Code is copied!
CLASS 12 ISC - Java Question Paper 2020
PART I
(Attempt all questions)
Question 1
(a) State the properties of zero in Boolean algebra.
Solution
Properties of zero: A + 0 = A and A • 0 = 0
(b)
Find the complement of the following Boolean expression using De Morgan’s law:
F(P,Q,R) = P + (Q′ • R)
Solution
F(P,Q,R) = P + (Q' • R)
= ( P + (Q’ • R) )’
= P’ • (Q’ • R )’
= P’ • ( Q + R’)
(c)
Find the dual of: (A′ + 0) • (B′ + 1) = A′
Solution
Dual = A’•1 + B’• 0 = A’
(d)
State whether the following proposition is a tautology, contradiction or a contingency:
F = (P => Q) V (Q => ~P)
Solution
F = (P→Q) + (Q→P)
= P' + Q + Q' + P'
= P' + 1 = 1
Hence, it is a TAUTOLOGY
(e)
Study the diagram given below and answer the questions that follow:
(i) Name the basic gate which is represented by the diagram.
(ii) What will be the value of X when A=1 and B=0 ?
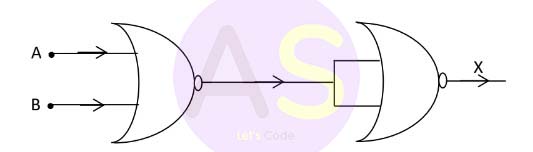
Solution
(i) OR gate
(ii) 1
(a)
State the difference between a Binary Tree structure and a single Linked List.
Solution
Binary tree | Single Linked list |
---|---|
It is a recursive data structure | It is a linear data structure |
It allocates memory randomly non sequentially | It allocates memory sequently |
(b) A matrix B[10][20] is stored in the memory with each element requiring 2 bytes of storage. If the base address at B[2][1] is 2140, find the address of B[5][4] when the matrix is stored in Column Major Wise.
Solution
B[i][j] = BA + W [ (j – lc)* row + (i – lr]
= 2140 + 2[ (4-1) * 10 + (5-2)]
= 2140 + 2[ 33 ]
= 2140 + 66
= 2206
(c)
Convert the following infix notation to prefix form:
(X + Y) / (Z * W / V)
Solution
Infix to Prefix : (X + Y) / (Z * W / V)
= +XY / ( * Z W / V )
= +XY / /*ZWV
= /+XY/*ZWV
= /+XY/*ZWV
(d) State the best case and the worst case complexity for bubble sort algorithm.
Solution
O(n): Best case for bubble sort algorithm.
O(n2 ): Worst case for bubble sort algorithm.
(e) What is the significance of the keyword ‘new’ in Java? Mention the areas where it is used.
Solution
‘new’ is a dynamic memory allocation operator, which is used to allot memory to non- primitive data types (example: class, array etc.). Areas of use: To declare an object, to declare an array.
The following function check( ) is a part of some class. What will the function check( )
return when the value of (i) n=25 and (ii) n=10. Show the dry run/ working.
check( 25 )
int check(int n) { if(n<=1) return 1; if( n%2==0) return 1 + check(n/2); else return 1 + check(n/2 + 1); }
Solution
check( 25 )
1+ check(12+1)
1+ check(6+1)
1+ check(3+1)
1+ check(2)
1+ check(1)
1
Output: (i) 1+1+1+1+1+1 = 6
(ii) check( 10 )
1+ check( 5 )
1+ check(2+1)
1+ check(1+1)
1+ check(1)
1
Output: (ii) 1+1+1+1+1 = 5
PART II
SECTION A
(Attempt any two questions from this Section.)
Question 4
(a) Given the Boolean function: F(A, B, C, D) = Ʃ (0, 1, 2, 3, 4, 5, 8, 9, 10, 11, 12, 13,14).
(i) Reduce the above expression by using 4-variable Karnaugh map, showing the
various groups (i.e. octal, quads and pairs).
(ii) Draw the logic gate diagram for the reduced expression. Assume that the
variables and their complements are available as inputs.
(b) Given the Boolean function: F(A, B, C, D) = π ( 3, 4, 6, 9, 11, 12, 13, 14, 15 ).
(i) Reduce the above expression by using 4-variable Karnaugh map, showing the
various groups (i.e. octal, quads and pairs).
(ii) Draw the logic gate diagram for the reduced expression. Assume that the
variables and their complements are available as inputs.
Solution
(a) (i)
F = B' + C' + AD'
(ii)
(b) (i)
(ii)
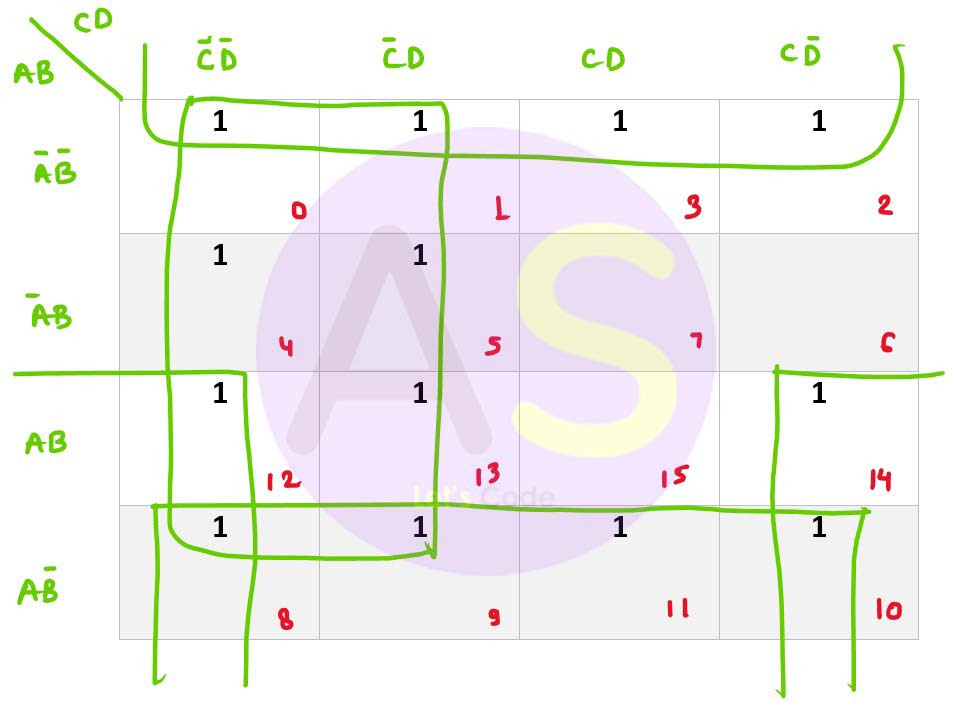
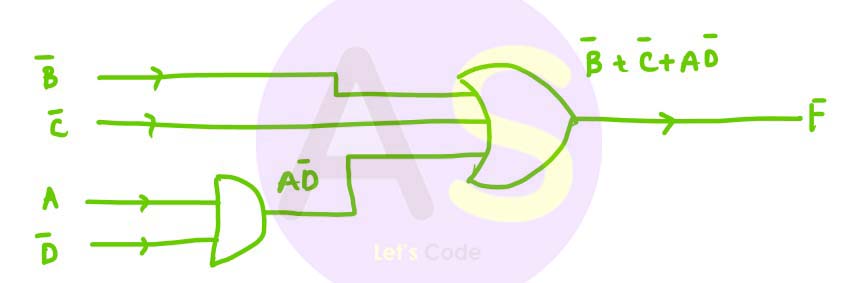
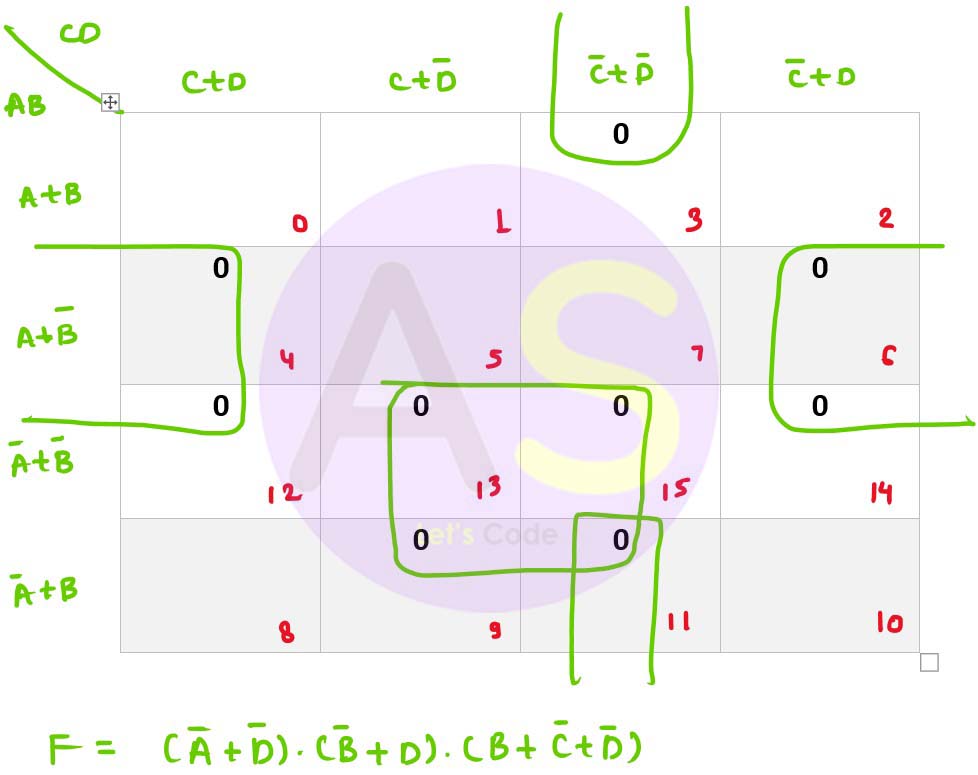
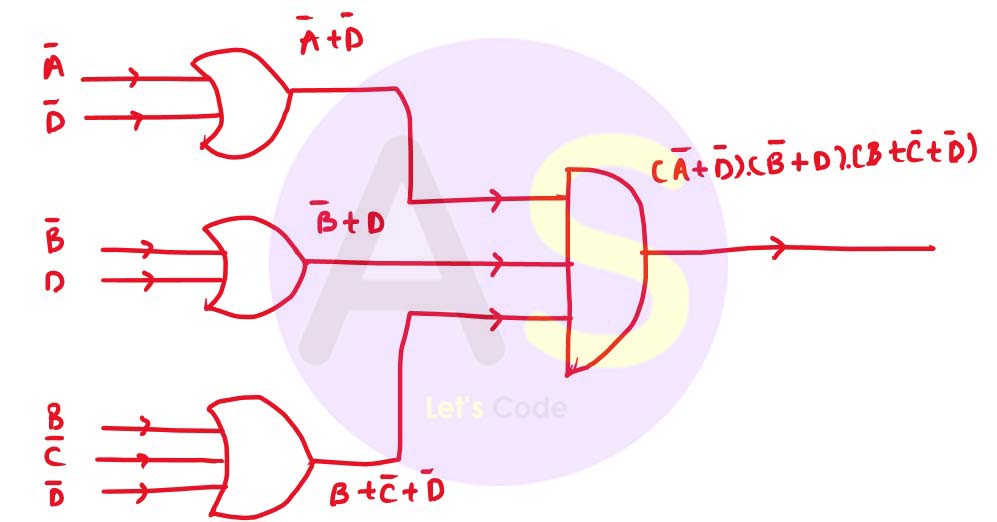
(a) Draw the logic circuit diagram for an octal to binary encoder and explain its working
when a particular digit is pressed. Also, state the difference between encoders and
decoders.
(b) Draw the circuit of a two input XOR gate with the help of NOR gates.
(c) Convert the following expression to its cardinal SOP form:
F(P,Q,R) = P′Q′R + P′QR + PQ′R′ + PQR′
Solution
(a) An encoder is a combinational circuit which inputs 2n or fewer lines and outputs ‘n’ lines.
It converts HLL to LLL e.g. octal, decimal and hexadecimal to binary
A decoder is a combinational circuit which inputs ‘n’ lines and outputs 2n or fewer lines.
It converts LLL to HLL e.g. binary to octal, decimal and hexadecimal.
(b) XOR gate using only NOR gates:
(c) F(P,Q,R) = P’Q’R + P’QR + PQ’R’ + PQR’
= 001 011 100 110
F(P,Q,R) = ∑( 1,3,4,6 )
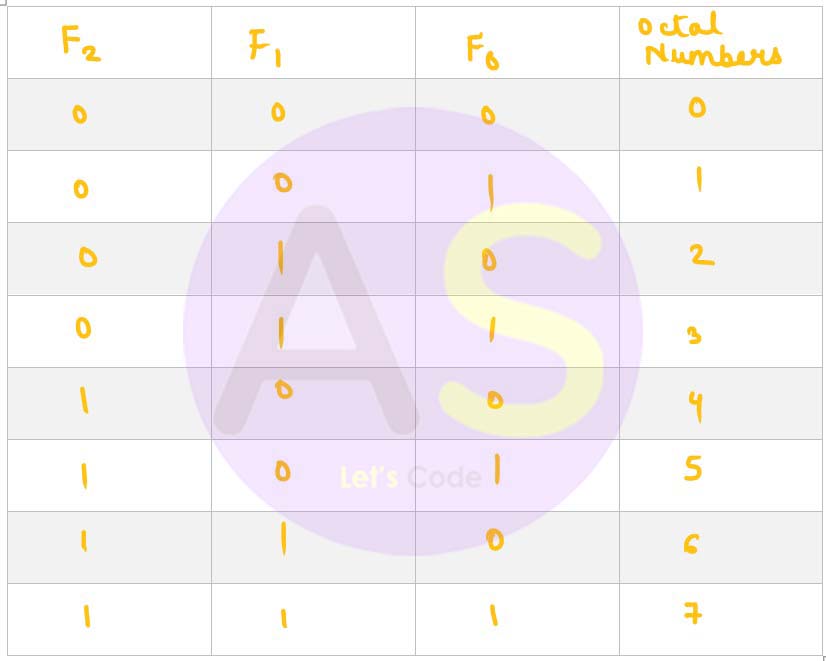
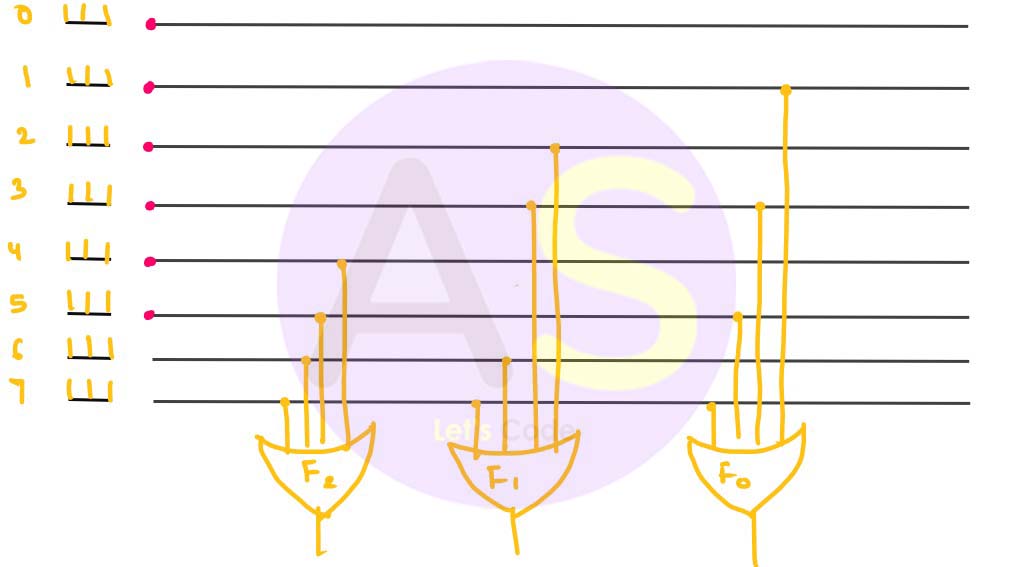
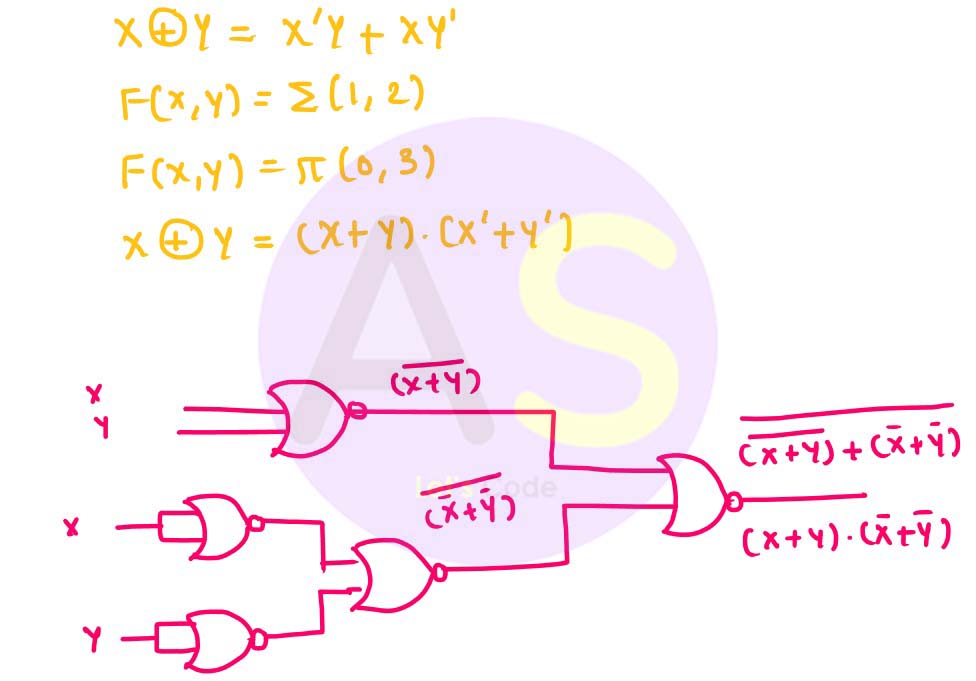
(a) A company intends to develop a device to show the high-status power load for a
household invertor depending on the criteria given below:
• If Air conditioner and Geyser are on
OR
• If Air conditioner is off, but Geyser and Refrigerator are on
OR
• If Geyser is off, but Air conditioner and Water purifier are on
OR
• When all are on
The inputs are:
A - Air conditioner is on
G - Geyser is on
R - Refrigerator is on
W - Water purifier is on
(In all the above cases 1 indicates yes and 0 indicates no.)
Output: X [1 indicates high power, 0 indicates low power for all cases]
Draw the truth table for the inputs and outputs given above and write the SOP
expression for X(A,G,R,W).
(b) Draw the truth table and derive an SOP expression for sum and carry for a full adder.
Also, draw the logic circuit for the carry of a full adder.
(c) Simplify the following expression using Boolean laws:
F = [ (X′ + Y) • (Y′ + Z) ]′ + (X′ + Z)
Solution
(a)
(b)
(c) (Simplify :[(X’+Y)•(Y’+Z)]’ + (X’+Z)
= (X’+Y)’ + (Y’+Z)’ + (X’+Z)
= XY’ + YZ’ + X’ + Z
= X’ + XY’ + Z + YZ’
= (X’+X)•(X’+Y’)+(Y+Z)•(Z+Z’)
= X’+Y’+Z +Y
= 1 as ( Y + Y’ =1)
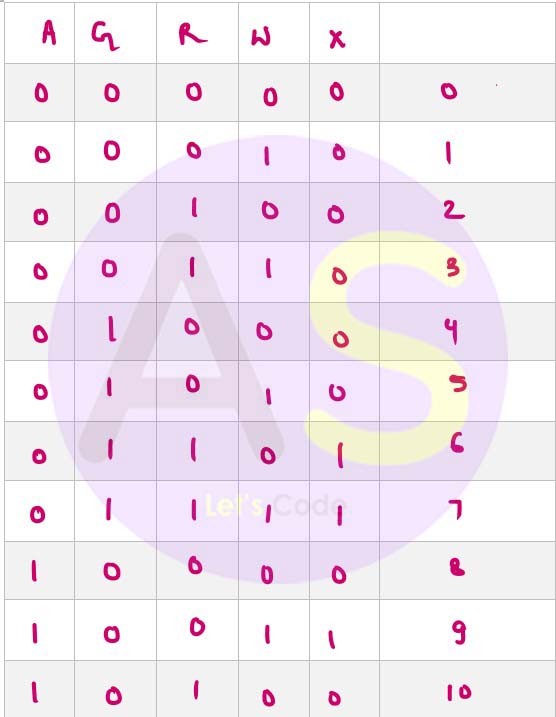
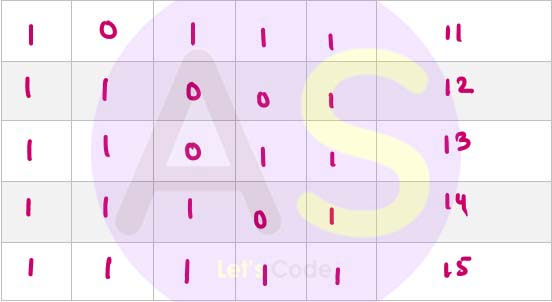
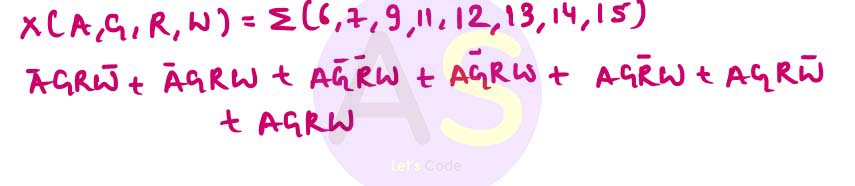
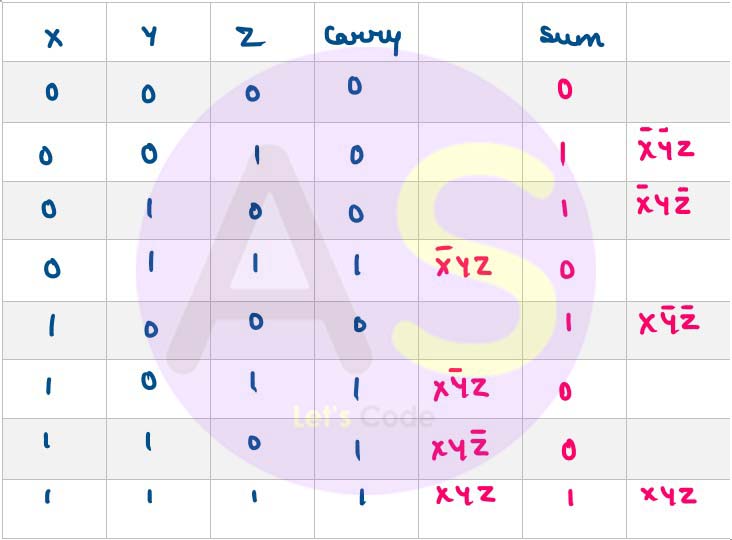
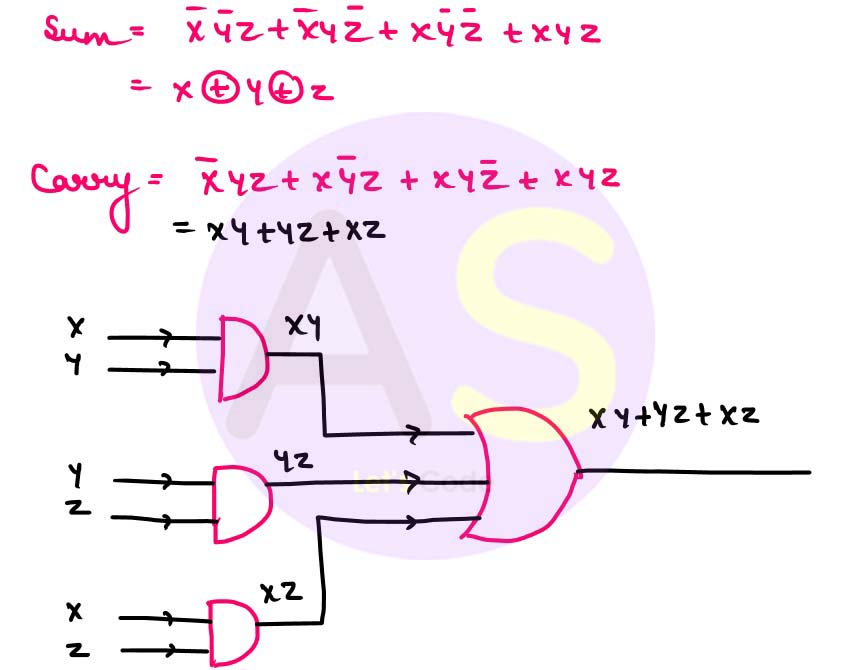
SECTION B
(Attempt all questions from this Section.)
Question 7
Design a class Convert to find the date and the month from a given day number for a
particular year.
Example: If day number is 64 and the year is 2020, then the corresponding date would
be:
March 4, 2020 i.e. (31 + 29 + 4 = 64)
Some of the members of the class are given below:
Classname : Convert
Data members/instance variables:
n : integer to store the day number
d : integer to store the day of the month (date)
m integer to store the month
y : integer to store the year
Methods/Member functions:
Convert ( ) : constructor to initialize the data members with legal initial values
void accept( ) : to accept the day number and the year
void day_to_date( ) : converts the day number to its corresponding date for a particular year and stores the date in ‘d’ and the month in ‘m’
void display( ) : displays the month name, date and year
Specify the class Convert giving details of the constructor( ),void accept( ), void
day_to_date( ) and voiddisplay( ). Define a main( ) function to create an object and call the functions accordingly to enable the task.
Solution:
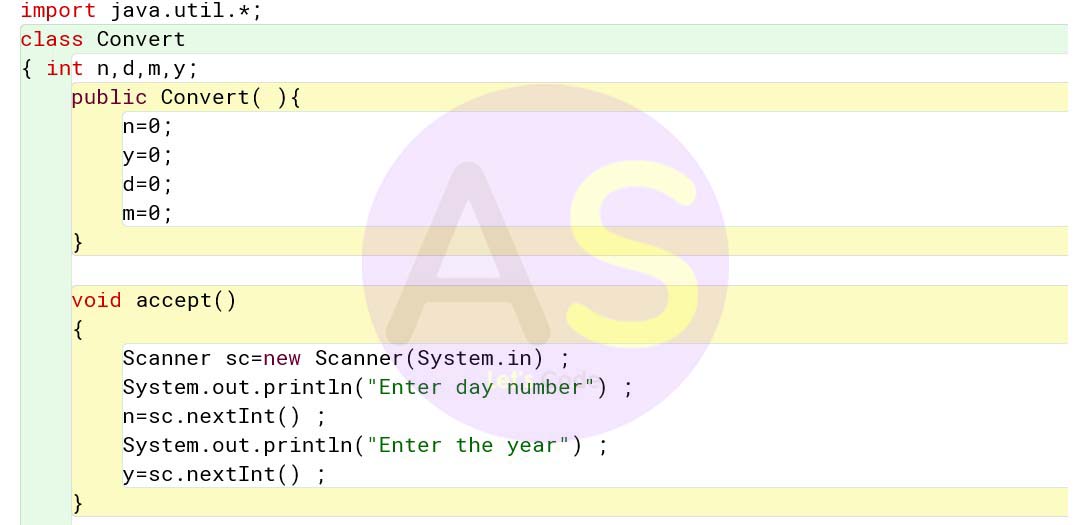
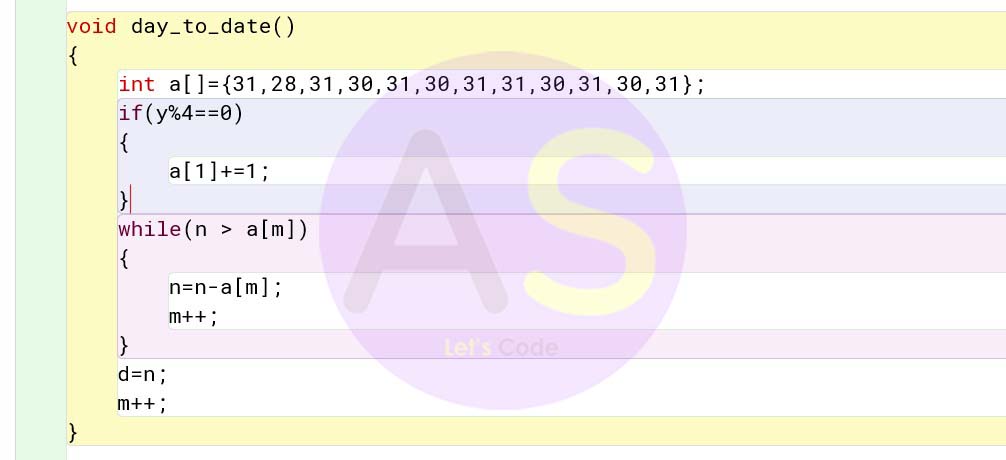
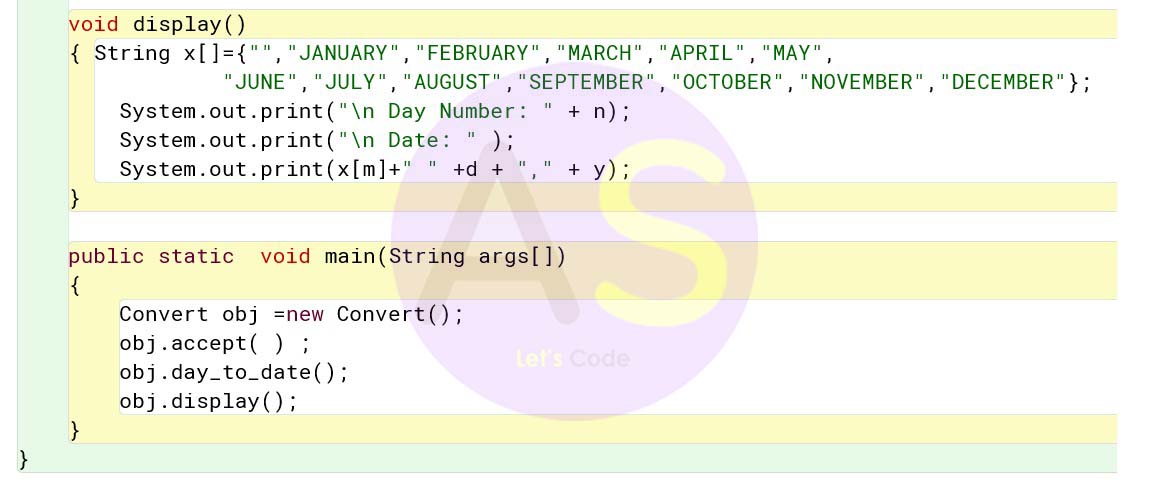
import java.util.*; class Convert { int n,d,m,y; public Convert( ){ n=0; y=0; d=0; m=0; } void accept() { Scanner sc=new Scanner(System.in) ; System.out.println("Enter day number") ; n=sc.nextInt() ; System.out.println("Enter the year") ; y=sc.nextInt() ; } void day_to_date() { int a[]={31,28,31,30,31,30,31,31,30,31,30,31}; if(y%4==0) { a[1]+=1; } while(n > a[m]) { n=n-a[m]; m++; } d=n; m++; } void display() { String x[]={"","JANUARY","FEBRUARY","MARCH","APRIL","MAY", "JUNE","JULY","AUGUST","SEPTEMBER","OCTOBER","NOVEMBER","DECEMBER"}; System.out.print("\n Day Number: " + n); System.out.print("\n Date: " ); System.out.print(x[m]+" " +d + "," + y); } static void main() { Convert obj =new Convert(); obj.accept( ) ; obj.day_to_date(); obj.display(); } }
Question 8
Design a class BinSearch to search for a particular value in an array.
Some of the members of the class are given below:
Classname : BinSearch
Data members/instance variables:
arr[ ] : to store integer elements
n : integer to store the size of the array
Member functions/methods:
BinSearch(int nn ) : parameterized constructor to initialize
n=nn
void fillarray( ) : to enter elements in the array
void sort( ) : sorts the array elements in ascending order using any standard sorting
technique
int bin_search(int l,int u,int v) : searches for the value ‘v’ using binary
search and recursive technique and returns its location if found otherwise returns -1
Define the class BinSearch giving details of the constructor( ), void fillarray( ), void
sort( ) and int bin_search(int,int,int). Define the main( ) function to create an object and call the functions accordingly to enable the task.
Solution:
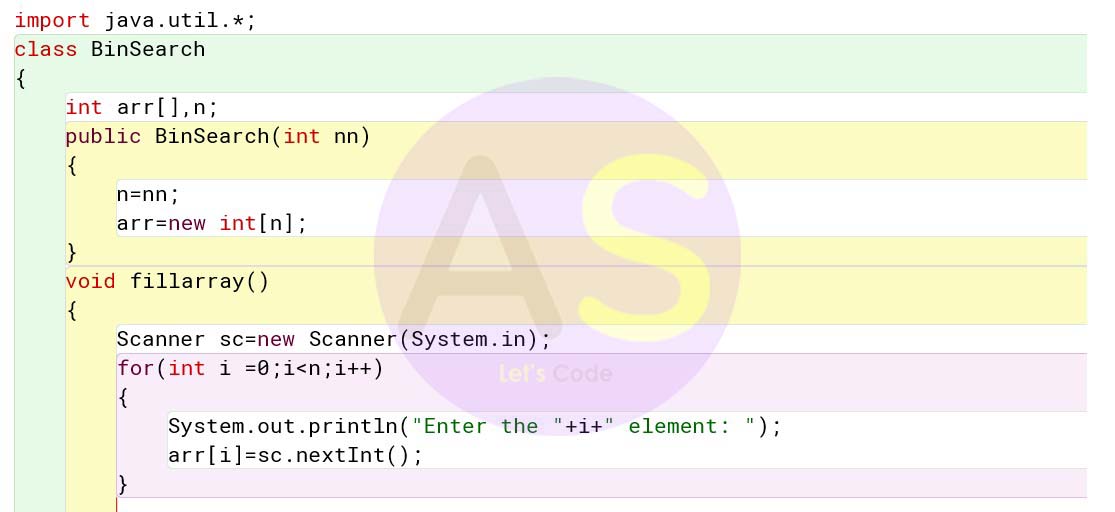
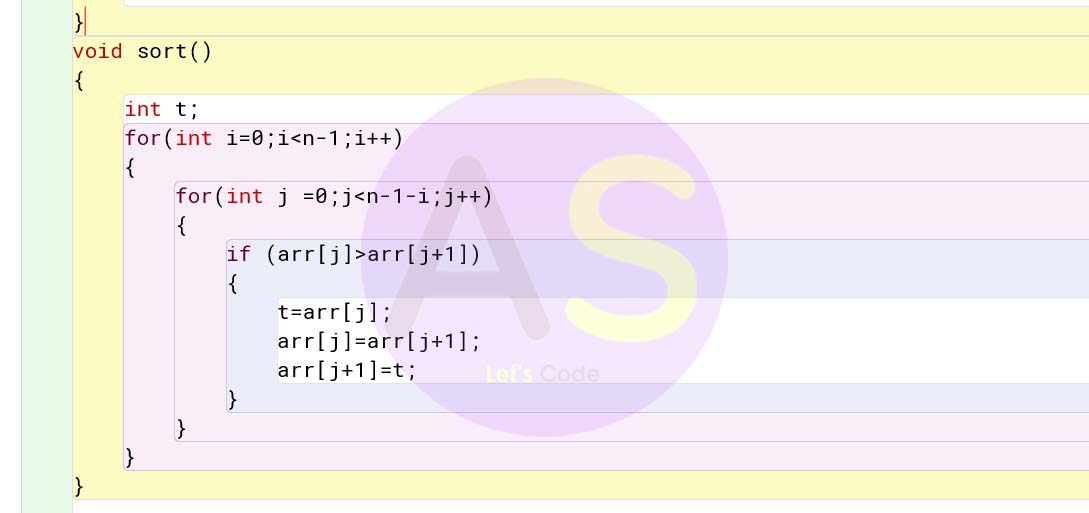
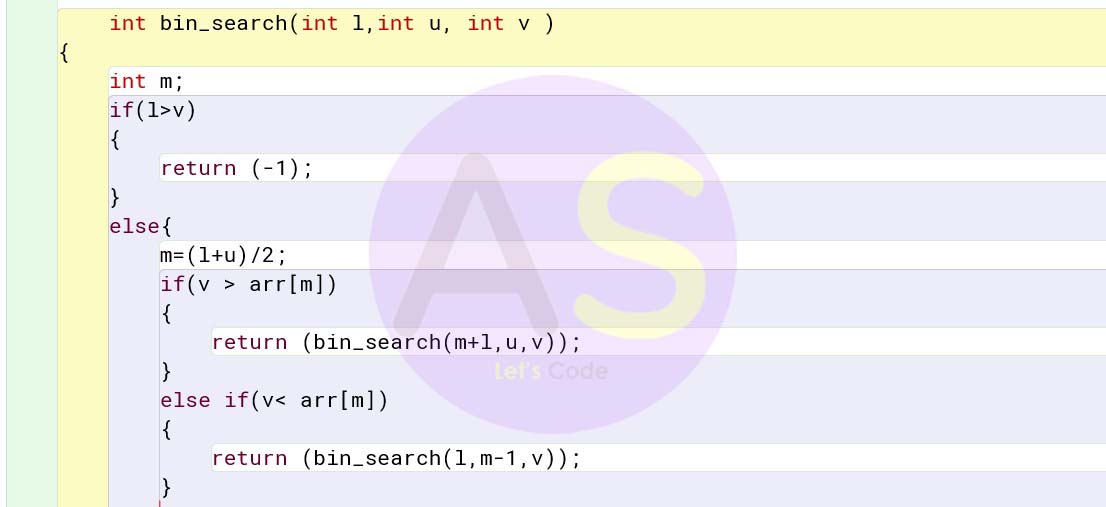
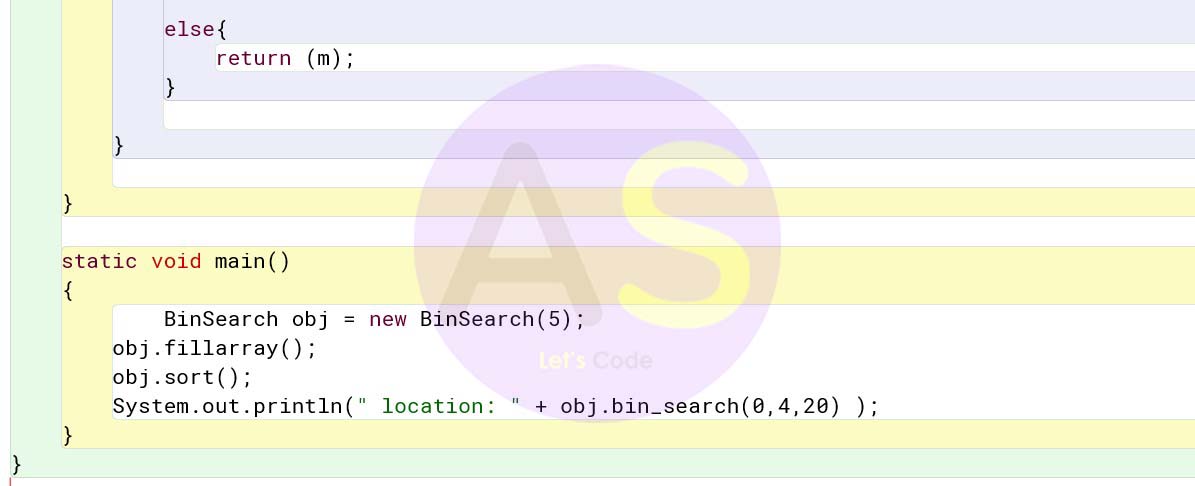
import java.util.*; class BinSearch { int arr[],n; public BinSearch(int nn) { n=nn; arr=new int[n]; } void fillarray() { Scanner sc=new Scanner(System.in); for(int i =0;i< n;i++) { System.out.println("Enter the "+i+" element: "); arr[i]=sc.nextInt(); } } void sort() { int t; for(int i=0;i< n-1;i++) { for(int j =0;j< n-1-i;j++) { if (arr[j]>arr[j+1]) { t=arr[j]; arr[j]=arr[j+1]; arr[j+1]=t; } } } } int bin_search(int l,int u, int v ) { int m; if(l>v) { return (-1); } else{ m=(l+u)/2; if(v > arr[m]) { return (bin_search(m+l,u,v)); } else if(v< arr[m]) { return (bin_search(l,m-1,v)); } else{ return (m); } } } static void main() { BinSearch obj = new BinSearch(5); obj.fillarray(); obj.sort(); System.out.println(" location: " + obj.bin_search(0,4,20) ); } }
Question 9
A class Mix has been defined to mix two words, character by character, in the following
manner:
The first character of the first word is followed by the first character of the second word and so on. If the words are of different length, the remaining characters of the longer word are put at the end.
Example: If the First word is “JUMP” and the second word is “STROLL”, then the required
word will be “JSUTMRPOLL”
Some of the members of the class are given below:
Classname : Mix
Data member/instance variable:
wrd : to store a word
len : to store the length of the word
Member functions/methods:
Mix( ) : default constructor to initialize the data members with legal initial values
void feedword( ) : to accept the word in UPPER case
void mix_word( Mix P, Mix Q): mixes the words of objects P and Q asstated above and stores the resultant word in the current object
void display( ) : displays the word
Specify the class Mix giving the details of the constructor( ), void feedword( ), void
mix_word( Mix, Mix ) and void display( ). Define the main( ) function to create objects
and call the functions accordingly to enable the task.
Solution:
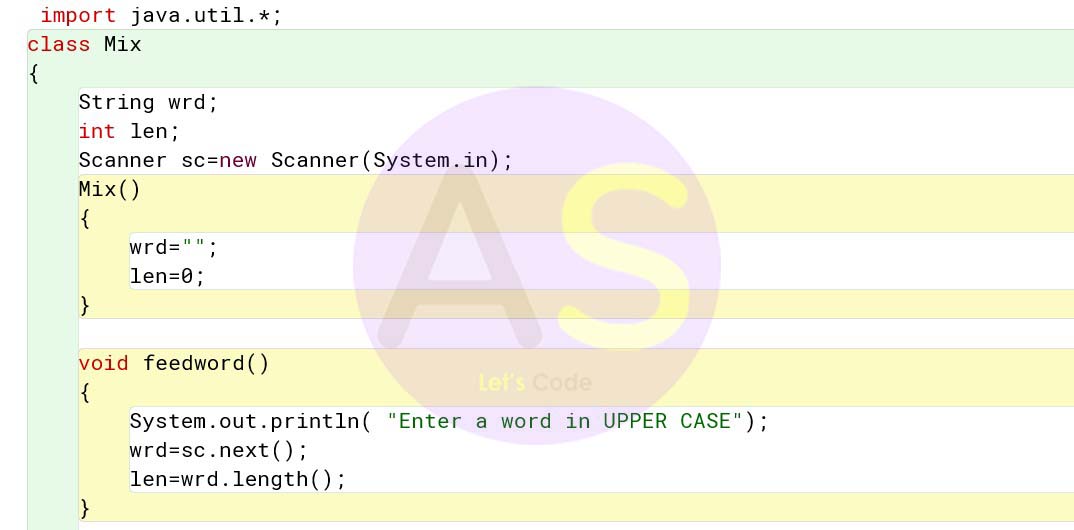
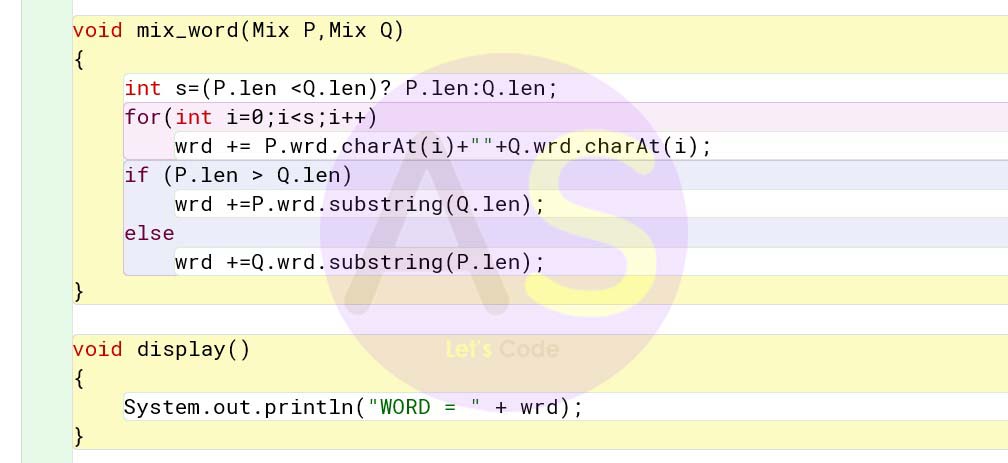
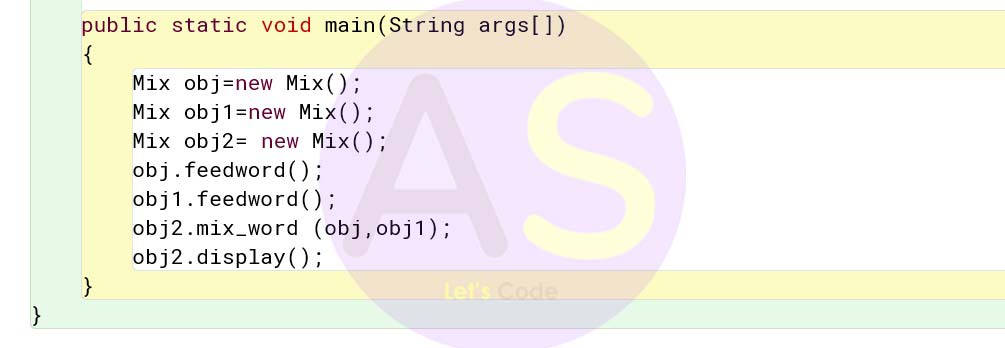
import java.util.*; class Mix { String wrd; int len; Scanner sc=new Scanner(System.in); Mix() { wrd=""; len=0; } void feedword() { System.out.println( "Enter a word in UPPER CASE"); wrd=sc.next(); len=wrd.length(); } void mix_word(Mix P,Mix Q) { int s=(P.len < Q.len)? P.len:Q.len; for(int i=0;i< s;i++) wrd += P.wrd.charAt(i)+""+Q.wrd.charAt(i); if (P.len > Q.len) wrd +=P.wrd.substring(Q.len); else wrd +=Q.wrd.substring(P.len); } void display() { System.out.println("WORD = " + wrd); } static void main() { Mix obj=new Mix(); Mix obj1=new Mix(); Mix obj2= new Mix(); obj.feedword(); obj1.feedword(); obj2.mix_word (obj,obj1); obj2.display(); } }
Question 10
A Circular queue is a linear data structure which works on the principle of FIFO, enables the user to enter data from the rear end and remove data from the front end with the rear end connected to the front end to form a circular pattern. Define a class CirQueue with the following details:
Class name : CirQueue
Data member/instance variable:
cq[ ] : array to store the integers
cap : stores the maximum capacity of the array
front : to point the index of the front end
rear : to point the index of the rear end
Member functions/methods:
CirQueue (int max) : constructor to initialize the data member
cap=max, front=0 and rear=0
void push(int n) : to add integer in the queue from the rear end if possible, otherwise display the message “QUEUE IS FULL”
int pop( ) : removes and returns the integer from the front end of the queue if any, else returns -9999
void show( ) : displays the queue elements
Specify the class CirQueue giving details of the functions void push(int) and int pop( ).Assume that the other functions have been defined.
The main function and algorithm need NOT be written.
Solution:
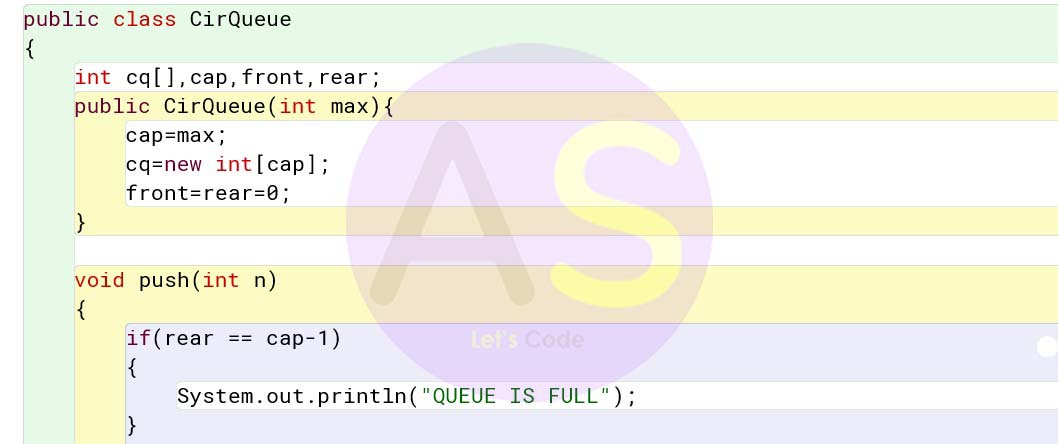
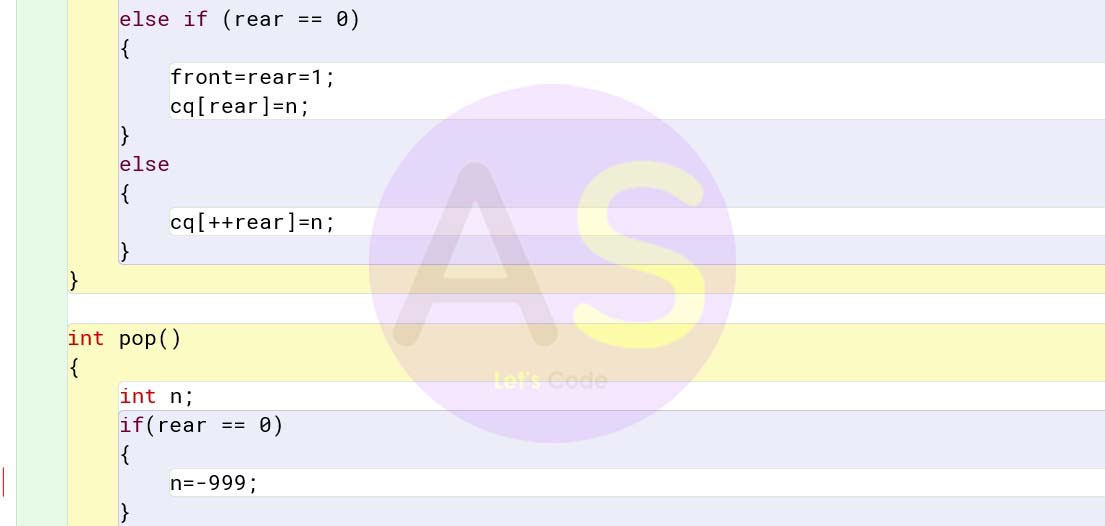
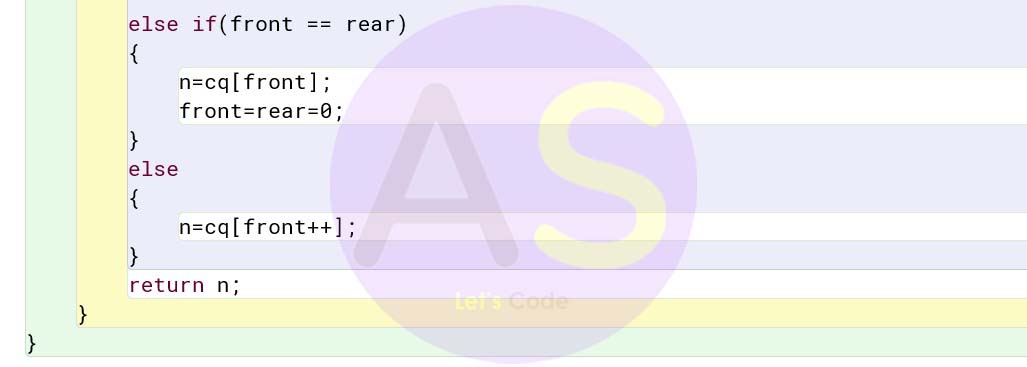
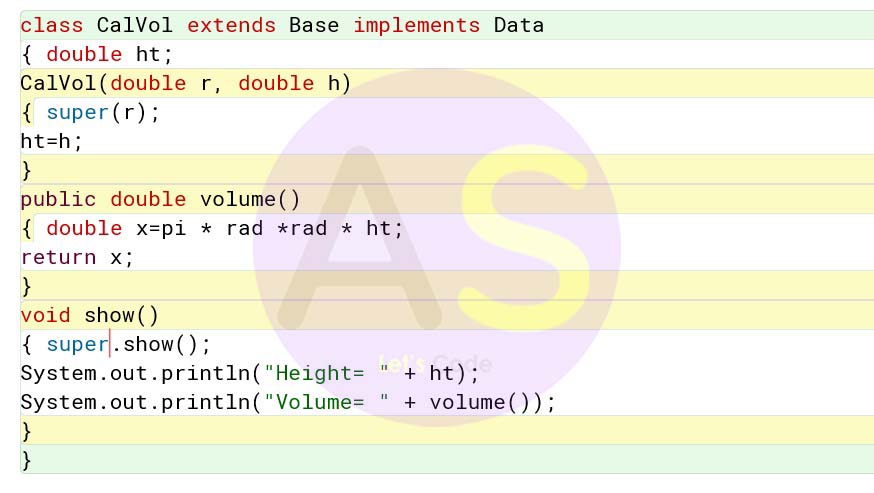
public class CirQueue { int cq[],cap,front,rear; public CirQueue(int max){ cap=max; cq=new int[cap]; front=rear=0; } void push(int n) { if(rear == cap-1) { System.out.println("QUEUE IS FULL"); } else if (rear == 0) { front=rear=1; cq[rear]=n; } else { cq[++rear]=n; } } int pop() { int n; if(rear == 0) { n=-999; } else if(front == rear) { n=cq[front]; front=rear=0; } else { n=cq[front++]; } return n; } }
Question 12
A linked list is formed from the objects of the class Node. The class structure of the
Node is given below:
class Node
{
int n;
Node next;
}
Write an Algorithm OR a Method to find the product of the integer numbers from an
existing linked list.
The method declaration is as follows:
void Product_Node( Node str )
(b) Answer the following questions from the diagram of a Binary Tree given below:
(i) Write the post-order traversal of the left subtree of the above structure.
(ii) State the degree of the Nodes E and H.
(iii) Mention the external nodes of the right subtree.
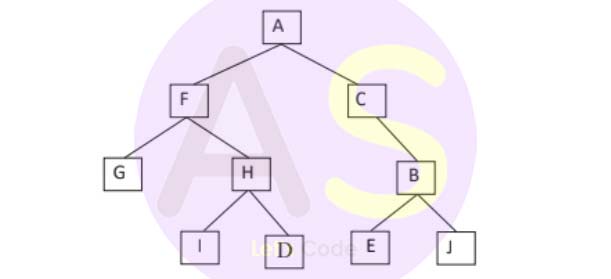