Code is copied!
CLASS 10 ICSE Computer Science - Java Question Paper 2019
Maximum Marks:100
Time allowed: Two hours
Answers to this Paper must be written on the paper provided separately.
You will not be allowed to write during the first 15 minutes.
This time is to be spent in reading the question paper.
The time given at the head of this Paper is the time allowed for writing the answers.
This Paper is divided into two Sections.
Attempt all questions from Section A and any four questions from Section B.
Answers to this Paper must be written on the paper provided separately.
The intended marks for questions or parts of questions are given in brackets[] .
SECTION A
(Attempt all questions from this Section.)
Question 1
(a)
Name any two basic principles of Object-oriented Programming.
Solution
1. Encapsulation
2. Inheritance
2. Inheritance
(b)
Write a difference between unary and binary operator.
Solution
unary operators operate on a single operand whereas binary operators operate on two operands.
(c)
Name the keyword which:
1. indicates that a method has no return type.
2. makes the variable as a class variable
Solution
1. void
2. static
2. static
(d)
Write the memory capacity (storage size) of short and float data type in bytes.
Solution
short — 2 bytes
float — 4 bytes
float — 4 bytes
(e)
Identify and name the following tokens:
1. public
2. 'a'
3. ==
4. { }
Solution
1. Keyword
2. Literal
3. Operator
4. Separator
2. Literal
3. Operator
4. Separator
Question 2
(a) Differentiate between if else if and switch-case statements
Solution
if else if | switch-case |
---|---|
The if and else blocks are executed depending on the condition in the if statement | The switch statement has multiple cases, and the code block corresponding to that case is executed |
Used for integer, character, pointer, floating-point type, or Boolean type. | Used for character expressions and integers. |
(b)
Give the output of the following code:
String P = "20", Q ="19";
int a = Integer.parseInt(P);
int b = Integer.valueOf(Q);
System.out.println(a+""+b);
Solution
Output of the above code is:
2019
(c) What are the various types of errors in Java?
Solution
There are 3 types of errors in Java:
1. Syntax Error
2. Runtime Error
3. Logical Error
(d)
State the data type and value of res after the following is executed:
char ch = '9';
res= Character.isDigit(ch);
Solution
Data type of res is boolean . Its value is true.
(e) What is the difference between the linear search and the binary search technique?
Solution
Linear Search | Binary Search |
---|---|
Linear search works on sorted and unsorted arrays. | Binary search works on only sorted arrays. |
In Linear search, each element of the array is checked with the target value until the element is found. | In Binary search, array is further divided into 2 halves and the target element is searched either in the first half or in the second half. |
Question 3
(a)
Write a Java expression for the following:
|x2 + 2xy|
Solution
Math.abs(x * x + 2 * x * y)
(b)
Write the return data type of the following functions:
1. startsWith()
2. random()
Solution
1. boolean
2. double
(c)
If the value of basic=1500, what will be the value of tax after the following statement is executed?
tax = basic > 1200 ? 200 :100;
Solution
Value of tax will be 200.
(d)
Give the output of following code and mention how many times the loop will execute?
int i;
for( i=5; i>=1; i--)
{
if(i%2 == 1)
continue;
System.out.print(i+" ");
}
Solution
Output of the above code is:
4 2
Loop executes 5 times.
(e) State a difference between call by value and call by reference.
Solution
In call by value, actual parameters are copied to formal parameters. Any changes to formal parameters are not reflected onto the actual parameters.
In call by reference, formal parameters refer to actual parameters. The changes to formal parameters are reflected onto the actual parameters.
(f)
Give the output of the following:
Math.sqrt(Math.max(9,16))
Solution
The output is 4.0.
(g)
Write the output for the following:
String s1 = "phoenix"; String s2 ="island";
System.out.println(s1.substring(0).concat(s2.substring(2)));
System.out.println(s2.toUpperCase());
String s1 = "phoenix"; String s2 ="island";
System.out.println(s1.substring(0).concat(s2.substring(2)));
System.out.println(s2.toUpperCase());
Solution
The output of above code is:
phoenixland
ISLAND
(h)
Evaluate the following expression if the value of x=2, y=3 and z=1.
v=x + --z + y++ + y
Solution
v = x + --z + y++ + y
⇒ v = 2 + 0 + 3 + 4
⇒ v = 9
(i)
String x[] = {"Artificial intelligence", "IOT", "Machine learning", "Big data"};
Give the output of the following statements:
1. System.out.println(x[3]);
2. System.out.println(x.length);
Solution
1. Big data
2. 4
(j) What is meant by a package? Give an example.
Solution
A package is a named collection of Java classes that are grouped on the basis of their functionality. For example, java.util.
SECTION B
(Attempt any four questions from this Section)
Question 4
Design a class name ShowRoom with the following description :
Instance variables/ Data members :
String name – To store the name of the customer
long mobno – To store the mobile number of the customer
double cost – To store the cost of the items purchased
double dis – To store the discount amount
double amount – To store the amount to be paid after discount
Member methods: –
ShowRoom() – default constructor to initialize data members
void input() – To input customer name, mobile number, cost
void calculate() – To calculate discount on the cost of purchased items, based on following criteria
void display() – To display customer name, mobile number, amount to be paid after discount
Write a main method to create an object of the class and call the above member methods
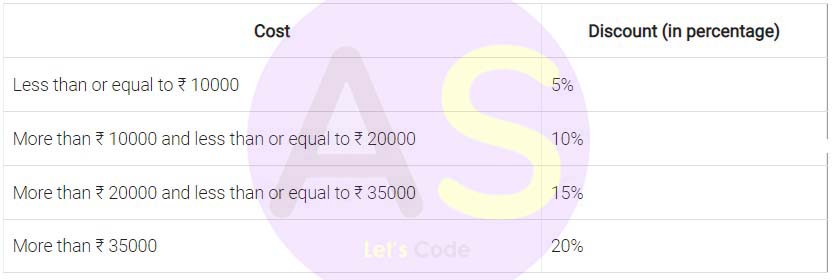
Solution:
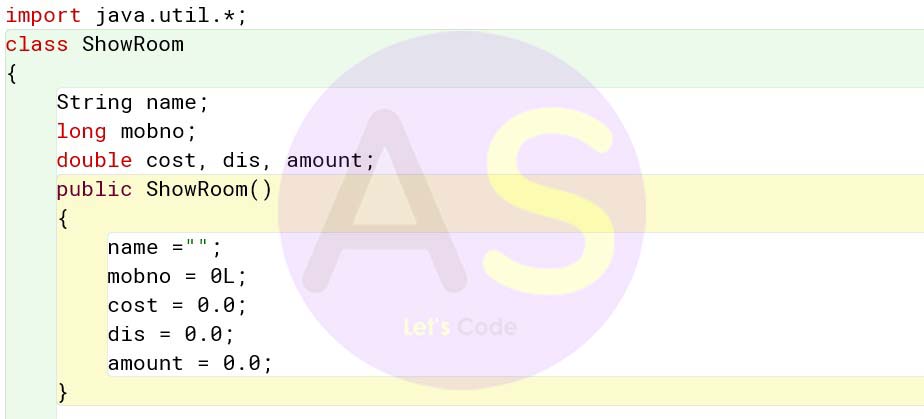
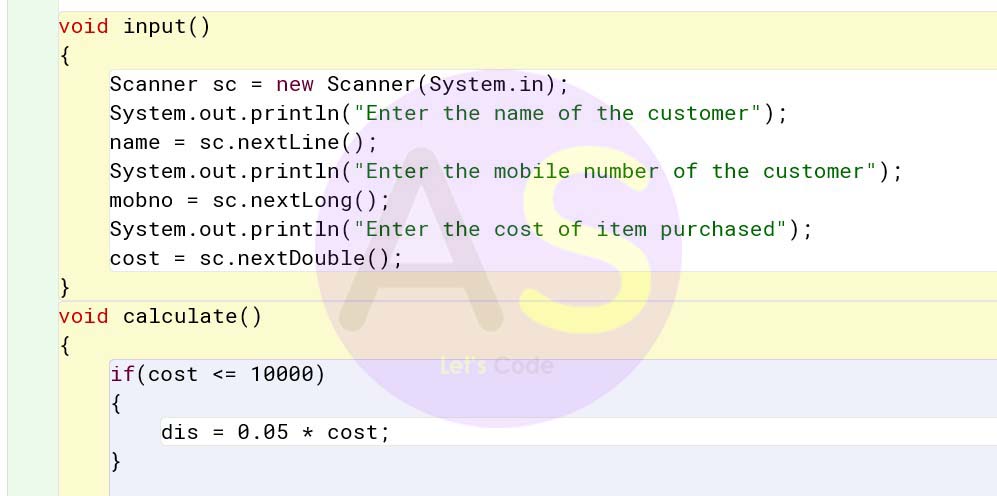
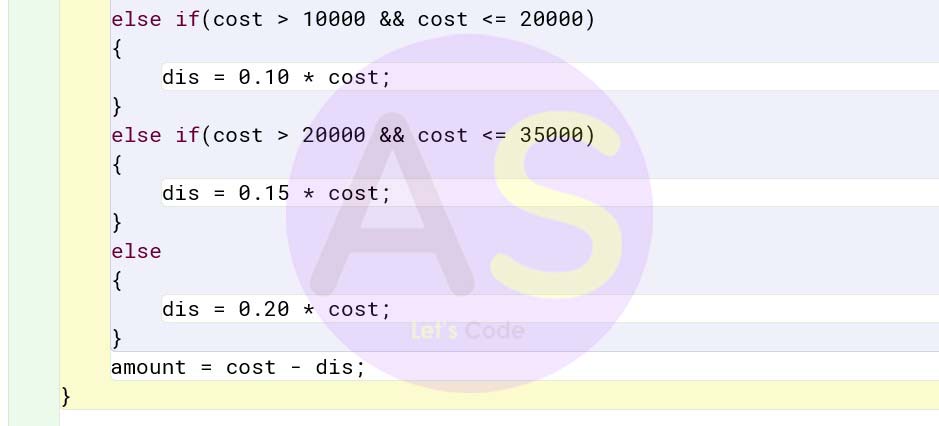
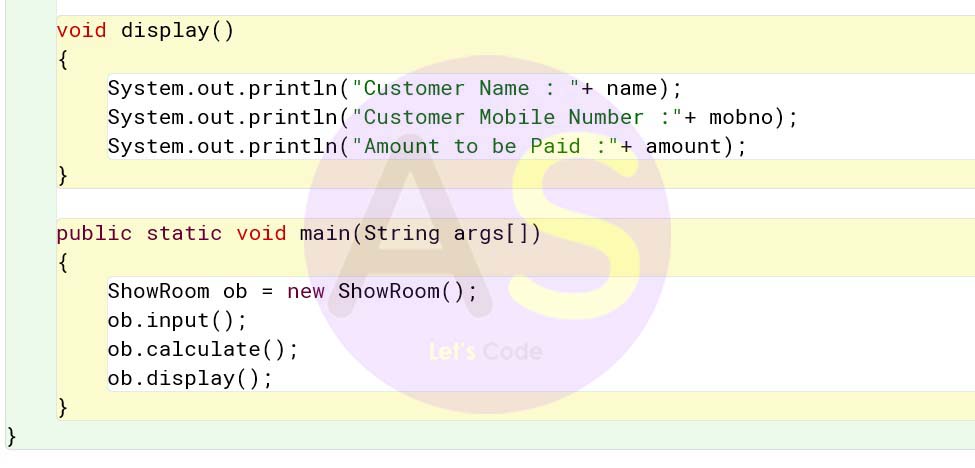
import java.util.*; class ShowRoom { String name; long mobno; double cost, dis, amount; public ShowRoom() { name =""; mobno = 0L; cost = 0.0; dis = 0.0; amount = 0.0; } void input() { Scanner sc = new Scanner(System.in); System.out.println("Enter the name of the customer"); name = sc.nextLine(); System.out.println("Enter the mobile number of the customer"); mobno = sc.nextLong(); System.out.println("Enter the cost of item purchased"); cost = sc.nextDouble(); } void calculate() { if(cost <= 10000) { dis = 0.05 * cost; } else if(cost > 10000 && cost <= 20000) { dis = 0.10 * cost; } else if(cost > 20000 && cost <= 35000) { dis = 0.15 * cost; } else { dis = 0.20 * cost; } amount = cost - dis; } void display() { System.out.println("Customer Name : "+ name); System.out.println("Customer Mobile Number :"+ mobno); System.out.println("Amount to be Paid :"+ amount); } public static void main(String args[]) { ShowRoom ob = new ShowRoom(); ob.input(); ob.calculate(); ob.display(); } }
Question 5
Using the switch-case statement, write a menu driven program to do the following :
(a) To generate and print Letters from A to Z and their Unicode Letters Unicode
Letters Unicode
A 65
B 66
.
.
.
Z 90
(b) Display the following pattern using iteration (looping) statement:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Solution:
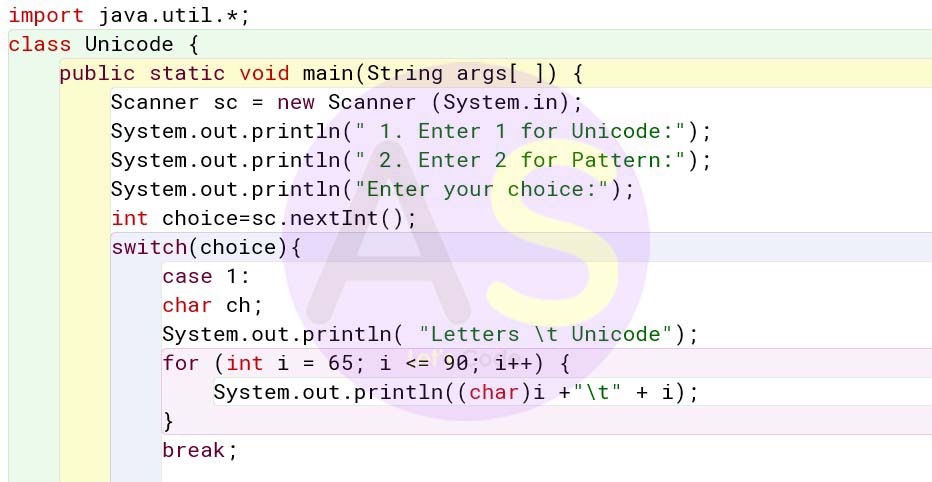
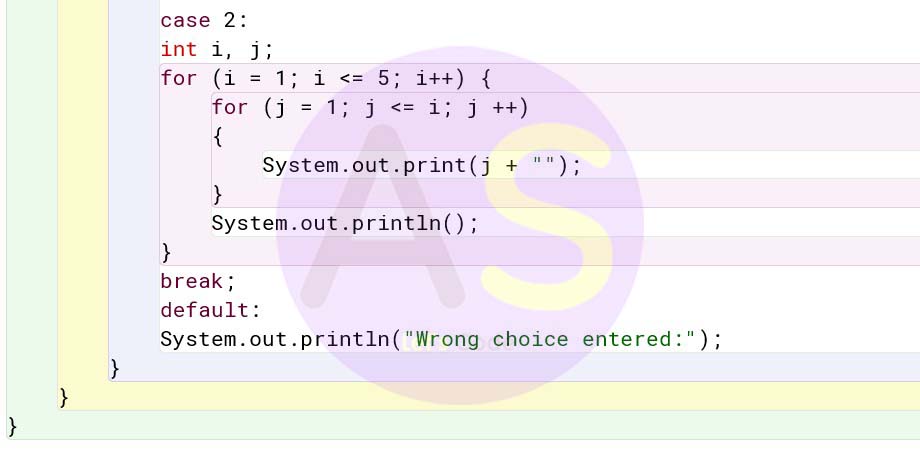
import java.util.*; class Unicode { public static void main(String args[ ]) { Scanner sc = new Scanner (System.in); System.out.println(" 1. Enter 1 for Unicode:"); System.out.println(" 2. Enter 2 for Pattern:"); System.out.println("Enter your choice:"); int choice=sc.nextInt(); switch(choice){ case 1: char ch; System.out.println( "Letters \t Unicode"); for (int i = 65; i <= 90; i++) { System.out.println((char)i +"\t" + i); } break; case 2: int i, j; for (i = 1; i <= 5; i++) { for (j = 1; j <= i; j ++) { System.out.print(j + ""); } System.out.println(); } break; default: System.out.println("Wrong choice entered:"); } } }
Question 6
Write a program to input 15 integer elements in an array and sort them in ascending order using the bubble sort technique.
Solution:
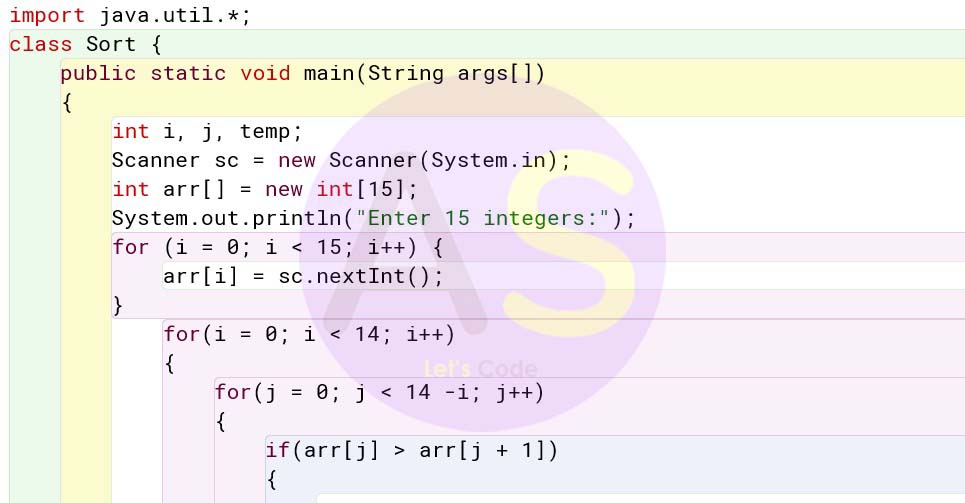
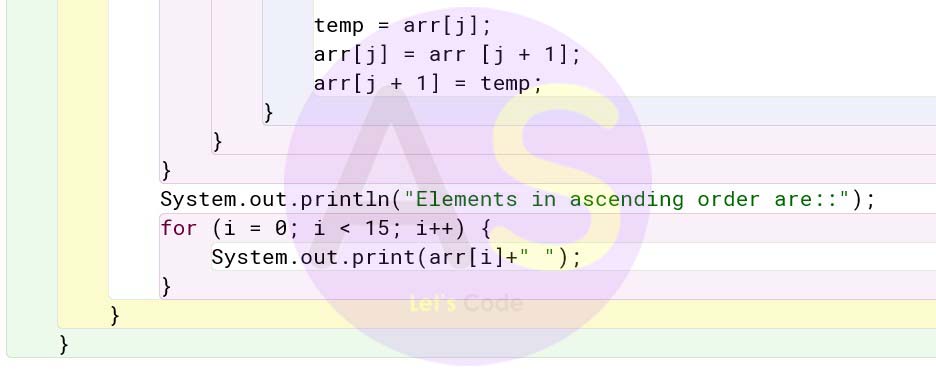
import java.util.*; class Sort { public static void main(String args[]) { int i, j, temp; Scanner sc = new Scanner(System.in); int arr[] = new int[15]; System.out.println("Enter 15 integers:"); for (i = 0; i < 15; i++) { arr[i] = sc.nextInt(); } for(i = 0; i < 14; i++) { for(j = 0; j < 14 -i; j++) { if(arr[j] > arr[j + 1]) { temp = arr[j]; arr[j] = arr [j + 1]; arr[j + 1] = temp; } } } System.out.println("Elements in ascending order are::"); for (i = 0; i < 15; i++) { System.out.print(arr[i]+" "); } } }
Question 7
Design a class to overload a function series() as follows:
(a) void series (int x, int n) – To display the sum of the series given below:
x1 + x2 + x3 + ……………. xn terms
(b) void series (int p) – To display the following series:
0, 7, 26, 63 p terms.
(c) void series () – To display the sum of the series given below:
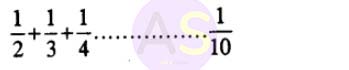
Solution:
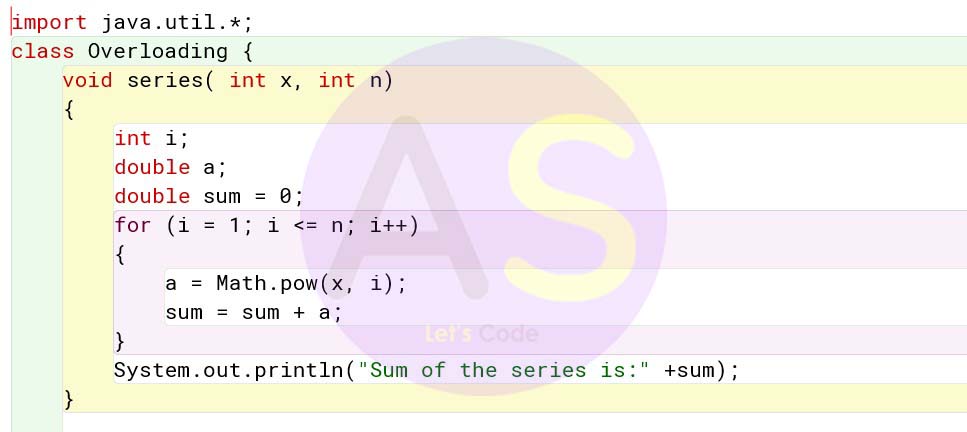
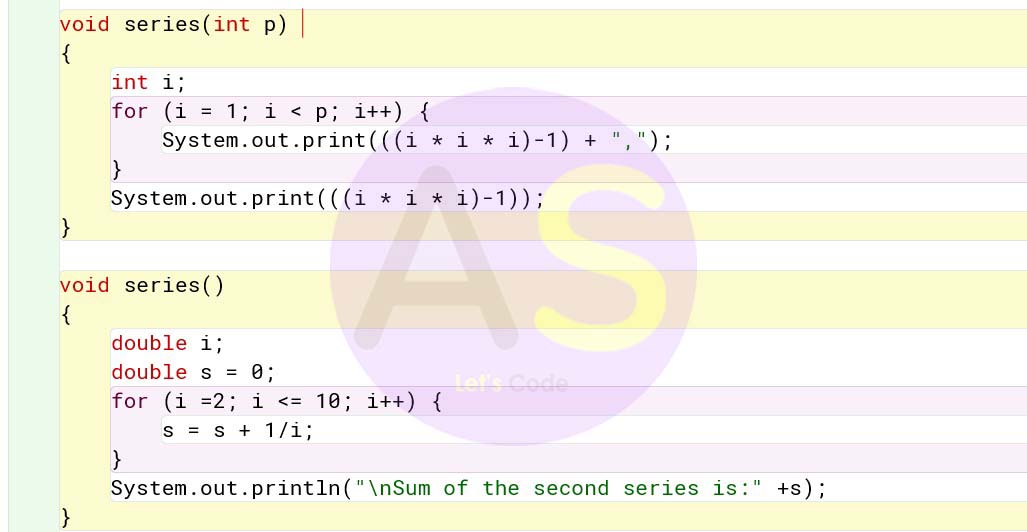
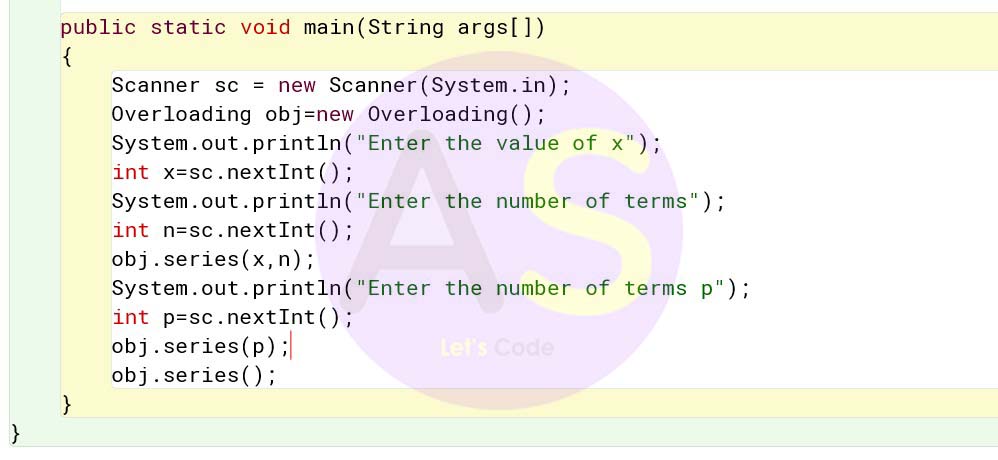
import java.util.*; class Overloading { void series( int x, int n) { int i; double a; double sum = 0; for (i = 1; i <= n; i++) { a = Math.pow(x, i); sum = sum + a; } System.out.println("Sum of the series is:" +sum); } void series(int p) { int i; for (i = 1; i < p; i++) { System.out.print(((i * i * i)-1) + ","); } System.out.print(((i * i * i)-1)); } void series() { double i; double s = 0; for (i =2; i <= 10; i++) { s = s + 1/i; } System.out.println("\nSum of the second series is:" +s); } public static void main(String args[]) { Scanner sc = new Scanner(System.in); Overloading obj=new Overloading(); System.out.println("Enter the value of x"); int x=sc.nextInt(); System.out.println("Enter the number of terms"); int n=sc.nextInt(); obj.series(x,n); System.out.println("Enter the number of terms p"); int p=sc.nextInt(); obj.series(p); obj.series(); } }
Question 8
Write a program to input a sentence and convert it into uppercase and count and display the total number of words starting with a letter ‘A’.
Example:
Sample Input: ADVANCEMENT AND APPLICATION OF INFORMATION TECHNOLOGY ARE EVER CHANGING.
Sample Output : Total number of words starting with letter A = 4.
Solution:
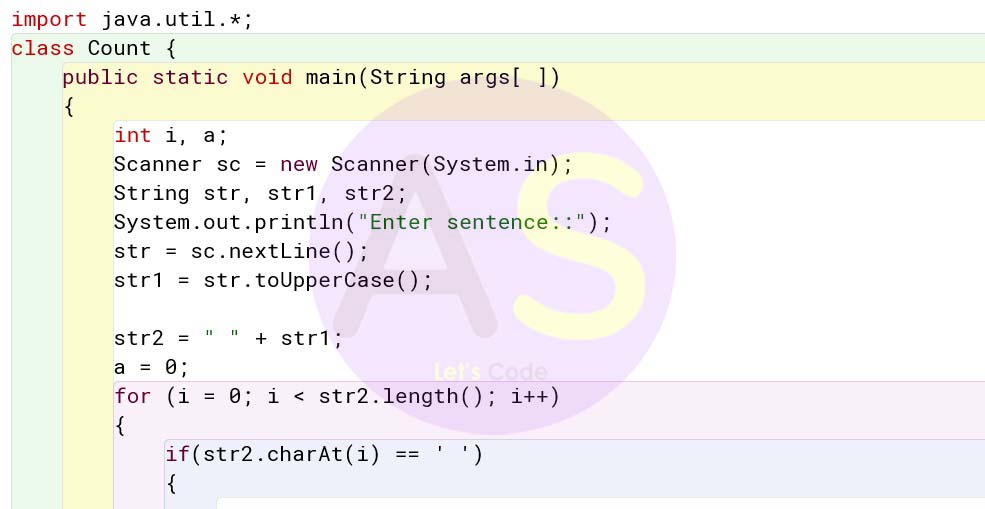
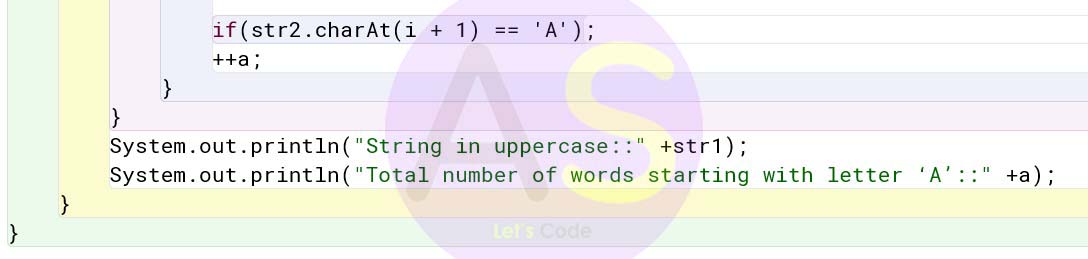
import java.util.*; class Count { public static void main(String args[ ]) { int i, a; Scanner sc = new Scanner(System.in); String str, str1, str2; System.out.println("Enter sentence::"); str = sc.nextLine(); str1 = str.toUpperCase(); str2 = " " + str1; a = 0; for (i = 0; i < str2.length(); i++) { if(str2.charAt(i) == ' ') { if(str2.charAt(i + 1) == 'A'); ++a; } } System.out.println("String in uppercase::" +str1); System.out.println("Total number of words starting with letter ‘A’::" +a); } }
Question 9
A tech number has even number of digits. If the number is split in two equal halves, then the square of sum of these halves is equal to the number itself. Write a program to generate and print all four digit tech numbers.
Example :
Consider the number 3025
Square of sum of the halves of 3025 = (30+25)2
= (55)2
= 3025 is a tech number.
Solution:
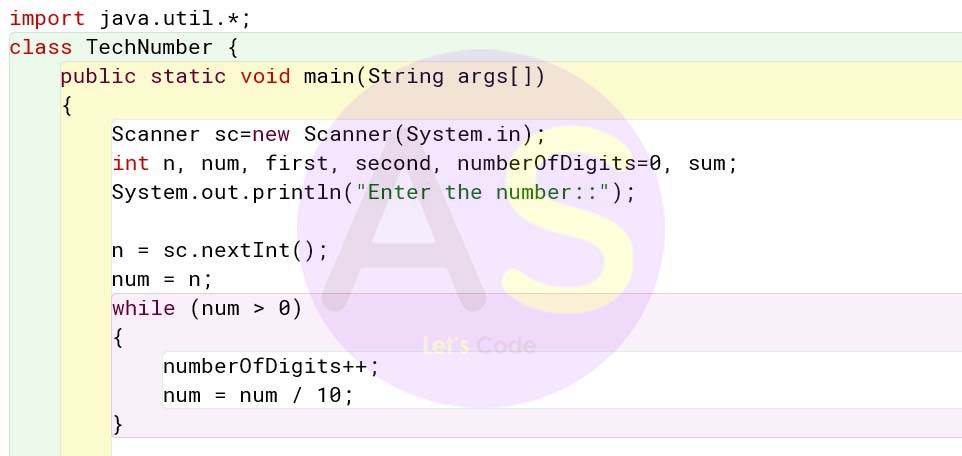
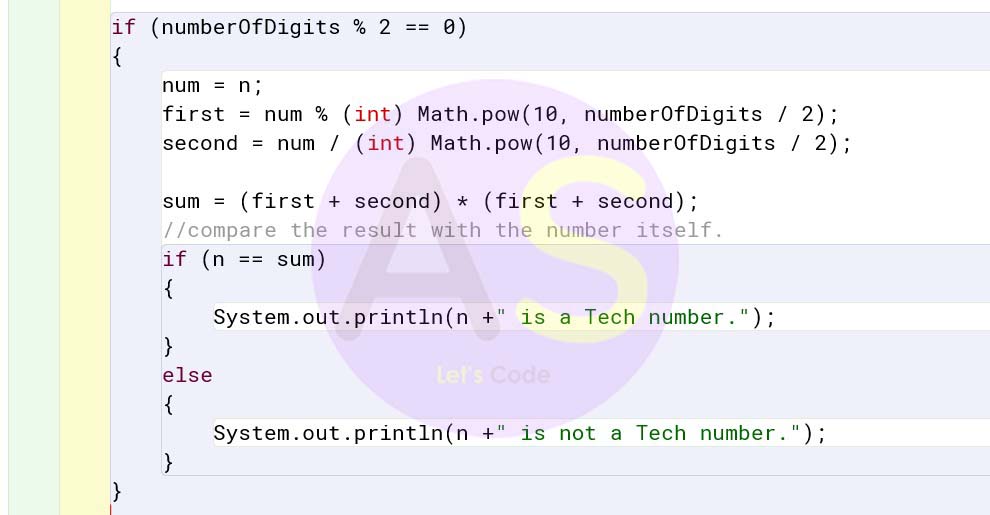
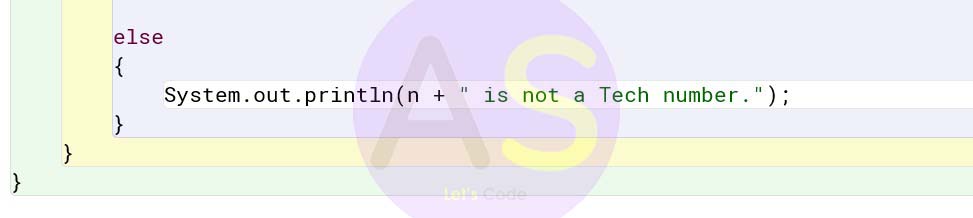
import java.util.*; class TechNumber { public static void main(String args[]) { Scanner sc=new Scanner(System.in); int n, num, first, second, numberOfDigits=0, sum; System.out.println("Enter the number::"); n = sc.nextInt(); num = n; while (num > 0) { numberOfDigits++; num = num / 10; } if (numberOfDigits % 2 == 0) { num = n; first = num % (int) Math.pow(10, numberOfDigits / 2); second = num / (int) Math.pow(10, numberOfDigits / 2); sum = (first + second) * (first + second); //compare the result with the number itself. if (n == sum) { System.out.println(n +" is a Tech number."); } else { System.out.println(n +" is not a Tech number."); } } else { System.out.println(n + " is not a Tech number."); } } }