Class 11 CBSE - Python
Python is designed to understand the user’s responsibility, the protocol, and the ethical issues related to the field of computing.
Python in class 11 for cbse is very handy when it comes to practice on computers but for the beginers there might be some errors and problems such as exception. But it's always advisable to practice program on computer than writing it on paper because it cherishes your practical skills , knowledge and typing speed and you'll come to know new things and if you want to be a software developer someday then its a must call for you.
Lists
A list is a collection of comma-separated values within square brackets. Python lists allow us to work with multiple types of elements at once. List are mutable i.e. values in the list can be modified which means new list will not created when you make changes to an element of a list
For Example :
# a list of different languages
['French', 'English', 'Hindi']
# a list of different languages ['French', 'English', 'Hindi']
Creating Lists
In Python, a list is created by placing elements inside square brackets [], separated by commas.
# list of integers
l = [1, 2, 3]
A list can have any number of items and they can be of different types (integer, float, string, etc.).
# empty list
l = []
# list with different data types
l1 = [1, "Hello", 3.4]
A list can also have another list inside it. This is called a nested list.
# nested list
l = ["Hello", [8, 4, 6], ['a','b','c']]
# list of integers l = [1, 2, 3]
# empty list l = [] # list with different data types l1 = [1, "Hello", 3.4]
# nested list l = ["Hello", [8, 4, 6], ['a','b','c']]
Accessing List Elements
There are various ways in which we can access the elements of a list.
Indexing :
In Python, indexing starts from 0. We can access an item in a list through []. So, a list having 5 elements will have an index from 0 to 4.
The index must be an integer. We can't use float or other types, this will result in "TypeError".
Nested lists are accessed using nested indexing.
Let us see the Example below :
Output :
Negative Indexing :
Python also allows negative indexing for lists . The index of -1 refers to the last item, -2 to the second last item and so on.
Let us see the Example below :
Output :
Slicing :
In Python we can access a section of items from the list through slicing using [:] operator. It is an operation in which we slice a particular range of items from that list. A slice of a list is basically its sub-list.
Let us see the Example below :
Output :
Here,
- mylist[2:5] returns a list with items from index 2 to index 4.
- mylist[5:] returns a list with items from index 5 to the end.
- mylist[:] returns all list items
Note : When we slice lists, the start index is included but the end index is excluded (i.e. including start index to (end index-1)).
Membership Testing :
Membership Testing is an operation to check if an item exists in the list or not using 'in' keyword. For example,
Let us see the Example below :
Here,
- 'Hindi' is not present in lang, 'Hindi' in lang evaluates to False.
- 40 is present in lang, 40 in lang evaluates to True.
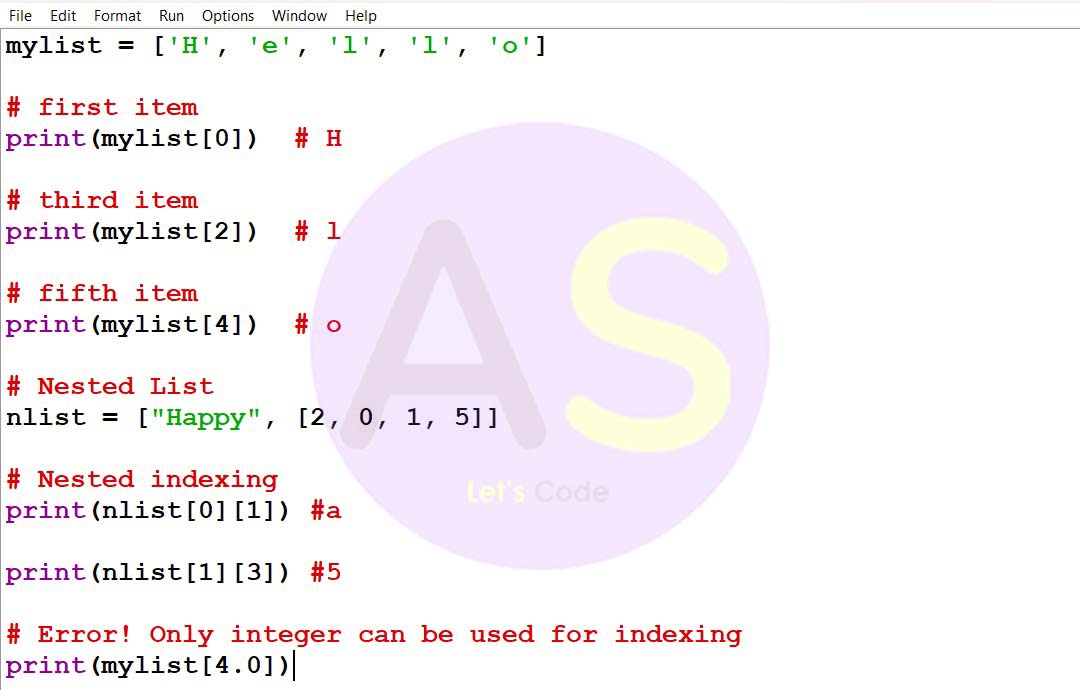

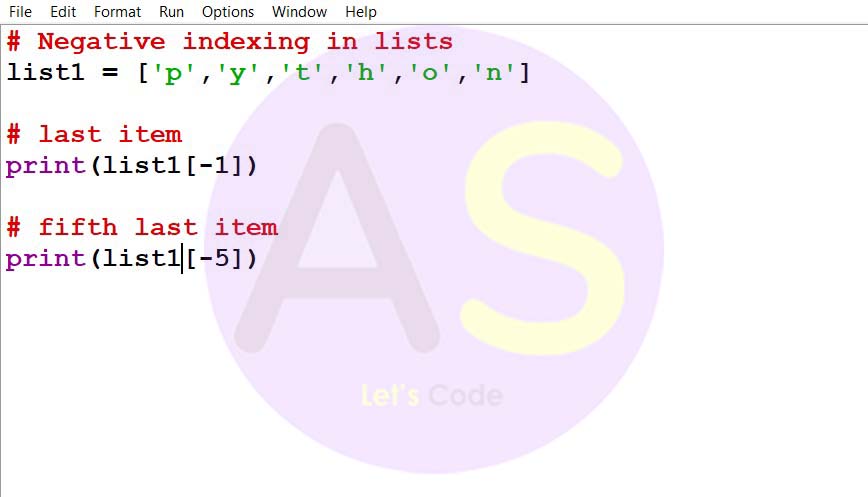

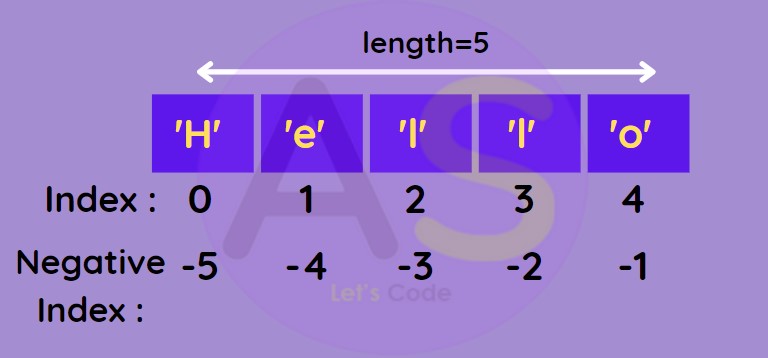
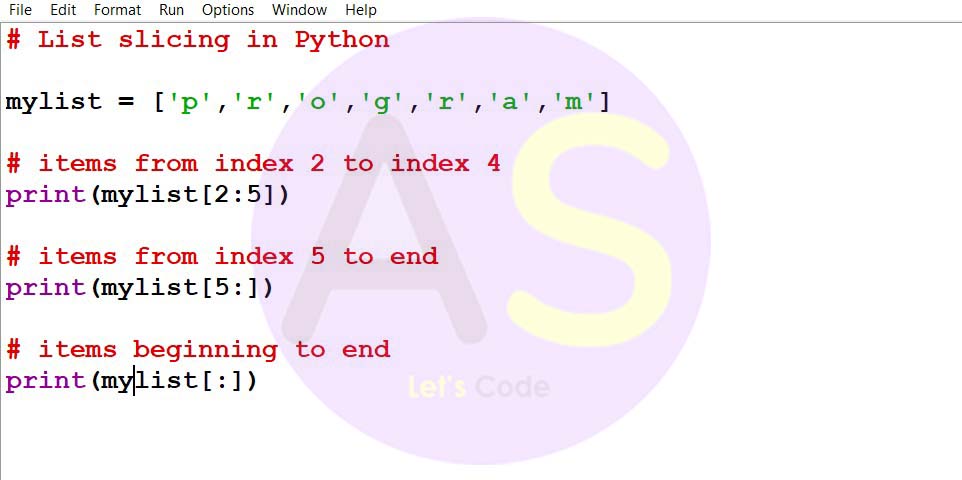

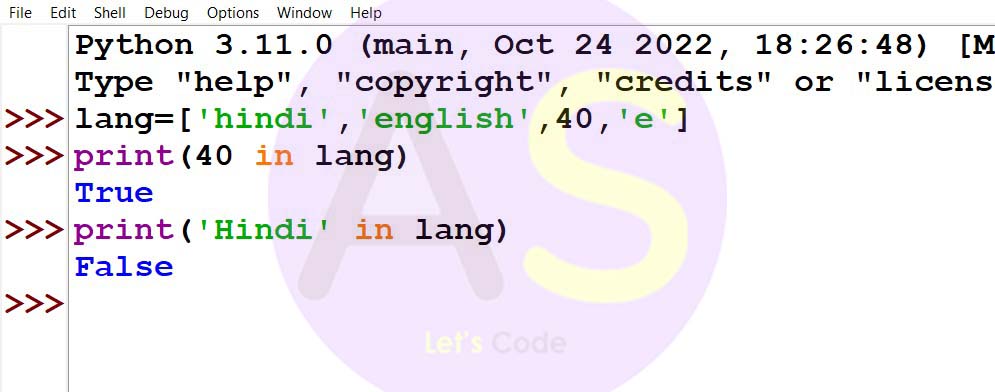
len() Function
In Python, we use the len() method to find the number of elements present in a list.
For Example,
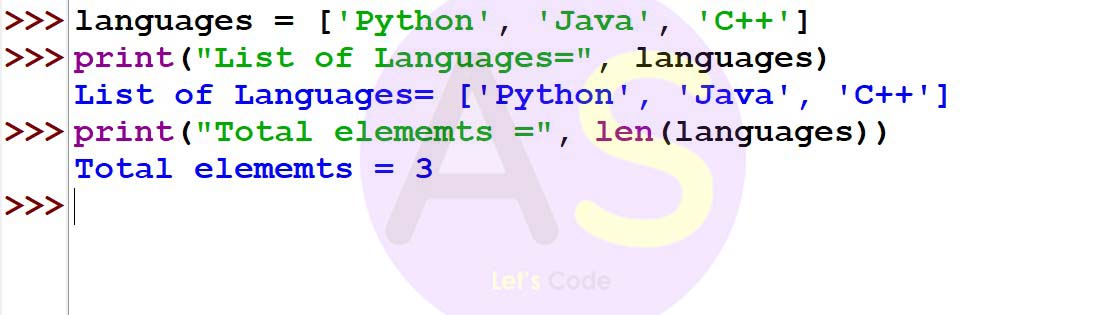
Python List Methods
Python has many useful list methods that makes it really easy to work with lists.
Built-in Methods of Python Lists
insert()
This function is used to insert an element at a specified index. This function takes 2 argument the index where an item to be inserted and the item itself
For example,
extend()
We use the extend() method to add all items of one list to another.
For example,
index()
It returns the index of first matched item from the list. It returns the first occurrence of an item for which the index position is to be searched in the list.
For example,
remove()
It removes the first occurrence of item from the list. A ValueError exception is raised if item is not found in the list.
For example,
sort()
Sort the elements of the list in ascending order (i.e. from the lowest value to the highest value). By default it sorts the element in ascending order, if we want to sort the list in descending order we need to write
>>> list_name.sort(reverse=True)
For example,
reverse()
It reverses the order of the elements in a list. This function reverses the item of the list. It replaces a new value "in place" of item that already exists in the list i.e. it does not create a new list.
For example,
count()
It counts how many times an element has occurred in a list and returns it.
For example,
pop()
It remove the element from the specified index and also returns the element which was removed
For example,
This function is used to insert an element at a specified index. This function takes 2 argument the index where an item to be inserted and the item itself
For example,
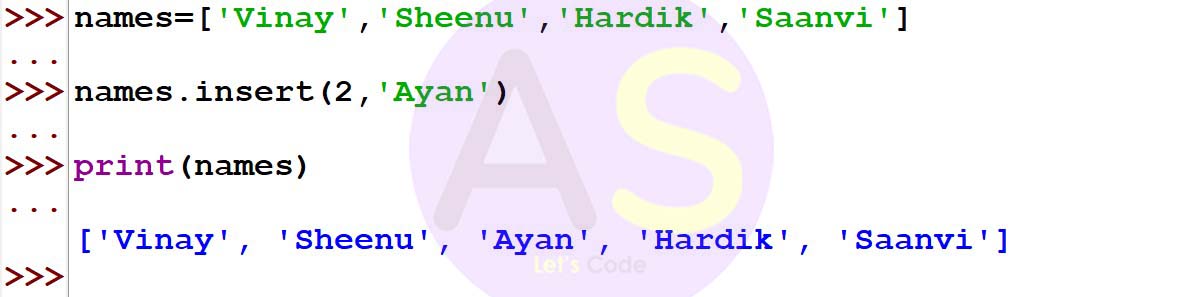
We use the extend() method to add all items of one list to another.
For example,
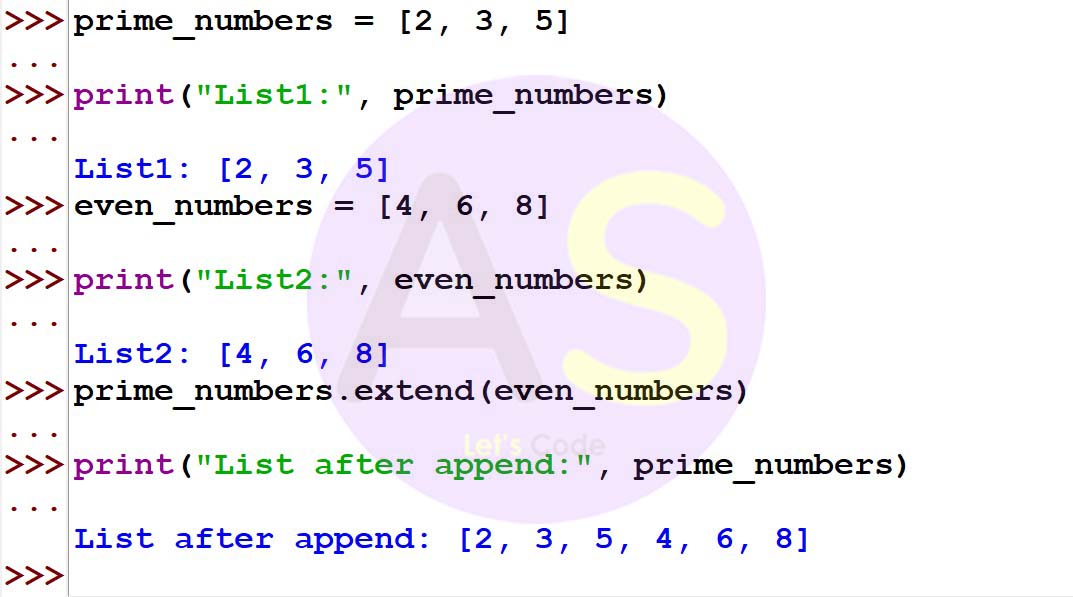
It returns the index of first matched item from the list. It returns the first occurrence of an item for which the index position is to be searched in the list.
For example,
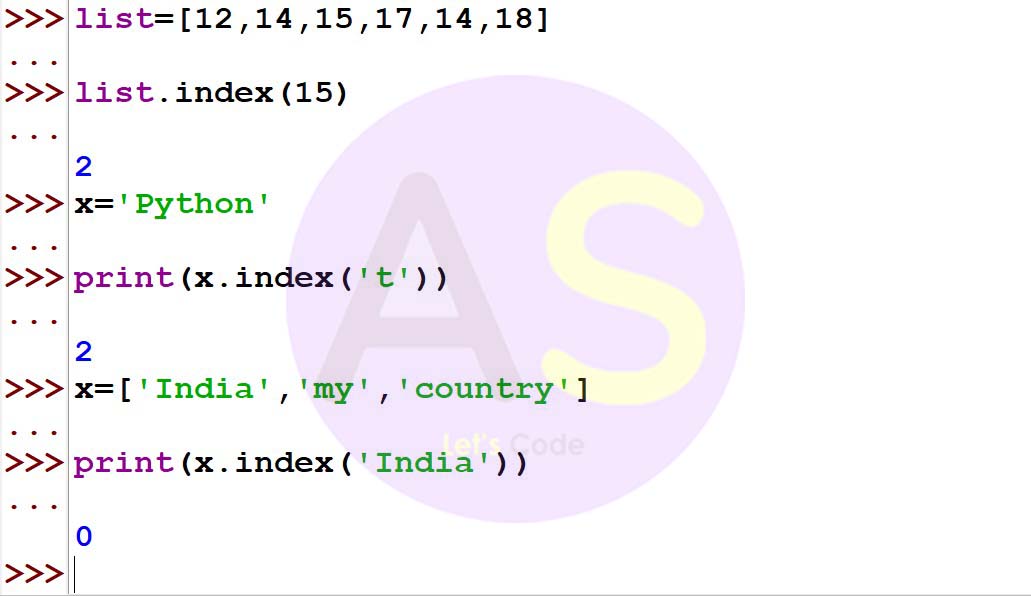
It removes the first occurrence of item from the list. A ValueError exception is raised if item is not found in the list.
For example,
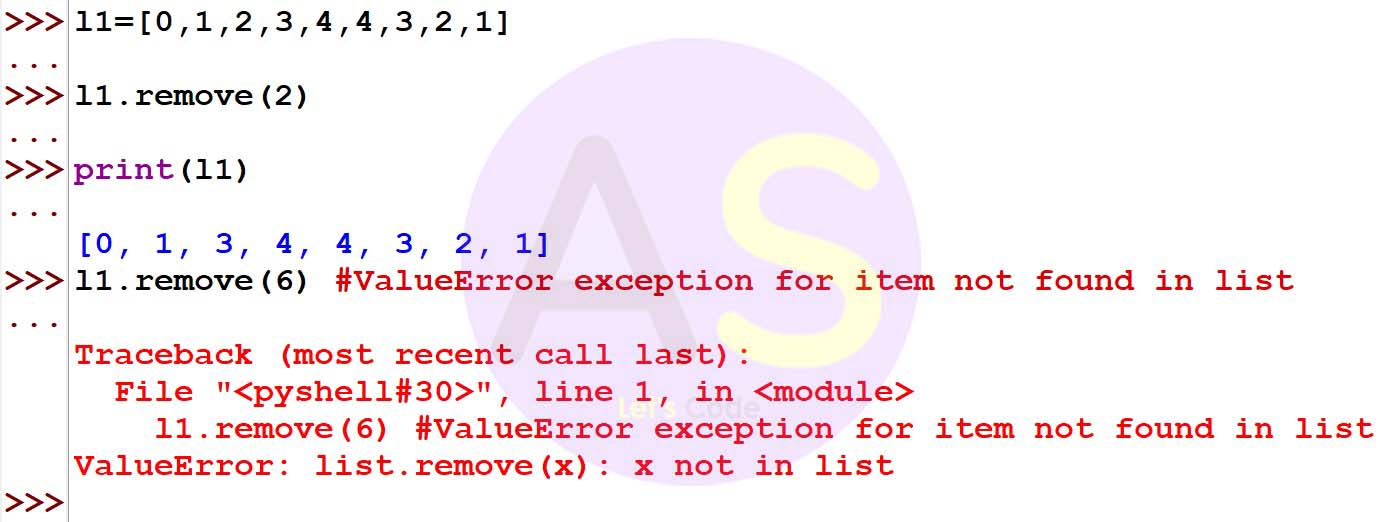
Sort the elements of the list in ascending order (i.e. from the lowest value to the highest value). By default it sorts the element in ascending order, if we want to sort the list in descending order we need to write
>>> list_name.sort(reverse=True)
For example,
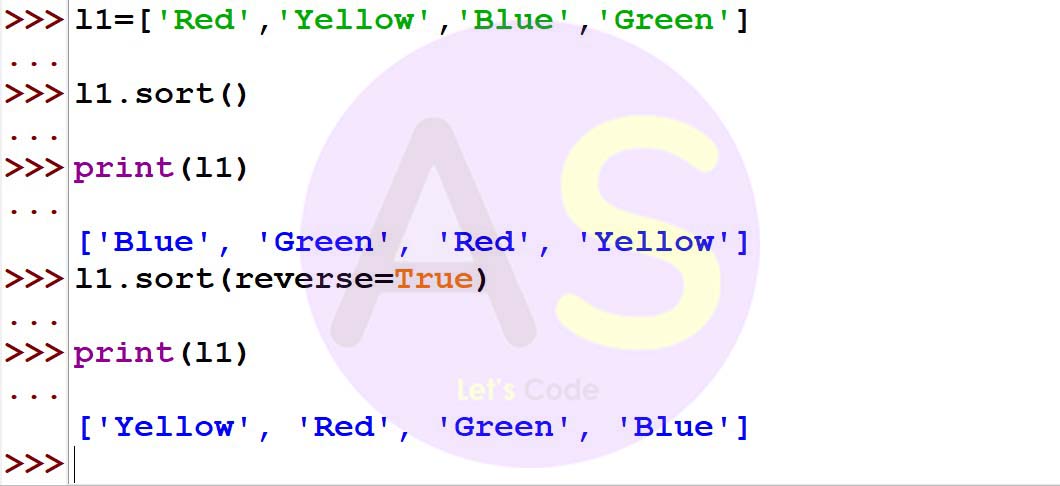
It reverses the order of the elements in a list. This function reverses the item of the list. It replaces a new value "in place" of item that already exists in the list i.e. it does not create a new list.
For example,
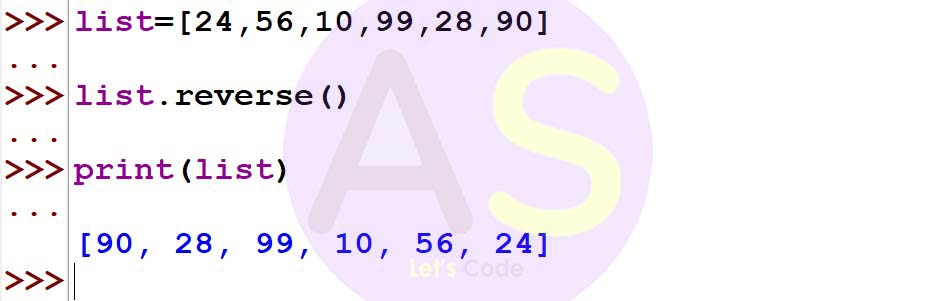
It counts how many times an element has occurred in a list and returns it.
For example,
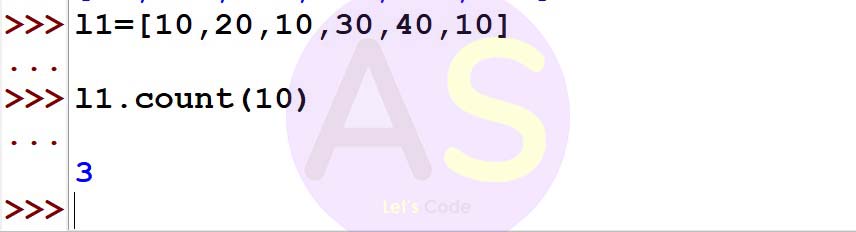
It remove the element from the specified index and also returns the element which was removed
For example,
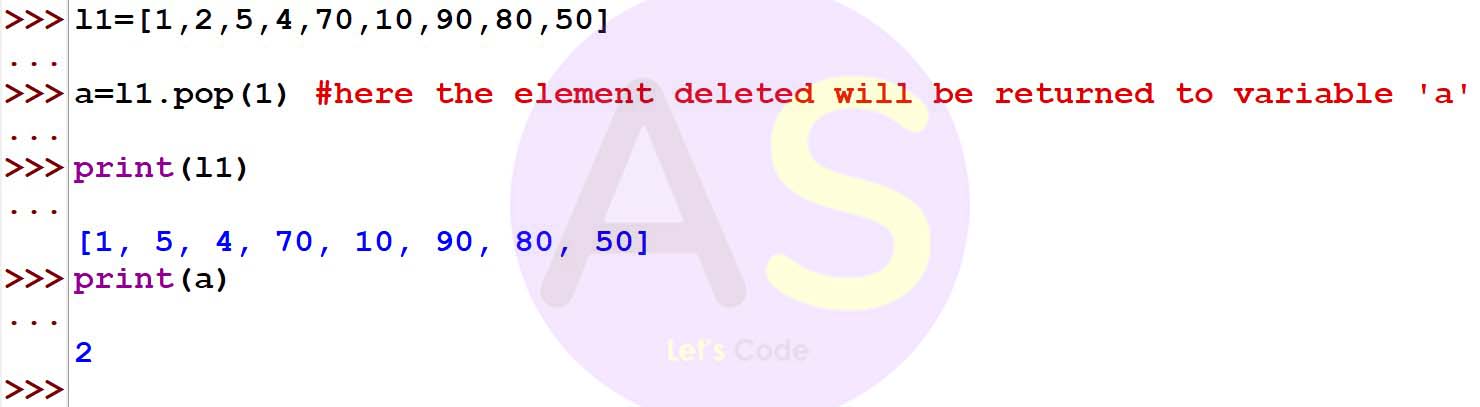
del Statement
del statement remove the specified element from the list but does not return the deleted value.
del statement with slicing :
Consider the following example below :
The above del statement removed 3rd and 4th element from the list. As we know that slice select all the elements from 2nd index to 3rd index.
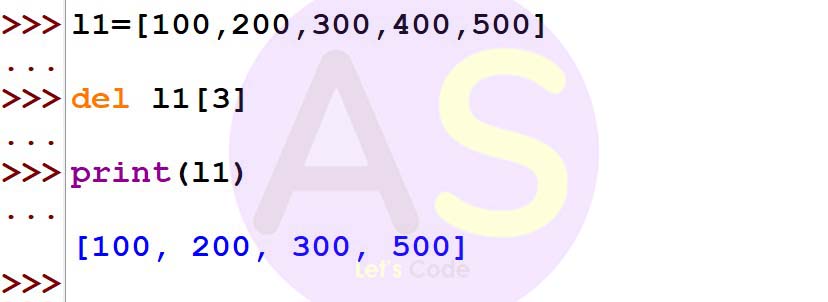
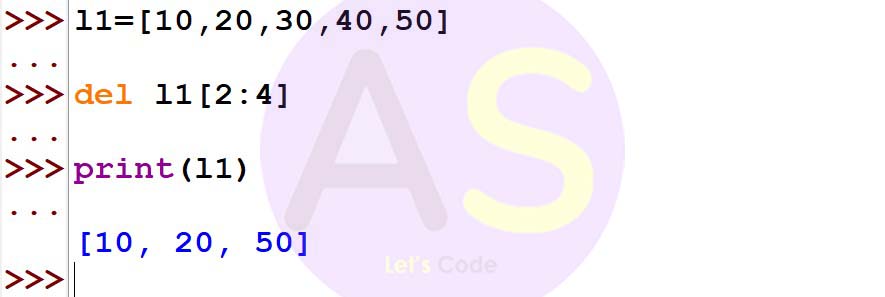