Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Basics of strings Programs
Program 1: Write a program to print the string using string literal
Solution:
class literal { public static void main(String args[]) { String a="aman"; System.out.println("Value of string a="+a); String b="aman"; System.out.println("Value of string b="+b); a.concat("singh"); System.out.println("Value of string a="+a);//String objects are immutable } }
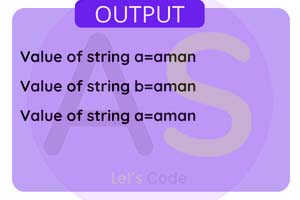
In the above program,we can observe that two strings are created using strings literals that
is
string a and string b.
String a="aman";
String b="aman";
These two strings have same content as "aman", so on executing this program, rather than creating
two different object as "aman",jvm will refer these two variables named as a and b to same object
"aman" which is stored in string constant pool.
Further if we try to change the value of a like a.concat("singh"),then it
wont change because string objects are immutable,you can only change the value of a by making a new
instance variable with same name as a and store the value in that variable
a=a.concat("singh");
It will create a new variable which will refer to new object as "amansingh".
Program 2: Write a program to print the string using new keyword
Solution:
class newkey { public static void main(String args[]) { String a=new String("khushi"); System.out.println("Value of string a="+a); String b=new String("khushi"); System.out.println("Value of string b="+b); a.concat("singh"); System.out.println("Value of string a="+a);//String objects are immutable a=a.concat("singh"); System.out.println("Value of new variable String a="+a);//String objects are immutable } }
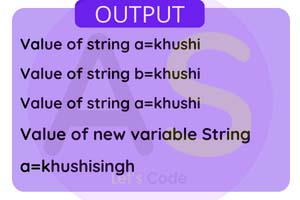
In the above program,we can observe that two strings are created using new keyword that
is
string a and string b.
String a=new String("khushi");
String b=new String("khushi");
These two strings have same content as "khushi", so on executing this program, rather than creating
two different object as "khushi",jvm will refer these two variables named as a and b to same object
"khushi" which is stored in main heap memory.
Further if we try to change the value of a like a.concat("singh"),then it
wont change because string objects are immutable,you can only change the value of a by making a new
instance variable with same name as a and store the value in that variable
a=a.concat("singh");
It will create a new variable which will refer to new object as "khushisingh".
Program 3: Write a program to print the employee name and salary using new keyword
Solution:
class employee { String name; String salary; public void display(String name,String salary) { System.out.println("Employee name is="+name); System.out.println("Employee salary is="+salary); } public static void main(String args[]) { employee em=new employee(); String str1=new String("abhay"); String str2=new String("100000"); em.display(str1,str2); } }
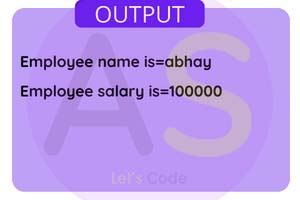