Class 12 ISC- Java
Class 12th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 12th java topics includes revision of class 11th, constructors, user-defined methods, objects and classes, library classes , etc.
Inheritance
Inheritance
In Java, one class can easily inherit the attributes and methods from some other class. This mechanism of acquiring objects and properties from some other class is known as inheritance in Java.
Inheritance is used to borrow properties & methods from an existing class.
Inheritance helps us create classes based on existing classes, which increases the code's reusability.
Let us understand by an example:-
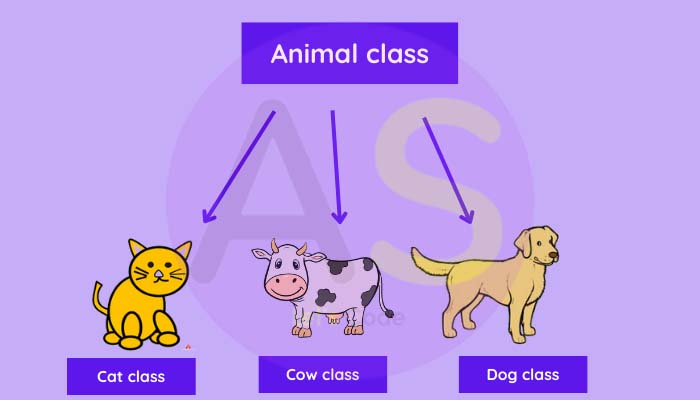
In the above example here animal class is a parent class,and these
dog class, cow
class and cat class are child classes which are derived from animal class,and these child
classes
inherit some common properties
and
Behavior from
animal class.
Important terminologies used in inheritance :
Parent class/Superclass: The class from which a class inherits methods and attributes is known as parent class.
Child class/Sub-class: The class that inherits some other class's methods and attributes is known as child class.
How to achieve inheritance :
The extends keyword is used to inherit a subclass from a superclass
Syntax :
class Subclass-name extends Superclass-name
{
//methods and fields
}
Types of Inheritance
Below are the different types of inheritance which are supported by Java.
1. Single Inheritance:
In single inheritance, subclasses inherit the features of one superclass
2. Multilevel Inheritance:
In Multilevel Inheritance, a derived class will be inheriting a base class and as well as the derived class also act as the base class to other class.
3. Hierarchical Inheritance:
In Hierarchical Inheritance, one class serves as a superclass (base class) for more than one subclass.
4. Multiple Inheritance:
In Multiple inheritances, one class can have more than one superclass and inherit features from all parent classes. Java does not support multiple inheritances with classes. It can only be achieved through Interfaces.
5. Hybrid Inheritance:
Hybrid inheritance is the combination of two or more types of inheritance.
Super Keyword
The super keyword refers to parent class objects.
It is used to call superclass methods, and to access superclass constructor.
It's commonly used to eliminate the confusion between superclasses and subclasses when both have methods with the same name.
Output :
Method overriding
If the same method is defined in both the superclass and the subclass, then the method of the subclass class overrides the method of the superclass. This is known as method overriding.
Output :
Example 1: Write a program to show the working of inheritance.
class baseclass
{
public void firstmethod()
{
System.out.println("Parent class is called");
}
}
class childclass extends baseclass
{
public void secondmethod()
{
System.out.println("child class is called");
}
public static void main(String args[])
{
childclass ob=new childclass();
ob.firstmethod();//super class method is called
ob.secondmethod();//childclass local method is called
}
}
Output :
Parent class is called
child class is called
Example 2 : Write a program to calculate area of rectangle and circle using inheritance concept.
class Shape {
public double getArea() {
return 0;
}
}
class Rectangle extends Shape
{
// extended form the Shape class
double width;
double height;
public Rectangle(double w, double h) {
width = w;
height = h;
}
public double getArea() {
return width * height;
}
}
class Circle extends Shape
{
double radius;
public Circle(double r) {
radius = r;
}
public double getArea() {
return 3.14 * radius * radius;
}
}
class driver {
public static void main(String args[]) {
Circle obj= new Circle(2.2);
Rectangle obj2= new Rectangle(2.3,2.5);
System.out.println("Area of the Circle: " + obj.getArea());
System.out.println("Area of the Rectangle: " + obj2.getArea());
}
}
Output :
Area of the Circle: 15.2053084434
Area of the Rectangle: 5.75
class Subclass-name extends Superclass-name { //methods and fields }
1. Single Inheritance:
In single inheritance, subclasses inherit the features of one superclass
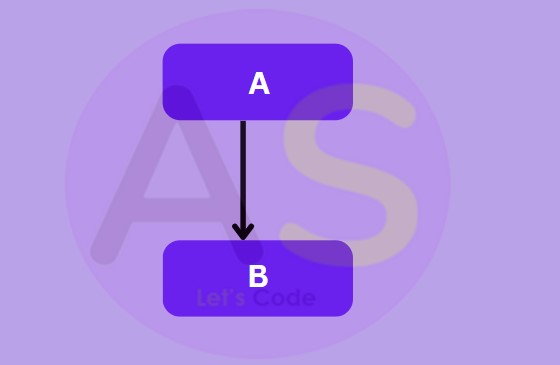
2. Multilevel Inheritance:
In Multilevel Inheritance, a derived class will be inheriting a base class and as well as the derived class also act as the base class to other class.
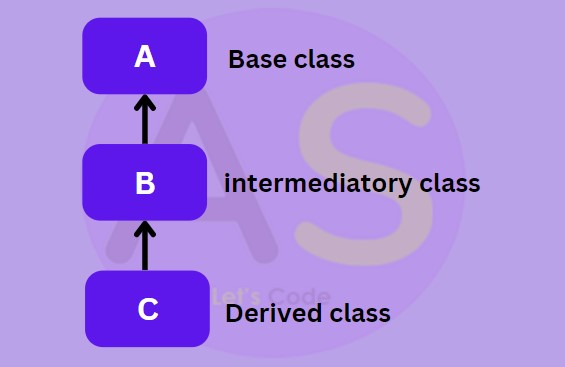
3. Hierarchical Inheritance:
In Hierarchical Inheritance, one class serves as a superclass (base class) for more than one subclass.
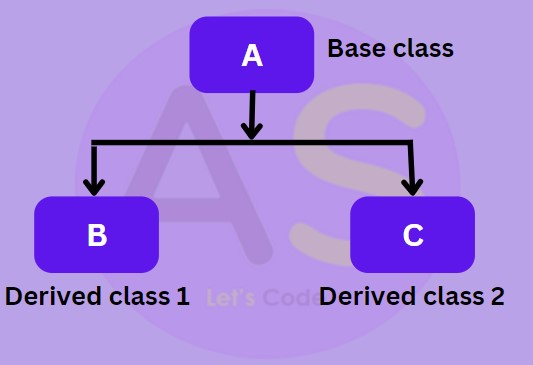
4. Multiple Inheritance:
In Multiple inheritances, one class can have more than one superclass and inherit features from all parent classes. Java does not support multiple inheritances with classes. It can only be achieved through Interfaces.
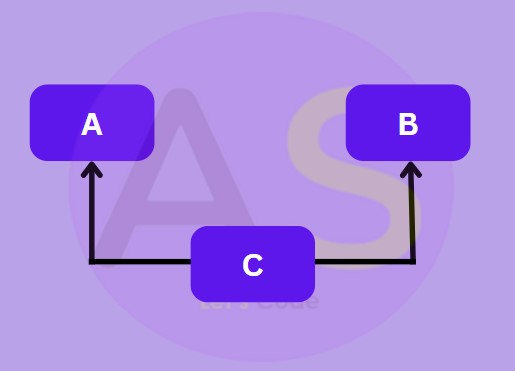
5. Hybrid Inheritance:
Hybrid inheritance is the combination of two or more types of inheritance.
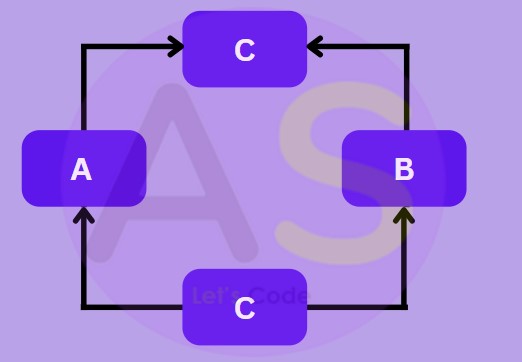
Super Keyword
The super keyword refers to parent class objects.
It is used to call superclass methods, and to access superclass constructor.
It's commonly used to eliminate the confusion between superclasses and subclasses when both have methods with the same name.
Output :
Method overriding
If the same method is defined in both the superclass and the subclass, then the method of the subclass class overrides the method of the superclass. This is known as method overriding.
Output :
Example 1: Write a program to show the working of inheritance.
class baseclass
{
public void firstmethod()
{
System.out.println("Parent class is called");
}
}
class childclass extends baseclass
{
public void secondmethod()
{
System.out.println("child class is called");
}
public static void main(String args[])
{
childclass ob=new childclass();
ob.firstmethod();//super class method is called
ob.secondmethod();//childclass local method is called
}
}
Output :
Parent class is called
child class is called
Example 2 : Write a program to calculate area of rectangle and circle using inheritance concept.
class Shape {
public double getArea() {
return 0;
}
}
class Rectangle extends Shape
{
// extended form the Shape class
double width;
double height;
public Rectangle(double w, double h) {
width = w;
height = h;
}
public double getArea() {
return width * height;
}
}
class Circle extends Shape
{
double radius;
public Circle(double r) {
radius = r;
}
public double getArea() {
return 3.14 * radius * radius;
}
}
class driver {
public static void main(String args[]) {
Circle obj= new Circle(2.2);
Rectangle obj2= new Rectangle(2.3,2.5);
System.out.println("Area of the Circle: " + obj.getArea());
System.out.println("Area of the Rectangle: " + obj2.getArea());
}
}
Output :
Area of the Circle: 15.2053084434
Area of the Rectangle: 5.75
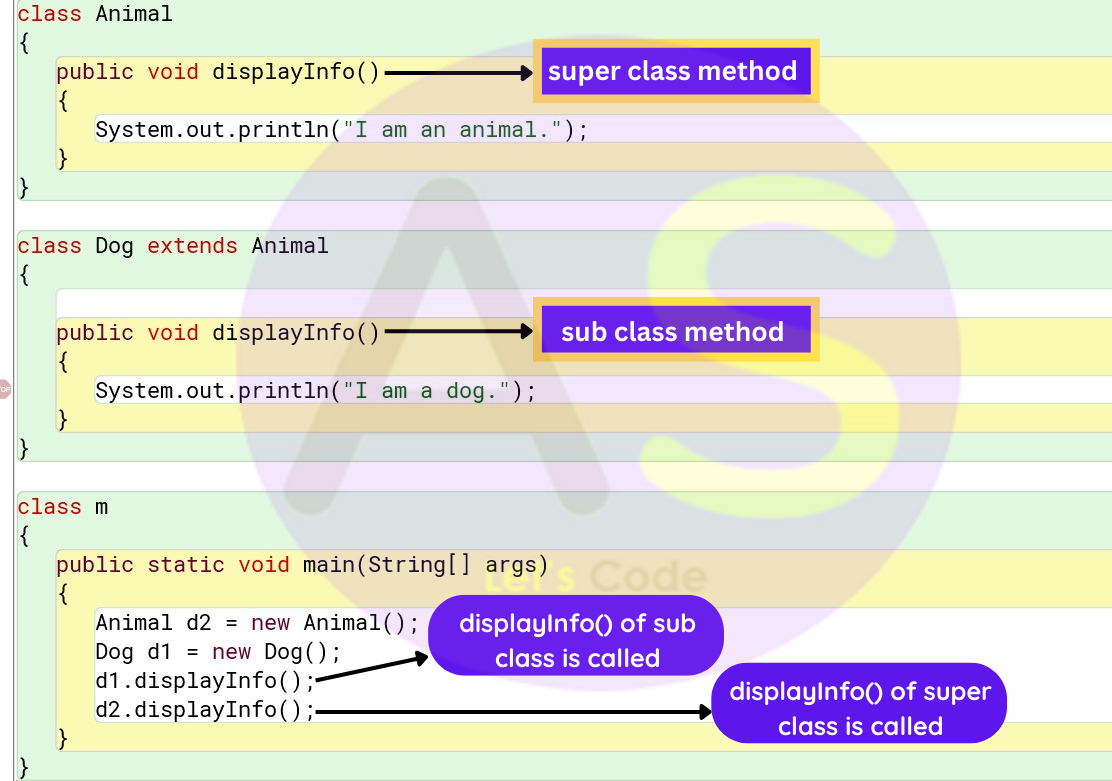
Output :
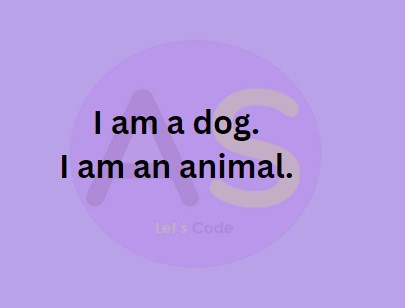
Example 1: Write a program to show the working of inheritance.
class baseclass { public void firstmethod() { System.out.println("Parent class is called"); } } class childclass extends baseclass { public void secondmethod() { System.out.println("child class is called"); } public static void main(String args[]) { childclass ob=new childclass(); ob.firstmethod();//super class method is called ob.secondmethod();//childclass local method is called } }
Output :
Parent class is called child class is called
Example 2 : Write a program to calculate area of rectangle and circle using inheritance concept.
class Shape { public double getArea() { return 0; } } class Rectangle extends Shape { // extended form the Shape class double width; double height; public Rectangle(double w, double h) { width = w; height = h; } public double getArea() { return width * height; } } class Circle extends Shape { double radius; public Circle(double r) { radius = r; } public double getArea() { return 3.14 * radius * radius; } } class driver { public static void main(String args[]) { Circle obj= new Circle(2.2); Rectangle obj2= new Rectangle(2.3,2.5); System.out.println("Area of the Circle: " + obj.getArea()); System.out.println("Area of the Rectangle: " + obj2.getArea()); } }
Output :
Area of the Circle: 15.2053084434 Area of the Rectangle: 5.75