Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Wrapper class Programs
Program 1: Write a program to create all wrapper class objects and print it.
Solution:
class wrap { public static void main(String args[]) { Integer a=10; Short b=20; Float f=12.0F; Double du=89.0D; Character c='s'; Boolean val=true; Byte d=3; System.out.println("All wrapper class objects values are:"); System.out.println("\nInteger class object value="+a); System.out.println("Short class object value="+b); System.out.println("Float class object value="+f); System.out.println("Double class object value="+du); System.out.println("Character class object value="+c); System.out.println("Byte class object value="+d); } }
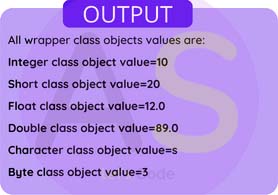
In the above program,we can observe that every primitive datatype ,has specific wrapper class object and how we can create and use that in program.
Program 2: Write a program to convert primitive datatype to wrapper class object using different methods
Solution:
import java.util.*; class wrap { public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the integer value"); int i=sc.nextInt(); Integer obj=new Integer(i); System.out.println("using constructor:"+obj); Integer obj1=Integer.valueOf(i); System.out.println("using integer class method:"+obj1); } }
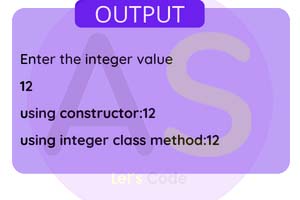
In the above program,we have created a primitive datatype variable having user defined value:
int i=sc.nextInt();
Then we have converted this datatype by first method as: Integer constructor and then by
using integer class methods named as static factory method
Program 3: Write a program to input an integer value and convert it into string using wrapper class methods and compare it with other string.
Solution:
import java.util.*; class wrap { public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the integer value"); int i=sc.nextInt(); Integer j=new Integer(i); String obj=j.toString(); // wrapper class methods String obj1="123"; if(obj.compareTo(obj1)==0) { System.out.println("Both are equal"); } else { System.out.println("Both are not equal"); } } }
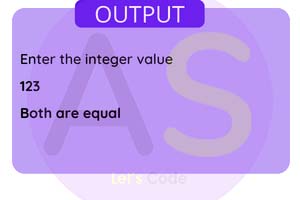
In the above program,we have created a primitive datatype variable having user defined value:
int i=sc.nextInt();
Then we have converted this datatype by constructor method and then compared it with string.
Program 4: Write a program to use compareTo() method of wrapper class object.
Solution:
import java.util.*; class wrap { public static void main(String args[]) { Integer a=23; Integer b=230; Integer c=89; Integer d=230; System.out.println("result on using function:"+(a.compareTo(c))); System.out.println("result on using function:"+(b.compareTo(c))); System.out.println("result on using function:"+(b.compareTo(d))); } }
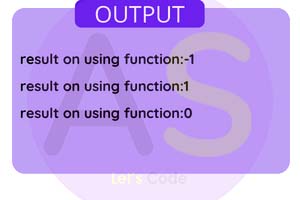