Code is copied!
CLASS 10 ICSE Computer Science - Java Question Paper 2017
Maximum Marks:100
Time allowed: Two hours
Answers to this Paper must be written on the paper provided separately.
You will not be allowed to write during the first 15 minutes.
This time is to be spent in reading the question paper.
The time given at the head of this Paper is the time allowed for writing the answers.
This Paper is divided into two Sections.
Attempt all questions from Section A and any four questions from Section B.
Answers to this Paper must be written on the paper provided separately.
The intended marks for questions or parts of questions are given in brackets[] .
SECTION A
(Attempt all questions from this Section.)
Question 1
(a)
What is inheritance?
Solution
Inheritance is the process by which a sub class acquires the properties and methods of super class.
(b)
Name the operators listed below:
1. <
2. ++
3. &&
4. ? :
Solution
1. Less than operator. (It is a relational operator)
2. Increment operator. (It is an arithmetic operator)
3. Logical AND operator. (It is a logical operator)
4. Ternary operator. (It is a conditional operator)
2. Increment operator. (It is an arithmetic operator)
3. Logical AND operator. (It is a logical operator)
4. Ternary operator. (It is a conditional operator)
(c)
State the number of bytes occupied by char and int data types.
Solution
char = 2 bytes
int = 4 bytes.
int = 4 bytes.
(d)
Write one difference between / and % operator.
Solution
/ operator gives us the quotient whereas % operator gives us the remainder.
(e)
String x[] = {"SAMSUNG", "NOKIA", "SONY", "MICROMAX", "BLACKBERRY"};
Give the output of the following statements:
1. System.out.println(x[1]);
2. System.out.println(x[3].length());
Solution
1. The output of System.out.println(x[1]); is NOKIA.
2. The output of System.out.println(x[3].length()); is 8.
2. The output of System.out.println(x[3].length()); is 8.
Question 2
(a)
Name the following:
1. A keyword used to call a package in the program.
2. Any one reference data type.
Solution
1. import
2. Array
(b) What are the two ways of invoking functions?
Solution
The two ways of invoking functions is :
Call by value and call by reference.
(c)
State the data type and value of res after the following is executed:
char ch='t';
res= Character.toUpperCase(ch);
Solution
Data type of res is char and its value is T.
(d)
Give the output of the following program segment and also mention the number of times the loop is executed:
int a,b;
for (a = 6, b = 4; a <= 24; a = a + 6)
{
if (a%b == 0)
break;
}
System.out.println(a);
Solution
Output of the above code is
12
loop executes 2 times.
(e)
Write the output:
char ch = 'F';
int m = ch;
m=m+5;
System.out.println(m + " " + ch);
Solution
Output of the above code is:
75 F
Question 3
(a)
Write a Java expression for the following:
ax5 + bx3 + c
Solution
a * Math.pow(x, 5) + b * Math.pow(x, 3) + c
(b)
What is the value of x1 if x=5?
x1= ++x – x++ + --x
Solution
x1 = ++x – x++ + --x
⇒ x1 = 6 - 6 + 6
⇒ x1 = 0 + 6
⇒ x1 = 6
(c) Why is an object called an instance of a class?
Solution
A class can create objects with different characteristics and common behaviour. So, we can say that an object represents a specific state of the class. Hence, an Object is called an instance of a class.
(d)
Convert following do-while loop into for loop.
int i = 1;
int d = 5;
do {
d=d*2;
System.out.println(d);
i++ ; } while ( i<=5);
Solution
int d = 5;
for (int i = 1; i <= 5; i++) {
d=d*2;
System.out.println(d);
}
(e) Differentiate between constructor and function.
Solution
Constructor | Function |
---|---|
Constructor is used to initialize a newly created object. | Function is a block containing set of statements used to perform a specific task. |
Constructor has the same name as class name. | Function should have a different name than class name. |
Constructor has no return type not even void. | Function requires a valid return type. |
(f)
Write the output for the following:
String s="Today is Test";
System.out.println(s.indexOf('T'));
System.out.println(s.substring(0,7) + " " +"Holiday");
Solution
Output of the above code is:
0
Today i Holiday
(g)
What are the values stored in variables r1 and r2:
1. double r1 = Math.abs(Math.min(-2.83, -5.83));
2. double r2 = Math.sqrt(Math.floor(16.3));
Solution
1. r1 = 5.83
2. r2 = 4.0
(h)
Give the output of the following code:
String A ="26", B="100";
String D=A+B+"200";
int x= Integer.parseInt(A);
int y = Integer.parseInt(B);
int d = x+y;
System.out.println("Result 1 = " + D);
System.out.println("Result 2 = " + d);
Solution
Output of the above code is:
Result 1 = 26100200
Result 2 = 126
(i)
Analyze the given program segment and answer the following questions:
for(int i=3;i<=4;i++ ) {
for(int j=2;j< i;j++ ) {
System.out.print("" ); }
System.out.println("WIN" ); }
1. How many times does the inner loop execute?
2. Write the output of the program segment.
Solution
Inner loop executes 3 times.
(For 1st iteration of outer loop, inner loop executes once. For 2nd iteration of outer loop, inner loop executes two times.)
Output:
WIN
WIN
(j) What is the difference between the Scanner class functions next() and nextLine()?
Solution
next() | nextLine() |
---|---|
It can read only a single word i.e. it reads the input only till space. | It can read a full sentence including spaces i.e. it reads the input till the end of line. |
It places the cursor in the same line. | It places the cursor in the next line. |
SECTION B
(Attempt any four questions from this Section)
Question 4
Define a class ElectricBill with the following specifications :
class : ElectricBill
Instance variables /data member :
String n – to store the name of the customer
int units – to store the number of units consumed
double bill – to store the amount to be paid
Member methods :
void accept ( ) – to accept the name of the customer and number of units consumed
void calculate ( ) – to calculate the bill as per the following tariff :
A surcharge of 2.5% charged if the number of units consumed is above 300 units.
void print() - to print the details as follows:
Name of the customer:.........
Number of units consumed:.........
Bill amount:.......
Write a main method to create an object of the class and call the above member methods.

Solution:
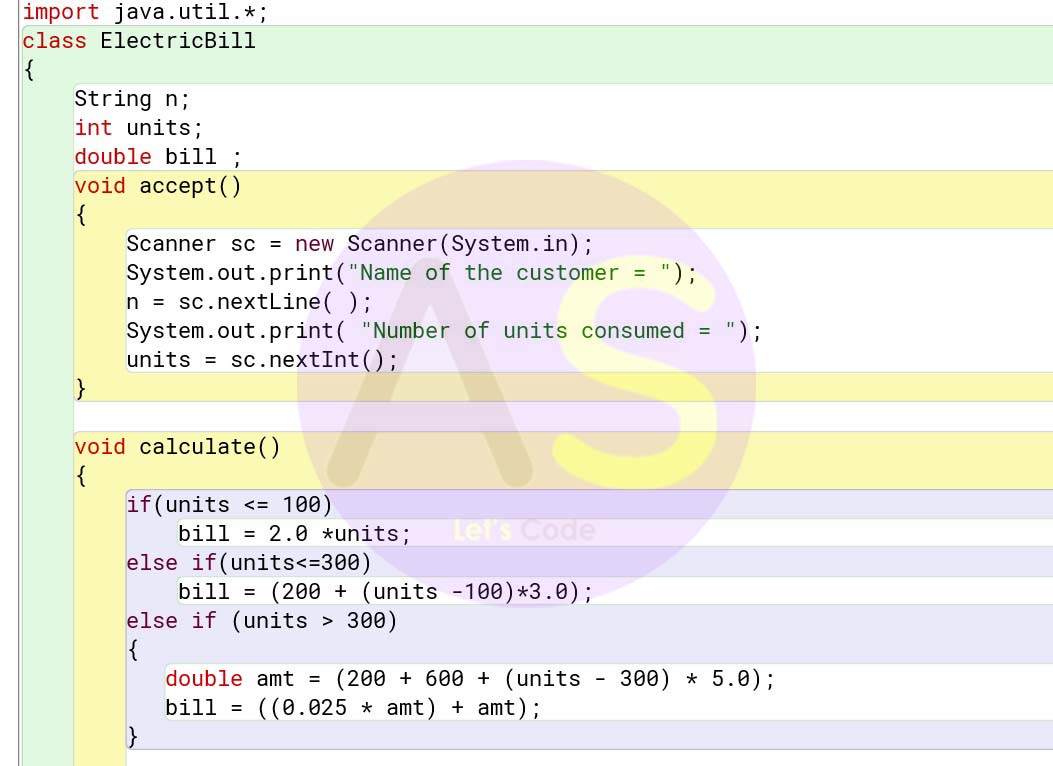
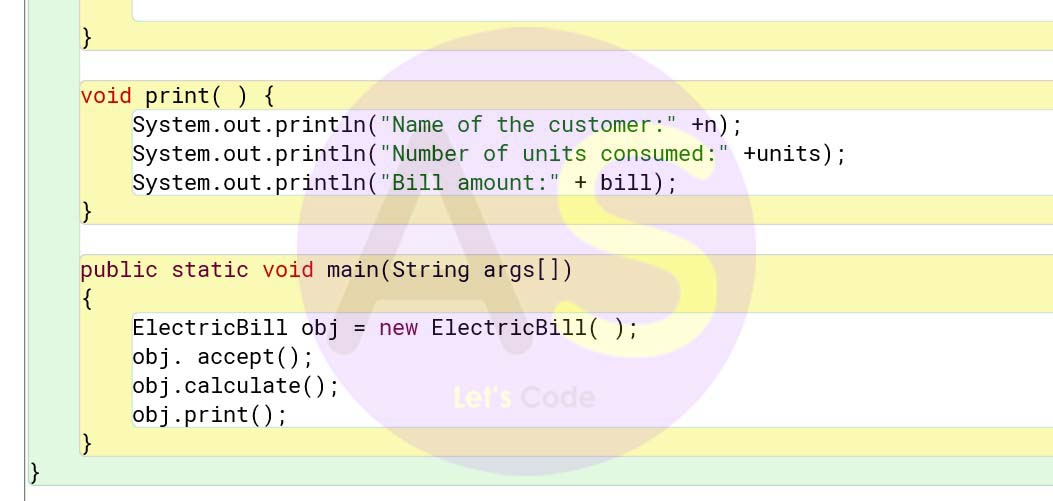
import java.util.*; class ElectricBill { String n; int units; double bill ; void accept() { Scanner sc = new Scanner(System.in); System.out.print("Name of the customer = "); n = sc.nextLine( ); System.out.print( "Number of units consumed = "); units = sc.nextInt(); } void calculate() { if(units <= 100) bill = 2.0 *units; else if(units<=300) bill = (200 + (units -100)*3.0); else if (units > 300) { double amt = (200 + 600 + (units - 300) * 5.0); bill = ((0.025 * amt) + amt); } } void print( ) { System.out.println("Name of the customer:" +n); System.out.println("Number of units consumed:" +units); System.out.println("Bill amount:" + bill); } public static void main(String args[]) { ElectricBill obj = new ElectricBill( ); obj. accept(); obj.calculate(); obj.print(); } }
Question 6
Using switch statement, write a menu driven program for the following :
(i) To find and display the sum of the series given below :
S = x1 -x2 +x2 – x4 + x5 – x20
(where x = 2)
(ii) To display the following series :
1 11 111 1111 11111
For an incorrect option, an appropriate error message should be displayed.
Solution:
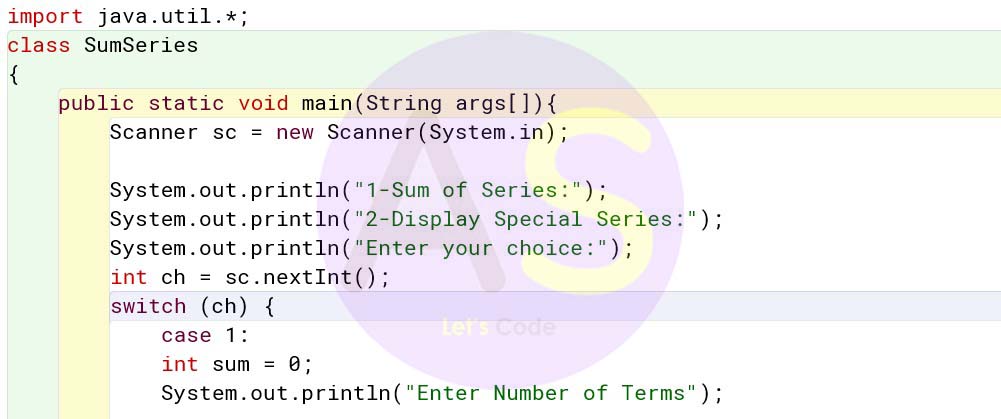
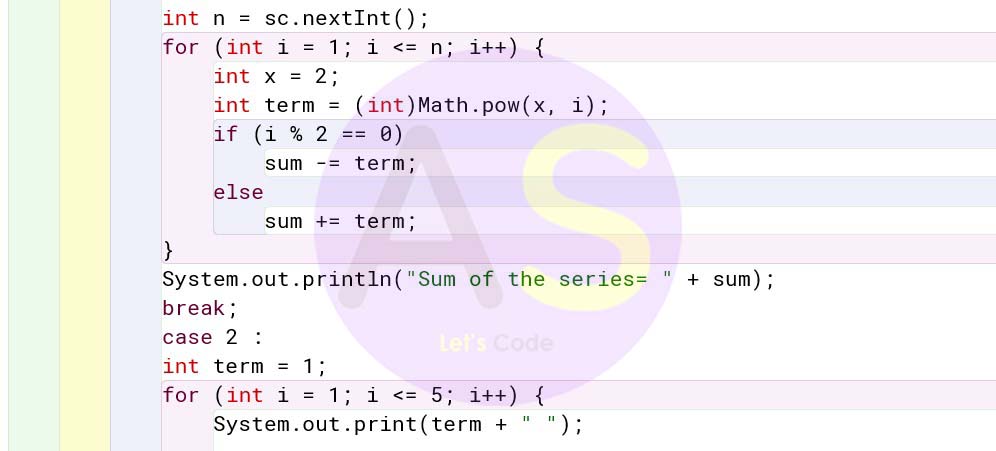
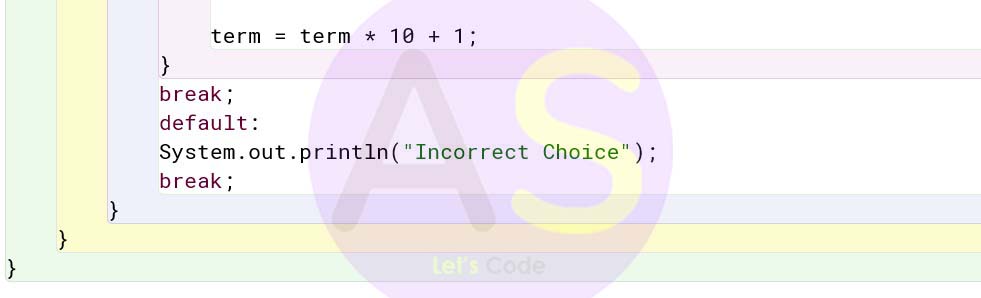
import java.util.*; class SumSeries { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("1-Sum of Series:"); System.out.println("2-Display Special Series:"); System.out.println("Enter your choice:"); int ch = sc.nextInt(); switch (ch) { case 1: int sum = 0; System.out.println("Enter Number of Terms"); int n = sc.nextInt(); for (int i = 1; i <= n; i++) { int x = 2; int term = (int)Math.pow(x, i); if (i % 2 == 0) sum -= term; else sum += term; } System.out.println("Sum of the series= " + sum); break; case 2 : int term = 1; for (int i = 1; i <= 5; i++) { System.out.print(term + " "); term = term * 10 + 1; } break; default: System.out.println("Incorrect Choice"); break; } } }
Question 7
Write a program to input integer elements into an array of size 20 and perform the following operations:
(i) Display largest number from the array.
(ii) Display smallest number from the array.
(iii) Display sum of all the elements of the array.
Solution:
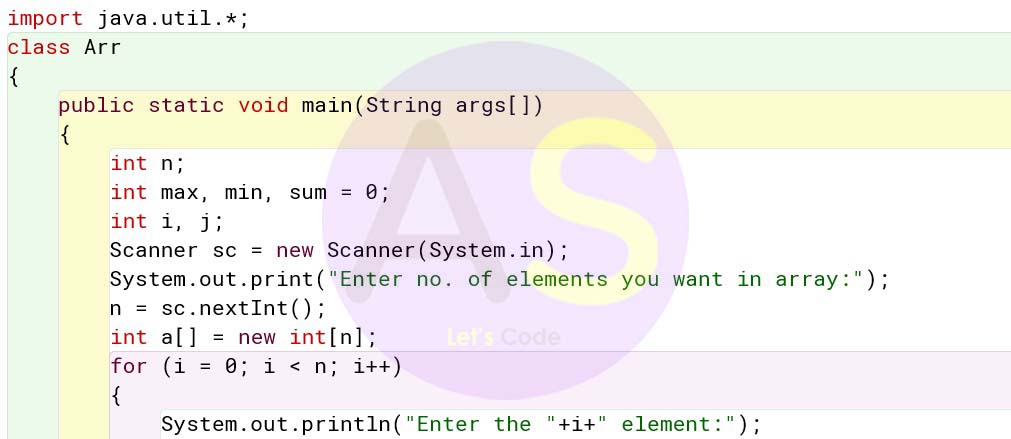
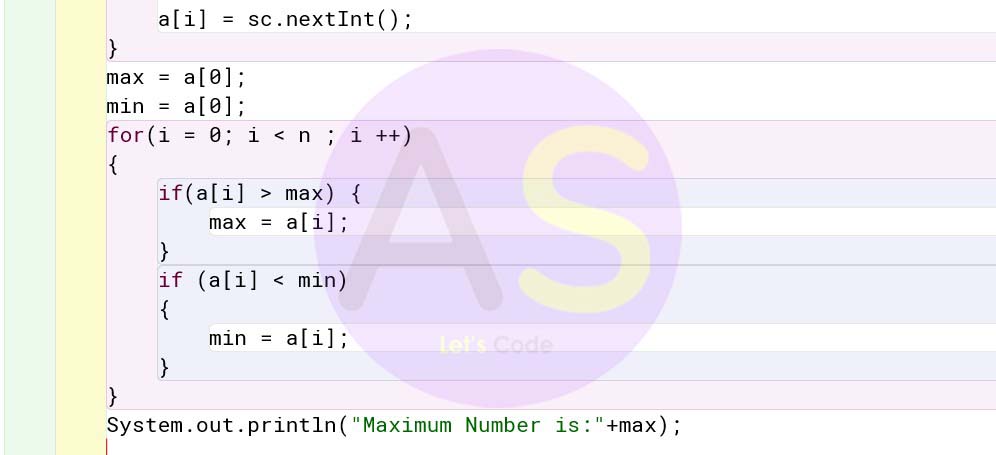
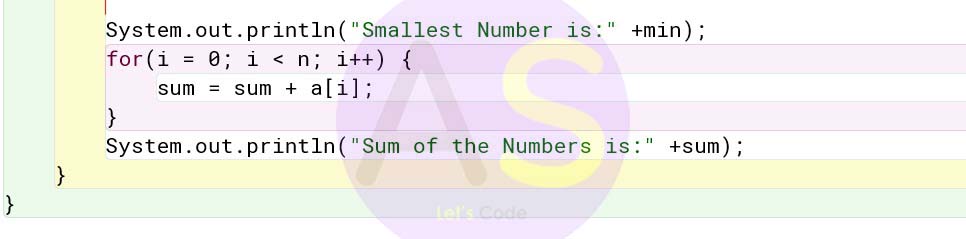
import java.util.*; class Arr { public static void main(String args[]) { int n; int max, min, sum = 0; int i, j; Scanner sc = new Scanner(System.in); System.out.print("Enter no. of elements you want in array:"); n = sc.nextInt(); int a[] = new int[n]; for (i = 0; i < n; i++) { System.out.println("Enter the "+i+" element:"); a[i] = sc.nextInt(); } max = a[0]; min = a[0]; for(i = 0; i < n ; i ++) { if(a[i] > max) { max = a[i]; } if (a[i] < min) { min = a[i]; } } System.out.println("Maximum Number is:"+max); System.out.println("Smallest Number is:" +min); for(i = 0; i < n; i++) { sum = sum + a[i]; } System.out.println("Sum of the Numbers is:" +sum); } }
Question 8
Design a class to overload a function check ( ) as follows :
(i) void check (String str, char ch) – to find and print the frequency of a character in a string.
Example :
Input:
str = “success”
ch = ‘s’ .
Output:
number of s present is = 3
(ii) void check(String si) – to display only vowels from string si, after converting it to lower case.
Example:
Input:
s1 = “computer”
Output:
o u e
Solution:
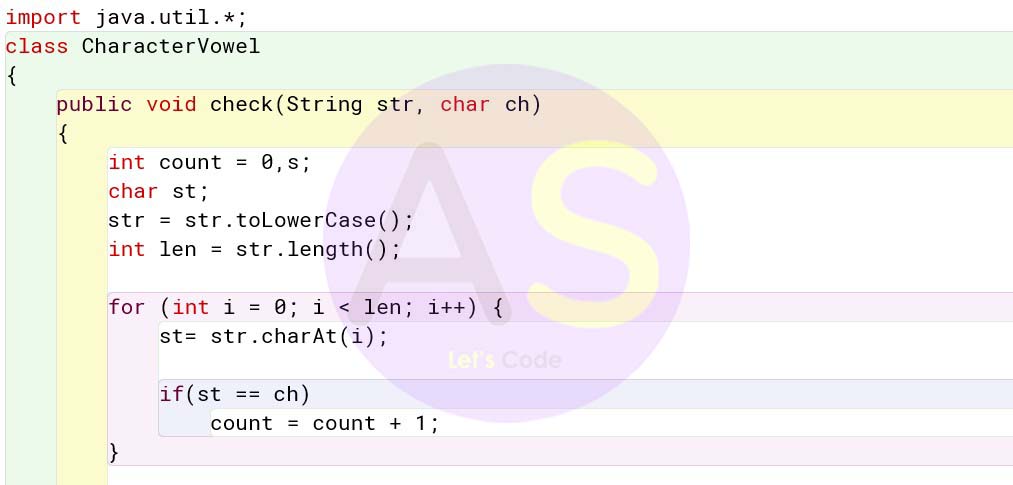
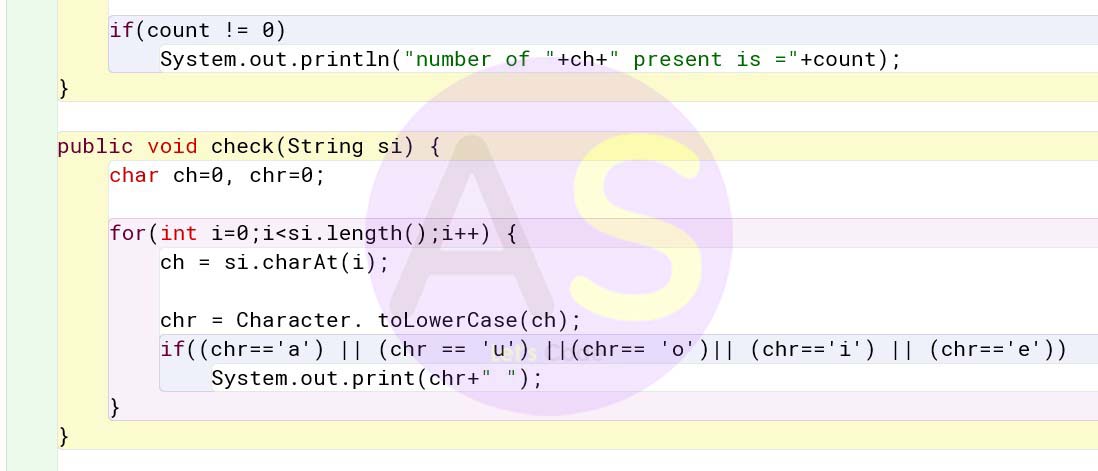
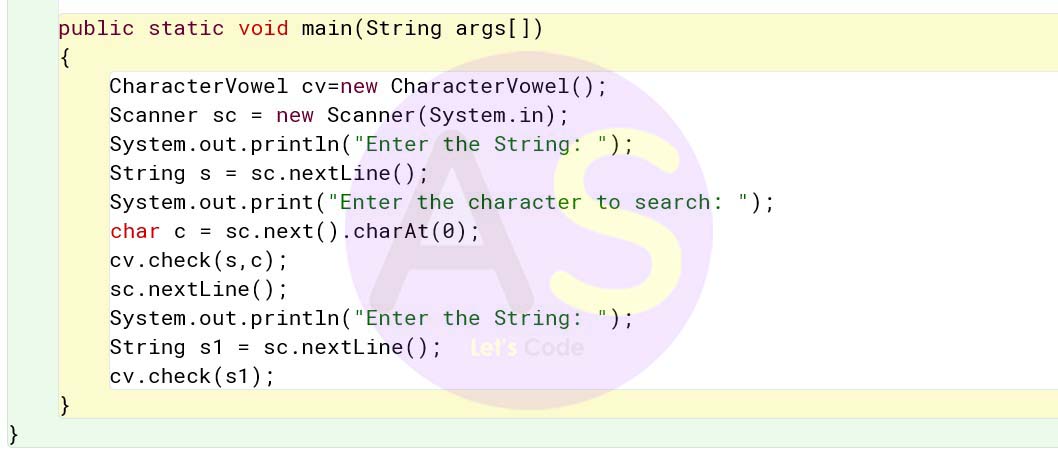
import java.util.*; class CharacterVowel { public void check(String str, char ch) { int count = 0,s; char st; str = str.toLowerCase(); int len = str.length(); for (int i = 0; i < len; i++) { st= str.charAt(i); if(st == ch) count = count + 1; } if(count != 0) System.out.println("number of "+ch+" present is ="+count); } public void check(String si) { char ch=0, chr=0; for(int i=0;i< si.length();i++) { ch = si.charAt(i); chr = Character. toLowerCase(ch); if((chr=='a') || (chr == 'u') ||(chr== 'o')|| (chr=='i') || (chr=='e')) System.out.print(chr+" "); } } public static void main(String args[]) { CharacterVowel cv=new CharacterVowel(); Scanner sc = new Scanner(System.in); System.out.println("Enter the String: "); String s = sc.nextLine(); System.out.print("Enter the character to search: "); char c = sc.next().charAt(0); cv.check(s,c); sc.nextLine(); System.out.println("Enter the String: "); String s1 = sc.nextLine(); cv.check(s1); } }
Question 9
Write a program to input forty words in an array. Arrange these words in descending order of alphabets, using selection sort technique. Print the sorted array.
Solution:
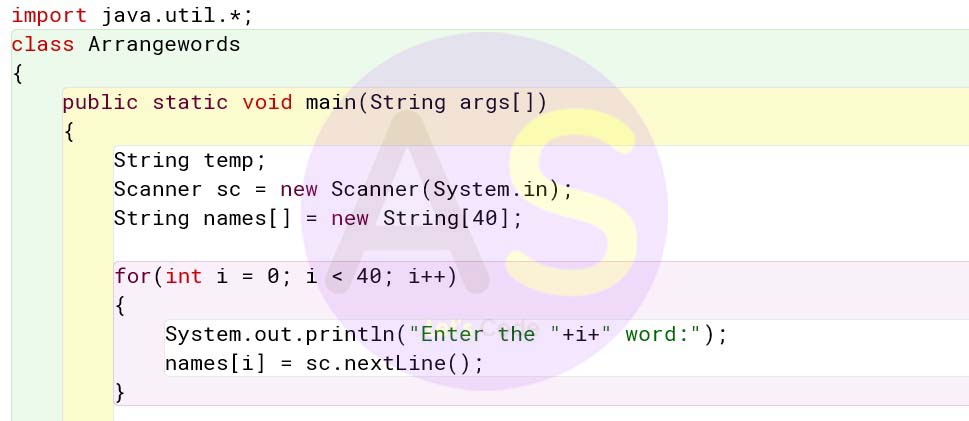
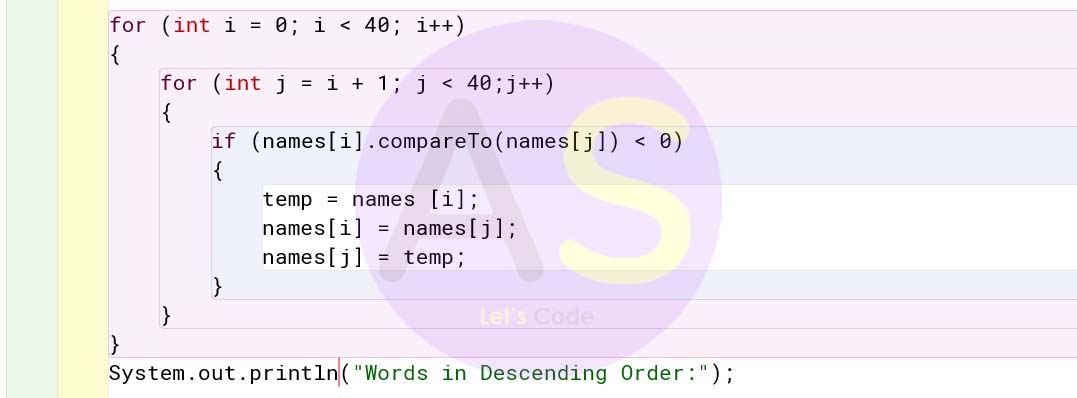
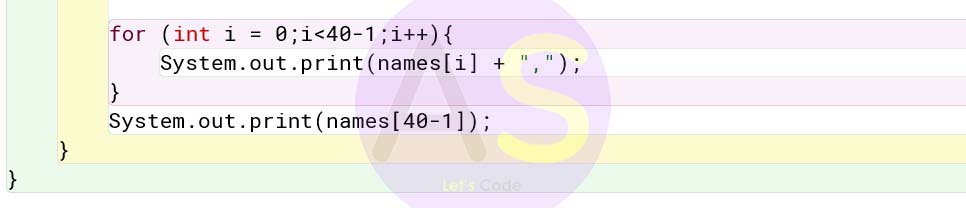
import java.util.*; class Arrangewords { public static void main(String args[]) { String temp; Scanner sc = new Scanner(System.in); String names[] = new String[40]; for(int i = 0; i < 40; i++) { System.out.println("Enter the "+i+" word:"); names[i] = sc.nextLine(); } for (int i = 0; i < 40; i++) { for (int j = i + 1; j < 40;j++) { if (names[i].compareTo(names[j]) < 0) { temp = names [i]; names[i] = names[j]; names[j] = temp; } } } System.out.println("Words in Descending Order:"); for (int i = 0;i< 40-1;i++){ System.out.print(names[i] + ","); } System.out.print(names[40-1]); } }