Code is copied!
CLASS 10 ICSE Computer Application - Java Question Paper 2022
Maximum Marks:50
Time allowed: One and a half hours
Answers to this Paper must be written on the paper provided separately.
You will not be allowed to write during the first 10 minutes.
This time is to be spent in reading the question paper.
The time given at the head of this Paper is the time allowed for writing the answers.
This Paper is divided into two Sections.
Attempt all questions from Section A and any four questions from Section B.
Answers to this Paper must be written on the paper provided separately.
The intended marks for questions or parts of questions are given in brackets[] .
SECTION A
(Attempt all questions from this Section.)
Choose the correct answers to the questions from the given options. (Do not copy the question. Write the correct answer only.)
Question 1
(a)
Return data type of isLetter(char) is........... :
(a) Boolean
(c) bool
(b) boolean
(d) char
Solution
(a) Boolean
Explanation : The isLetter() function takes a
character as input and returns in Boolean True/
False whether, the argument received is a
character or not.
Explanation : The isLetter() function takes a character as input and returns in Boolean True/ False whether, the argument received is a character or not.
(ii)
Method that converts a character to uppercase is___.
(a) toUpper()
(b) ToUpperCase()
(c) toUppercase()
(d) toUpperCase(char)
Solution
(c) tOUppercase()
Explanation : The toUppercase() function takes a
character as input and returns the same converted
to uppercase.
Explanation : The toUppercase() function takes a character as input and returns the same converted to uppercase.
(iii)
Give the output of the following String methods:
"SUCESS" indexOf('S')+ "SUCESS".lastIndexOf('S’)
(a) 0
(c) 6
(b) 5
(d) -5
Solution
(b) 5
Explanation : "SUCESS".indexOf('S')+"SUCESS".lastIndexOf('S')
indexOf() returns the index of the first occurance
of 'S' and lastIndexOf(’S’) returns the index of last
occurance of ‘S’. Hence 0 + 5 =5
"SUCESS" indexOf('S')+ "SUCESS".lastIndexOf('S’)
(a) 0
(c) 6
(b) 5
(d) -5
(b) 5
Explanation : "SUCESS".indexOf('S')+"SUCESS".lastIndexOf('S')
indexOf() returns the index of the first occurance of 'S' and lastIndexOf(’S’) returns the index of last occurance of ‘S’. Hence 0 + 5 =5
Explanation : "SUCESS".indexOf('S')+"SUCESS".lastIndexOf('S')
indexOf() returns the index of the first occurance of 'S' and lastIndexOf(’S’) returns the index of last occurance of ‘S’. Hence 0 + 5 =5
(iv)
Corresponding wrapper class of float data type is
(a) FLOAT
(c) Float
(b) float
(d) Floating
Solution
(c) Float
Explanation : In java class names start with
Uppercase. The wrapper class for float data type
is Float
Explanation : In java class names start with Uppercase. The wrapper class for float data type is Float
(v)
.............class is used to convert a primitive data type to
its corresponding object.
(a) String
(c) System
(b) Wrapper
(d) Math
Solution
(b) Wrapper
Explanation : Every primitive data type has a corresponding wrapper class that converts the
data of primitive type to a class object.
Explanation : Every primitive data type has a corresponding wrapper class that converts the data of primitive type to a class object.
(vi)
Give the output of the following code:
System.out.printIn(“Good” concat(”Day”));
(a) GoodDay
(c) Goodday
(b) Good Day
(d) goodDay
Solution
(a) GoodDay
Explanation : The concat() function concatenates a
String with another string, hence “Good”.concat(“Day”)
returns GoodDay.
(vii)
A single dimensional array contains N elements. What,
will be the last subscript?
(a) N
(c) N-2
(b) N-1
(d) N+1
Solution
(b) N-1
Explanation : The indexes of an array of N
elements start from 0 to N-1. Hence the index of
the last element will be N-1
(viii)
The access modifier that gives least accessibility is:
(a) private
(c) protected
(b) public
(d) package
Solution
(a) Private
Explanation : Private members of a class are
accessible only within the class and nowhere else.
Hence providing the least accessibility.
(ix)
Give the output of the following code:
String A ="56.0", B="94.0";
double C= Double .parseDouble(A);
double D = Double .parseDouble(B);
System.out.println((C+D));
(a) 100
(c) 100.0
(b) 150.0
(d) 150
Solution
(b) 150.0
Explanation : Double.parseDouble() converts
a string to double . hence the strings “56.0” and
“94.0” are converted to 56.0 and 94.0 , which when
added give 150.0
(x)
What will be the output of the following code?
System.out.println("Lucknow" .substring(0,4));
(a) Lucknow
(c) Luck
(b) Luckn
(d) luck
Solution
(c) Luck
Explanation : The substring function returns a
part of a string from start index to end index - 1.
“Lucknow” substring(0,4) returns characters
from 0 to 3 indexes . Hence output is Luck.
SECTION B
(Attempt any four questions.)
Question 2
Define a class to perform binary search on a list of integers given below, to search for an element input by the user, if it is found display the element along with
is position, otherwise display the message “Search element not found”.
2,5,7, 10, 15, 20, 29, 30, 46, 50
Solution:
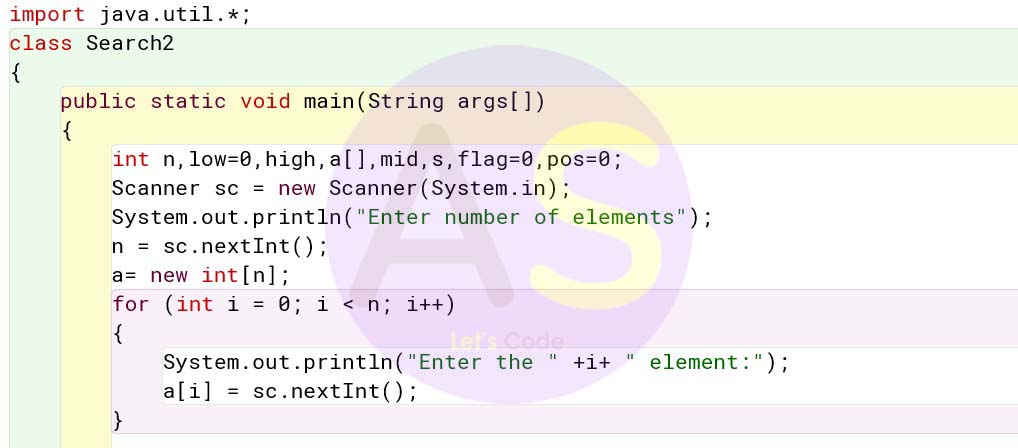
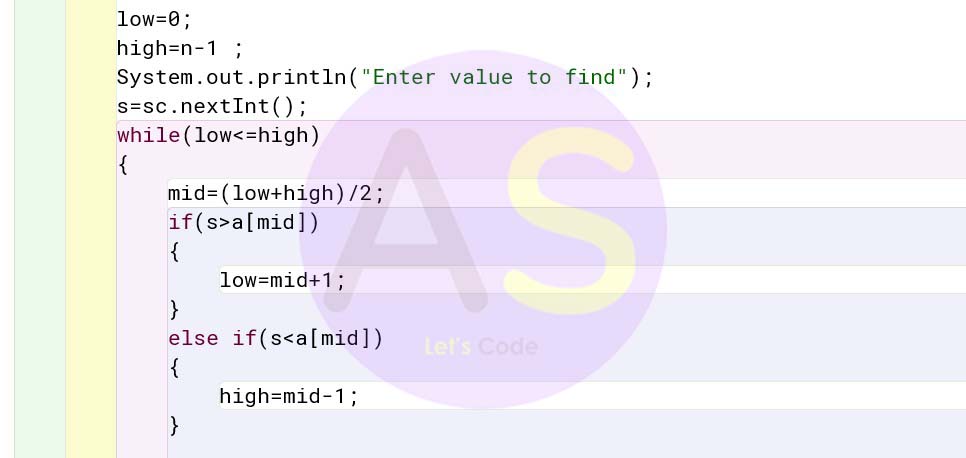
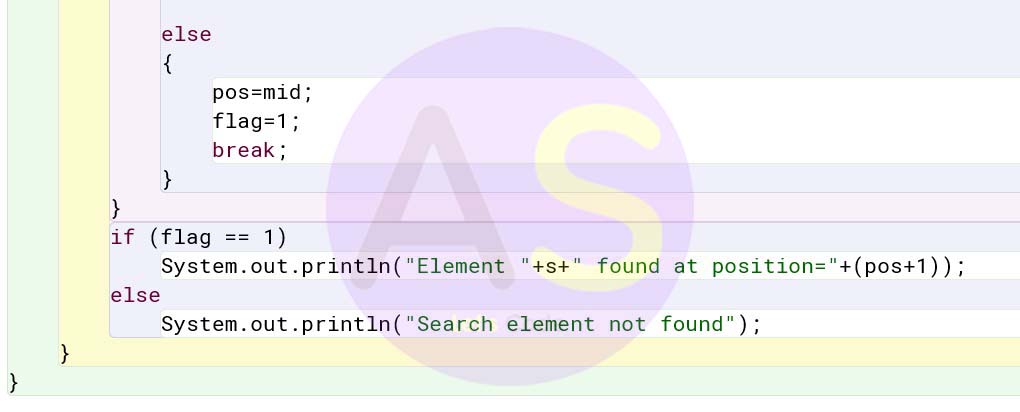
import java.util.*; class Search2 { public static void main(String args[]) { int n,low=0,high,a[],mid,s,flag=0,pos=0; Scanner sc = new Scanner(System.in); System.out.println("Enter number of elements"); n = sc.nextInt(); a= new int[n]; for (int i = 0; i < n; i++) { System.out.println("Enter the " +i+ " element:"); a[i] = sc.nextInt(); } low=0; high=n-1 ; System.out.println("Enter value to find"); s=sc.nextInt(); while(low<=high) { mid=(low+high)/2; if(s>a[mid]) { low=mid+1; } else if(s< a[mid]) { high=mid-1; } else { pos=mid; flag=1; break; } } if (flag == 1) System.out.println("Element "+s+" found at position="+(pos+1)); else System.out.println("Search element not found"); } }
Question 3
Define a class to declare a character array of size ten,accept the characters into the array and display the
characters with highest and lowest ASCII (America Standard Code for Information Interchange) value.
EXAMPLE:
INPUT:
‘R’, 'z', ‘q’, 'A’, 'N’, ‘p’, ‘m', ‘U’, ‘Q’, 'F’
OUTPUT:
Character with highest ASCII value =z
Character with lowest ASCII value =A
Solution:
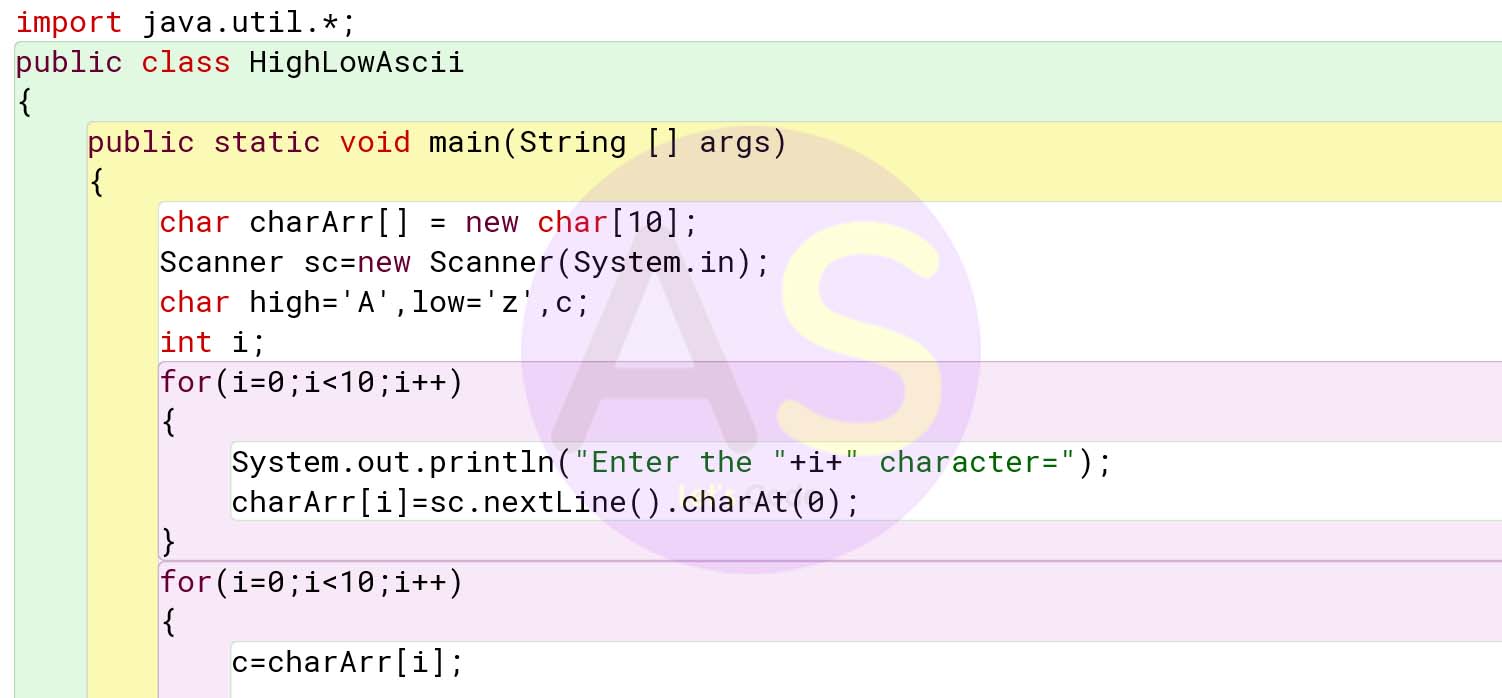
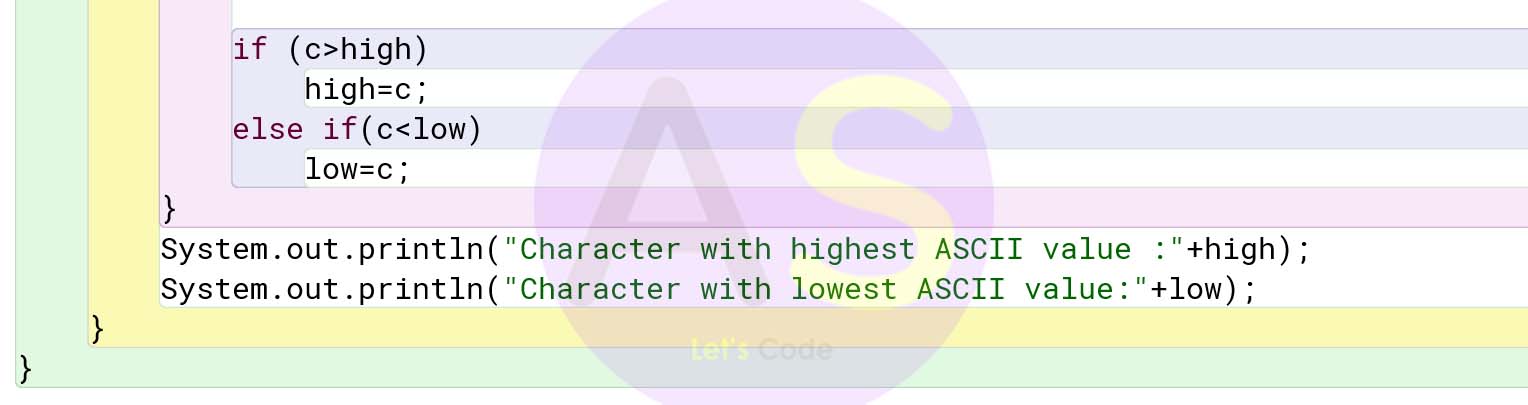
import java.util.*; public class HighLowAscii { public static void main(String [] args) { char charArr[] = new char[10]; Scanner sc=new Scanner(System.in); char high='A',low='z',c; int i; for(i=0;i< 10;i++) { System.out.println("Enter the "+i+" character="); charArr[i]=sc.nextLine().charAt(0); } for(i=0;i< 10;i++) { c=charArr[i]; if (c>high) high=c; else if(c< low) low=c; } System.out.println("Character with highest ASCII value :"+high); System.out.println("Character with lowest ASCII value:"+low); } }
Question 4
Define a class to declare an array of size twenty of double datatype, accept the elements into the array and perform the following:
- Calculate and print the product of all the elements.
- print the square of each element of the array.
Solution:
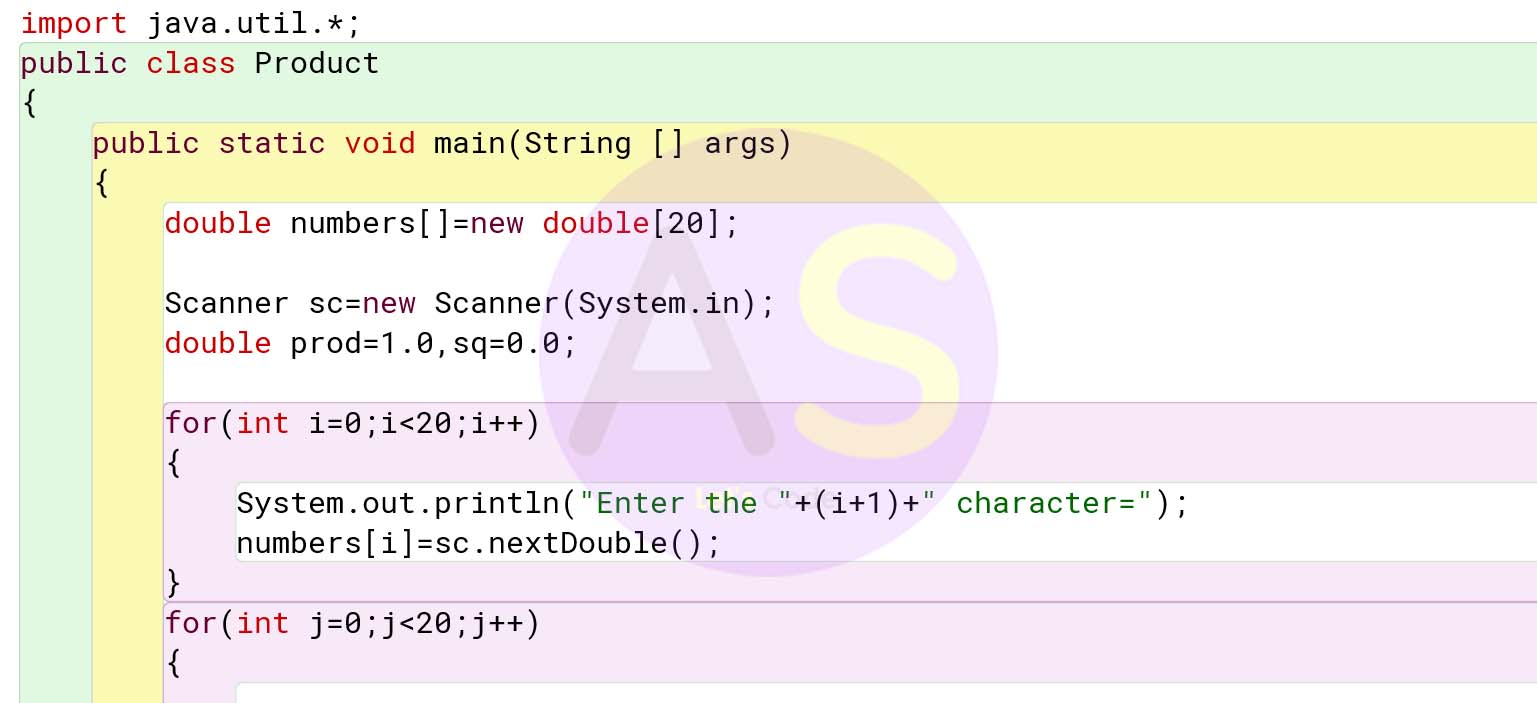
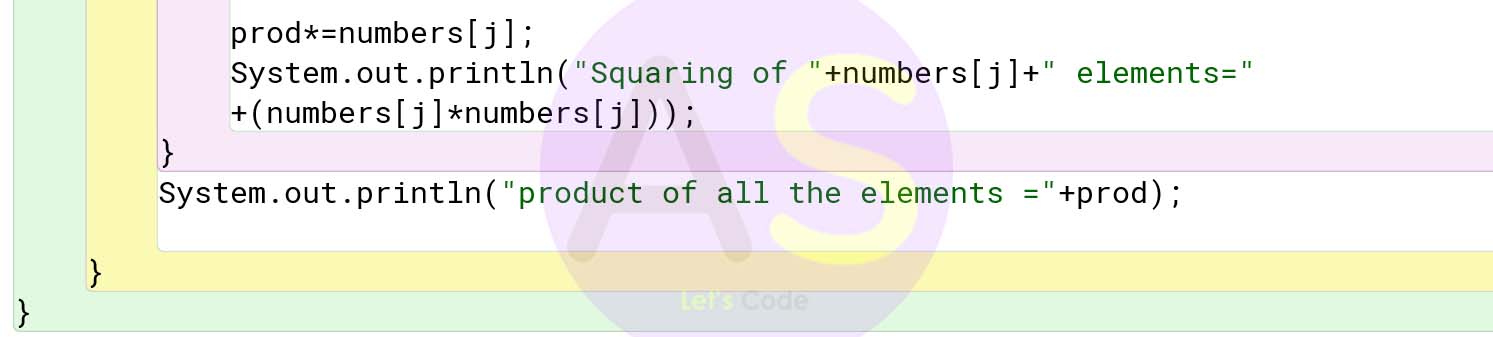
import java.util.*; public class Product { public static void main(String [] args) { double numbers[]=new double[20]; Scanner sc=new Scanner(System.in); double prod=1.0,sq=0.0; for(int i=0;i< 20;i++) { System.out.println("Enter the "+(i+1)+" character="); numbers[i]=sc.nextDouble(); } for(int j=0;j< 20;j++) { prod*=numbers[j]; System.out.println("Squaring of "+numbers[j]+" elements="+(numbers[j]*numbers[j])); } System.out.println("product of all the elements ="+prod); } }
Question 5
Define a class to accept a string, and print the characters with the uppercase and lowercase reversed, but all the other characters should remain the same as before.
EXAMPLE: INPUT : WelCoMe_2022
OUTPUT : wELcOmE_2022
Solution:
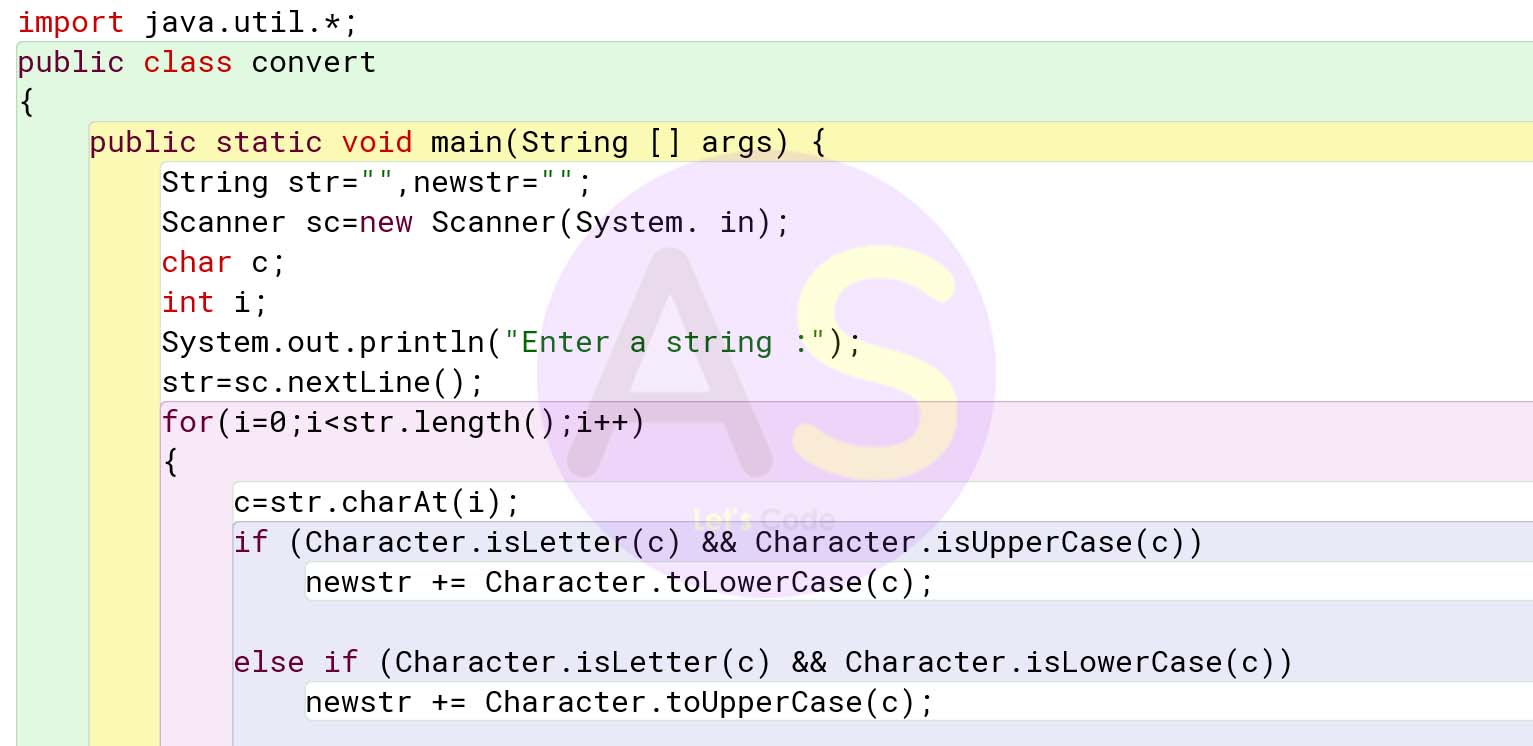

import java.util.*; public class Words { public static void main(String [] args) { String s[]=new String[10]; Scanner sc= new Scanner(System.in); for(int i=0;i< 10;i++) { System.out.println("Enter the "+(i+1)+" word:"); s[i]=sc.next(); s[i]=s[i].toUpperCase(); } char cb,ce; for(int i=0;i< s.length;i++) { cb=s[i].charAt(0); ce=s[i].charAt(s[i].length()-1); if((cb=='A') && (ce =='A')) System.out.println(s[i]); } } }
Question 6
Define a class to declare an array to accept and storeten words. Display only those words which begin with
the letter ‘A’ or ‘a’ and also end with the letter 'A' or ‘a’.
Example:
Input: Hari, Anita, Akash, Amrita, Alina, Devi, Rishab, John, Farha, AMITHA
Output: Anita
Amrita
Alina
AMITHA
Solution:
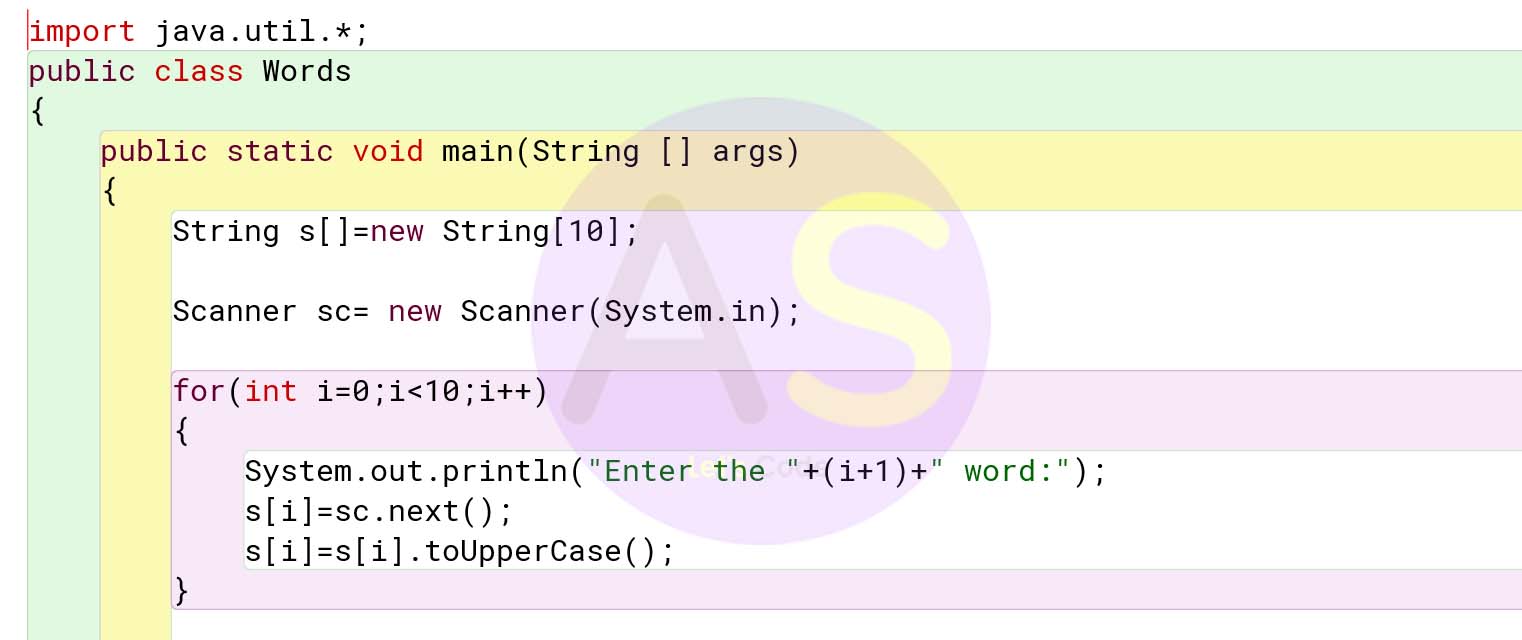
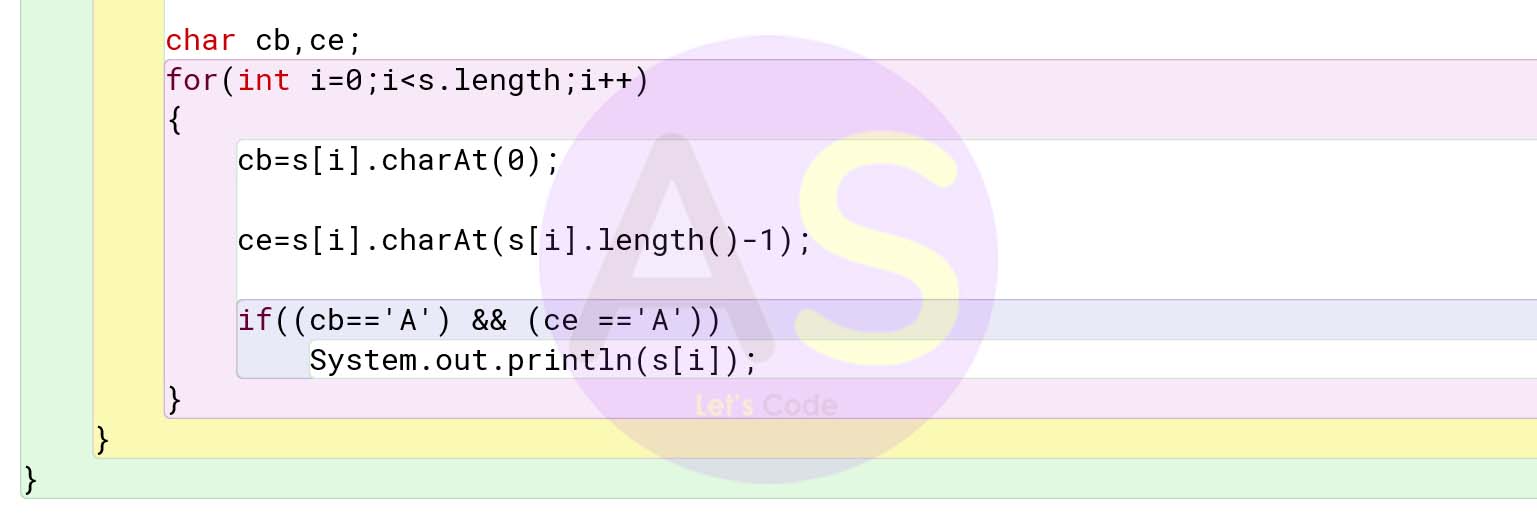
import java.util.*; public class Words { public static void main(String [] args) { String s[]=new String[10]; Scanner sc= new Scanner(System.in); for(int i=0;i< 10;i++) { System.out.println("Enter the "+(i+1)+" word:"); s[i]=sc.next(); s[i]=s[i].toUpperCase(); } char cb,ce; for(int i=0;i< s.length;i++) { cb=s[i].charAt(0); ce=s[i].charAt(s[i].length()-1); if((cb=='A') && (ce =='A')) System.out.println(s[i]); } } }
Question 7
Define a class to accept two strings of same length and form a new word in such a way that, the first character
of the first word is followed by the first character of the second word and so on.
Example:
Input string 1 - BALL
Input string 2 - WORD
Output : BNWAOLRLD
Solution:
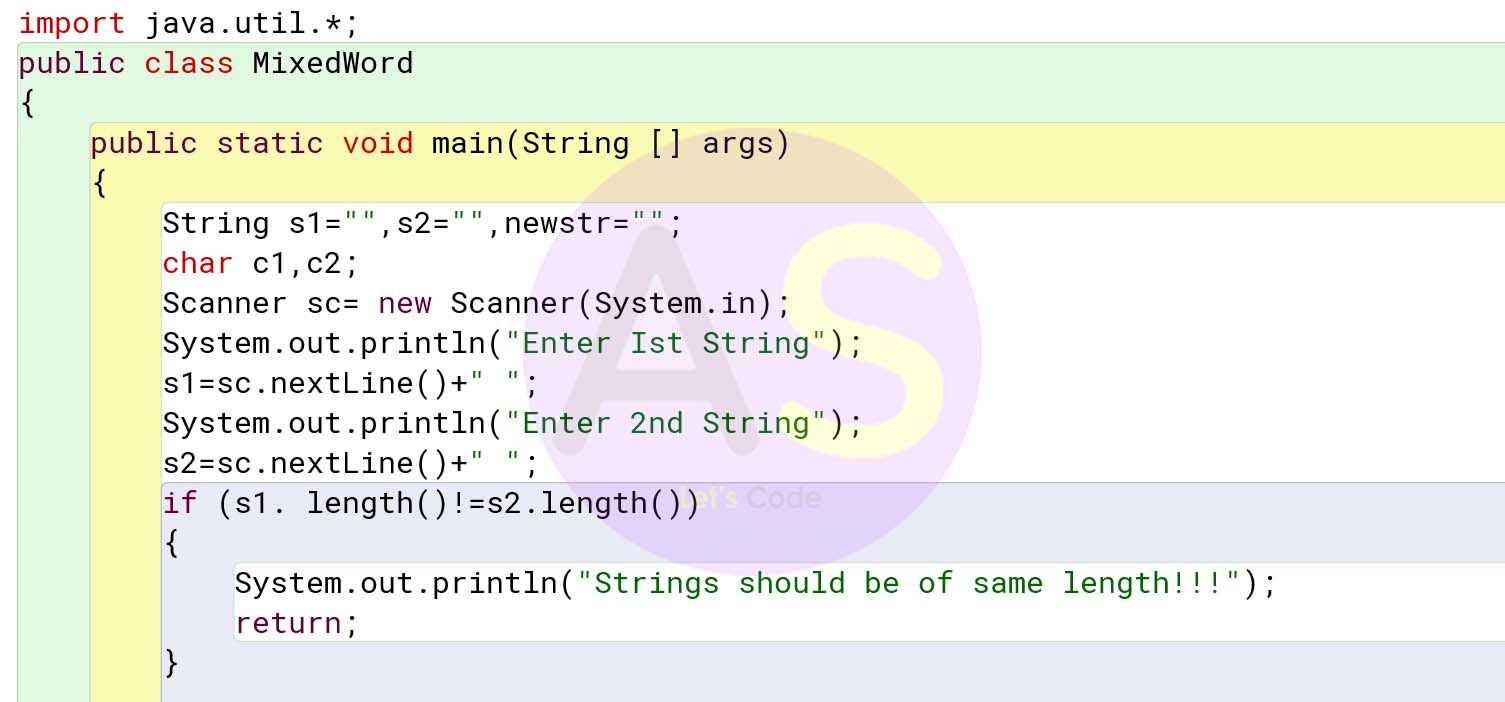
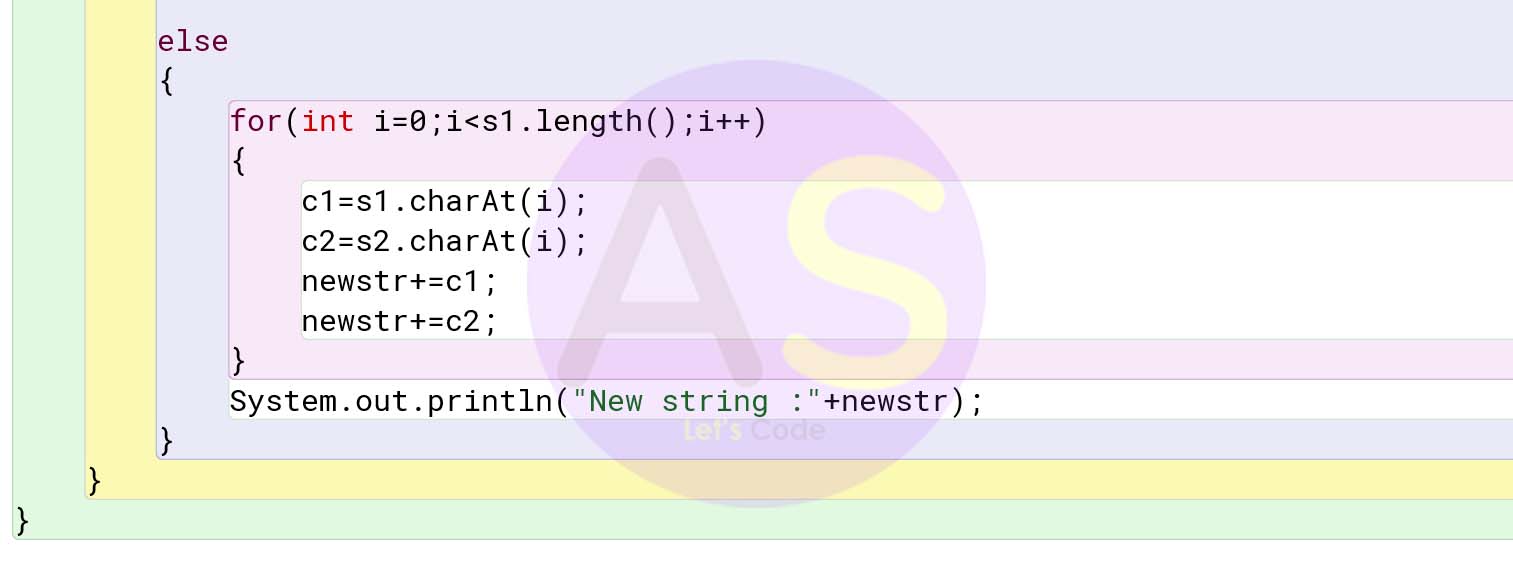
import java.util.*; public class MixedWord { public static void main(String [] args) { String s1="",s2="",newstr=""; char c1,c2; Scanner sc= new Scanner(System.in); System.out.println("Enter Ist String"); s1=sc.nextLine()+" "; System.out.println("Enter 2nd String"); s2=sc.nextLine()+" "; if (s1. length()!=s2.length()) { System.out.println("Strings should be of same length!!!"); return; } else { for(int i=0;i< s1.length();i++) { c1=s1.charAt(i); c2=s2.charAt(i); newstr+=c1; newstr+=c2; } System.out.println("New string :"+newstr); } } }