Class 11 CBSE - Python
Python is designed to understand the user’s responsibility, the protocol, and the ethical issues related to the field of computing.
Python in class 11 for cbse is very handy when it comes to practice on computers but for the beginers there might be some errors and problems such as exception. But it's always advisable to practice program on computer than writing it on paper because it cherishes your practical skills , knowledge and typing speed and you'll come to know new things and if you want to be a software developer someday then its a must call for you.
Error & Execption Handling
Whenever a program is written for solving any problem or for executing any particular task ,it may behave abnormally or unexpectedly because of some errors. An error also termed as a 'bug' is anything in the code that prevent a program from compiling and running correctly.
In Python there are 3 kinds of errors :
- Compile-time errors
- Runtime errors
- Exceptions
Compile-time errors
As the name suggests, the errors that occurs while compilation or at the compile-time are called compile-time errors. These error occurs when you violate the rules of writing syntax.
Most frequent compile time errors are :
(i) Missing parenthesis
(ii) Printing the value of variable without declaring it.
Compile-time errors are classified into 2 categories :
- Syntax errors
- Semantic errors
Syntax errors :
Syntax error are the errors that occur due to the incorrect format of a Python statement. You can understand it as a structure of rules and conventions that you have to follow to construct that structure.
For Example :
In the above example, we can see a syntax error. We know that in Python, the syntax for if statement is:
if(condition):
In the example “:” was missing, hence the compilation stopped and there was an error. The correct code would be:
Semantic errors :
Semantics refers to the meaning of a statement. So, when a statement doesn’t make any sense and is not meaningful then we can say it is a Semantic error.
For Example :
In the above example, we can see a syntax error but actually it's a semantic error, because the statement: 'sum + n = sum'
doesn’t make any sense. This is because an expression cannot come on the left side of an assignment according to Python language’s rule.
The correct statement would be: sum = sum + n
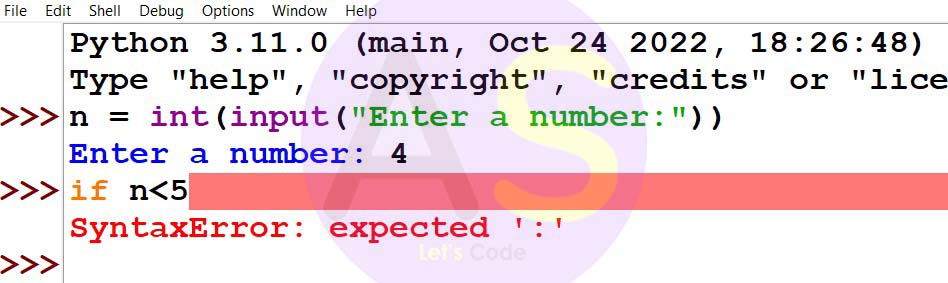
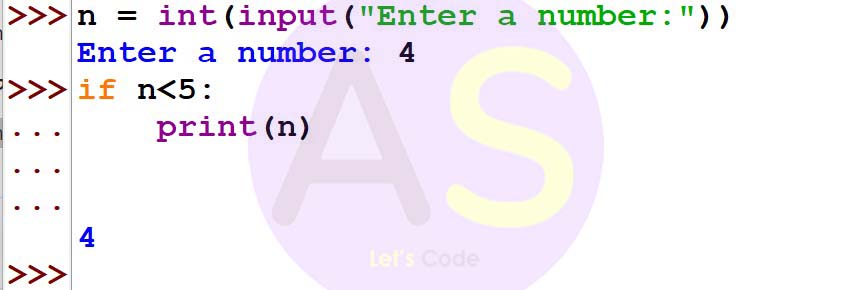
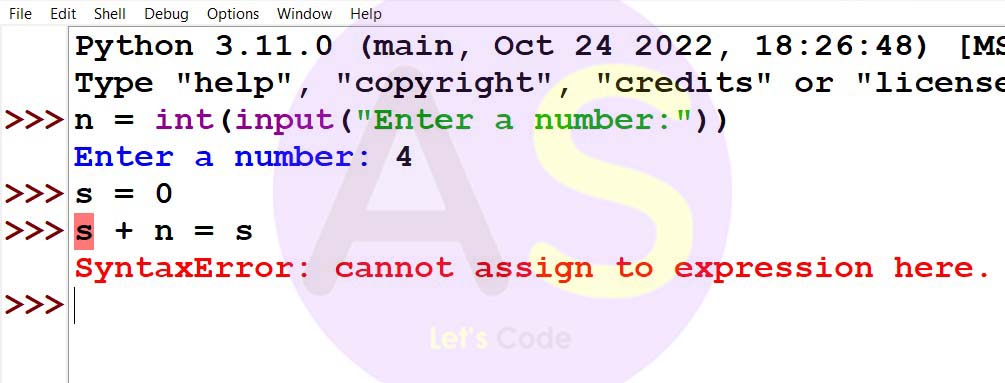
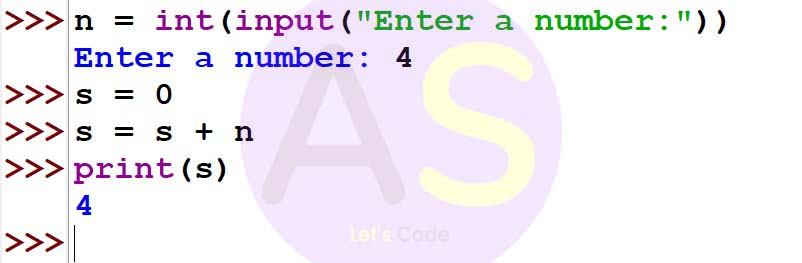
Runtime Errors
This type of errors occurs after the compilation of the code, when execution is going on. Due to which the program suddenly stops running and displays an error dialog box.
For Example :
- Divide by zero
- Invalid function call
- Wrong value as input
- Infinite loop
The above statement is syntactically correct but won't let yield any output and will generate an error because division of any number by zero results in an error and leads to the illegal program termination.
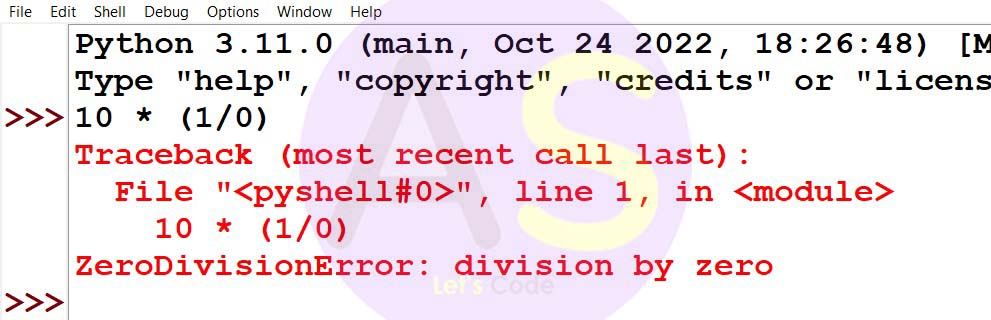
What is an exception ?
Errors occurred during execution are called exception. In other words, an exception is an error that occurs while a program is running. They indicate that something unusual has happened in the program.
Thus, an exception can be described as an interruption during the normal flow of the execution of a program, it is to be handled carefully, when an error occurs an exception is raised in Python.
Let us discuss few important terms related to Exception handling :
- Exception : an exception is an error that occurs while a program is running. They indicate that something unusual has happened in the program.
- Exception Handling : Python provides as a way to handle exception so that the programme doesn't terminate but execute some specified code when an exception occurs. This concept is called Exception Handling.
- Traceback : The lengthy error message that is shown when an exception occurs during the program run is called traceback.
- 'Try' block : Exceptional handling in Python is done using try statement. Try block constitutes a set of code that might have an exception thrown in it, if any code within the try statement causes an error execution of the code will stop and it will jump to the except statement.
- 'except' block or 'catch' block : Whenever some error exception is to be handled, it is done using the except block which stands for exception.
- 'throw' or 'raise' block : 'throw' is an action in response to an exception (error/unusual condition).
- Unhandled, uncaught exception : An exception which leads to abnormal termination of a program due to non-execution of exception thrown is termed as unhandled or uncaught exception.
Common Python Exceptions
NameError
This type of exception occurs when you are trying to use a variable that does not exist in the current environment.
For example,
TypeError
This type of error raised when an operation or function is attempted that is invalid for the specified data type.
For example,
ValueError
This exception is raised when we try to perform operation on incorrect type of value.
For example,
ZeroDivisionError
This exception is raised when division or modulo by zero takes place for all numeric types.
For example,
AttributeError
This exception is raised when an object does not find an attribute.
For example,
KeyError
This type of error occurs when you are trying to access an element of a dictionary
using a key that the dictionary does not contain.
For example,
IndentationError
Indentation is one of the important factors that should be taken care of
while writing Python programs. Each statement written in the block should have the same
indentation level. Violation of this rule results in indentation error.
For example,
IOError
This exception occurs whenever there is an error related to input/output such
as opening a file which does not exist, trying to delete a file in use, removing USB while
read/write operation is going on
For example,
IndexError
This exception is raised when an index is not found in a sequence. In
other words, it occurs whenever we try to access an index that is out of a valid range.
For example,
This type of exception occurs when you are trying to use a variable that does not exist in the current environment.
For example,
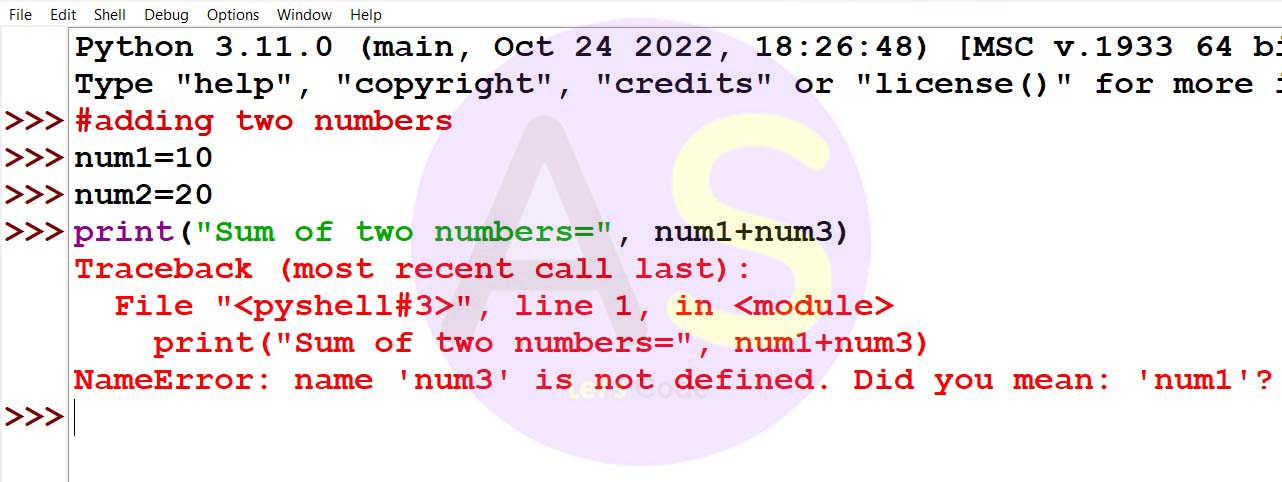
This type of error raised when an operation or function is attempted that is invalid for the specified data type.
For example,
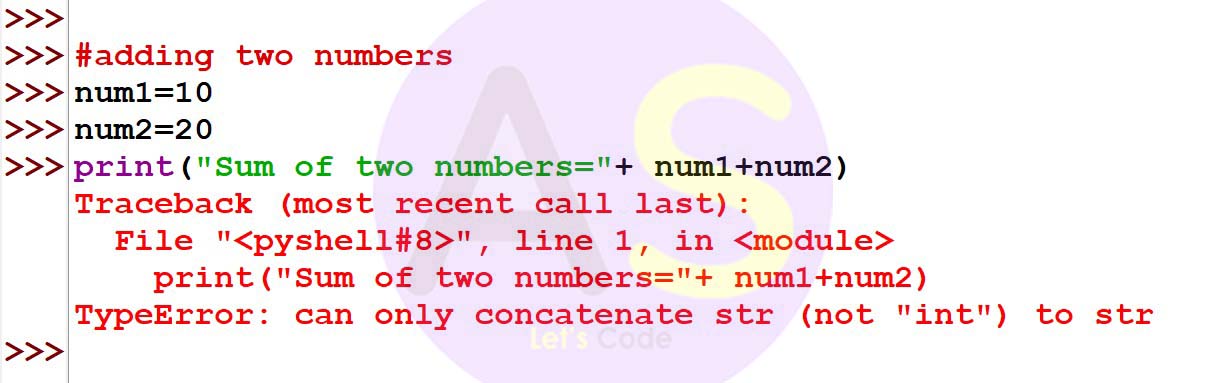
This exception is raised when we try to perform operation on incorrect type of value.
For example,
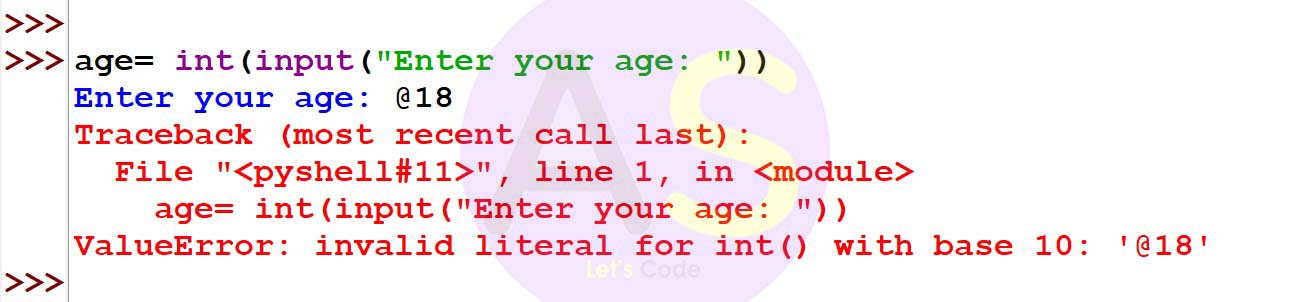
This exception is raised when division or modulo by zero takes place for all numeric types.
For example,
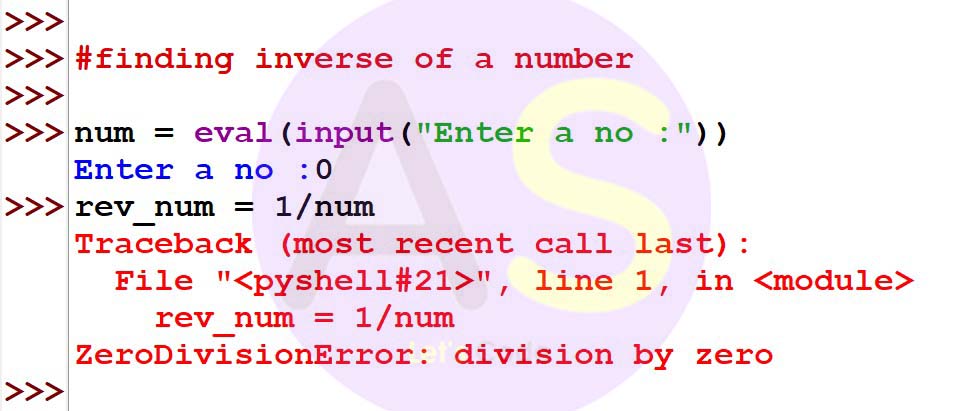
This exception is raised when an object does not find an attribute.
For example,
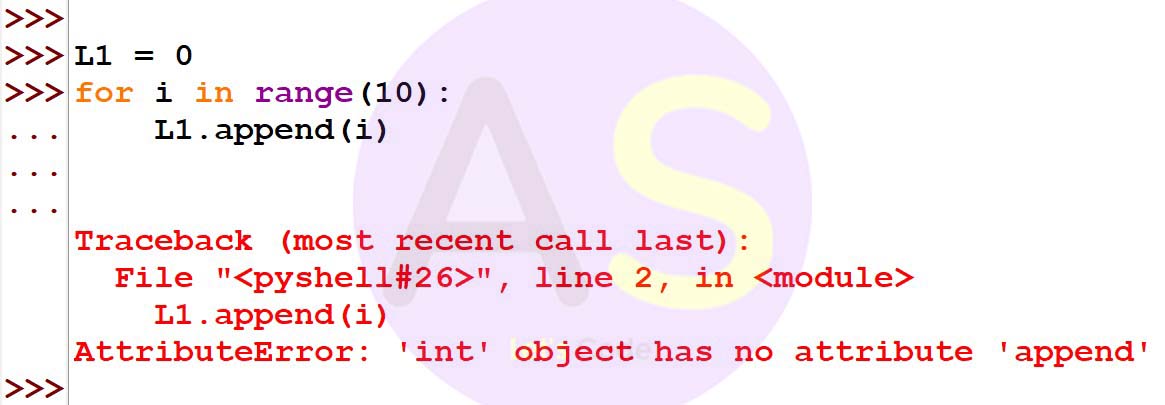
This type of error occurs when you are trying to access an element of a dictionary using a key that the dictionary does not contain.
For example,
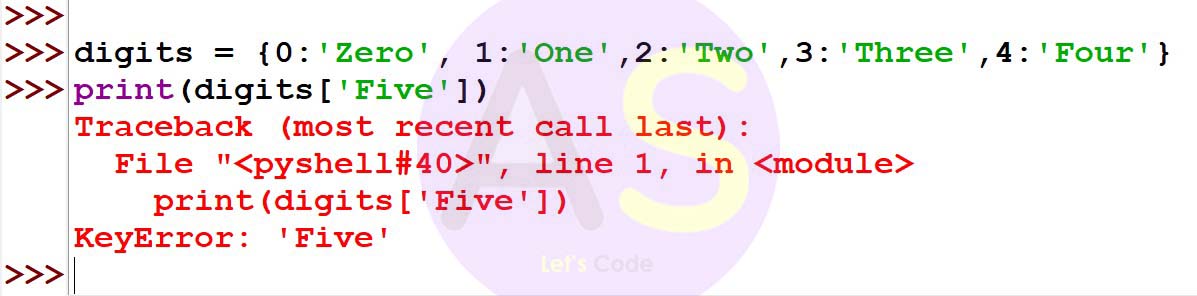
Indentation is one of the important factors that should be taken care of while writing Python programs. Each statement written in the block should have the same indentation level. Violation of this rule results in indentation error.
For example,
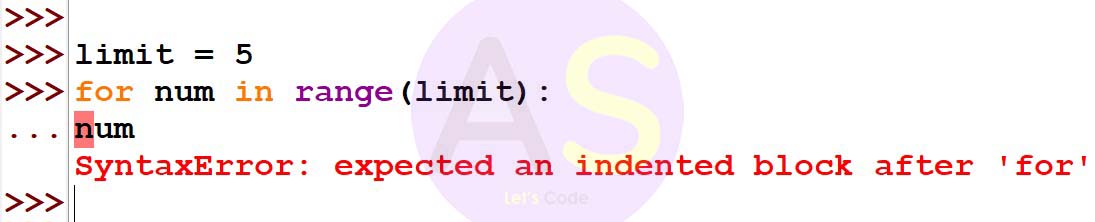
This exception occurs whenever there is an error related to input/output such as opening a file which does not exist, trying to delete a file in use, removing USB while read/write operation is going on
For example,

This exception is raised when an index is not found in a sequence. In other words, it occurs whenever we try to access an index that is out of a valid range.
For example,
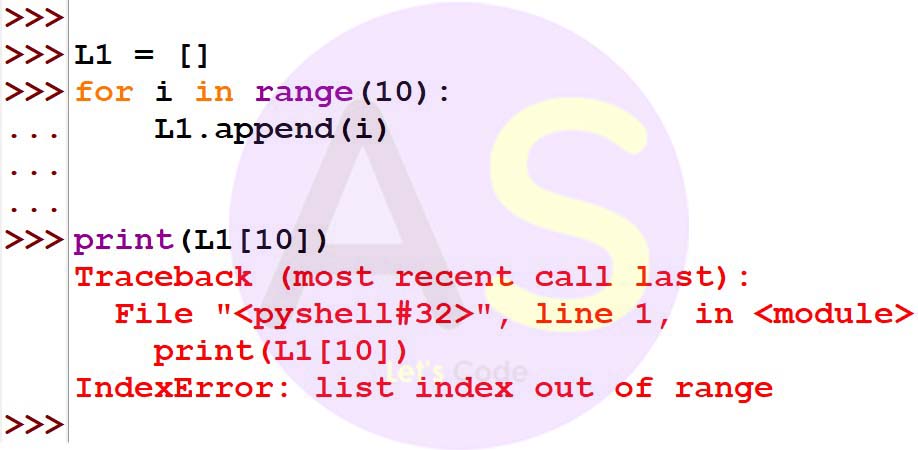
Handling Exception in Python
Python raises exceptions whenever there is an error at run-time .This is a point where your program crashes.That is the interpreter stops the execution of program and displays a traceback which includes error name,its description and a line number,point of the occurrence of the error.
And, the process of handling these errors is called exception handling
Python provides various exception handling techniques ,let us discuss these techniques summarily
Using try and except block
When an error occurs,python stops the program abruptly and generate an exception message.So it highly recommended to handle exceptions
So to handle exceptions, try and except block in python is used.try block contains the code which python executes as a normal part of the program.
And the except block contains the response corresponding to any exceptions in the preceding try block
Syntax:
All the risky and suspicious code that can raise an exception should be placed inside the try block
Else block is also used along with the try and except block, the code in the else block is only executed when there is no exception
Syntax:
When an exception occurs in try block,the execution of try block stops and transferred down to the except block
Let us see the examples of the above concept:
Program1 :Using try except block
Output:
Program2 :Using try except and else block
Output:
Multiple except block
We can also handle multiple exceptions raised by try block,by writing multiple except clauses with exception names to handle specific exceptions, as shown below:
At the occurance of an exception this script will work as:
- Control is transferred to the corresponding except clause to handle the exception occurred
-
If that particular exception is not specified in any except clause. Then by default except will execute without any exception name
-
If neither of the above two cases is there,then the program stops abruptly.
Example:
Program1 :Using Multiple except block
Output:
- Control is transferred to the corresponding except clause to handle the exception occurred
- If that particular exception is not specified in any except clause. Then by default except will execute without any exception name
- If neither of the above two cases is there,then the program stops abruptly.