Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Static & Non Static Methods Programs
Static Methods Programs
Program 1: Write a program to check whether number is duck number or not (A Duck number is a number which has zeroes present in it. For example 3210)
Solution:
import java.util.*; public class duck { public static void calculate(int no) {int r; boolean check=false; while(no>0) { r=no%10; if(r==0) { check=true; } no=no/10; } if(check){ System.out.println("Duck Number"); } else{ System.out.println("Not Duck Number"); } } public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the number"); int n=sc.nextInt(); duck du=new duck(); duck.calculate(n); } }
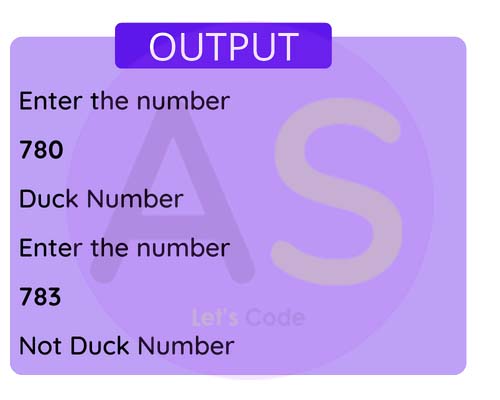
In the above program, when void main() starts executing,it will first
input from user,that is:
int n=sc.nextInt();
then we create a class object as:
duck du=new duck();
After that,to ivoke a static method,we dont require class object,it can be invoked
using the class name only.
duck.calculate(n);
Program 2: Write a program to convert a number into words
Solution:
import java.util.*; class word { public static void numwords(int n) { String s1=""; String words[]=new String[]{"Zero","One","two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"}; while (n!=0){ int num=n%10; String s=words[num]; s1=s+" "+s1; n=n/10; } System.out.println("\nnumber in words is:"); System.out.println(s1+"\n"); } public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the number"); int num=sc.nextInt(); word wo=new word(); word.numwords(num); } }
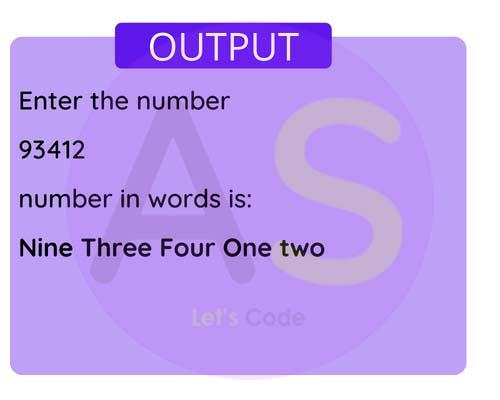
In the above program, when void main() starts executing,it will first
input from user,that is:
int num=sc.nextInt();
then we create a class object as:
word wo=new word();
After that,to invoke a static method,we dont require class object,it can be invoked
using the class name only.
word.numwords(num);
Non Static Methods Programs
Program 3: Write a program to print fibonacci series till n number of terms.
Solution:
import java.util.*; class fibonacci { public void fibo(int n) { System.out.println("Fibonacci series till "+n+"terms is:"); int firstno=0,secondno=1; for(int i=1;i<=n;i++) { System.out.print(firstno+","); int thirdno=firstno+secondno; firstno=secondno; secondno=thirdno; } } public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the number of terms"); int num=sc.nextInt(); fibonacci fb=new fibonacci(); fb.fibo(num); } }
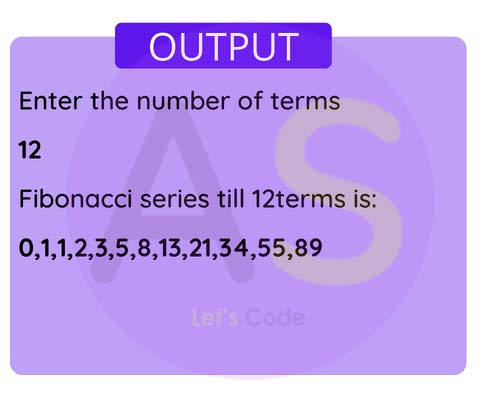
In the above program, when void main() starts executing,it will first
input from user,that is:
int num=sc.nextInt();
then we create a class object as:
fibonacci fb=new fibonacci();
After that,to invoke a non-static method,we require class object,it can be invoked
using the class object only.
fb.fibo(num);
Program 4: Write a program to find whether number is automorphic or not
Solution:
import java.util.*; class automorphic { public void calculate(int n) { boolean check=true; int sum=n*n; while(n>0) { if ((n%10)!=(sum%10)) { check=false; } n=n/10; sum=sum/10; } if(check==true) { System.out.println("Automorphic number"); } else{ System.out.println("Not Automorphic number"); } } public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the number:"); int number=sc.nextInt(); automorphic auto=new automorphic(); auto.calculate(number); } }
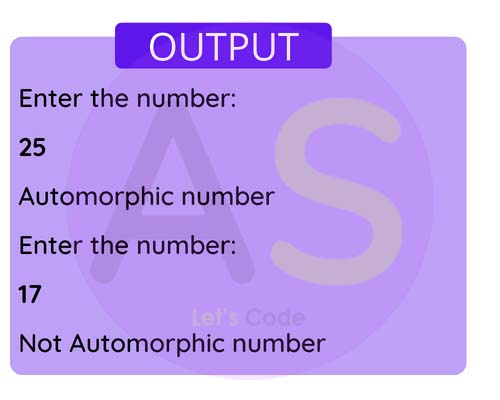
In the above program, when void main() starts executing,it will first
input from user,that is:
int number=sc.nextInt();
then we create a class object as:
automorphic auto=new automorphic();
After that,to invoke a non-static method,we require class object,it can be invoked
using the class object only.
auto.calculate(number);
Program 5: Write a program to find GCD of two numbers
Solution:
import java.util.*; class gcd { public void find(int n,int n1) { int no=1; for(int i=1;i<=n && i<=n1;i++) { if(n%i==0 && n1%i==0) { no=i; } } System.out.println("GCD of "+n+" and "+n1+" is="+no); } public static void main(String args[]) { Scanner sc=new Scanner(System.in); System.out.println("Enter the first number:"); int num=sc.nextInt(); System.out.println("Enter the Second number:"); int num1=sc.nextInt(); gcd ob=new gcd(); ob.find(num,num1); } }
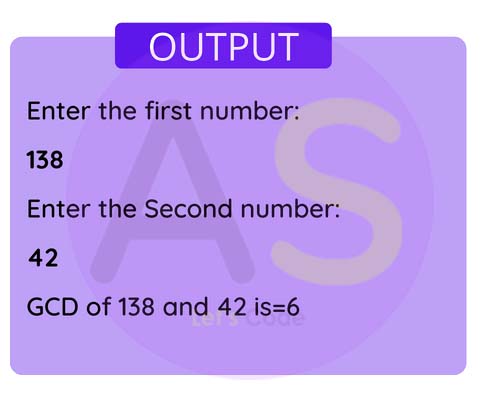