Code is copied!
Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Class as a composite Programs
Program 1: Write a program to input student details and print it.
Solution:
import java.util.*; class register { String name; int rollno; String jod; public void input() { Scanner sc=new Scanner(System.in); System.out.println("Enter the name of student:"); name=sc.next(); System.out.println("Enter the roll number of student:"); rollno=sc.nextInt(); System.out.println("Enter the Joining date of student:"); jod=sc.next(); } public void display() { System.out.println("The name of student is="+name); System.out.println("The name of student is="+rollno); System.out.println("Joining date of student is="+jod); } public static void main(String args[]) { register st=new register(); st.input(); st.display(); } }
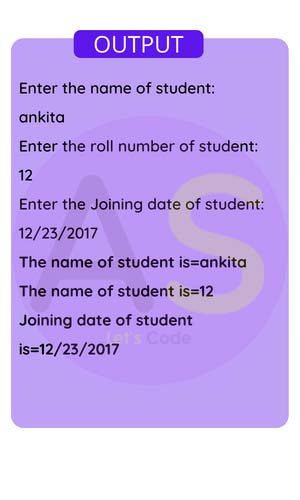
In the above program,we can observe that class register,is a composite datatypes as there are groups
of primitive datatype as
String name;
int rollno;
String jod;
And there are user-defined methods also inside the register class which shows that it is a
composite-datatype.
Program 2: write a program to calculate area and paramter of circle
Solution:
import java.util.*; class circle { int radius; double pi=3.14; public void input() { Scanner sc=new Scanner(System.in); System.out.println("Enter the radius of circle:"); radius=sc.nextInt(); } public void calculate() { double area,perimeter; perimeter=2*pi*radius; System.out.println("Perimeter of the Circle is ="+perimeter); area=pi*radius*radius; System.out.println("Area of the Circle ="+area); } public static void main(String args[]) { circle ci=new circle(); ci.input(); ci.calculate(); } }
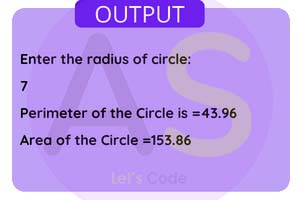
In the above program,we can observe that class circle,is a composite datatypes as there are groups
of primitive datatype as
int radius;
double pi=3.14;
And there are user-defined methods also inside the circle class which shows that it is a
composite-datatype.
Program 3: write a program to calculate ISBN number
Solution:
import java.util.*; class cal { String isbn; public void input() { System.out.println("enter 10 digit ISBN Number"); Scanner sc=new Scanner(System.in); isbn=sc.nextLine(); } public Boolean isValidISBN() { int sum = 0; for (int i = 0; i < 9; i++) { //converting string number to in int digit = isbn.charAt(i) - '0'; if (0 > digit || 9 < digit) return false; sum += (digit * (10 - i)); } char last = isbn.charAt(9); if (last != 'X' && (last < '0' || last > '9')) return false; sum += ((last == 'X') ? 10 : (last - '0')); return (sum % 11 == 0); } // Driver code public static void main(String[] args) { cal obj=new cal(); obj.input(); boolean check=obj.isValidISBN(); if (check) System.out.print("This a Valid ISBN"); else System.out.print("This in not a valid ISBN"); } }
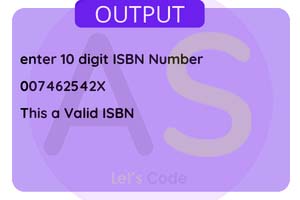
Program 4: write a program to swap the value of two numbers
Solution:
import java.util.*; class swap { int a,b; public void input() { Scanner sc=new Scanner(System.in); System.out.println("Enter the value of a:"); a=sc.nextInt(); System.out.println("Enter the value of b:"); b=sc.nextInt(); } public void calculate() { System.out.println("Value of a ="+a); System.out.println("Value of b="+b); int temp=0; temp=a; a=b; b=temp; System.out.println("Value of a after swapping="+a); System.out.println("Value of b after swapping="+b); } public static void main(String args[]) { swap sw=new swap(); sw.input(); sw.calculate(); } }
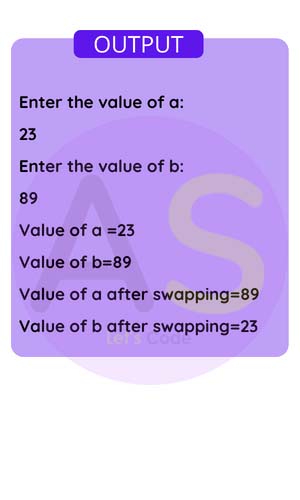
Program 5: write a program to print the reverse of the number.
Solution:
import java.util.*; class reversenumber{ int ans; public int reverse(int num){ if(num!=0) { int temp=num%10; ans=ans*10+temp; reverse(num/10); } return ans; } public static void main(String args[]){ reversenumber r=new reversenumber(); Scanner sc=new Scanner(System.in); System.out.println("enter the number:"); int n=sc.nextInt(); System.out.println("Reversed Number:"); int rev=r.reverse(n); System.out.print(rev); } }
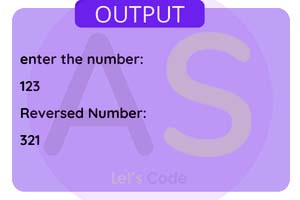