Code is copied!
CLASS 12 ISC - Java Question Paper 2018
PART I
(Attempt all questions)
Question 1
(a) State the Commutative law and prove it with the help of a truth table
Solution
(a) Commutative law states that the interchanging of the order of operands in a Boolean equation does
not change its result.
Using OR operator → A + B = B + A
Using AND operator → A * B = B * A,
Truth Table for Commutative Law
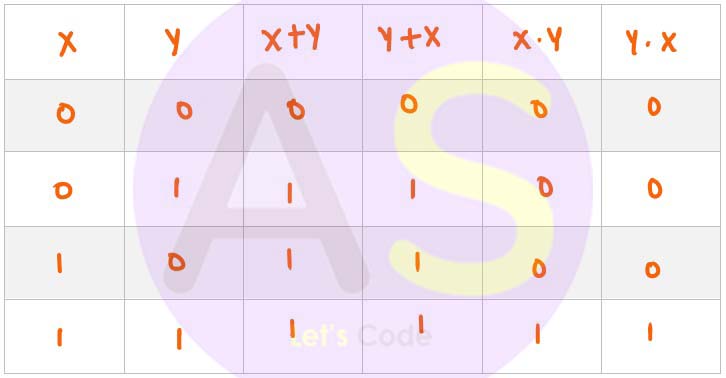
(b)
Convert the following expression into its canonical POS form:
F(X, Y, Z) = (X + Y) . (Y’ + Z)
Solution
(b) F(X, Y, Z) = (X + Y’).(Y’ + Z)
By De-Morgan’s theorem, we have
((X + Y’).(Y’ + Z))’ = (X + Y’)’ + (Y’+ Z)’
=X’.Y” + Y”.Z’
= X’.Y + Y.Z’
= Y.(X’ + Z’)
Again applying De-Morgan’s theorem, we have
(Y.(X’ + Z’))’ = (X’ + Z’)’
(c)
Find the dual of
(A’ + B) . (1 + B’) = A’+B
Solution
In Principle of Duality
replace (+) by (.)
replace (.) by (+)
replace 1 by 0
Given : (A’ + B) . (1 + B’) = A’+B
Taking L.H.S. = (A’ + B).(1 + B’)
= (A’.B) + (0.B’) [Property of 0]
= A’.B
Taking R.H.S.= A’+ B
= A’.B
(d)
Verify the following proposition with the help of a truth table:
(P∧Q)∨(P∧~Q) = P
Solution
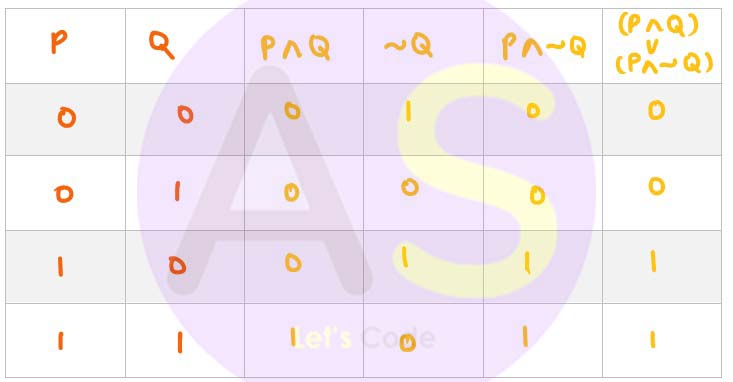
(e) If F(A, B, C) = A'(BC’ + B’C), then find F’.
Solution
F(A,B,C) = A'.(BC'+ B'C)
F' = (A'.(BC'+ B'C))
=((A')') + (BC' + B'C)'
= A +(B.C')'.(B'.C)'
= A + (B'+C).(B+C')
(a)
What are the Wrapper classes? Give any two examples.
Solution
(a) A Wrapper class is a class whose object wraps or contains primitive data types. When we create an
object to a wrapper class, it contains a field and in this field, we can store primitive data types. In
other words, we can wrap a primitive value into a wrapper class object.
Ex - Character, Integer
(b) A matrix A[m] [m] is stored in the memory with each element requiring 4 bytes of storage. If the base address at A[1] [1] is 1500 and the address of A[4][5] is 1608, determine the order of the matrix when it is stored in Column Major Wise.
Solution
(b) Base Address, B = 1500
W = 4 Bytes
i = 4, j = 5, r = 1, c = 1
Formula for Column Major Wise
A[4][5] = B + W[m(j – c) + (I – r)]
1608 = 1500 + 4[m(5 – 1) + (4 – 1)]
1608 – 1500 = 16m + 12
16m = 96
m = 6
(c)
Convert the following infix notation to postfix form:
A + (B – C*(D/E) * F)
Solution
=A + (B-C(DE/)*F)
=A + (B - CDE/**F)
=A + (BCDE/*F*-)
=ABCDE/*F*-+
(d) Define Big ‘O’ notation. State the two factors which determine the complexity of an algorithm.
Solution
(d) Big ‘O’ notation is a particular tool for assessing algorithm efficiency and is used to describe the
performance or complexity of an algorithm. While analyzing an algorithm, we mostly consider time
complexity and space complexity.
The time complexity of an algorithm quantifies the amount of time taken by an algorithm to run as a
function of the length of the input. Similarly, the Space complexity of an algorithm quantifies the
amount of space or memory taken by an algorithm to run as a function of the length of the input.
(e) What is exceptional handling? Also, state the purpose of finally block in a try-catch statement
Solution
(e) The exception handling in java is one of the powerful mechanism to handle the runtime errors so that
the normal flow of the application can be maintained.
Purpose of finally block in a try catch statement is to execute the statements placed in the finally
block irrespective of the error occurring.
The following is a function of some class which checks if a positive integer is a Palindrome number by
returning true or false. (A number is said to be palindrome if the reverse of the number is equal to the
original number.) The function does not use the modulus (%) operator to extract digit. There are some places
in the code marked by ?1?, ?2?, ?3?, ?4?, ?5? which may be replaced by a statement/expression so that the
function works properly.
1. What is the statement or expression at ?1?
2. What is the statement or expression at ?2?
3. What is the statement or expression at ?3?
4. What is the statement or expression at ?4?
5. What is the statement or expression at ?5?
1. 0
boolean PalindromeNum(int N) { int rev=?1?; int num=N; while(num>0) { int f=num/10; int s= ?2?; int digit = num-?3?; rev= ?4? + digit; num/= ?5?; } if(rev==N) return true; else return false; }
1. What is the statement or expression at ?1?
2. What is the statement or expression at ?2?
3. What is the statement or expression at ?3?
4. What is the statement or expression at ?4?
5. What is the statement or expression at ?5?
Solution
1. 0
2. 0
3. 1
4. rev*10
5. 10
PART II
SECTION A
(Attempt any two questions from this Section.)
Question 4
(a) Given the Boolean function F(A, B, C, D) = Σ (0, 2, 4, 8, 9, 10, 12, 13).
(i) Reduce the above expression by using 4-variable Karnaugh map, showing the various groups (i.eoctal,
quads and pairs).
(ii) Draw the logic gate diagram for the reduced expression. Assume that the variables and their
complements are available as inputs.
(b) Given the Boolean function: F(A, B, C, D) = π(3, 4, 5, 6, 7, 10, 11, 14, 15).
(i) Reduce the above expression by using the 4-variable Karnaugh map, showing the various groups (i.e.,
octal, quads and pairs).
(ii) Draw the logic gate diagram for the reduced expression. Assume that the variables and their
complements are available as inputs.
Solution
(a) (i)
Reduced expression is C'D’ + AC’ + B’.D'
(ii)
(b) (i)
Reduced Expression is (A + B’).(C’ + D’).(A’ + C’)
(ii)
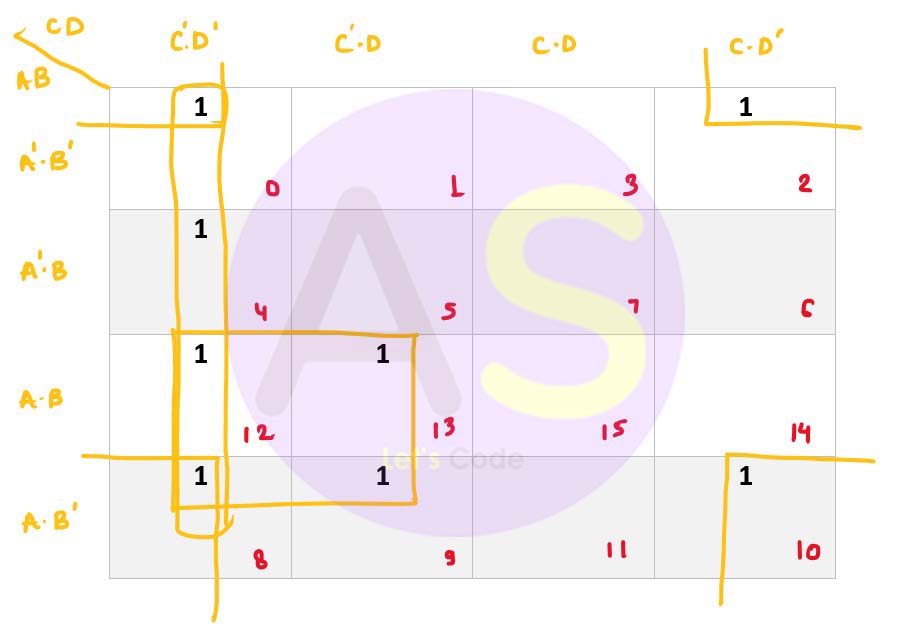
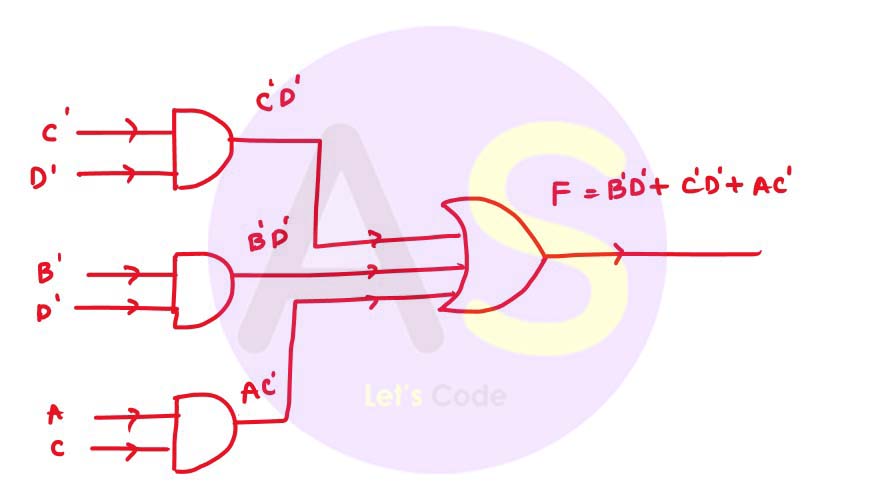
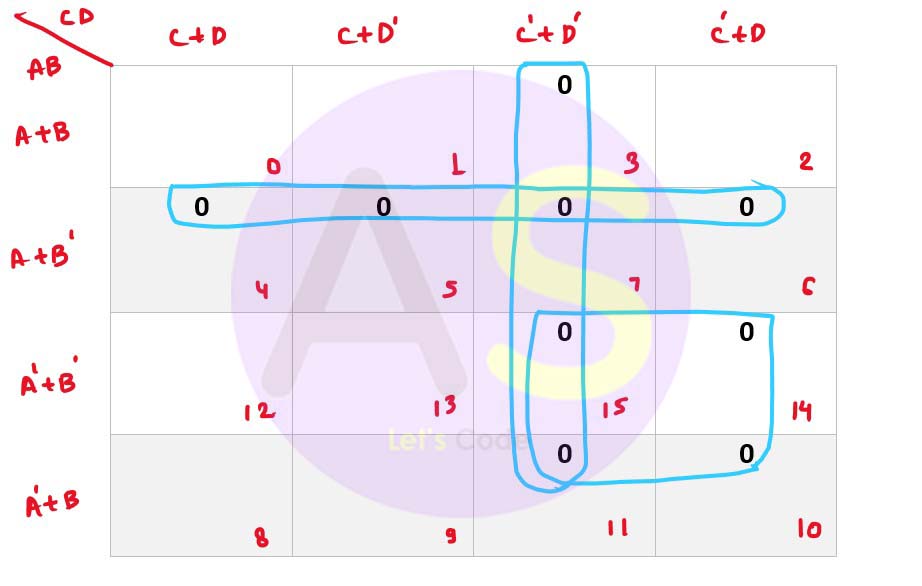
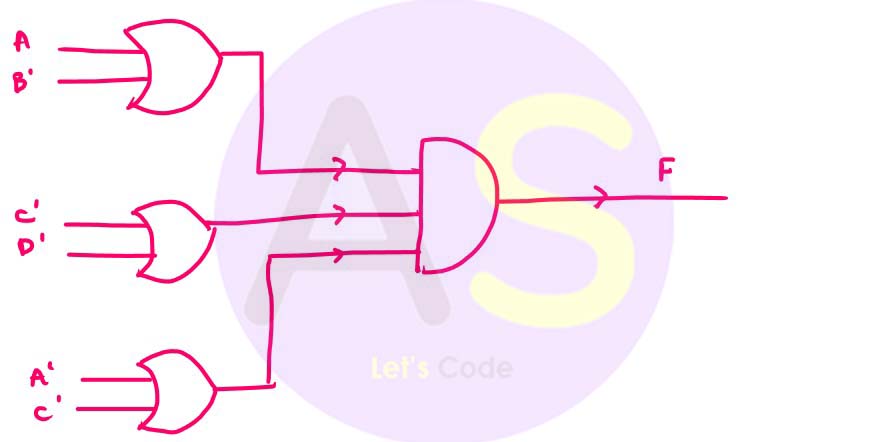
(a) A training institute intends to give scholarships to its students as per the criteria given
below:
The student has excellent academic record but is financially weak.
OR
The student does not have an excellent academic record and belongs to a backward class.
OR
The student does not have an excellent academic record and is physically impaired.
The inputs are
A - Has excellent academic record.
F - Financially sound
C - Belongs to a backward class
I - Is physically impaired
(In all the above cases 1 indicates Yes and 0 indicates No).
Output: X [1 indicates Yes, 0 indicates No for all cases]
Draw the truth table for the inputs and outputs given above and write the SOP expression for X(A, F, C,
I).
(b) Using the truth table, state whether the following proposition is a tautology, contingency or a
contradiction:
~(A∧B)∨(~A=>B)
(c) Simplify the following expression, using Boolean laws:
A.(A’ + B).C.(A + B)
Solution
(a)
(b)
It is a tautology.
(c) A.(A’+B).C.(A + B)
= (AA’ + AB).C.(AA + AB)
= (0 + AB).C.(A + AB) (∵ AA’ = 0)
= (AB).C.(A + AB) (∵ A + AB = A)
= AB.CA
= AA.BC (∵ A.A = A)
= A.B.C
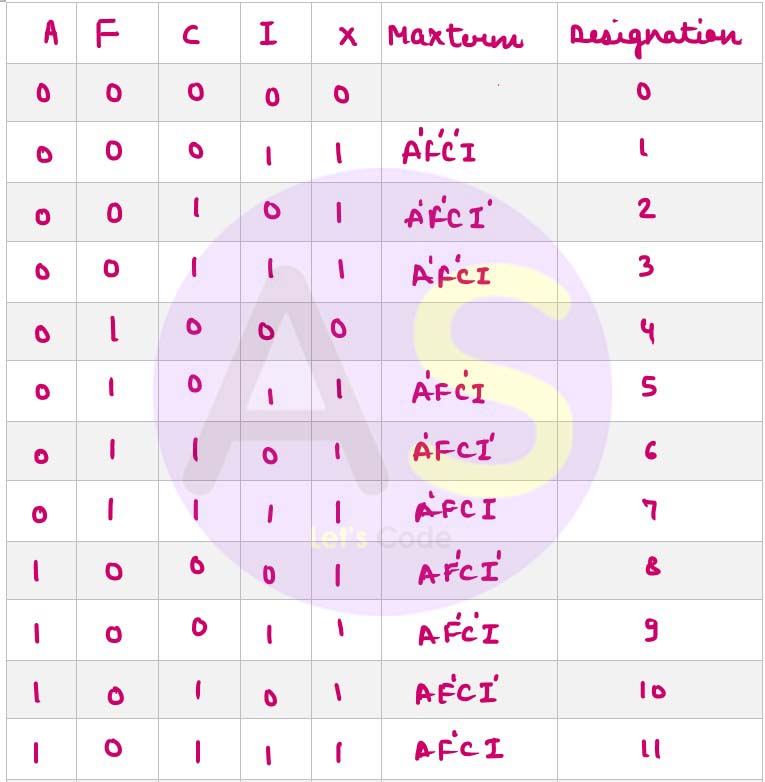
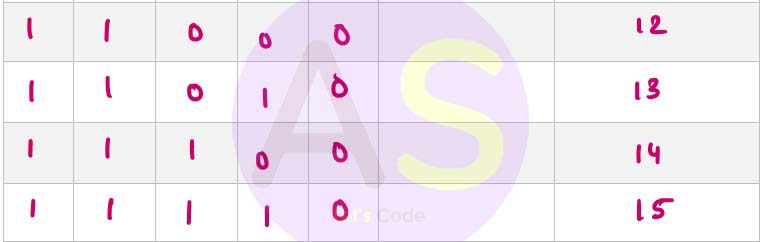
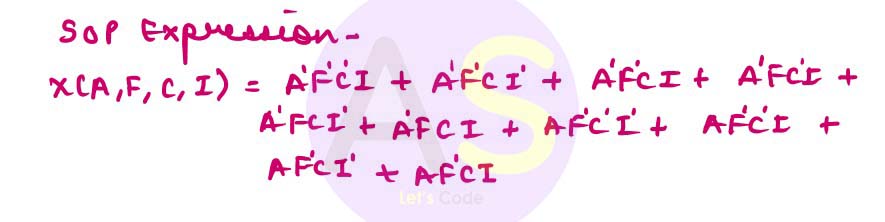
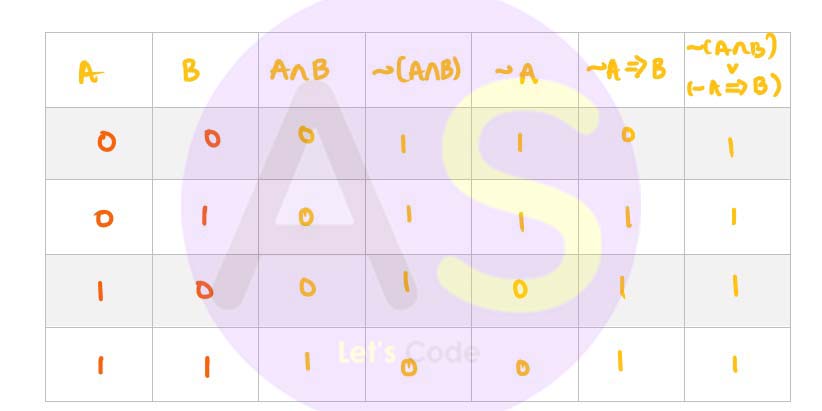
(a) What is an Encoder? Draw the Encoder circuit to convert A-F hexadecimal numbers to binary. State an
application of a Multiplexer.
(b) Differentiate between Half Adder and Full Adder. Draw the logic circuit diagram for a Full
Adder.
(c) Using only NAND gates, draw the logic circuit diagram for A’ + B.
Solution
(a) An Encoder is a combinational circuit that performs the reverse operation of Decoder. It has maximum
of 2n input lines and ‘n’ output lines, hence it encodes the information from 2n
inputs into an n-bit code. Therefore, the encoder encodes 2n input lines with ‘n’ bits.
Application of a multiplexer:
The multiplexer is used to perform high-speed switching in networking
(b) Half adder adds two input binary digits and output 2 binary bits ,whereas full adder add 3 input
binary digits and outputs 2 binary bits
(c)
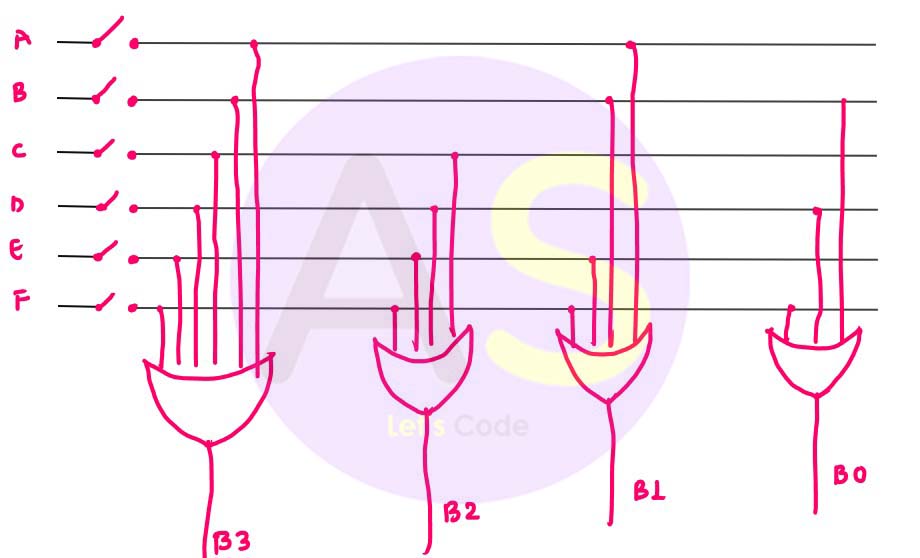
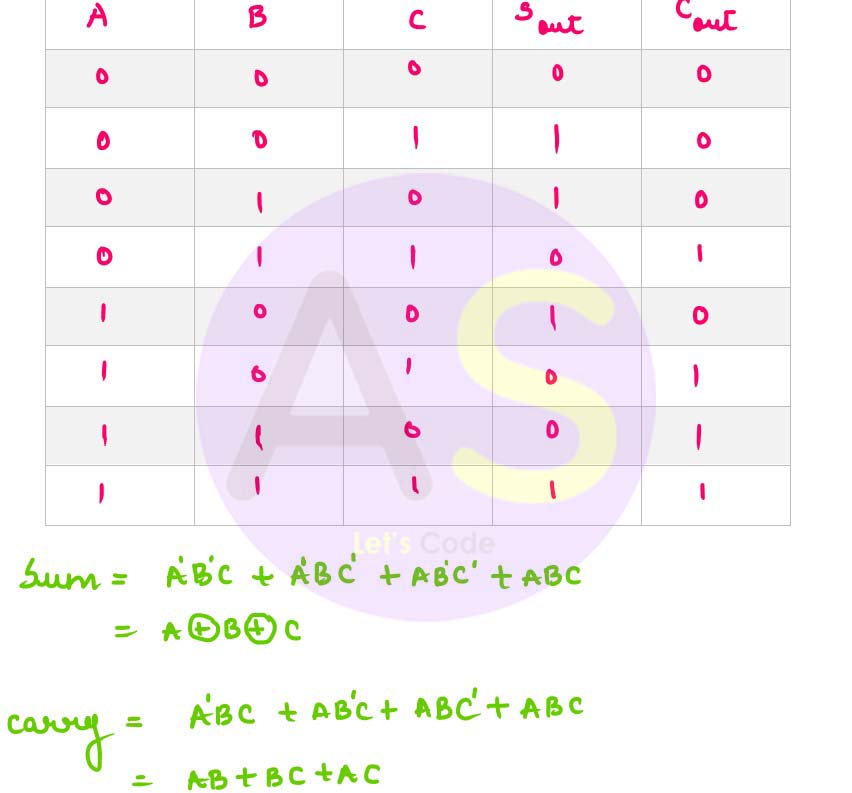
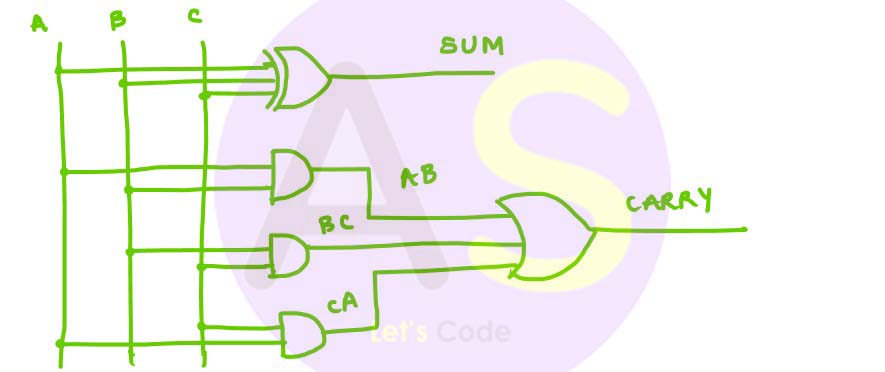
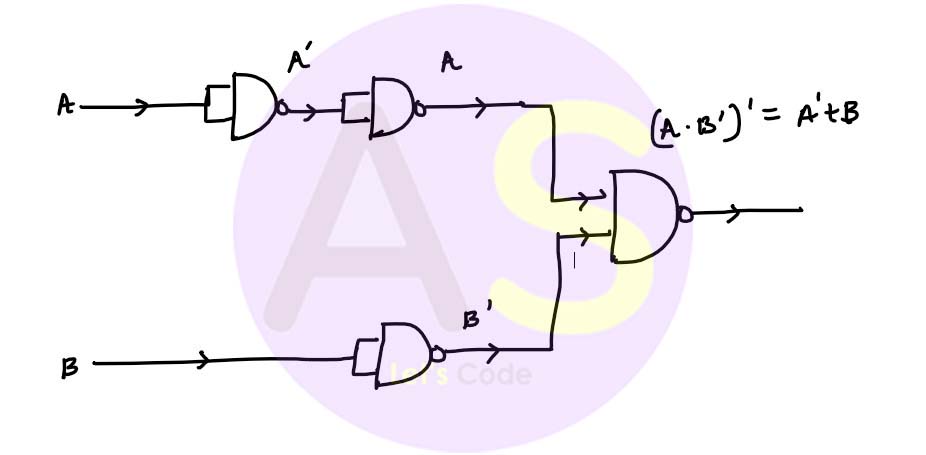
SECTION B
(Attempt all questions from this Section.)
Question 7
Design a class Perfect to check if a given number is a perfect number or not. [A number is said to be perfect if sum of the factors of the number excluding itself is equal to the original number]
Example: 6 = 1 + 2 + 3 (where 1, 2 and 3 are factors of 6, excluding itself)
Some of the members of the class are given below:
Class name: Perfect
Data members/instance variables:
num: to store the number
Methods/Member functions:
Perfect (int nn): parameterized constructor to initialize the data member num=nn
int sum_of_factors(int i): returns the sum of the factors of the number(num), excluding itself, using a recursive technique
void check(): checks whether the given number is perfect by invoking the function sum_of_factors() and displays the result with an appropriate message
Specify the class Perfect giving details of the constructor(), int sum_of_factors(int) and void check(). Define a main() function to create an object and call the functions accordingly to enable the task
Solution:
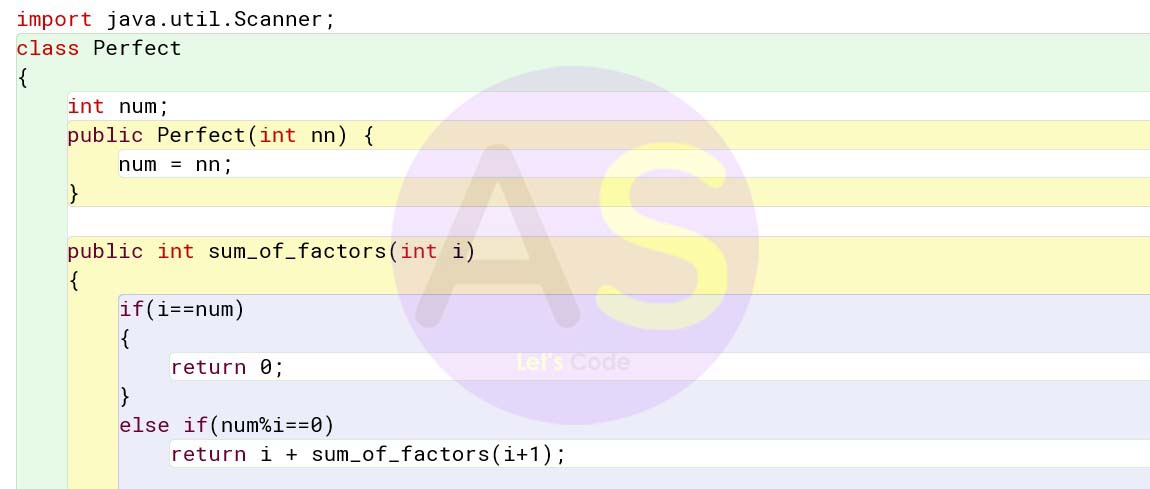
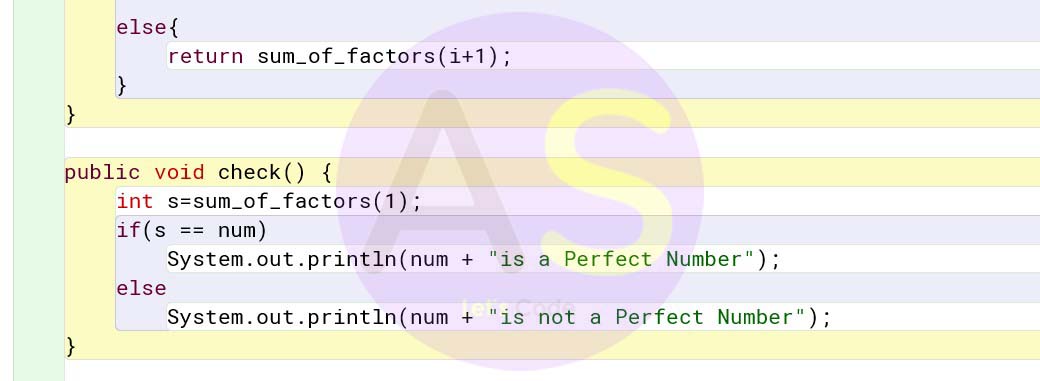
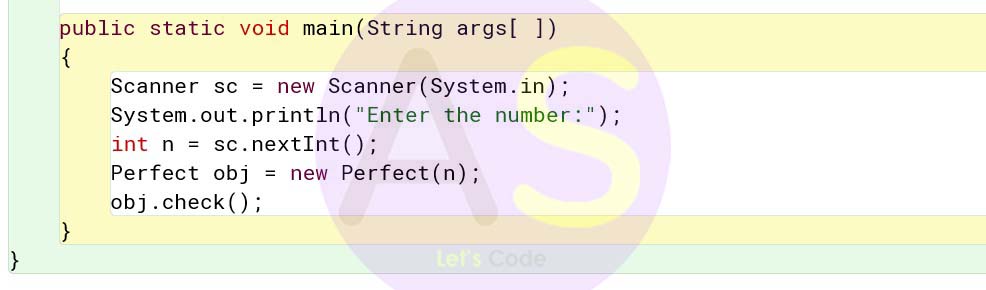
import java.util.Scanner; class Perfect { int num; public Perfect(int nn) { num = nn; } public int sum_of_factors(int i) { if(i==num) { return 0; } else if(num%i==0) return i + sum_of_factors(i+1); else{ return sum_of_factors(i+1); } } public void check() { int s=sum_of_factors(1); if(s == num) System.out.println(num + "is a Perfect Number"); else System.out.println(num + "is not a Perfect Number"); } public static void main(String args[ ]) { Scanner sc = new Scanner(System.in); System.out.println("Enter the number:"); int n = sc.nextInt(); Perfect obj = new Perfect(n); obj.check(); } }
Question 8
Two matrices are said to be equal if they have the same dimension and their corresponding elements are equal.
For example, the two matrices A and B is given below are equal:
Design a class EqMat to check if two matrices are equal or not. Assume that the two matrices have the same dimension.
Some of the members of the class are given below :
Class name: EqMat
Data members/instance variables:
a[][] : to store integer elements
m: to store the number of rows
n: to store the number of columns
Member functions/methods:
EqMat: parameterized constructor to initialise the data members m = mm and n = nn
void readArray(): to enter elements in the array
int check(EqMat P, EqMat Q): checks if the parameterized objects P and Q are equal and returns 1 if true, otherwise returns 0
void print(): displays the array elements
Define the class EqMat giving details of the constructor(), void readarray( ), int check(EqMat, EqMat) and void print( ). Define the main( ) function to create objects and call the functions accordingly to enable the task.
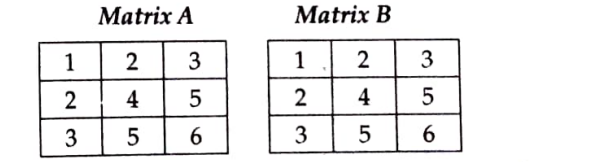
Solution:
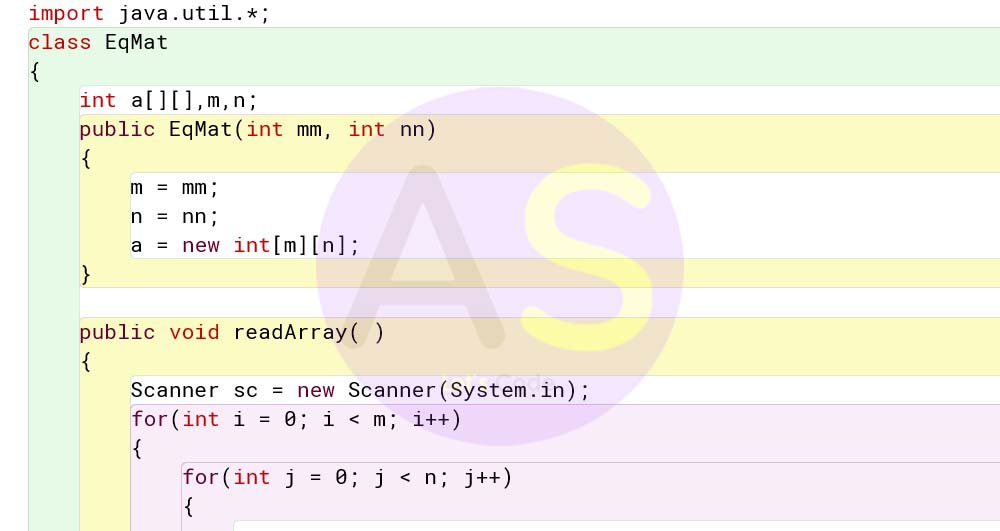
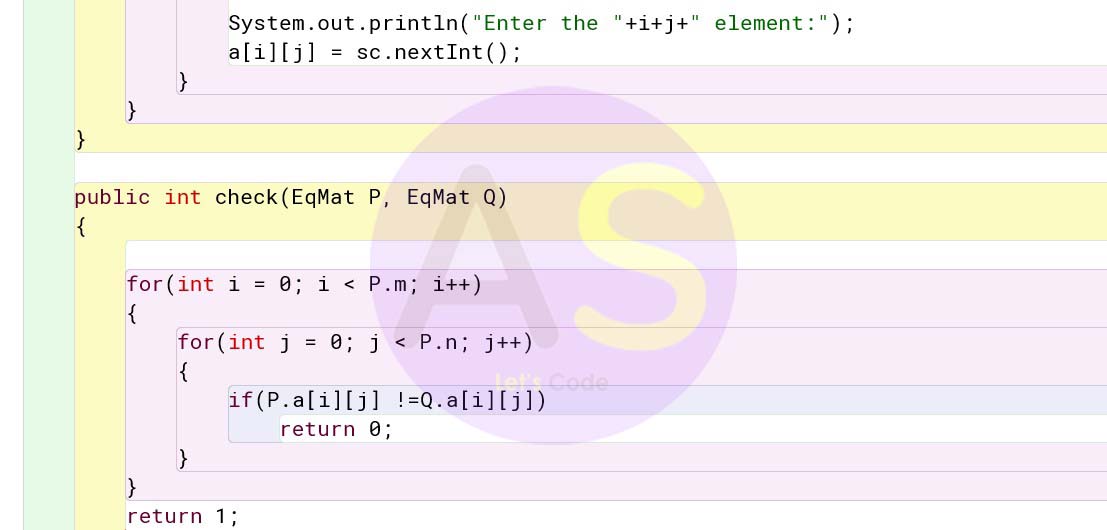
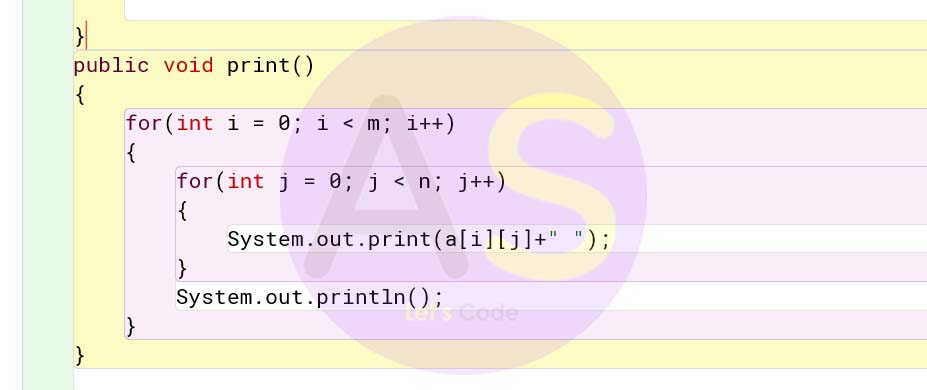
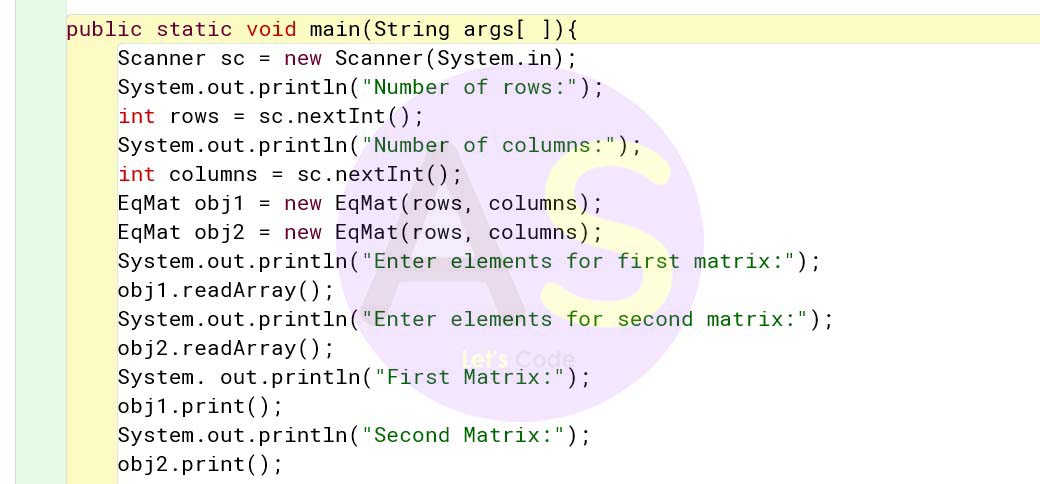
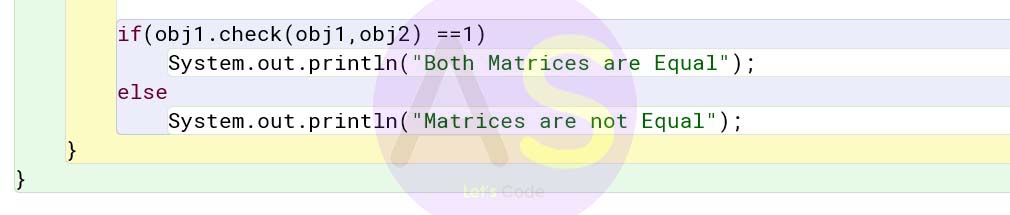
import java.util.*; class EqMat { int a[][],m,n; public EqMat(int mm, int nn) { m = mm; n = nn; a = new int[m][n]; } public void readArray( ) { Scanner sc = new Scanner(System.in); for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.println("Enter the "+i+j+" element:"); a[i][j] = sc.nextInt(); } } } public int check(EqMat P, EqMat Q) { for(int i = 0; i < P.m; i++) { for(int j = 0; j < P.n; j++) { if(P.a[i][j] !=Q.a[i][j]) return 0; } } return 1; } public void print() { for(int i = 0; i < m; i++) { for(int j = 0; j < n; j++) { System.out.print(a[i][j]+" "); } System.out.println(); } } public static void main(String args[ ]){ Scanner sc = new Scanner(System.in); System.out.println("Number of rows:"); int rows = sc.nextInt(); System.out.println("Number of columns:"); int columns = sc.nextInt(); EqMat obj1 = new EqMat(rows, columns); EqMat obj2 = new EqMat(rows, columns); System.out.println("Enter elements for first matrix:"); obj1.readArray(); System.out.println("Enter elements for second matrix:"); obj2.readArray(); System. out.println("First Matrix:"); obj1.print(); System.out.println("Second Matrix:"); obj2.print(); if(obj1.check(obj1,obj2) ==1) System.out.println("Both Matrices are Equal"); else System.out.println("Matrices are not Equal"); } }
Question 9
A class Capital has been defined to check whether a sentence has words beginning with a capital letter or not.
Some of the members of the class are given below:
Class name: Capital
Data member/instance variable:
sent: to store a sentence
freq: stores the frequency of words beginning with a capital letter
Member functions/methods:
Capital () : default constructor
void input (): to accept the sentence
boolean isCap(String w): checks and returns true if the word begins with a capital letter, otherwise returns false
void display(): displays the sentence along with the frequency of the words beginning with a capital letter
Specify the class Capital, giving the details of the constructor( ), void input( ), boolean isCap(String) and void display( ). Define the main( ) function to create an object and call the functions accordingly to enable the task.
Solution:
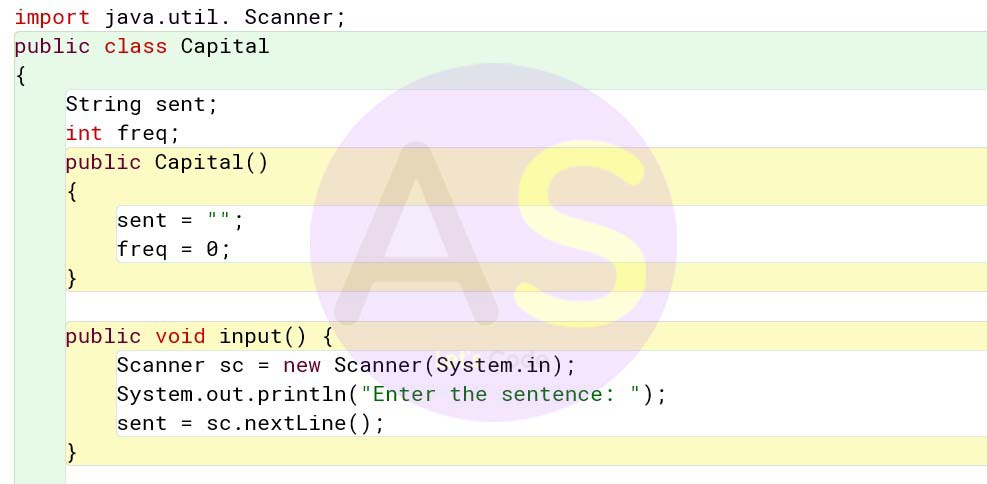
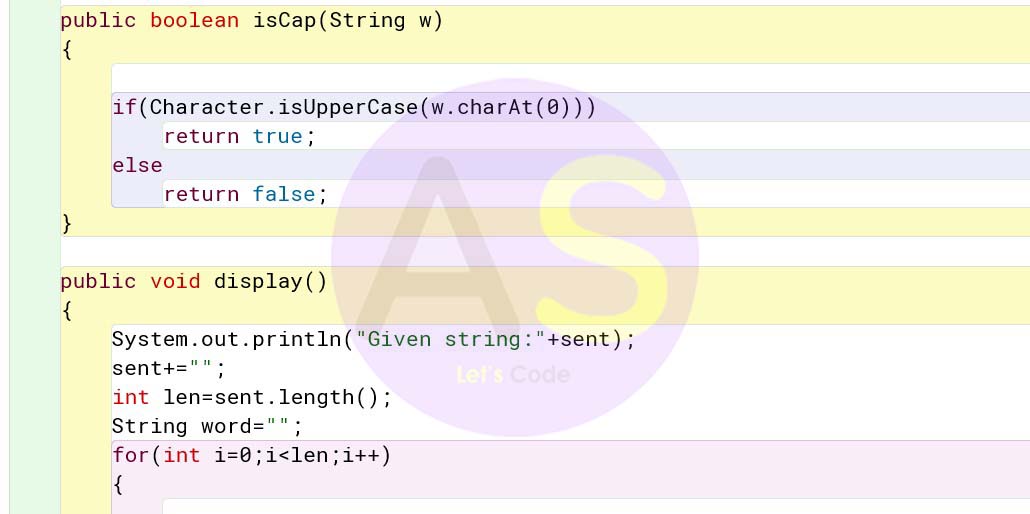
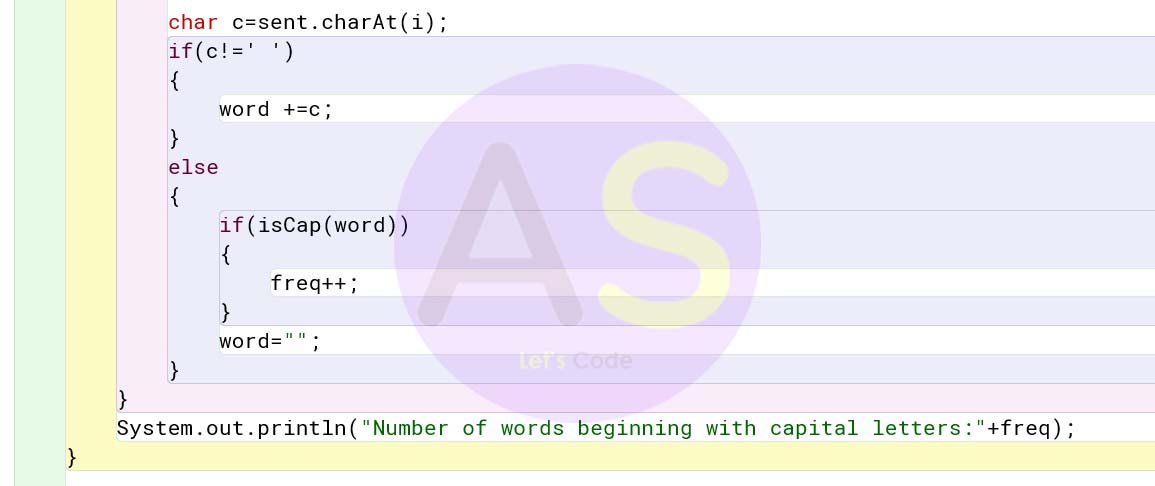
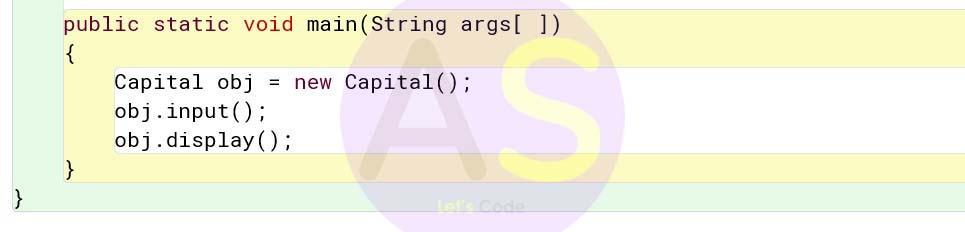
import java.util.*; public class Capital { String sent; int freq; public Capital() { sent = ""; freq = 0; } public void input() { Scanner sc = new Scanner(System.in); System.out.println("Enter the sentence: "); sent = sc.nextLine(); } public boolean isCap(String w) { if(Character.isUpperCase(w.charAt(0))) return true; else return false; } public void display() { System.out.println("Given string:"+sent); sent+=""; int len=sent.length(); String word=""; for(int i=0;i< len;i++) { char c=sent.charAt(i); if(c!=' ') { word +=c; } else { if(isCap(word)) { freq++; } word=""; } } System.out.println("Number of words beginning with capital letters:"+freq); } public static void main(String args[ ]) { Capital obj = new Capital(); obj.input(); obj.display(); } }
Question 10
A superclass Number is defined to calculate the factorial of a number. Define a subclass Series to find the sum of the series
S = 1! + 2! + 3! + 4! + ………. + n!
The details of the members of both classes are given below:
Class name: Number
Data member/instance variable:
n: to store an integer number
Member functions/methods:
Number(int nn): parameterized constructor to initialize the data member n=nn
int factorial(int a): returns the factorial of a number
(factorial of n = 1 × 2 × 3 × …… × n)
void display():displays the data members
Class name: Series
Data member/instance variable:
sum: to store the sum of the series
Member functions/methods:
Series(…) : parameterized constructor to initialize the data members of both the classes
void calsum(): calculates the sum of the given series
void display(): displays the data members of both the classes
Assume that the superclass Number has been defined. Using the concept of inheritance, specify the class Series giving the details of the constructor(…), void calsum() and void display().
The superclass, main function and algorithm need NOT be written.
Solution:
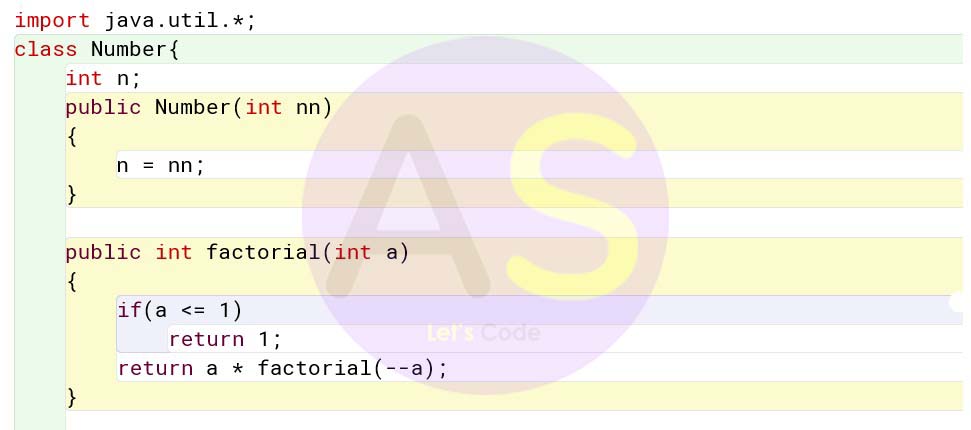
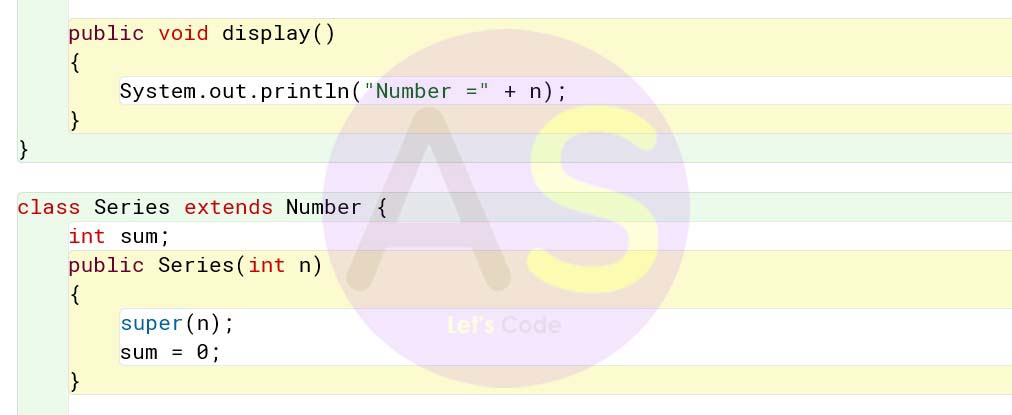
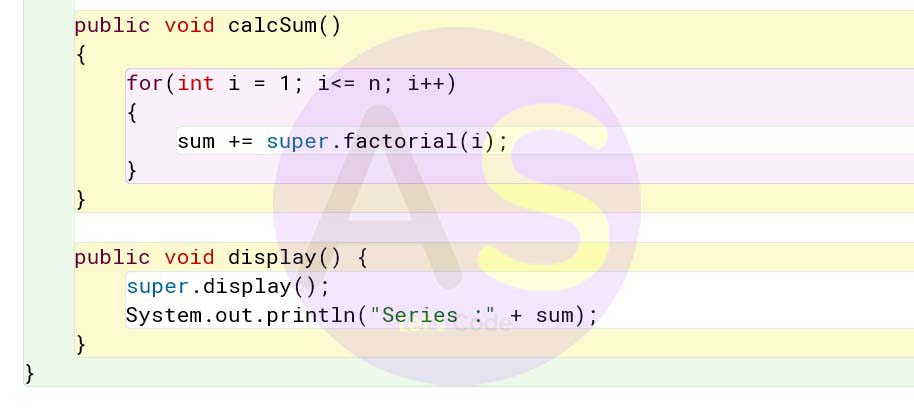
import java.util.*; class Number{ int n; public Number(int nn) { n = nn; } public int factorial(int a) { if(a <= 1) return 1; return a * factorial(--a); } public void display() { System.out.println("Number =" + n); } } class Series extends Number { int sum; public Series(int n) { super(n); sum = 0; } public void calcSum() { for(int i = 1; i<= n; i++) { sum += super.factorial(i); } } public void display() { super.display(); System.out.println("Series :" + sum); } } class Factorial { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number :"); int num = sc.nextInt(); Series obj = new Series(num); obj.calcSum(); obj.display(); } }
Question 11
A register is an entity which can hold a maximum of 100 names. The register enables the user to add and remove names from the topmost end only.
Define a class Register with the following details:
Class name: Register
Data members/instance variables:
stud[]: array to store the names of the students
cap: stores the maximum capacity of the array to point the index of the top end
top: to point the index of the top end
Member functions:
Register (int max) : constructor to initialize the data member cap = max, top = -1 and create the string array
void push(String n): to add names in the register at the top location if possible, otherwise display the message “OVERFLOW”
String pop(): removes and returns the names from the topmost location of the register if any, else returns “$$”
void display (): displays all the names in the register
(a) Specify the class Register giving details of the functions void push(String) and String pop().Assume that the other functions have been defined.
The main function and algorithm need NOT be written.
Solution:
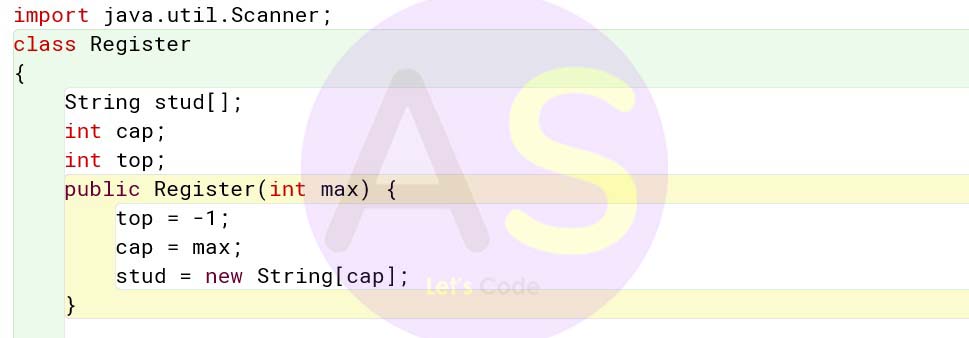
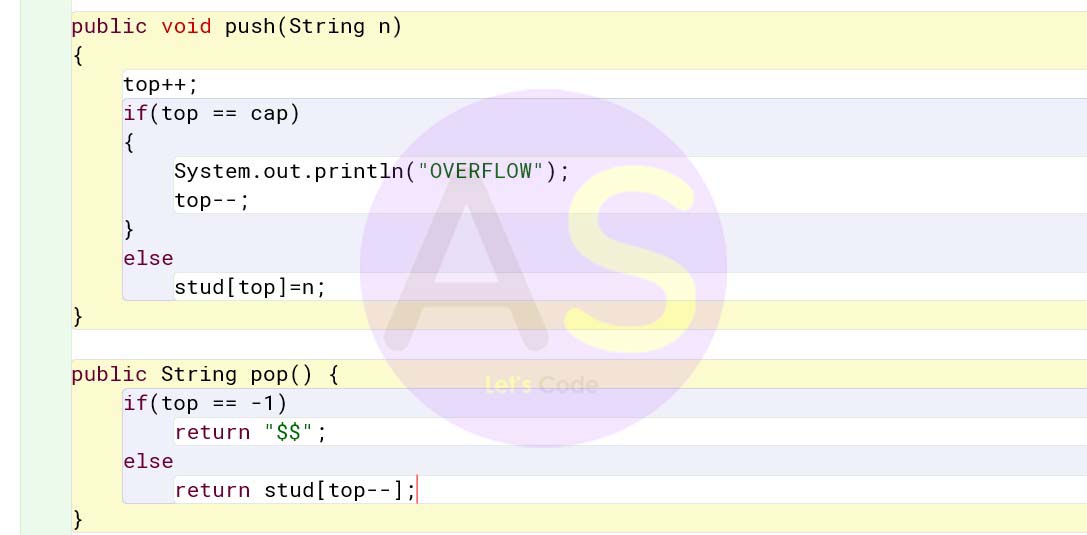
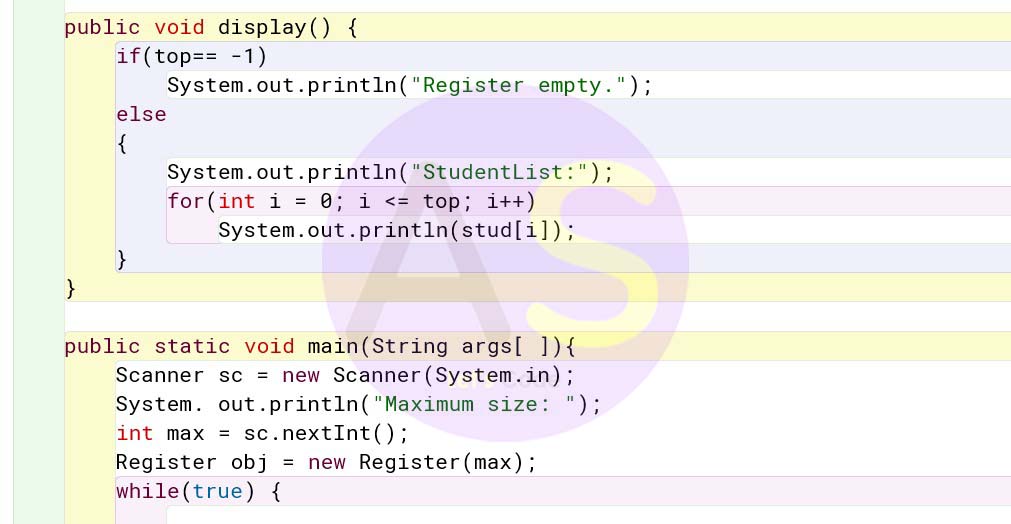
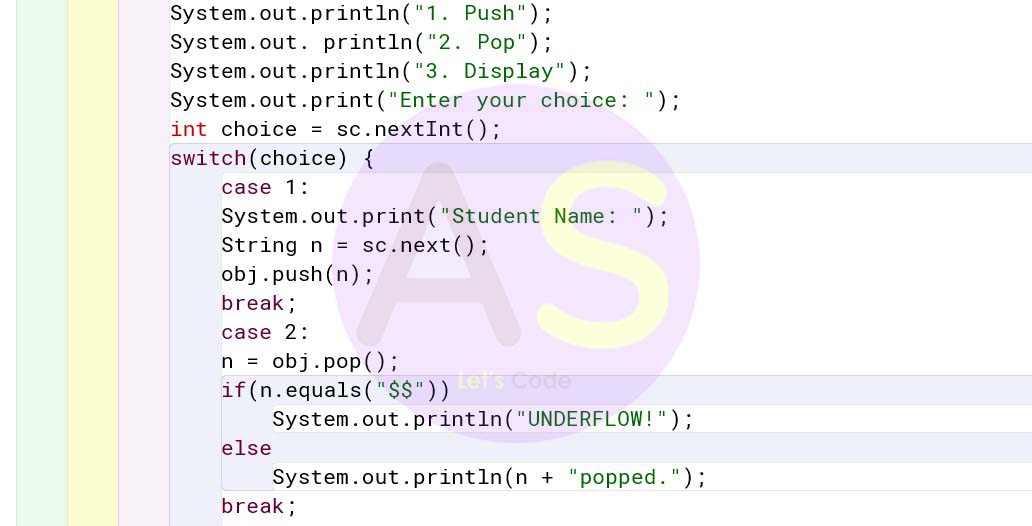
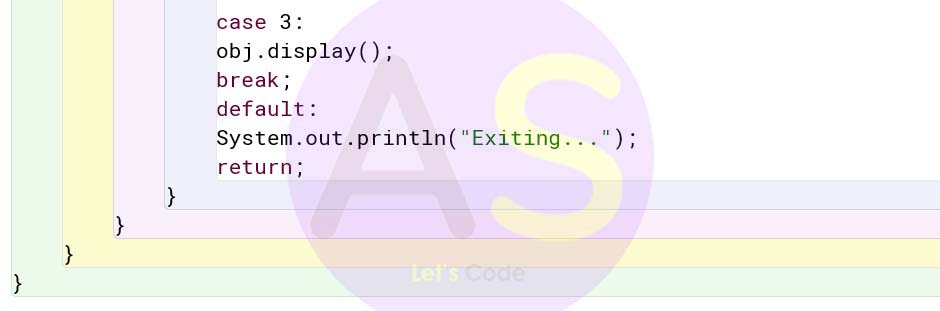
import java.util.*; class Register { String stud[]; int cap; int top; public Register(int max) { top = -1; cap = max; stud = new String[cap]; } public void push(String n) { top++; if(top == cap) { System.out.println("OVERFLOW"); top--; } else stud[top]=n; } public String pop() { if(top == -1) return "$$"; else return stud[top--]; } public void display() { if(top== -1) System.out.println("Register empty."); else { System.out.println("StudentList:"); for(int i = 0; i <= top; i++) System.out.println(stud[i]); } } public static void main(String args[ ]){ Scanner sc = new Scanner(System.in); System. out.println("Maximum size: "); int max = sc.nextInt(); Register obj = new Register(max); while(true) { System.out.println("1. Push"); System.out. println("2. Pop"); System.out.println("3. Display"); System.out.print("Enter your choice: "); int choice = sc.nextInt(); switch(choice) { case 1: System.out.print("Student Name: "); String n = sc.next(); obj.push(n); break; case 2: n = obj.pop(); if(n.equals("$$")) System.out.println("UNDERFLOW!"); else System.out.println(n + "popped."); break; case 3: obj.display(); break; default: System.out.println("Exiting..."); return; } } } }
Question 11
(a) A linked list is formed from the objects of the class Node. The class structure of the Node is given below:
class Node
{
int n;
Node link;
}
Write an Algorithm OR a Method to search for a number from an existing linked list.
The method declaration is as follows:
void FindNode(Node str, int b)
(b) Answer the following questions from the diagram of a Binary Tree given below:
(i) Write the inorder traversal of the above tree structure.
(ii) State the height of the tree, if the root is at level 0 (zero).
(iii) List the leaf nodes of the tree.
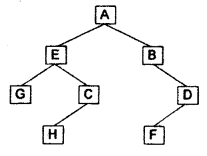