Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
OOPS Programs
Program 1: Write a program to perform addition,subtraction,multiplication using more than one object
Solution:
import java.util.*; class arthematic { public void add(int a,int b) { int sum=a+b; System.out.println("sum of a and b is="+sum); } public void subtract(int a,int b) { int diff=a-b; System.out.println("difference in a and b is="+diff); } public void multiply(int a,int b) { int prod=a*b; System.out.println("product of a and b is="+prod); } public static void main(String args[]) { Scanner sc=new Scanner(System.in); arthematic obj=new arthematic(); System.out.println("Enter value of a and b for first object"); int x=sc.nextInt(); int y=sc.nextInt(); System.out.println("*********first object**************"); obj.add(x,y); obj.subtract(x,y); obj.multiply(x,y); arthematic obj1=new arthematic(); System.out.println("Enter value of a and b for second object"); int i=sc.nextInt(); int j=sc.nextInt(); System.out.println("*********Second object**************"); obj1.add(x,y); obj1.subtract(x,y); obj1.multiply(x,y); } }
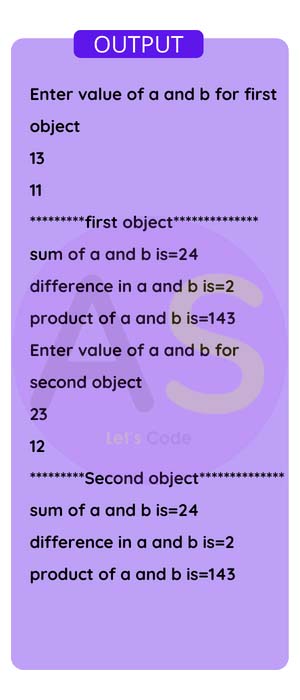
In the above program,we can observe that in a single class we can access or create multiple objects,which have different memory space and different values
Program 2: Write a program to show the working of inheritance.
Solution:
class baseclass { public void firstmethod() { System.out.println("Parent class is called"); } } class childclass extends baseclass { public void secondmethod() { System.out.println("child class is called"); } public static void main(String args[]) { childclass ob=new childclass(); ob.firstmethod();//super class method is called ob.secondmethod();//childclass local method is called } }
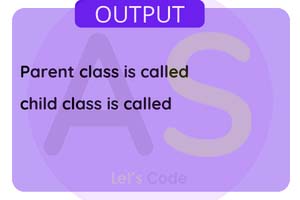
In the above program,two classes named as baseclass and childclass are created ,and
childclass inherits baseclass by keyword extends,this extends keyword is used while
inheriting
a
class.
And the method named as firstmethod is inherited from baseclass to childclass as shown in the
program above.
Program 3: Write a program to calculate area of rectangle and square using inheritance concept.
Solution:
import java.util.*; class area { int length,breadth; public void getValue(int l,int b) { length=l; breadth=b; } } class rectangle extends area { public void reccalculate() { System.out.println("Area of rectangle is="+(length* breadth)); } } class square extends area { public void squarecalculate() { System.out.println("Area of rectangle is="+(length*length)); } } import java.util.*; class display { public static void main(String args[]) { Scanner sc=new Scanner(System.in); rectangle obj=new rectangle(); square obj2=new square(); System.out.println("Enter value of length of breadth for rectangle"); int x=sc.nextInt(); int y=sc.nextInt(); obj.getValue(x,y); obj.reccalculate(); System.out.println("Enter value of length of breadth for square"); int i=sc.nextInt(); int j=sc.nextInt(); obj2.getValue(i,j); obj2.squarecalculate(); } }
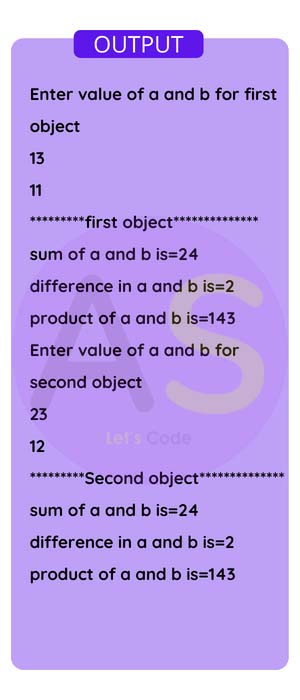
Program 4: Write a program to show polymorphism concept by details of student
Solution:
public class poly { public void studentdetails(String name) { System.out.println("Name of the student is="+name); } public void studentdetails(String name,int rollnumber) { System.out.println("Name of the student is="+name); System.out.println("Rollno of the student is="+rollnumber); } public void studentdetails(String name,int rollnumber,String joiningdate) { System.out.println("Name of the student is="+name); System.out.println("Rollno of the student is="+rollnumber); System.out.println("Rollno of the student is="+joiningdate); } public static void main(String args[]) { poly ob=new poly(); System.out.println("******Output******"); ob.studentdetails("Aditya"); ob.studentdetails("Aditya",5); ob.studentdetails("Aditya",5,"23/03/2009"); } }
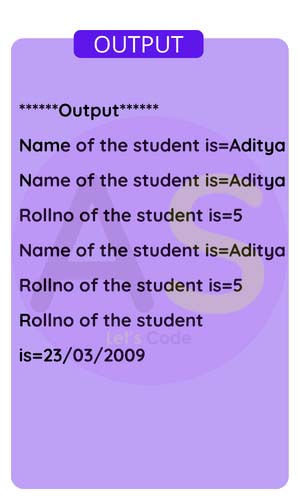
In the above program ,we can observe that there 3 methods with the same name as "studentdetails()" but with different set of arguments.This shows that single entity can have mutliple form as object ob can aim at different methods using the same name but different arguments.
Program 5: Write a program to show abstraction concept by creating abstract class as animals.
Solution:
abstract class animals{ public void eat(){ System.out.println("All Animals eat food"); } public abstract void sound(); } class dog extends animals{ @Override public void sound() { System.out.println("dog is barking"); } } class cat extends animals{ @Override public void sound() { System.out.println("cat is meowing"); } } class test { public static void main(String args[]) { dog d=new dog(); cat c=new cat(); d.sound(); d.eat(); c.sound(); c.eat(); } }
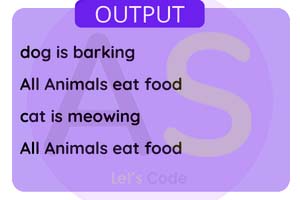