Code is copied!
CLASS 10 ICSE Computer Science - Java Question Paper 2018
Maximum Marks:100
Time allowed: Two hours
Answers to this Paper must be written on the paper provided separately.
You will not be allowed to write during the first 15 minutes.
This time is to be spent in reading the question paper.
The time given at the head of this Paper is the time allowed for writing the answers.
This Paper is divided into two Sections.
Attempt all questions from Section A and any four questions from Section B.
Answers to this Paper must be written on the paper provided separately.
The intended marks for questions or parts of questions are given in brackets[] .
SECTION A
(Attempt all questions from this Section.)
Question 1
(a)
Define abstraction.
Solution
It refers to the act of representing essential features without including the background details or explanation.
(b)
Differentiate between searching and sorting.
Solution
Searching | Sorting |
---|---|
Searching is a technique to search a particular value in an array. | Sorting is a technique which is used to arrange the elements of an array in ascending or descending order. |
Linear search and Binary search are examples of searching techniques. | Bubble sort and Selection sort are examples of sorting techniques. |
(c)
Write a difference between the functions isUpperCase() and toUpperCase().
Solution
isUpperCase() | toUpperCase() |
---|---|
It checks if a given character is in uppercase or not. | It converts a given character to uppercase. |
Its return type is boolean. | Its return type is char. |
(d)
How are private members of a class different from public members?
Solution
private members of a class can only be accessed by other member methods of the same class
public members of a class can be accessed by methods of other classes as well.
public members of a class can be accessed by methods of other classes as well.
(e)
Classify the following as primitive or non-primitive datatypes:
1. char
2. arrays
3. int
4. classes
Solution
1. Primitive
2. Non-Primitive
3. Primitive
4. Non-Primitive
2. Non-Primitive
3. Primitive
4. Non-Primitive
Question 2
(a)
(i) int res = 'A';
What is the value of res?
(ii) Name the package that contains wrapper classes.
Solution
(i) Value of res is 65
(ii) java.lang
(b) State the difference between while and do while loop.
Solution
while | do-while |
---|---|
It is an entry-controlled loop. | It is an exit-controlled loop. |
In this condition is checked first then statements are executed. | In this statements are executed first then condition is checked. |
(c)
System.out.print("BEST ");
System.out.println("OF LUCK");
Choose the correct option for the output of the above statements
1. BEST OF LUCK
2. BEST
OF LUCK
Solution
Option 1 — BEST OF LUCK
(d) Write the prototype of a function check which takes an integer as an argument and returns a character.
Solution
char check(int a)
(e)
Write the return data type of the following function.
1. endsWith()
2. log()
Solution
1. boolean
2. double
Question 3
(a)
Write a Java expression for the following:
√(3x + x2)/(a + b)
Solution
Math.sqrt(3 * x + x * x) / (a + b)
(b)
What is the value of y after evaluating the expression given below?
y+= ++y + y-- + --y; when int y=8
Solution
y+= ++y + y-- + --y
⇒ y = y + (++y + y-- + --y)
⇒ y = 8 + (9 + 9 + 7)
⇒ y = 8 + 25
⇒ y = 33
(c)
Give the output of the following:
1. Math.floor (-4.7)
2. Math.ceil(3.4) + Math.pow(2, 3)
Solution
1. -5.0
2. 12.0
(d) Write two characteristics of a constructor.
Solution
Two characteristics of a constructor are:
1. A constructor has the same name as its class.
2. A constructor does not have a return type.
(e)
Write the output for the following:
System.out.println("Incredible"+"\n"+"world");
System.out.println("Incredible"+"\n"+"world");
Solution
Output of the above code is:
Incredible
world
(f)
Convert the following if else if construct into switch case
if( var==1)
System.out.println("good");
else if(var==2)
System.out.println("better");
else if(var==3)
System.out.println("best");
else
System.out.println("invalid");
Solution
switch (var) {
case 1:
System.out.println("good");
break;
case 2:
System.out.println("better");
break;
case 3:
System.out.println("best");
break;
default:
System.out.println("invalid");
}
(g)
Give the output of the following string functions:
1. "ACHIEVEMENT".replace('E', 'A')
2. "DEDICATE".compareTo("DEVOTE")
Solution
1. ACHIAVAMANT
2. -18
(h)
Consider the following String array and give the output
String arr[]= {"DELHI", "CHENNAI", "MUMBAI", "LUCKNOW", "JAIPUR"};
System.out.println(arr[0].length() > arr[3].length());
System.out.print(arr[4].substring(0,3));
String arr[]= {"DELHI", "CHENNAI", "MUMBAI", "LUCKNOW", "JAIPUR"};
System.out.println(arr[0].length() > arr[3].length());
System.out.print(arr[4].substring(0,3));
Solution
Output of the above code is:
false
JAI
(i)
Rewrite the following using ternary operator:
if (bill > 10000 )
discount = bill * 10.0/100;
else
discount = bill * 5.0/100;
Solution
discount = bill > 10000 ? bill * 10.0/100 : bill * 5.0/100;
(j)
Give the output of the following program segment and also mention how many times the loop is executed:
int i;
for ( i = 5 ; i > 10; i ++ )
System.out.println( i );
System.out.println( i * 4 );
Solution
Output of the above code is:
20
Loop executes 0 times.
SECTION B
(Attempt any four questions from this Section)
Question 4
Design a class Railway Ticket with following description :
Instance variables/s data members :
String name : To store the name of the customer
String coach : To store the type of coach customer wants to travel
long mobno : To store customer’s mobile number
int amt : To store basic amount of ticket
int totalamt : To store the amount to be paid after updating the original amount
Member methods
void accept ( ) — To take input for name, coach, mobile number and amount
void update ( )— To update the amount as per the coach selected
void display( ) — To display all details of a customer such as name, coach, total amount and mobile number.
Write a main method to create an object of the class and call the above member methods.
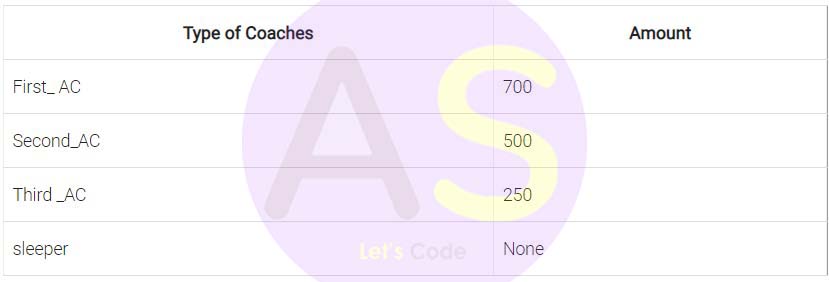
Solution:
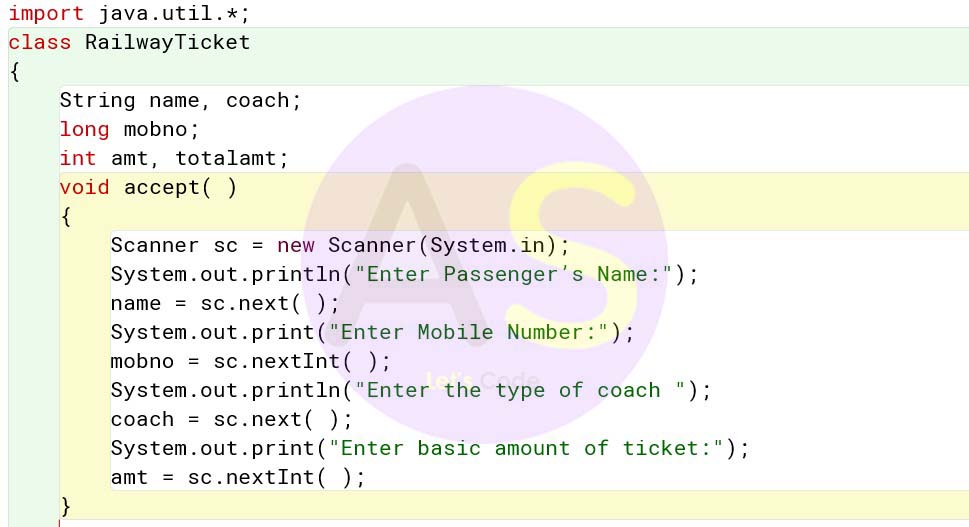
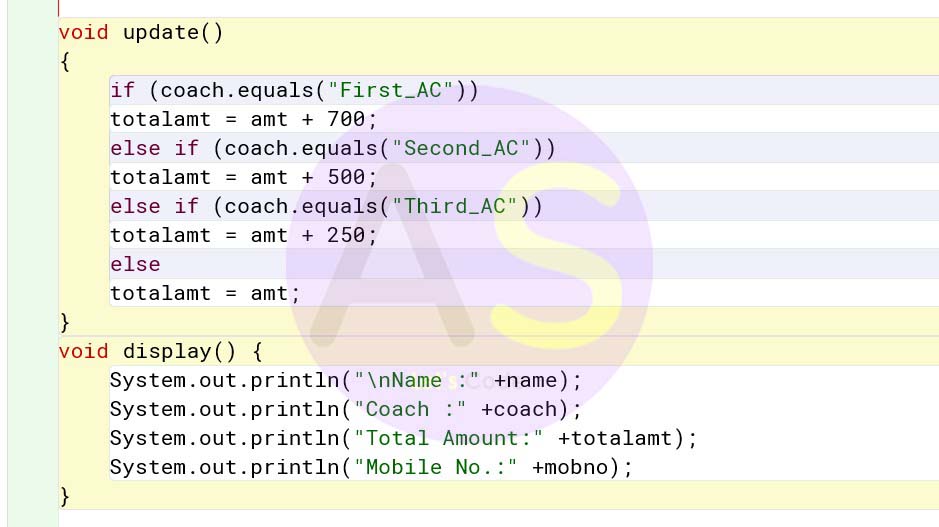
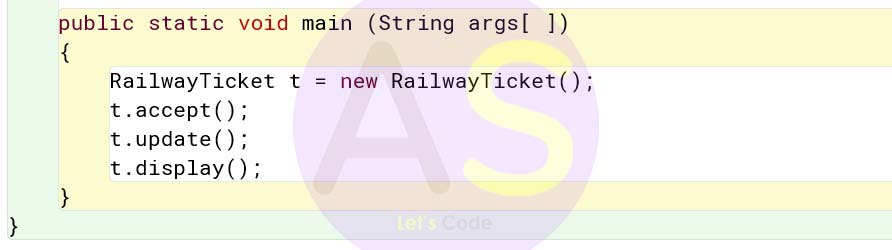
import java.util.*; class RailwayTicket { String name, coach; long mobno; int amt, totalamt; void accept( ) { Scanner sc = new Scanner(System.in); System.out.println("Enter Passenger’s Name:"); name = sc.next( ); System.out.print("Enter Mobile Number:"); mobno = sc.nextInt( ); System.out.println("Enter the type of coach "); coach = sc.next( ); System.out.print("Enter basic amount of ticket:"); amt = sc.nextInt( ); } void update() { if (coach.equals("First_AC")) totalamt = amt + 700; else if (coach.equals("Second_AC")) totalamt = amt + 500; else if (coach.equals("Third_AC")) totalamt = amt + 250; else totalamt = amt; } void display() { System.out.println("\nName :" +name); System.out.println("Coach :" +coach); System.out.println("Total Amount:" +totalamt); System.out.println("Mobile No.:" +mobno); } public static void main (String args[ ]) { RailwayTicket t = new RailwayTicket(); t.accept(); t.update(); t.display(); } }
Question 5
Write a program to input a number and check and print whether it is a Pronic number or not. (Pronic number is the number which is the product of two consecutive integers)
Examples :
12 = 3 × 4
20 = 4 × 5
42 = 6 × 7
Solution:
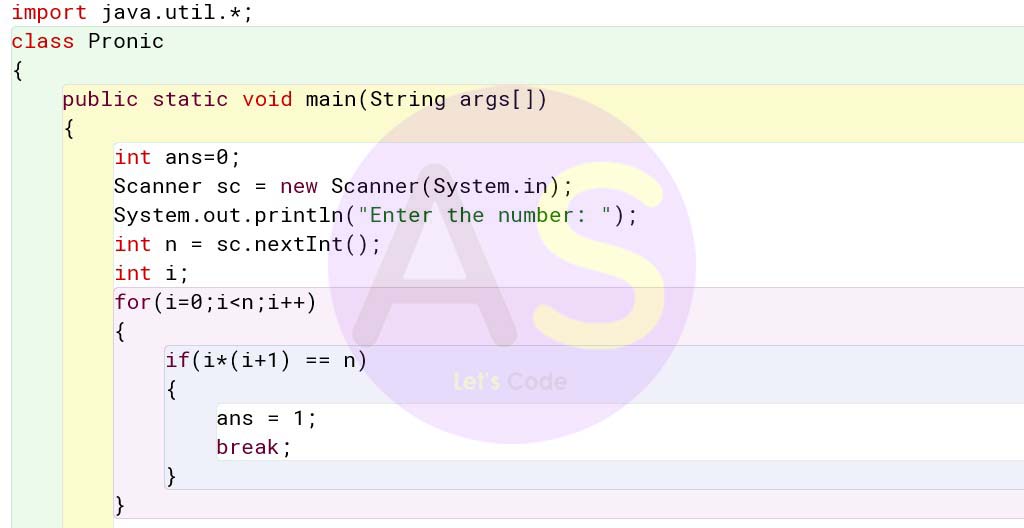
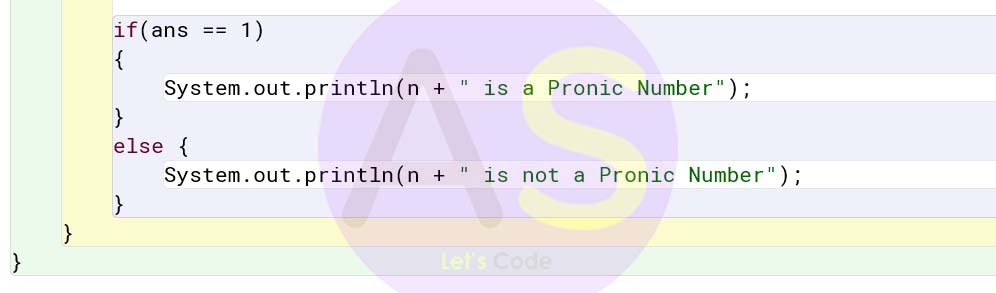
import java.util.*; class Pronic { public static void main(String args[]) { int ans=0; Scanner sc = new Scanner(System.in); System.out.println("Enter the number: "); int n = sc.nextInt(); int i; for(i=0;i< n;i++) { if(i*(i+1) == n) { ans = 1; break; } } if(ans == 1) { System.out.println(n + " is a Pronic Number"); } else { System.out.println(n + " is not a Pronic Number"); } } }
Question 6
Write a program in Java to accept a string in lower case and change the first letter of every word to upper case. Display the new string.
Sample input: we are in cyber world
Sample output : We Are In Cyber World
Solution:
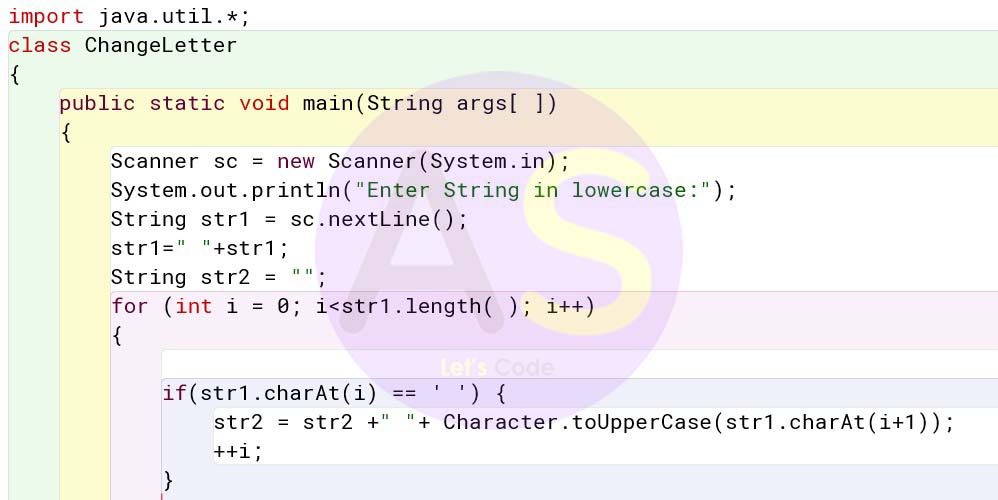
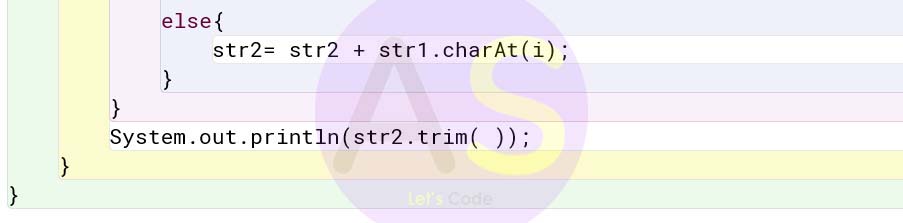
import java.util.*; class ChangeLetter { public static void main(String args[ ]) { Scanner sc = new Scanner(System.in); System.out.println("Enter String in lowercase:"); String str1 = sc.nextLine(); str1=" "+str1; String str2 = ""; for (int i = 0; i< str1.length( ); i++) { if(str1.charAt(i) == ' ') { str2 = str2 +" "+ Character.toUpperCase(str1.charAt(i+1)); ++i; } else{ str2= str2 + str1.charAt(i); } } System.out.println(str2.trim( )); } }
Question 7
Design a class to overload a function volume() as follows :
(i) double volume (double R) — with radius (R) as an argument, returns the volume of sphere using the formula.
V = 4/3 × 22/7 × R3
(ii) double volume (double H, double R) – with height(H) and radius(R) as the arguments, returns the volume of a cylinder using the formula.
V = 22/7 × R2 × H
(iii) double volume (double L, double B, double H) – with length(L), breadth(B) and Height(H) as the arguments, returns the volume of a cuboid using the formula.
Solution:
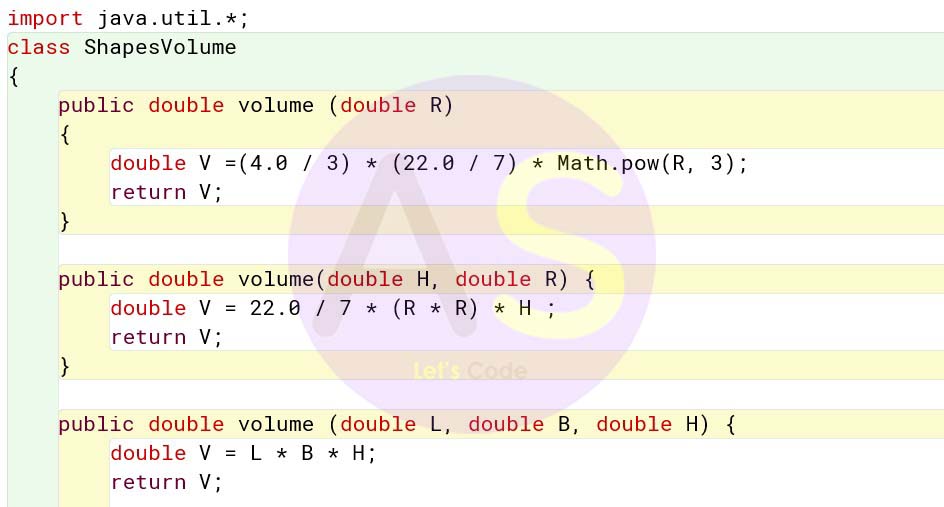
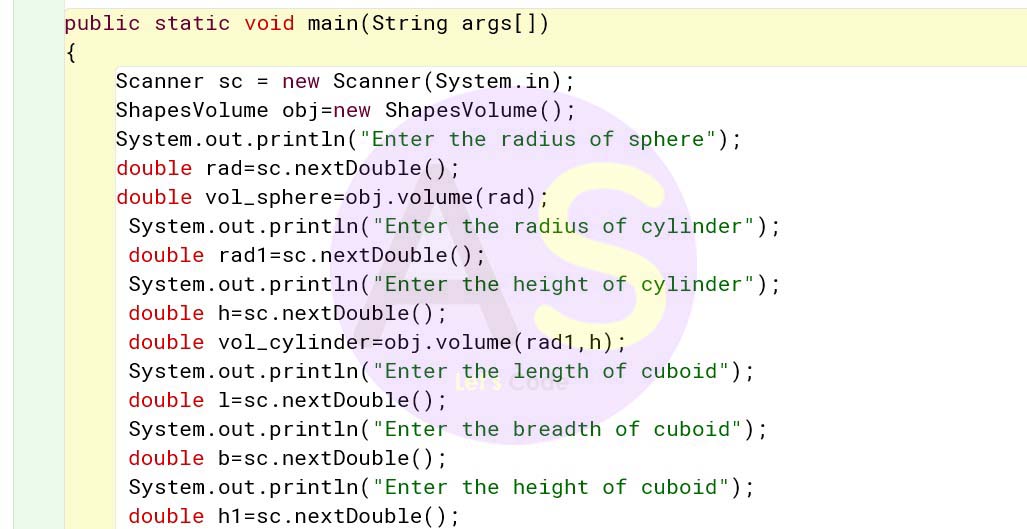
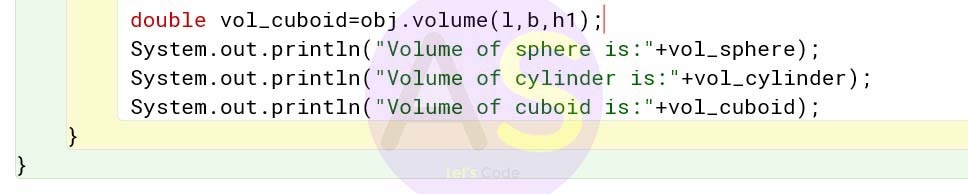
import java.util.*; class ShapesVolume { public double volume (double R) { double V =(4.0 / 3) * (22.0 / 7) * Math.pow(R, 3); return V; } public double volume(double H, double R) { double V = 22.0 / 7 * (R * R) * H ; return V; } public double volume (double L, double B, double H) { double V = L * B * H; return V; } public static void main(String args[]) { Scanner sc = new Scanner(System.in); ShapesVolume obj=new ShapesVolume(); System.out.println("Enter the radius of sphere"); double rad=sc.nextDouble(); double vol_sphere=obj.volume(rad); System.out.println("Enter the radius of cylinder"); double rad1=sc.nextDouble(); System.out.println("Enter the height of cylinder"); double h=sc.nextDouble(); double vol_cylinder=obj.volume(rad1,h); System.out.println("Enter the length of cuboid"); double l=sc.nextDouble(); System.out.println("Enter the breadth of cuboid"); double b=sc.nextDouble(); System.out.println("Enter the height of cuboid"); double h1=sc.nextDouble(); double vol_cuboid=obj.volume(l,b,h1); System.out.println("Volume of sphere is:"+vol_sphere); System.out.println("Volume of cylinder is:"+vol_cylinder); System.out.println("Volume of cuboid is:"+vol_cuboid); } }
Question 8
Write a menu driven program to display the pattern as per user’s choice.
Pattern 1
A B C D E
A B C D
A B C
A B
A
Pattern 2
B
L L
U U U
E E E E
For an incorrect option, an appropriate error message should be displayed.
Solution:
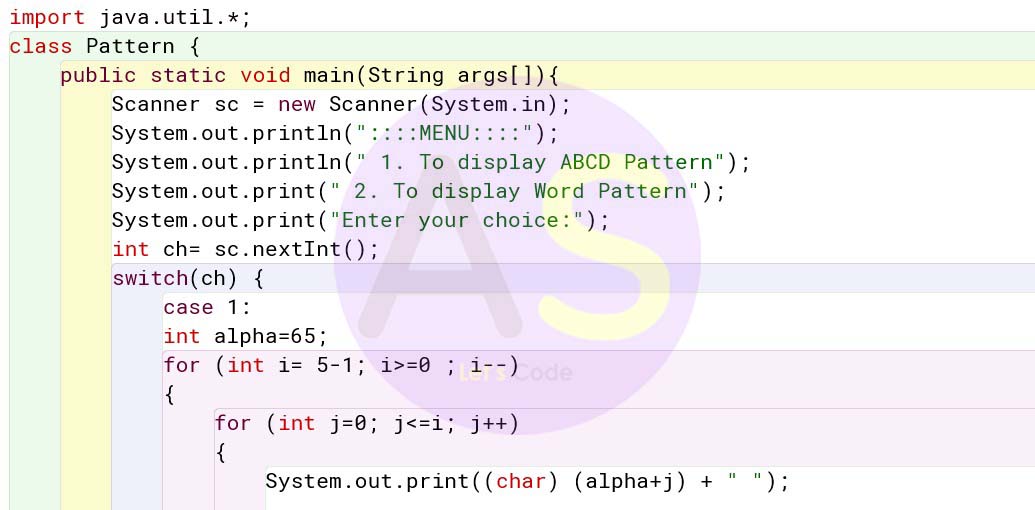
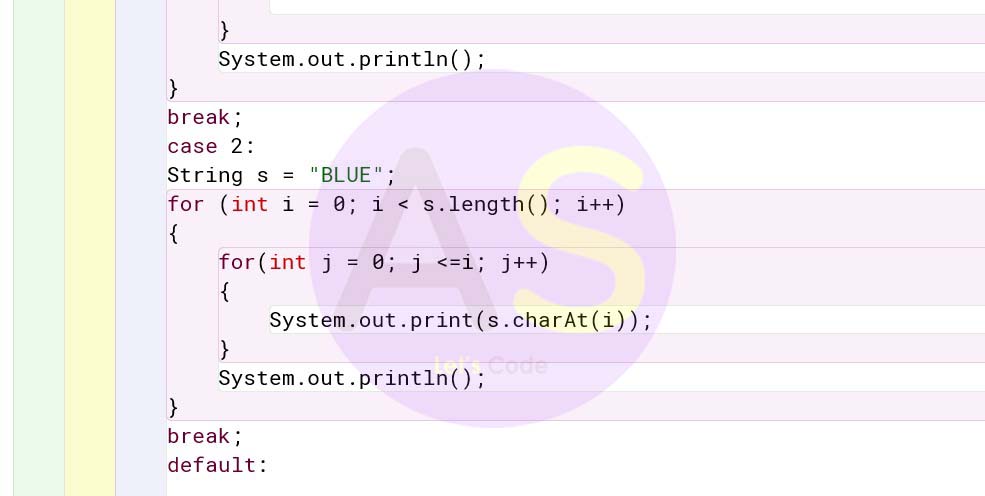
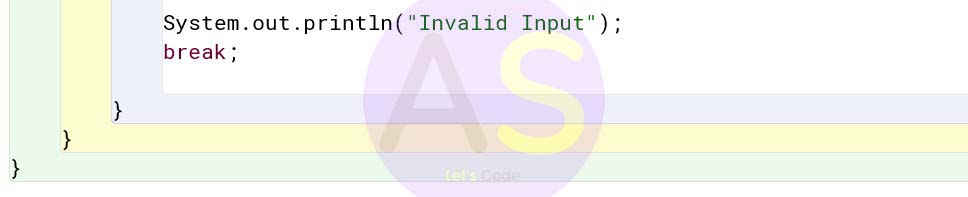
import java.util.*; class Pattern { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("::::MENU::::"); System.out.println(" 1. To display ABCD Pattern"); System.out.print(" 2. To display Word Pattern"); System.out.print("Enter your choice:"); int ch= sc.nextInt(); switch(ch) { case 1: int alpha=65; for (int i= 5-1; i>=0 ; i--) { for (int j=0; j<=i; j++) { System.out.print((char) (alpha+j) + " "); } System.out.println(); } break; case 2: String s = "BLUE"; for (int i = 0; i < s.length(); i++) { for(int j = 0; j <=i; j++) { System.out.print(s.charAt(i)); } System.out.println(); } break; default: System.out.println("Invalid Input"); break; } } }
Question 9
Write a program to accept name and total marks of N number of students in two single subscript array name[] and totalmarks[ ].
Calculate and print:
(i) The average of the total marks obtained by N Number of students.
[average = (sum of total marks of all the students)/N]
(ii) Deviation of each student’s total marks with the average.
[deviation = total marks of a student – average]
Solution:
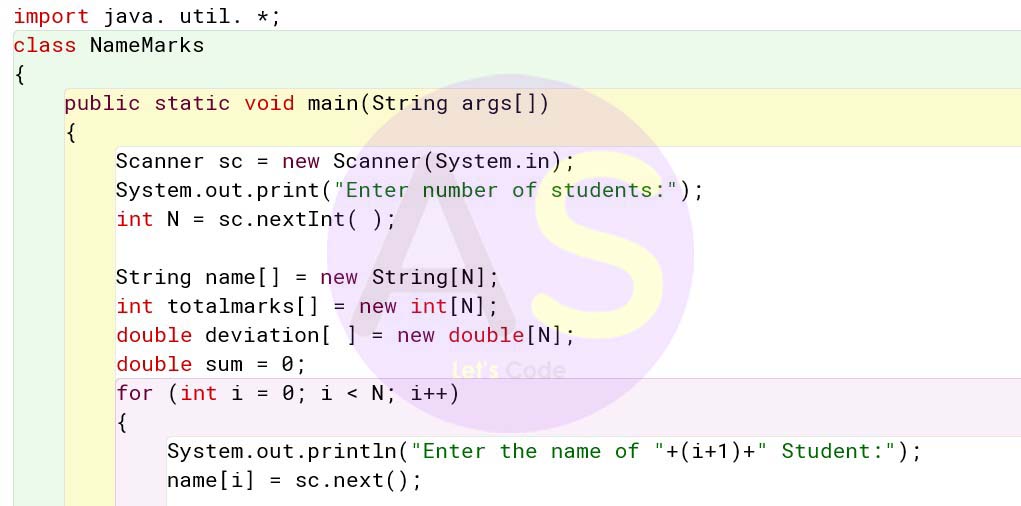
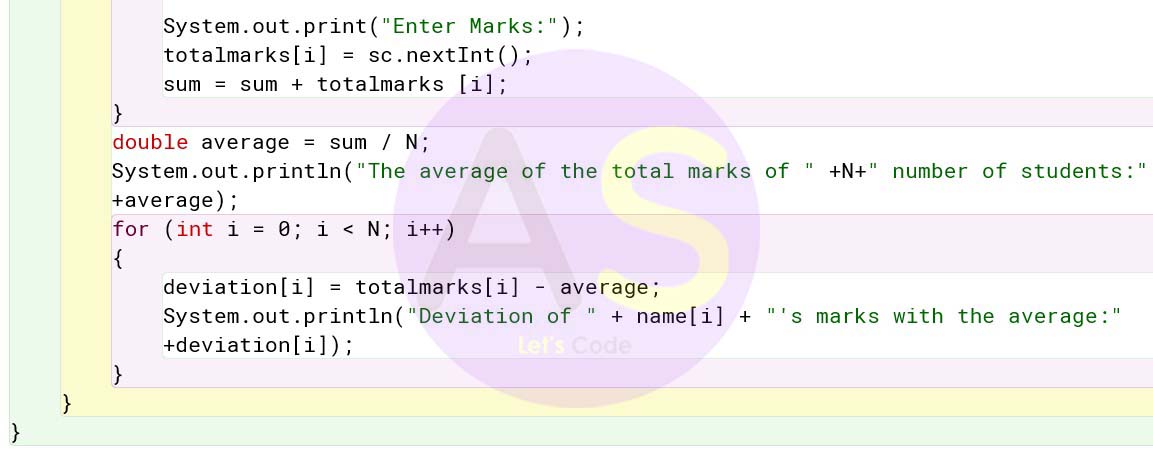
import java. util. *; class NameMarks { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter number of students:"); int N = sc.nextInt( ); String name[] = new String[N]; int totalmarks[] = new int[N]; double deviation[ ] = new double[N]; double sum = 0; for (int i = 0; i < N; i++) { System.out.println("Enter the name of "+(i+1)+" Student:"); name[i] = sc.next(); System.out.print("Enter Marks:"); totalmarks[i] = sc.nextInt(); sum = sum + totalmarks [i]; } double average = sum / N; System.out.println("The average of the total marks of " +N+" number of students:" +average); for (int i = 0; i < N; i++) { deviation[i] = totalmarks[i] - average; System.out.println("Deviation of " + name[i] + "'s marks with the average:" +deviation[i]); } } }