Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Values & datatype Programs
Program 1: Write a program to intialize and print all primitive datatypes.
Solution:
class primitive { boolean a; char b; byte c; short d; int e; long f; float g; double h; public void primitve()//default constructor to intialize all variables { a=false; b='\u0000'; c=0; d=0; e=0; f=0L; g=0.0f; h=0.0d; } public void display() { // add values to primitive datatype a=true; b='k'; c=7; d=2; e=9; f=12L; g=98.32f; h=21.70; System.out.println("Boolean datatype value="+a); System.out.println("Character datatype value="+b); System.out.println("Byte datatype value="+c); System.out.println("Short datatype value="+d); System.out.println("Integer datatype value="+e); System.out.println("long datatype value="+f); System.out.println("float datatype value="+g); System.out.println("double datatype value="+h); } public static void main(String args[]) { primitive ob=new primitive(); ob.display(); } }
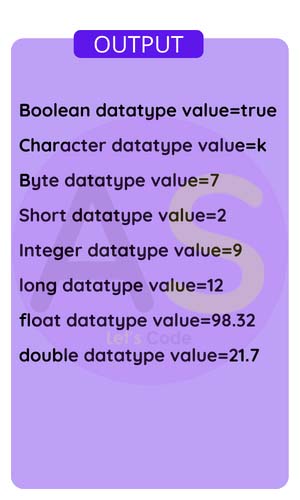
In the above program,we first intialized all the primitive datatypes by non-parameterized constructor.After that we added values in all datatypes which were intialized and displayed it.
Program 2: Write a program to show typecasting of variables from one primitive datatype to another primitive datatype
Solution:
class casting { public static void main(String args[]) { int intvar=100; //converting integer to double double doublevar=intvar; System.out.println("Converted int value to double value is="+doublevar); long longvar=1000L; int intvar2=(int)longvar; System.out.println("Converted float value to int value is="+intvar2); byte bytevar=7; short shortvar=(short)bytevar; System.out.println("Converted byte value to short value is="+shortvar); char ch='c'; int intvar3=ch; System.out.println("ASCII value of character to integer is="+intvar3); } }
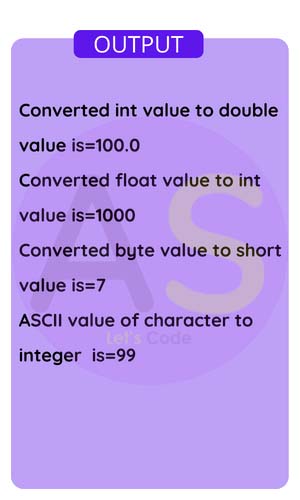
In the above program,we converted values from one primitive datatype to another primitive datatype and they are many more conversions.
Program 3: Write a program to convert numbers of minutes into years and days and print it.
Solution:
import java.util.*; class minutesdaysyears { public static void main(String args[]) { long minutes; Scanner sc=new Scanner(System.in); System.out.println("Enter the total minutes"); minutes=sc.nextLong(); long hours = minutes/60; long day = hours/24; long years = day/365; long remainingDays = day % 365; System.out.println(minutes + " minutes is " + years + " years and " + day + " days."); } }
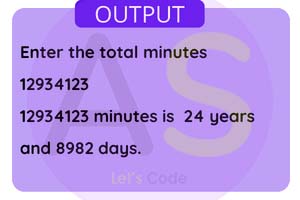
Program 4: Write a program to compare two words and print the largest word in upper case using non primitve datatype string
Solution:
import java.util.*; class stchk { public static void main(String args[]){ String s1,s2; Scanner sc=new Scanner(System.in); System.out.println("Enter the first String"); s1=sc.nextLine(); s1=s1.toUpperCase(); System.out.println("Enter the Second String"); s2=sc.nextLine(); s2=s2.toUpperCase(); if(!(s1.equalsIgnoreCase(s2))) { if((s1.length())>(s2.length())){ System.out.println("String with Highest length is="+s1); } else{ System.out.println("String with Highest length is="+s2); } } } }
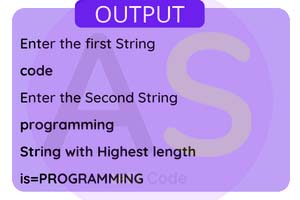