Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Operators in Java Programs
Program 1: Write a program to perform all arithmetic operations,using arithmetic Operators
Solution:
import java.util.*; class arithmetic { public static void main(String args[]) { int a,b,result; Scanner sc=new Scanner(System.in); System.out.println("Enter the value of a"); a=sc.nextInt(); System.out.println("Enter the value of b"); b=sc.nextInt(); result=a+b; System.out.println("Result after addition="+result); result=a-b; System.out.println("Result after subtraction="+result); result=a*b; System.out.println("Result after multiplication="+result); result=a/b; System.out.println("Result after division="+result); result=a%b; System.out.println("Result after modulus="+result); } }
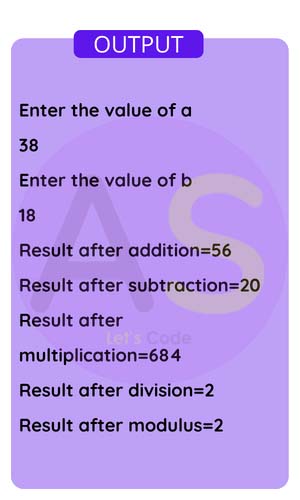
In the above program,we can observe that we used all arithmetic operators,to perform various arithmetic operations.
Program 2: Write a program to perform all relational operations,using relational operators
Solution:
import java.util.*; class relation { public static void main(String args[]) { int a,b; Scanner sc=new Scanner(System.in); System.out.println("Enter the value of a"); a=sc.nextInt(); System.out.println("Enter the value of b"); b=sc.nextInt(); System.out.println(a==b); System.out.println(a!=b); System.out.println(a>b); System.out.println(a< b); System.out.println(a>=b); System.out.println(a<=b); }
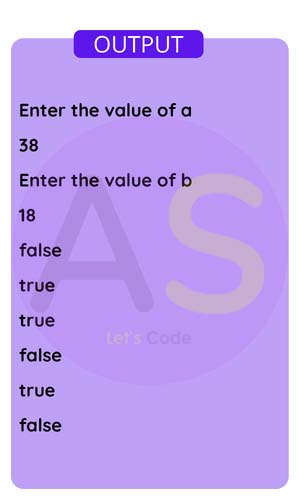
In the above program,we can observe that we used all relational operators, that resulting to true or false, that is boolean type value.
Program 3: Write a program to input marks and print grade according to inputed marks
Solution:
import java.util.*; class grade { public static void main(String args[]) { char grade; Scanner sc=new Scanner(System.in); System.out.println("Enter your score"); int score=sc.nextInt(); if ((score <=100) && (score >74)) { grade='A'; } else if ((score <=74) && (score >59)) { grade='B'; } else if ((score <=59) && (score >39)) { grade='C'; } else { grade='F'; } System.out.println("The grade for "+score+" is="+grade); } }
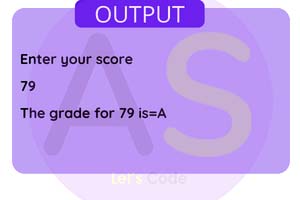
In the above program,we used a logical operator,that is logical AND,which will return true when both condition will be true.
Program 4: Write a program to check whether you are eligible to vote or not using conditional operator
Solution:
import java.util.*; class age { public static void main(String args[]) { int age; Scanner sc=new Scanner(System.in); System.out.println("Enter your age:"); age=sc.nextInt(); String str= (age>=18)?"You are elligible to vote" : "You are not elligible to vote"; System.out.println(str); } }
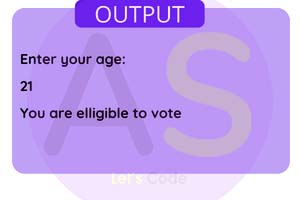
In the above program,we used ternary conditional operator which basically checks whether condition is true or not.This operator minmized the if else statements.
Program 5: Write a program to show post and prefix increment and decrement
Solution:
class incrementdecrement { public static void main(String args[]) { int a=10; //Post increment System.out.println("Post increment value for a++="+ a++); System.out.println("Post increment value for a then ="+ a); //Post decrement System.out.println("Post decrement value for a--="+ a--); System.out.println("Post decrement value for a then ="+ a); //Pre increment System.out.println("Post increment value for ++a="+ ++a); System.out.println("Post increment value for a then ="+ a); //Pre decrement System.out.println("Post decrement value for --a="+ --a); System.out.println("Post decrement value for a then ="+ a); } }
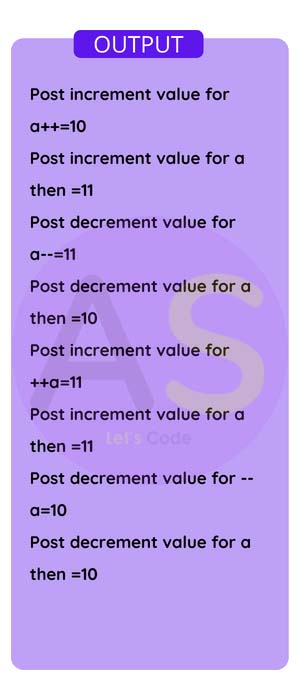