input( ), int power(int,int) and void cal( ).Define the main() function to create an object and call the member function accordingly to enable the task.
Solution:
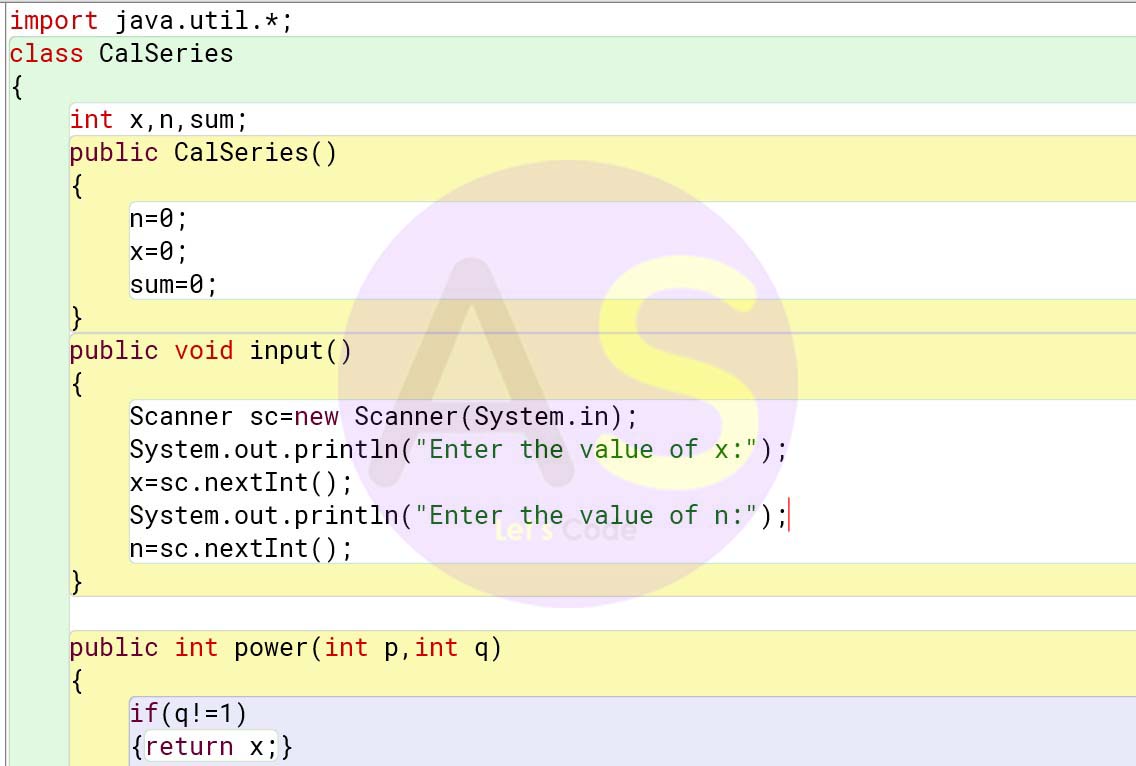
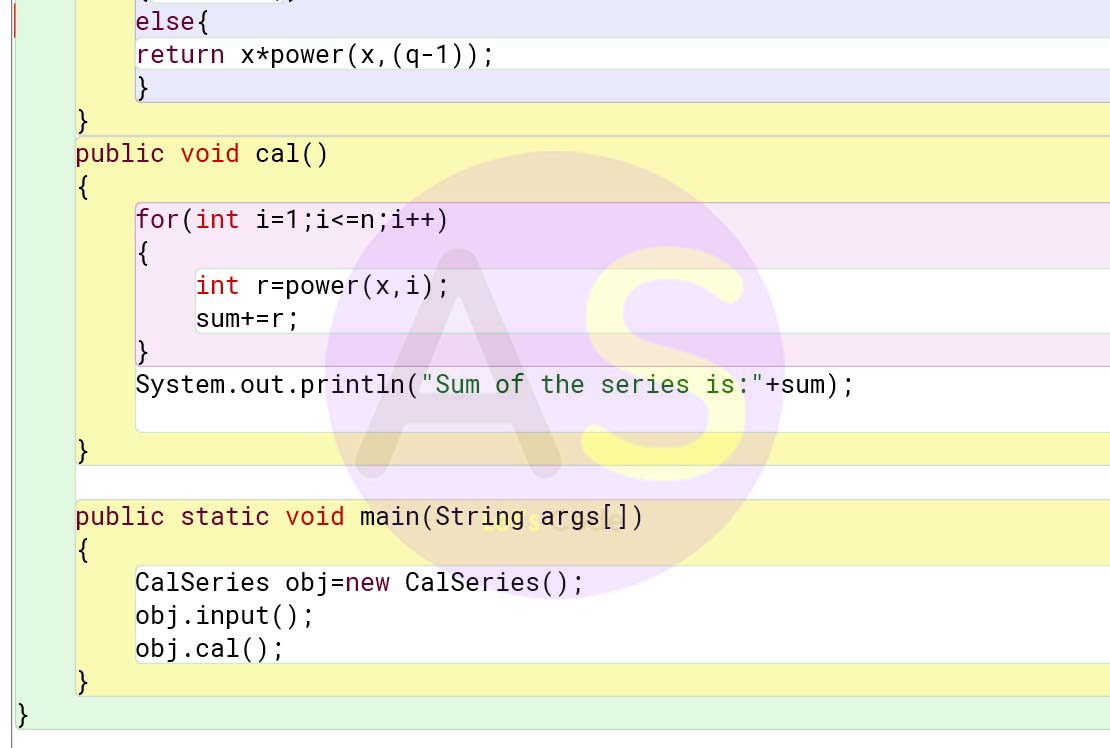
import java.util.*; class CalSeries { int x,n,sum; public CalSeries() { n=0; x=0; sum=0; } public void input() { Scanner sc=new Scanner(System.in); System.out.println("Enter the value of x:"); x=sc.nextInt(); System.out.println("Enter the value of n:"); n=sc.nextInt(); } public int power(int p,int q) { if(q!=1) {return x;} else{ return x*power(x,(q-1)); } } public void cal() { for(int i=1;i<=n;i++) { int r=power(x,i); sum+=r; } System.out.println("Sum of the series is:"+sum); } public static void main(String args[]) { CalSeries obj=new CalSeries(); obj.input(); obj.cal(); } }
Question 7
Design a class Revno which reverse an integer number.
Example: 94765 becomes 56749 on reversing the digits of the number.
Some of the members of the class are given below:
Class name : Revno
Data member/instance variable:
num : to store the integer number
Member functions/methods:
Revno(): default constructor
void inputnum(): to accept the number
int reverse(int nn): returns the reverse of a number by using recursive technique
void display():displays the original number along with its reverse by invoking the method reverse()
Specify the class Revno, giving details of the constructor, void inputnum( ), int reverse(int) and void display().Define the main() function to create an object and call the functions accordingly to enable the task.
Solution:
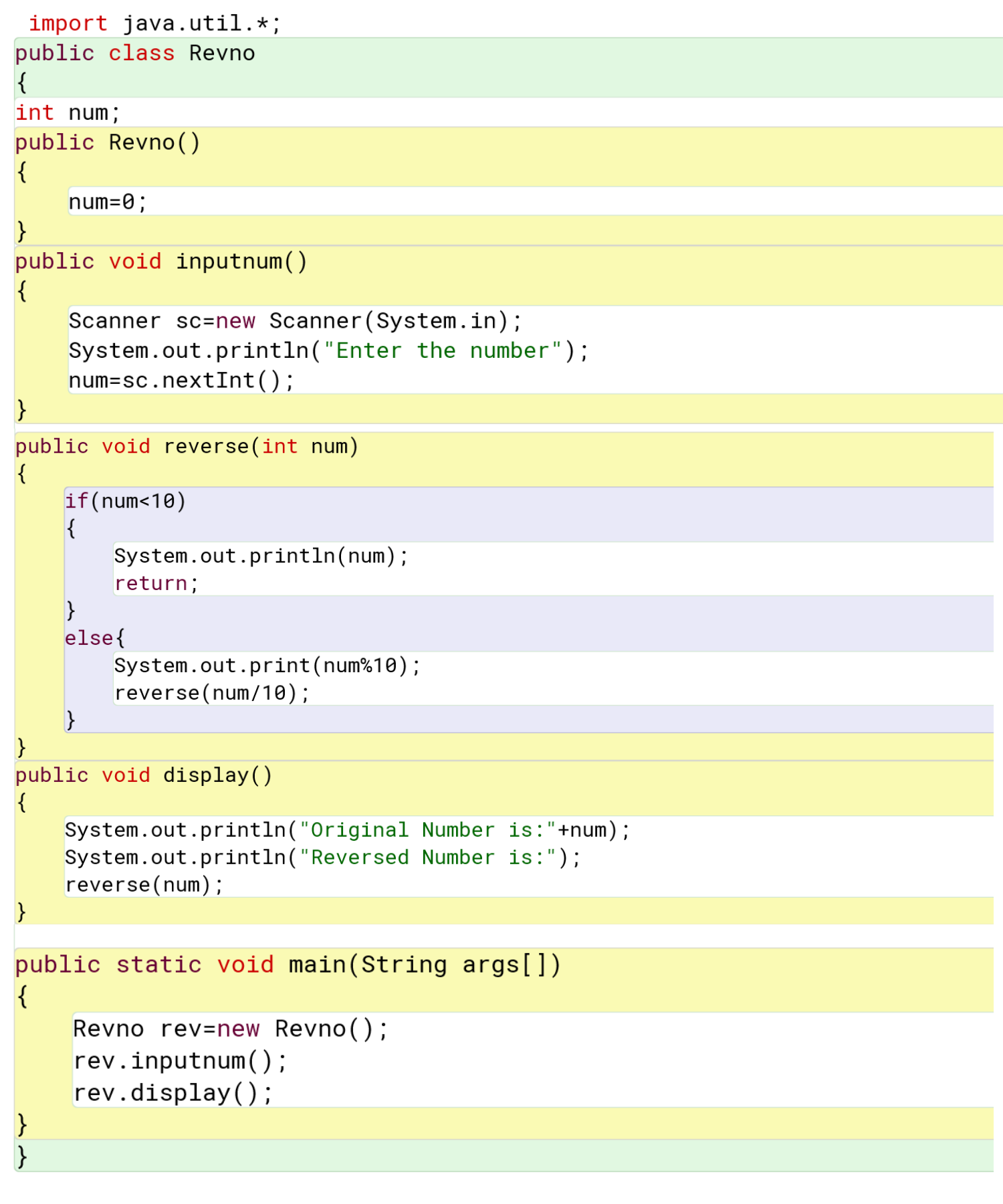
import java.util.*; public class Revno { int num; public Revno() { num=0; } public void inputnum() { Scanner sc=new Scanner(System.in); System.out.println("Enter the number"); num=sc.nextInt(); } public void reverse(int num) { if(num< 10) { System.out.println(num); return; } else{ System.out.print(num%10); reverse(num/10); } } public void display() { System.out.println("Original Number is:"+num); System.out.println("Reversed Number is:"); reverse(num); } public static void main(String args[]) { Revno rev=new Revno(); rev.inputnum(); rev.display(); } }
Question 8
A Super class Item has been defined to store the details of an item sold by a wholesaler to a retailer.Define a subclass Taxable to compute the total amount paid by retailer along with service tax.
Some of the members of both the classes are given below:
Class name:Item
Data member/instance variable:
name:to store the name of the item
code:integer to store the item code
amount:stores the total sale amount of the item (in decimals)
Member functions/methods:
Item (....): parameterized Constructor to assign value to the data members
void show( ) :to display the item detail
Class name:Sales
Data member/instance variable:
tax:to store the service tax (in decimals)
totamt: to store the total amount (in decimals)
Member functions/methods:
Taxable(…):parameterized constructor to assign values to data members of both the classes
void compute( ):calculates the service tax @ 10.2% of the actual sale amount
calculates the total amount paid by the retailer as (total sale amount + service tax)
void show( ): to display the item details along with the total amount
Assume that the super class Item has been defined. Using the concept of inheritance, specify the class Taxable giving the details of the constructor( …), void cal_tax( ) and void show( ).
The super class, main function and algorithm need NOT be written.
Solution:
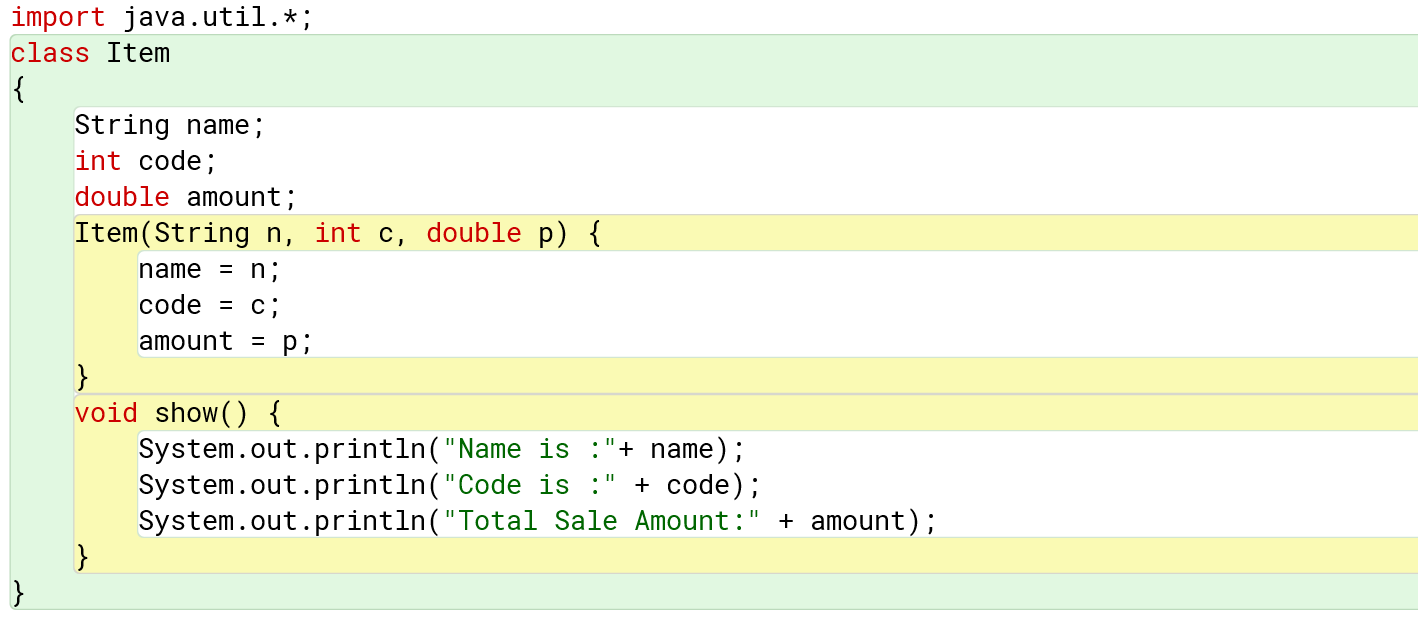
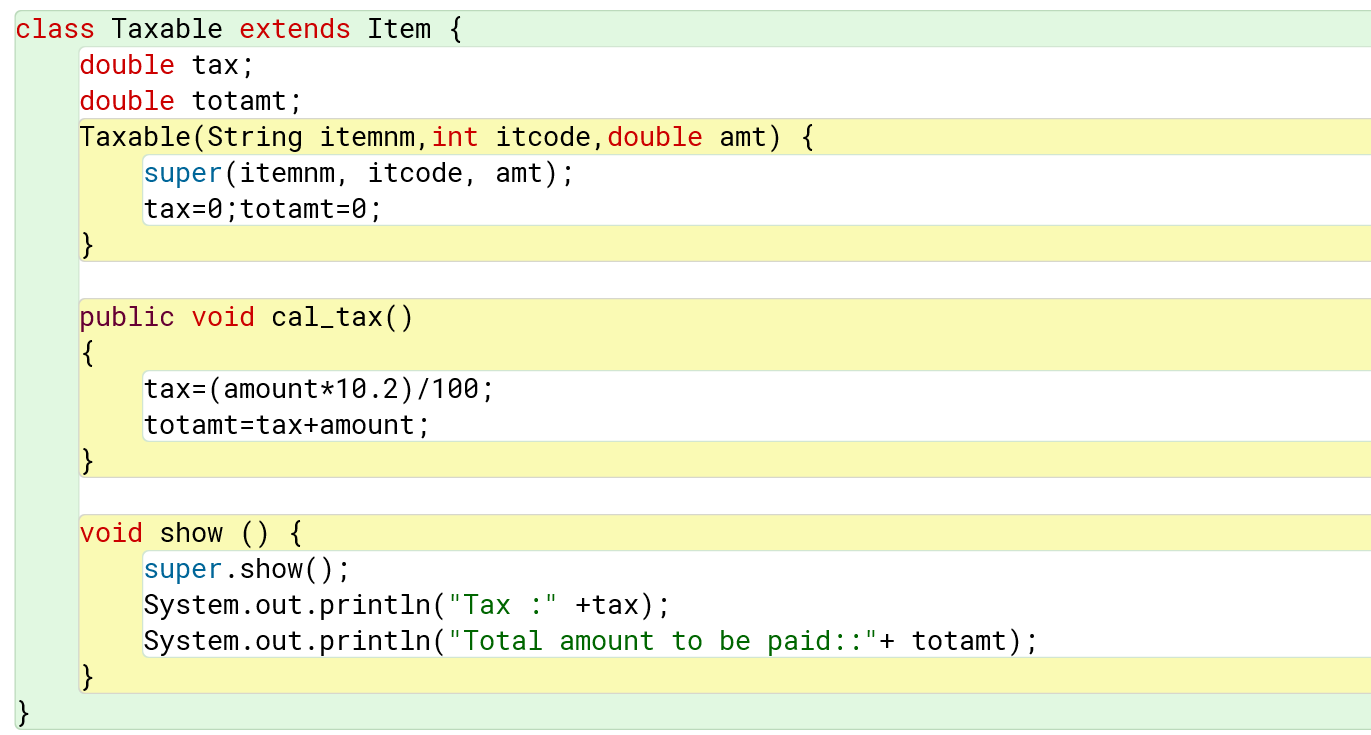
import java.util.*; class Item { String name; int code; double amount; Item(String n, int c, double p) { name = n; code = c; amount = p; } void show() { System.out.println("Name is :"+ name); System.out.println("Code is :" + code); System.out.println("Total Sale Amount:" + amount); } } class Taxable extends Item { double tax; double totamt; Taxable(String itemnm,int itcode,double amt) { super(itemnm, itcode, amt); tax=0;totamt=0; } public void cal_tax() { tax=(amount*10.2)/100; totamt=tax+amount; } void show () { super.show(); System.out.println("Tax :" +tax); System.out.println("Total amount to be paid::"+ totamt); } }
Question 9
A stack is a kind of data structure which can store elements with the restriction that an element can be added or removed from the top only.
The details of the class Stack is given below:
Class name: Stack
Data members/instance variables:
cha[]: the array to hold the names
size: the maximum capacity of the string array
top: the index of the topmost element of the stack
Member functions:
Stack(int mm): Constructor to initialize the the digits data member size = mm, top= -1 and create the character array
void push_char(char v): to add characters from the top end if possible else display the message("Stack full")
char pop_char(): to remove and return characters from the top end, if any, else return '$'
void display(): Display the elements of the stack
Specify the class Stack giving the details of void push_char(char) and char pop_char( ). Assume that the other functions have been defined
THE MAIN() FUNCTION AND ALGORITHM NEED NOT BE WRITTEN
Solution:
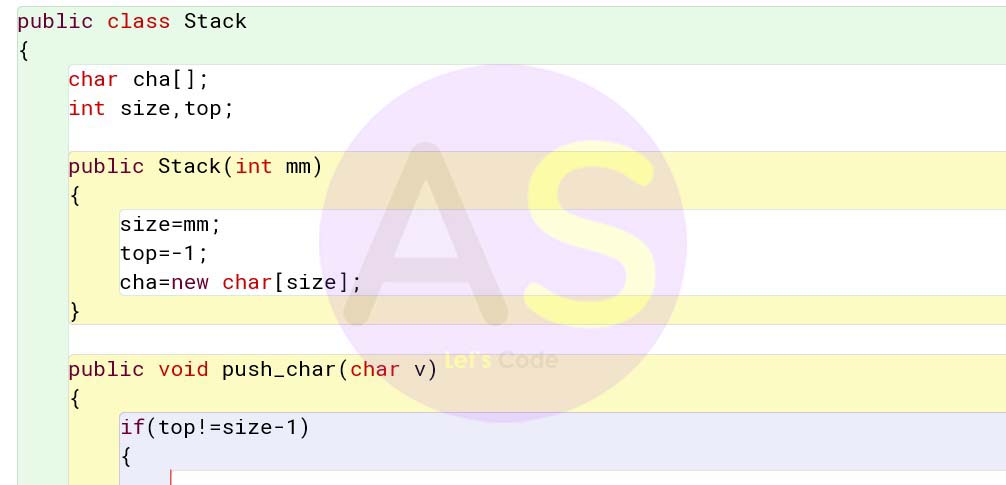
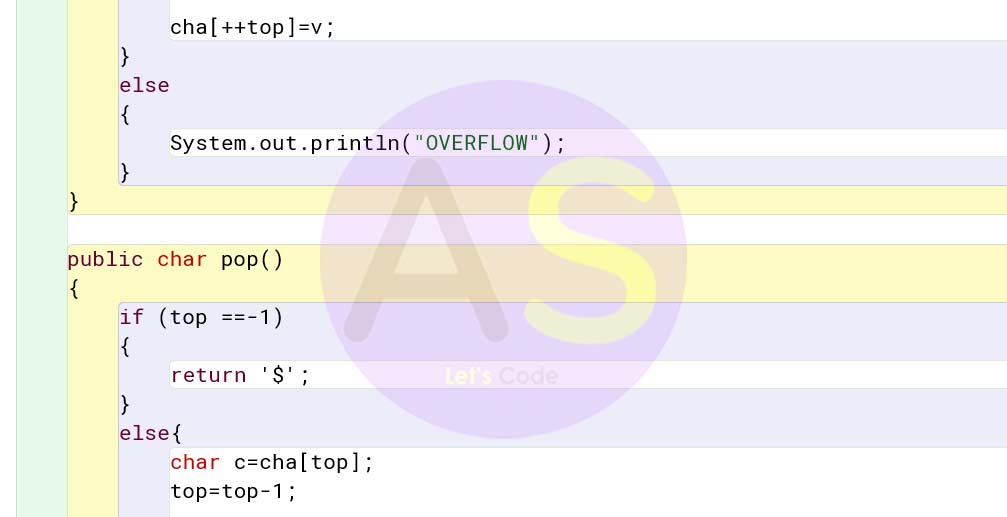
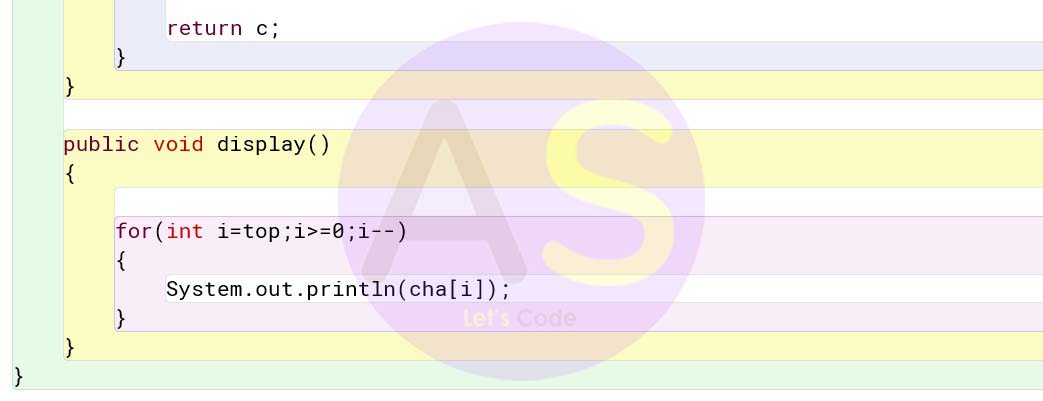
public class Stack { char cha[]; int size,top; public Stack(int mm) { size=mm; top=-1; cha=new char[size]; } public void push_char(char v) { if(top!=size-1) { cha[++top]=v; } else { System.out.println("OVERFLOW"); } } public char pop() { if (top ==-1) { return '$'; } else{ char c=cha[top]; top=top-1; return c; } } public void display() { for(int i=top;i>=0;i--) { System.out.println(cha[i]); } } }