Class 10 ICSE - Java
Class 10th Java aims to empower students by enabling them to build their own applications introducing some effective tools to enable them to enhance their knowledge, broaden horizons, foster creativity, improve the quality of work and increase efficiency.
It also develops logical and analytical thinking so that they can easily solve interactive programs. Students learn fundamental concepts of computing using object oriented approach in one computer language with a clear idea of ethical issues involved in the field of computing
Class 10th java topics includes revision of class 9th, constructors, user-defined methods, objects and classes, library classes , etc.
Call by Value & Call by reference
Call by value Programs
Program 1: Write a program to calculate the square of a number
Solution:
class square { public void calculate(int x) { int sum=1; x=(int)Math.pow(x,2); System.out.println("Value inside this method is="+x); } public static void main(String args[]) { int number=10; System.out.println("Original value of number="+number); square obj=new square(); obj.calculate(number); System.out.println("value of number after squaring="+number); } }
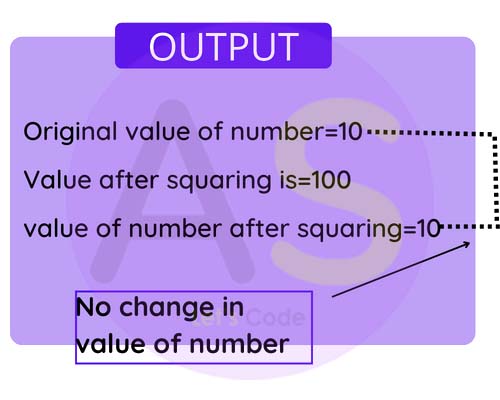
In the above program, when void main() starts executing,it will first
intialize number
as 10
int number=10;
After that when calculate() function is triggered,we can observe that
,the change in value
does not reflect in number.This is because only the value of formal
paramter changes.It wont affect
actual parameter value,as we are using copy of that actual value.
Program 2: Write a program to calculate sum of digits inputed by user.
Solution:
class sum { public void adddigits(int num) { int sum=1; while(num>0) { sum=sum+num%10; num=num/10; } System.out.println("Value inside method="+num); System.out.println("Sum of the digits is="+sum); } public static void main(String args[]) { int x=9234; System.out.println("Original value="+x); sum obj=new sum(); obj.adddigits(x); System.out.println("Value after calling adddigits func="+x); } }
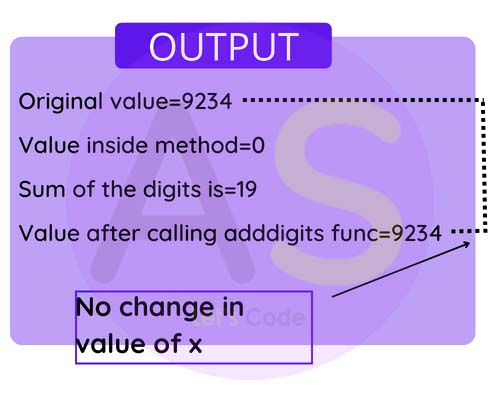
In the above program, when void main() starts executing,it will first
intialize x
as 9234
int x=9234;
After that when adddigits() function is triggered,we can observe that the
value of x becomes 0
, and the change in value
does not reflect in x.This is because only the value of formal
paramter changes.It wont affect
actual parameter value,as we are using copy of that actual value.
Program 3: Write a program to calculate reverse of a number
Solution:
class reverse { public void rev(int number) { int rev=0; while(number>0) { rev=(rev*10)+number%10; number=number/10; } number=rev; System.out.println("Reverse of a number is="+number); } public static void main(String args[]) { int num=1234; System.out.println("Original value="+num); reverse obj=new reverse(); obj.rev(num); System.out.println("Value after calling rev function="+num); } }
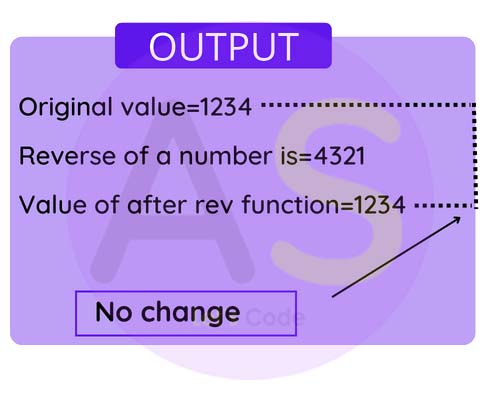
In the above program, when void main() starts executing,it will first
intialize num
as 1234
int x=1234;
After that when adddigits() function is triggered,we can observe that the
value of num changes to 4321
, and the change in value
does not reflect in num.This is because only the value of formal
paramter changes.It wont affect
actual parameter value,as we are using copy of that actual value.
Call by reference Programs
Program 4: Write a program to print the sum of factorial of a number
Solution:
public class factorial { int num; public void fact(factorial ob) { int sum=0; for(int i=1;i<=ob.num;i++) { sum=sum+i; } ob.num=sum; System.out.println("sum of factorial is="+ob.num); } public static void main(String args[]) { factorial obj=new factorial(); obj.num=10; System.out.println("Original value="+obj.num); obj.fact(obj); System.out.println("Value after called function="+obj.num); } }
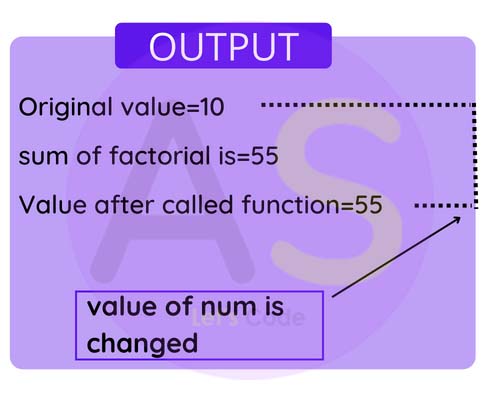
In the above program, when void main() starts executing,it will first
create a reference variable as obj.And also an
object say D will be created,and obj will point to that object
After that when fact() function,is triggered,we observe that value of num
changes from 10 to 55.This is because when
fact func. is triggered,it will create a new reference variable,which also point to object D.
So any changes made in the value of formal parameter get reflected in the value of actual parameter
as both are pointing to same object named as D.
Program 5: Write a program to calculate the product of digits in number
Solution:
class multiple { int x; public void multiplydigits(multiple obj) { int sum=1; while(obj.x>0) { sum=sum*(obj.x%10); obj.x=obj.x/10; } obj.x=sum; System.out.println("product of the digits is="+obj.x); } public static void main(String args[]) { multiple mu=new multiple(); mu.x=3781; System.out.println("Original value="+mu.x); mu.multiplydigits(mu); System.out.println("Value after calling adddigits func="+mu.x); } }
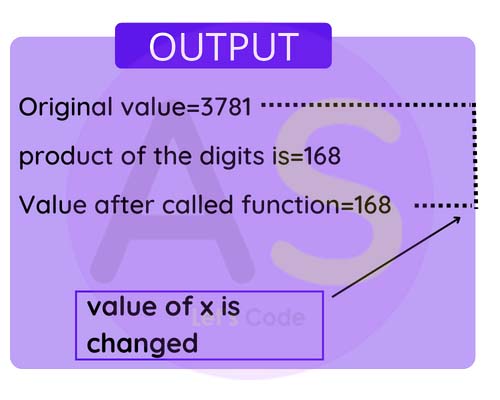