Code is copied!
CLASS 10 ICSE Computer Science - Java Question Paper 2016
Maximum Marks:100
Time allowed: Two hours
Answers to this Paper must be written on the paper provided separately.
You will not be allowed to write during the first 15 minutes.
This time is to be spent in reading the question paper.
The time given at the head of this Paper is the time allowed for writing the answers.
This Paper is divided into two Sections.
Attempt all questions from Section A and any four questions from Section B.
Answers to this Paper must be written on the paper provided separately.
The intended marks for questions or parts of questions are given in brackets[] .
SECTION A
(Attempt all questions from this Section.)
Question 1
(a)
Define Encapsulation.
Solution
The wrapping of data and methods into a single unit is called as encapsulation.
(b)
What are keywords ? Give an example.
Solution
Keywords are reserved words that have a special meaning to the Java compiler. Example: class, public, int, etc.
(c)
Name any two library packages.
Solution
Two library packages are:
1. java.io
2. java.util
1. java.io
2. java.util
(d)
Name the type of error ( syntax, runtime or logical error) in each case given below:
1. Math.sqrt (36 – 45)
2. int a;b;c;
Solution
1. Runtime Error
2. Syntax Error
2. Syntax Error
(e)
If int x[] = { 4, 3, 7, 8, 9, 10 }; what are the values of p and q ?
1. p = x.length
2. q = x[2] + x[5] * x[1]
Solution
1. 6
2. q = x[2] + x[5] * x[1]
q = 7 + 10 * 3
q = 7 + 30
q = 37
2. q = x[2] + x[5] * x[1]
q = 7 + 10 * 3
q = 7 + 30
q = 37
Question 2
(a) State the difference between == operator and equals() method.
Solution
equals() | == |
---|---|
It is a method. | It is a relational operator. |
It is used to check if the contents of two strings are same or not. | It is used to check if two variables are same or not. |
(b)
What are the types of casting shown by the following examples:
1. char c = (char) 120;
2. int x = ‘t’;
Solution
1. Explicit Cast.
2. Implicit Cast.
(c) Differentiate between formal parameter and actual parameter.
Solution
Formal Parameters | Actual Parameters |
---|---|
Formal parameters are those which appears in function definition. | Actual parameters are those which appears in function calling statement. |
They represent the values received by the called function | They represent the values passed to the called function. |
(d)
Write a function prototype of the following:
A function PosChar which takes a string argument and a character argument and returns an integer value.
Solution
int PosChar(String s, char ch)
(e) Name any two types of access specifiers.
Solution
1. public
2. private
Question 3
(a)
Give the output of the following string functions:
1. "MISSISSIPPI".indexOf('S') + "MISSISSIPPI".lastIndexOf('I')
2. "CABLE".compareTo("CADET")
Solution
1. 2 + 10 = 12
2. ASCII Code of B - ASCII Code of D
⇒ 66 - 68
⇒ -2
(b)
Give the output of the following Math functions:
1. Math.ceil(4.2)
2. Math.abs(-4)
Solution
1. 5.0
2. 4
(c) What is a parameterized constructor ?
Solution
A Parameterised constructor receives parameters at the time of creating an object and initializes the object with the received values.
(d)
Write down java expression for:
T = √(A2 + B2 + C2)
Solution
T = Math.sqrt(a*a + b*b + c*c)
(e)
Rewrite the following using ternary operator:
if (x%2 == 0)
System.out.print("EVEN");
else
System.out.print("ODD");
Solution
System.out.print(x%2 == 0 ? "EVEN" : "ODD");
(f)
Convert the following while loop to the corresponding for loop:
int m = 5, n = 10;
while (n>=1)
{
System.out.println(m*n);
n--;
}
Solution
int m = 5;
for (int n = 10; n >= 1; n--) {
System.out.println(m*n);
}
(g) Write one difference between primitive data types and composite data types.
Solution
Primitive Data Types | Composite Data Types |
---|---|
They are pre-defined / inbuilt data types. | These data type are defined by the user and made up of primitive data types values |
Example : byte , short, long, float, char, int, double, boolean | Example : Array, class |
(h)
Analyze the given program segment and answer the following questions:
1. Write the output of the program segment.
2. How many times does the body of the loop gets executed ?
for(int m = 5; m <= 20; m += 5)
{ if(m % 3 == 0)
break;
else
if(m % 5 == 0)
System.out.println(m);
continue;
}
Solution
Output:
5
10
Loop executes 3 times.
(i)
Give the output of the following expression:
a+= a++ + ++a + --a + a-- ; when a = 7
Solution
a+= a++ + ++a + --a + a--
⇒ a = a + (a++ + ++a + --a + a--)
⇒ a = 7 + (7 + 9 + 8 + 8)
⇒ a = 7 + 32
⇒ a = 39
(j)
Write the return type of the following library functions:
1. isLetterOrDigit(char)
2. replace(char, char)
Solution
1. boolean
2. String
SECTION B
(Attempt any four questions from this Section)
Question 4
Define a class named BookFair with the following description:
Instance variables/Data members :
String Bname — stores the name of the book
double price — stores the price of the book
Member methods :
(i) BookFair() — Default constructor to initialize data members
(ii) void Input() — To input and store the name and the price of the book.
(iii) void calculate() — To calculate the price after discount. Discount is calculated based on the following criteria.
(iv) void display() — To display the name and price of the book after discount. Write a main method to create an object of the class and call the above member methods.
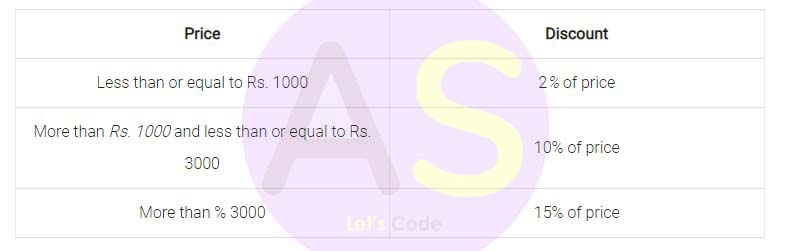
Solution:
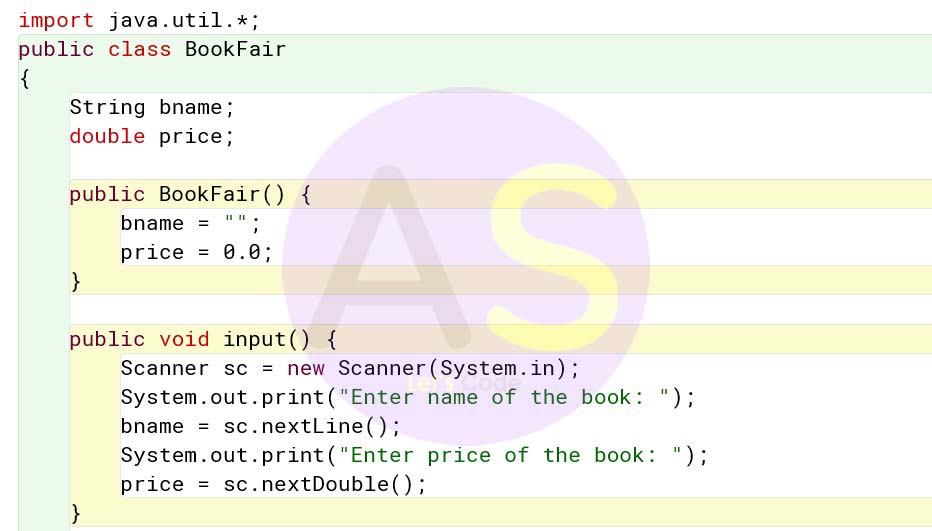
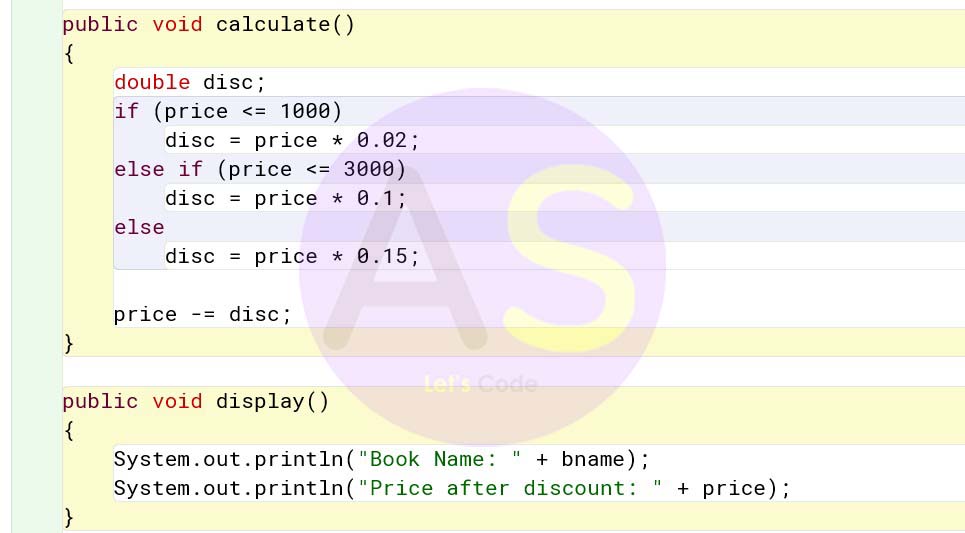
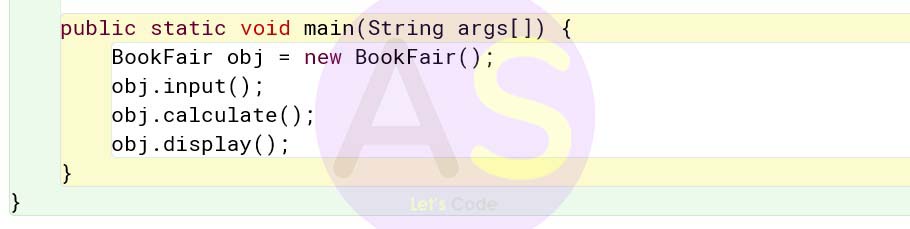
import java.util.*; public class BookFair { String bname; double price; public BookFair() { bname = ""; price = 0.0; } public void input() { Scanner sc = new Scanner(System.in); System.out.print("Enter name of the book: "); bname = sc.nextLine(); System.out.print("Enter price of the book: "); price = sc.nextDouble(); } public void calculate() { double disc; if (price <= 1000) disc = price * 0.02; else if (price <= 3000) disc = price * 0.1; else disc = price * 0.15; price -= disc; } public void display() { System.out.println("Book Name: " + bname); System.out.println("Price after discount: " + price); } public static void main(String args[]) { BookFair obj = new BookFair(); obj.input(); obj.calculate(); obj.display(); } }
Question 5
Using the switch statement, write a menu driven program for the following:
(i) To print the Floyd’s triangle [Given below]
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
(ii) To display the following pattern:
I
I C
I C S
I C S E
For an incorrect option, an appropriate error message should be displayed.
Solution:
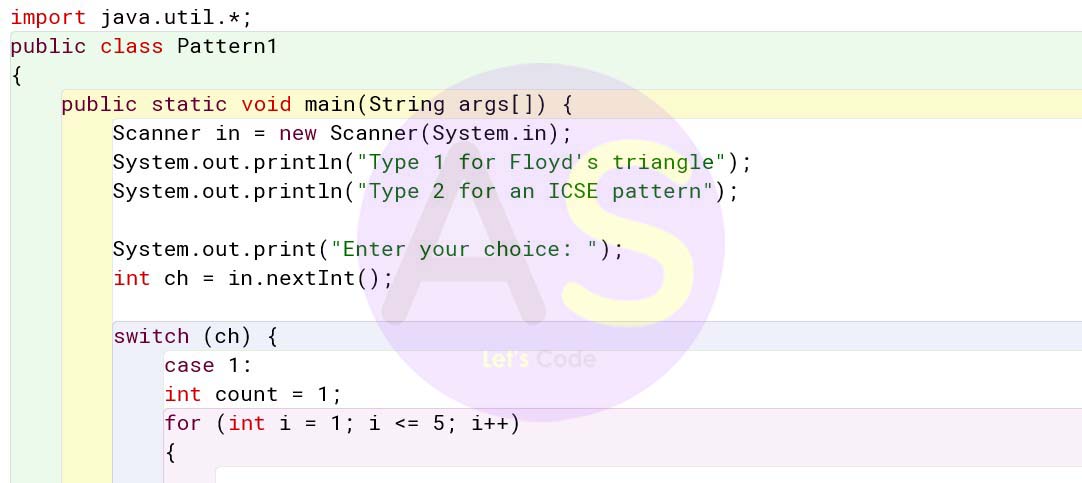
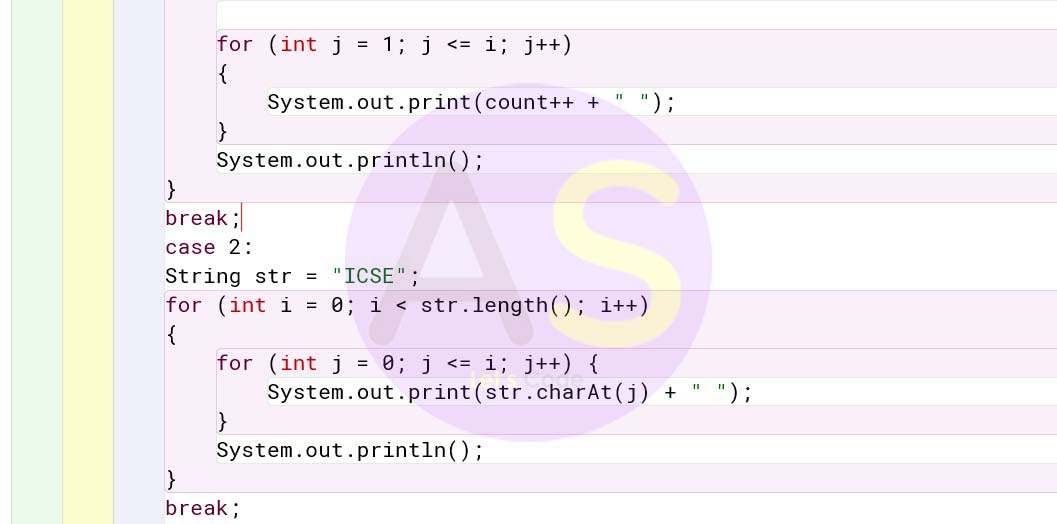

import java.util.*; public class Pattern1 { public static void main(String args[]) { Scanner in = new Scanner(System.in); System.out.println("Type 1 for Floyd's triangle"); System.out.println("Type 2 for an ICSE pattern"); System.out.print("Enter your choice: "); int ch = in.nextInt(); switch (ch) { case 1: int count = 1; for (int i = 1; i <= 5; i++) { for (int j = 1; j <= i; j++) { System.out.print(count++ + " "); } System.out.println(); } break; case 2: String str = "ICSE"; for (int i = 0; i < str.length(); i++) { for (int j = 0; j <= i; j++) { System.out.print(str.charAt(j) + " "); } System.out.println(); } break; default: System.out.println("Incorrect Choice"); } } }
Question 6
Special words are those words which starts and ends with the same letter.
Examples:
EXISTENCE
COMIC
WINDOW
Palindrome words are those words which read the same from left to right and vice versa
Examples:
MALAYALAM
MADAM
LEVEL
ROTATOR
CIVIC
All palindromes are special words, but all special words are not palindromes. Write a program to accept a word check and print Whether the word is a palindrome or only special word.
Solution:
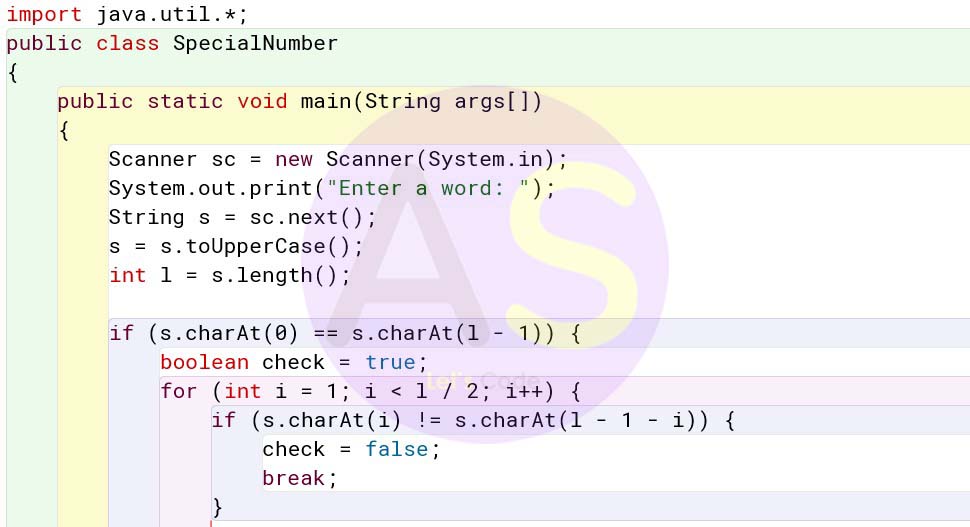
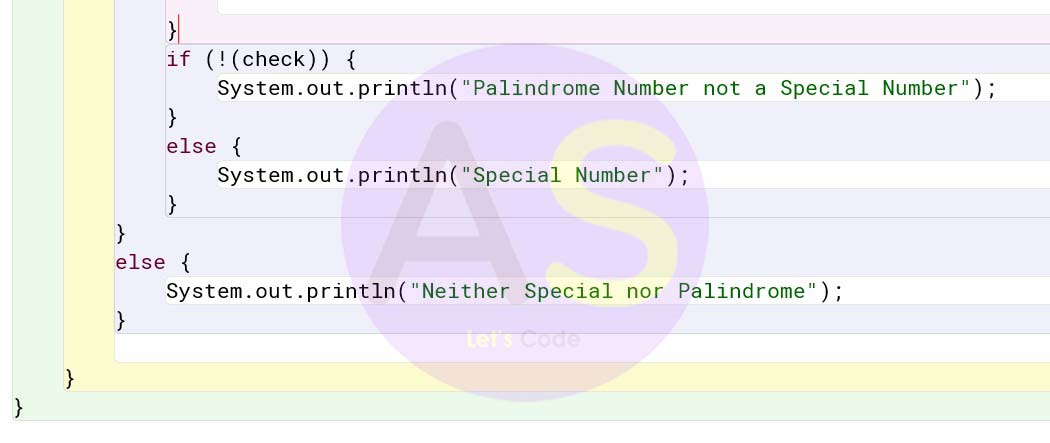
import java.util.*; public class SpecialNumber { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter a word: "); String s = sc.next(); s = s.toUpperCase(); int l = s.length(); if (s.charAt(0) == s.charAt(l - 1)) { boolean check = true; for (int i = 1; i < l / 2; i++) { if (s.charAt(i) != s.charAt(l - 1 - i)) { check = false; break; } } if (!(check)) { System.out.println("Palindrome Number not a Special Number"); } else { System.out.println("Special Number"); } } else { System.out.println("Neither Special nor Palindrome"); } } }
Question 7
Design n class to overload a function SumSeries as follows:
(i) void SumSeries(int n, double x) – with one integer argument and one double argument to find and display the sum of the series given below:
(ii) void SumSeries() – To find and display the sum of the following series:
s = 1 + (1 x 2) + (1 x 2 x 3) + ….. + (1 x 2 x 3 x 4 x 20)

Solution:
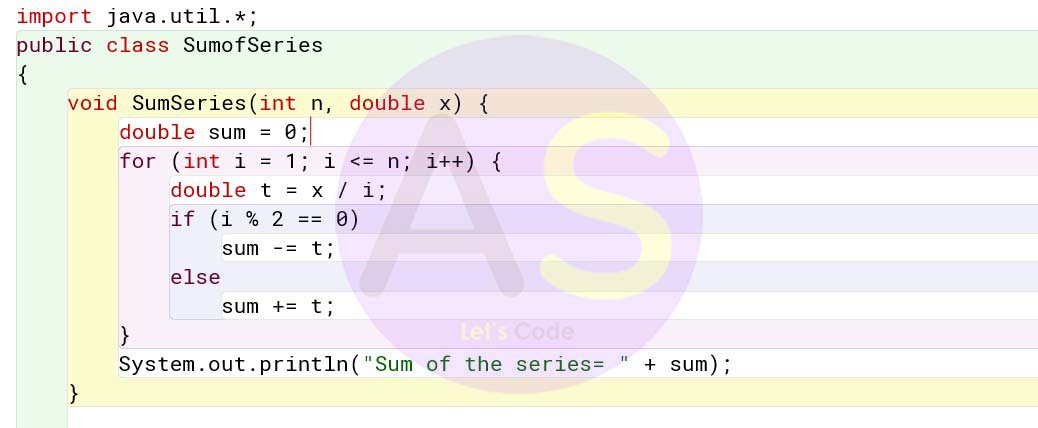
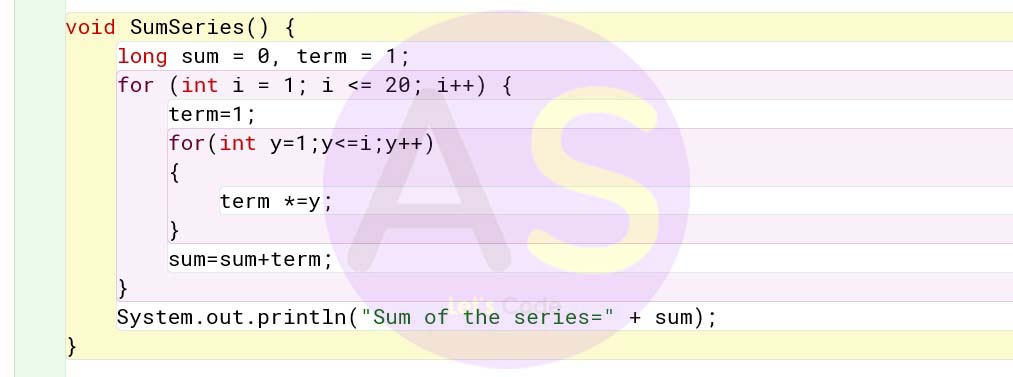
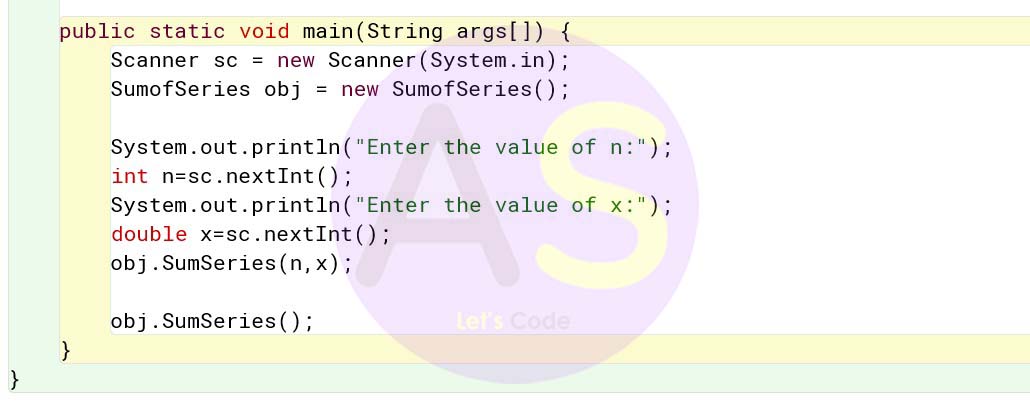
import java.util.*; public class SumofSeries { void SumSeries(int n, double x) { double sum = 0; for (int i = 1; i <= n; i++) { double t = x / i; if (i % 2 == 0) sum -= t; else sum += t; } System.out.println("Sum of the series= " + sum); } void SumSeries() { long sum = 0, term = 1; for (int i = 1; i <= 20; i++) { term=1; for(int y=1;y<=i;y++) { term *=y; } sum=sum+term; } System.out.println("Sum of the series=" + sum); } public static void main(String args[]) { Scanner sc = new Scanner(System.in); SumofSeries obj = new SumofSeries(); System.out.println("Enter the value of n:"); int n=sc.nextInt(); System.out.println("Enter the value of x:"); double x=sc.nextInt(); obj.SumSeries(n,x); obj.SumSeries(); } }
Question 8
Write a program to accept a number and check and display whether it is a Niven number or not.
(Niven number is that number which is divisible by its sum of digits).
Example:
Consider the number 126.
Sum of its digits is 1+2+6 = 9 and 126 is divisible by 9.
Solution:
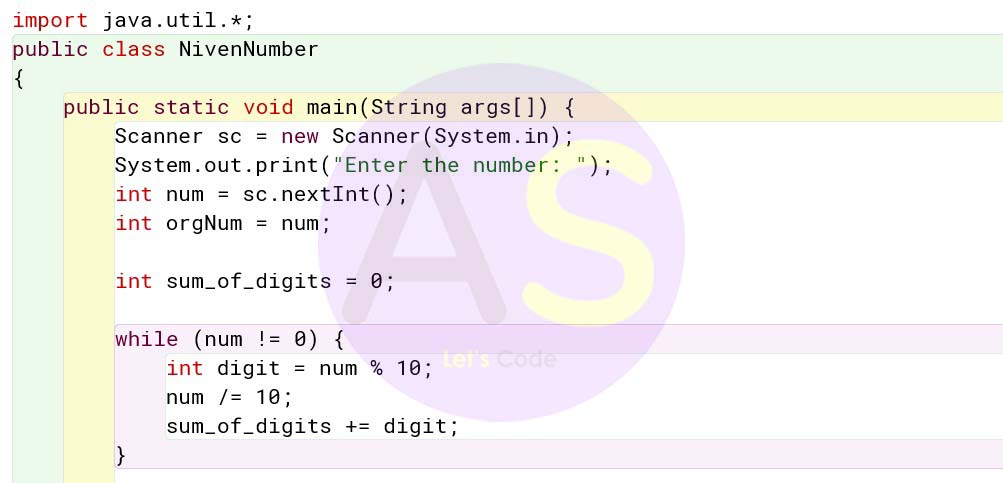

import java.util.*; public class NivenNumber { public static void main(String args[]) { Scanner sc = new Scanner(System.in); System.out.print("Enter the number: "); int num = sc.nextInt(); int orgNum = num; int sum_of_digits = 0; while (num != 0) { int digit = num % 10; num /= 10; sum_of_digits += digit; } if (orgNum % sum_of_digits == 0) System.out.println(orgNum + " is a Niven number"); else System.out.println(orgNum + " is not a Niven number"); } }
Question 9
Write a program to initialize the seven Wonders of the World along with their locations in two different arrays. Search for a name of the country input by the user. If found, display the name of the country along with its Wonder, otherwise display “Sorry Not Found!”.
Seven wonders — CHICHEN ITZA, CHRIST THE REDEEMER, TAJMAHAL, GREAT WALL OF CHINA, MACHU PICCHU, PETRA, COLOSSEUM
Locations — MEXICO, BRAZIL, INDIA, CHINA, PERU, JORDAN, ITALY
Example — Country Name: INDIA Output: INDIA-TAJMAHAL
Country Name: USA Output: Sorry Not Found!
Solution:
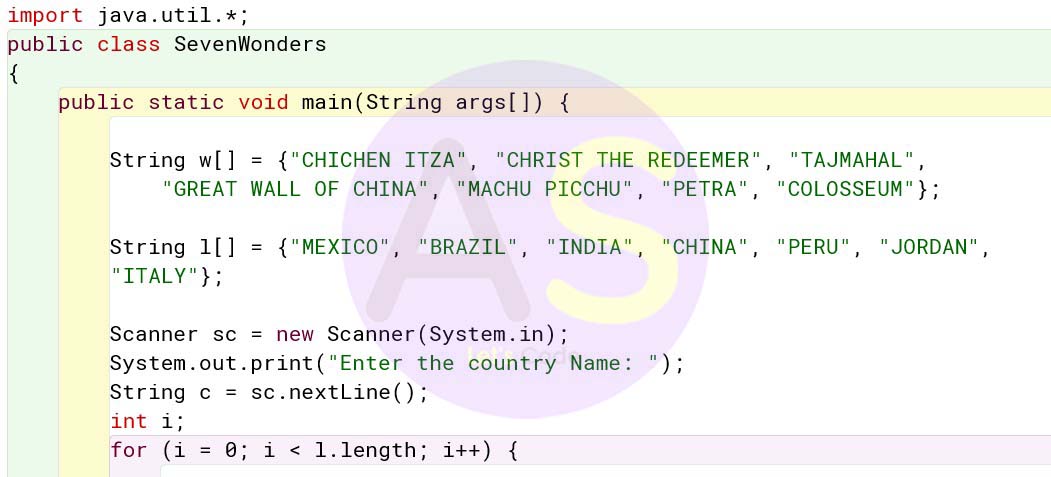
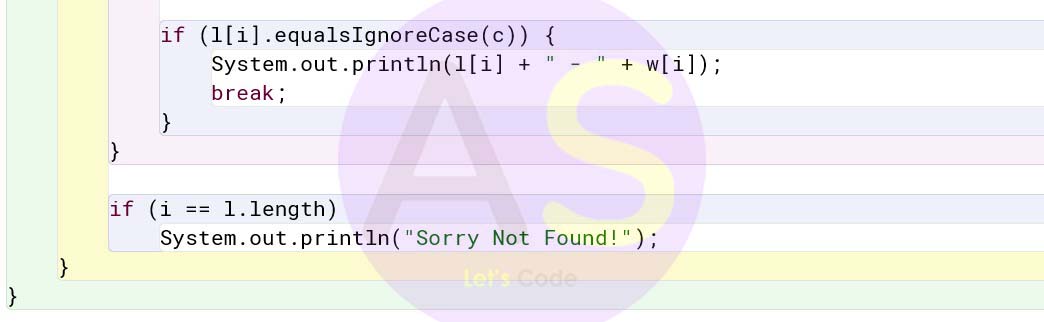
import java.util.*; public class SevenWonders { public static void main(String args[]) { String w[] = {"CHICHEN ITZA", "CHRIST THE REDEEMER", "TAJMAHAL", "GREAT WALL OF CHINA", "MACHU PICCHU", "PETRA", "COLOSSEUM"}; String l[] = {"MEXICO", "BRAZIL", "INDIA", "CHINA", "PERU", "JORDAN", "ITALY"}; Scanner sc = new Scanner(System.in); System.out.print("Enter the country Name: "); String c = sc.nextLine(); int i; for (i = 0; i < l.length; i++) { if (l[i].equalsIgnoreCase(c)) { System.out.println(l[i] + " - " + w[i]); break; } } if (i == l.length) System.out.println("Sorry Not Found!"); } }