Code is copied!
CLASS 10 ICSE Computer Application - Java Question Paper 2020
Maximum Marks:100
Time allowed: Two hours
Answers to this Paper must be written on the paper provided separately.
You will not be allowed to write during the first 15 minutes.
This time is to be spent in reading the question paper.
The time given at the head of this Paper is the time allowed for writing the answers.
This Paper is divided into two Sections.
Attempt all questions from Section A and any four questions from Section B.
Answers to this Paper must be written on the paper provided separately.
The intended marks for questions or parts of questions are given in brackets[] .
SECTION A
(Attempt all questions from this Section.)
Question 1
(a)
Define Java byte code.
Solution
It is a machine instruction for java Processor chip
called JVM.
(b)
Write a difference between class and an object.
Solution
Class is a set of objects that shares common
characteristics and behaviour whereas object is an
instance of a class.
(c)
Name the following:
(i) The keyword which converts variable into constant.
(ii) The method which terminates the entire program from any stage.
Solution
(i) final
(ii) System.exit(0)
(ii) System.exit(0)
(d)
Which of the following are primitive data types?
(i) double
(ii) String
(iii) char
(iv) Integer
Solution
(i) double , (iii) char are primitive datatypes.
(e)
What is an operator? Name any two types of operators used in Java.
Solution
Operator is a symbol which specifies the type of
operation to be performed on the operands.
Ex: Arithmetic Operator (+,-,*,/,%) or Relational
operator( >,<,>=,<=, ==, !=) etc.
Ex: Arithmetic Operator (+,-,*,/,%) or Relational operator( >,<,>=,<=, ==, !=) etc.
Question 2
(a) What is autoboxing in Java? Give an example.
Solution
Converting primitive types to corresponding wrapper class object is called Autoboxing. For eg: int to Integer, double to Double etc.
(b) State the difference between length and length() in Java.
Solution
Length is a variable used with arrays to find
its size whereas length() is a function used with
Strings to determine the no. of characters present
in it.
If arr[] = {1,2,3,4,5} then arr.length will return 5.
If s="JAVA” the s.length() will return 4.
(c) What is constructor overloading?
Solution
Defining more than one constructors having same
name but different signature is called constructor
overloading.
class Overload
{
String s;
public Overload()
{
s="DPC Jhansi”;
}
public Overload( String s1)
{
s=s1;
}
}
(d) What is the use of import statement in Java?
Solution
Import keyword is used to include predefined
classes and functions in our program which are
available in java.
import java.util.*; will include all the classes of
util package in our program.
(e) What is an infinite loop? Give an example.
Solution
Non terminating loop whose test condition is
always true is called infinite loop.
for(i=1 ; i> 0; i++)
{
System.out.println(“This is Infinite Loop”);
}
Question 3
(a)
Write a Java expression for the following:
√(b2 - 4ac)
Solution
Math.sqrt( b*b - 4*a*c)
(b)
Evaluate the following if the value of x=7, y=5
x+=x++ + x + ++y
Solution
x=28,y=6
(c)
Write the output for the following:
String s1 = ''Life is Beautiful'';
System.out.println (''Earth'' + s1.substring(4));
System.out.println( s1.endsWith(''L'') );
Solution
Earth is Beautiful
false
(d)
Write the output of the following statement:
System.out.println(''A picture is worth \t \''A thousand words.\'' '');
Solution
A picture is worth “A thousand words.”
(e)
Give the output of the following program segment and mention how many times
the loop will execute:
int k;
for ( k = 5 ; k < = 20 ; k + = 7 )
if ( k% 6==0 )
continue;
System.out.println ( k );
Solution
Loop will execute 3 times output 26.
(f)
What is the data type returned by the following library methods?
(i) isWhitespace()
(ii) compareToIgnoreCase()
Solution
(i) boolean
(ii) int
(g)
Rewrite the following program segment using logical operators:
if ( x > 5 )
if ( x > y )
System.out.println (x+y);
Solution
if(x>5&&x>y)
System.out.println( x + y ) ;
(h)
Convert the following if else if construct into switch case:
if (ch== 'c' || ch=='C')
System.out.print("COMPUTER");
else if (ch== 'h' || ch=='H')
System.out.print("HINDI");
else
System.out . print("PHYSICAL EDUCATION");
Solution
switch(ch)
{
case ‘c’:
case ‘C’: System.out.print("COMPUTER");
break;
case ‘h’:
case ‘H’ : System.out.print("HINDI");
break;
default: System.out.print
("PHYSICAL EDUCATION");
}
(i)
Give the output of the following:
(i) Math.pow (36,0.5) + Math.cbrt (125)
(ii) Math.ceil (4.2 ) + Math.floor (7.9)
Solution
(i) 6.0 + 5.0 = 11.0
(ii) 5.0 + 7.0 = 12.0
(j)
Rewrite the following using ternary operator:
if(n1>n2)
r = true;
else
r = false;
Solution
r=nl>n2? true : false;
SECTION B
(Attempt any four questions.)
Question 4
A private Cab service company provides service within the city at the following rates:
Design a class CabService with the Following description:
Member or variables / data members:
String Car-type: To store the type of car (AC or NON AC)
double km - To store the kilometer travelled
double bill - To calculate and store the bill amount
Member methods :
CabService() - Default constructor to initialize data members.
String data members to "" and double data members to 0.0.
void accept () - To accept car_type and km (using Scanner class
only).
void calculate () - To calculate the bill as per the rules given above.
void display() - To display the bill as per the following format
CAR TYPE:
KILOMETER TRAVELLED:
TOTAL BILL:
Create an object of the class in the main method and invoke the member methods.

Solution:
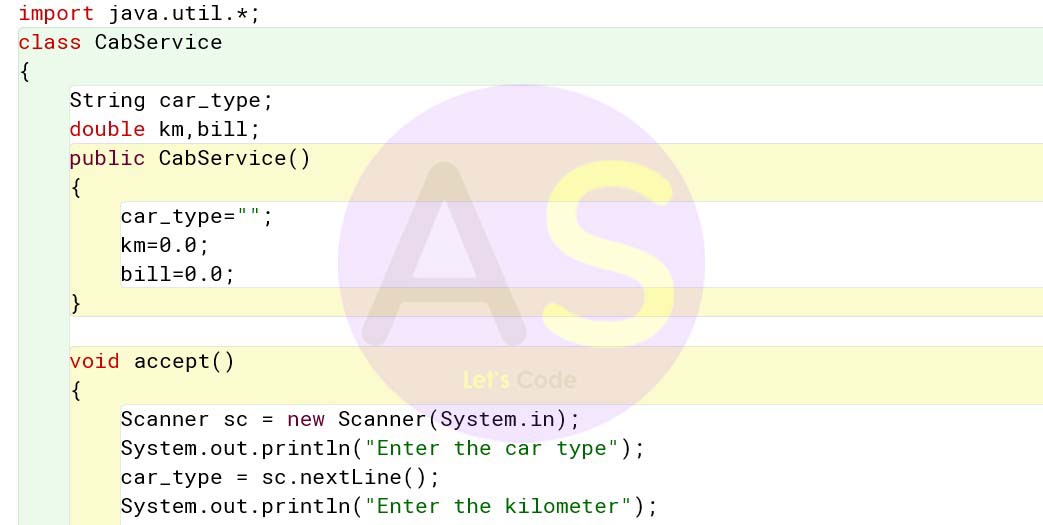
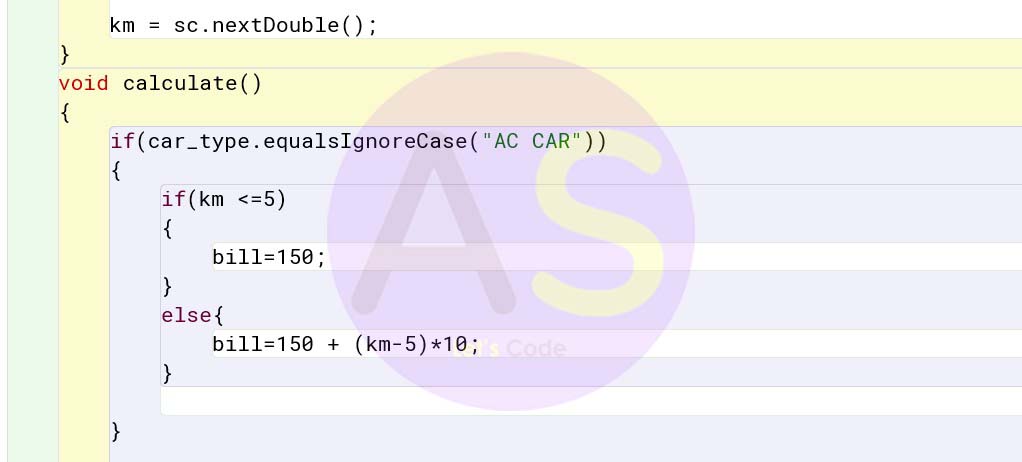
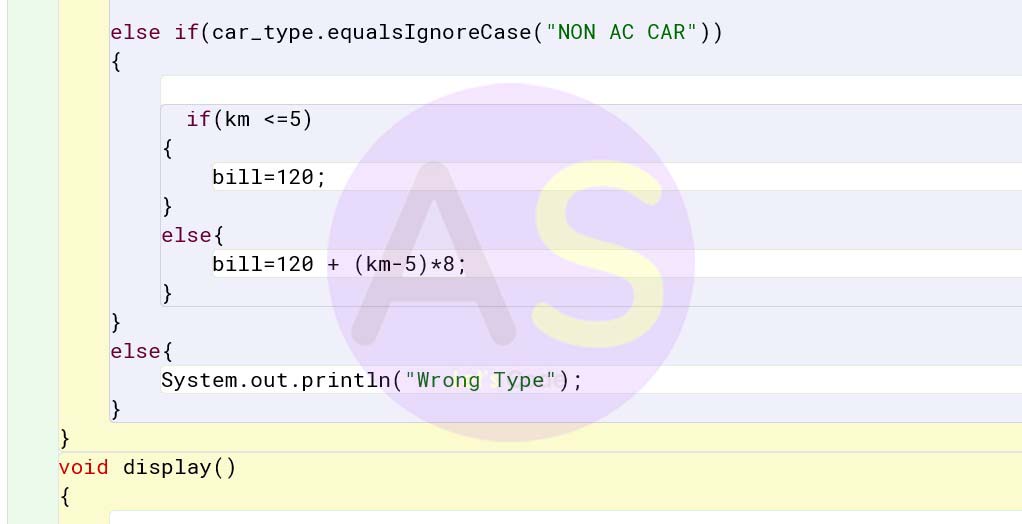
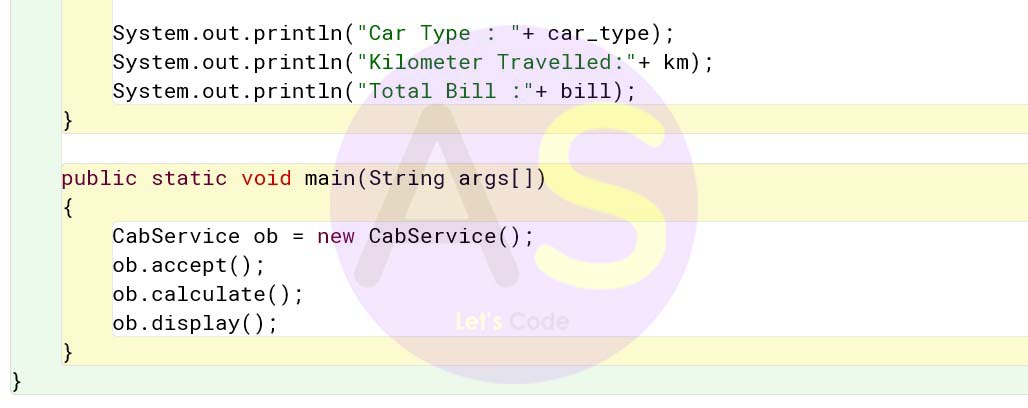
import java.util.*; class CabService { String car_type; double km,bill; public CabService() { car_type=""; km=0.0; bill=0.0; } void accept() { Scanner sc = new Scanner(System.in); System.out.println("Enter the car type"); car_type = sc.nextLine(); System.out.println("Enter the kilometer"); km = sc.nextDouble(); } void calculate() { if(car_type.equalsIgnoreCase("AC CAR")) { if(km <=5) { bill=150; } else{ bill=150 + (km-5)*10; } } else if(car_type.equalsIgnoreCase("NON AC CAR")) { if(km <=5) { bill=120; } else{ bill=120 + (km-5)*8; } } else{ System.out.println("Wrong Type"); } } void display() { System.out.println("Car Type : "+ car_type); System.out.println("Kilometer Travelled:"+ km); System.out.println("Total Bill :"+ bill); } public static void main(String args[]) { CabService ob = new CabService(); ob.accept(); ob.calculate(); ob.display(); } }
Question 5
Write a program to search for an integer value input by the user in the sorted list given below using binary search technique. If found display "Search Successful" and print the element, otherwise display "Search Unsuccessful"
Solution:
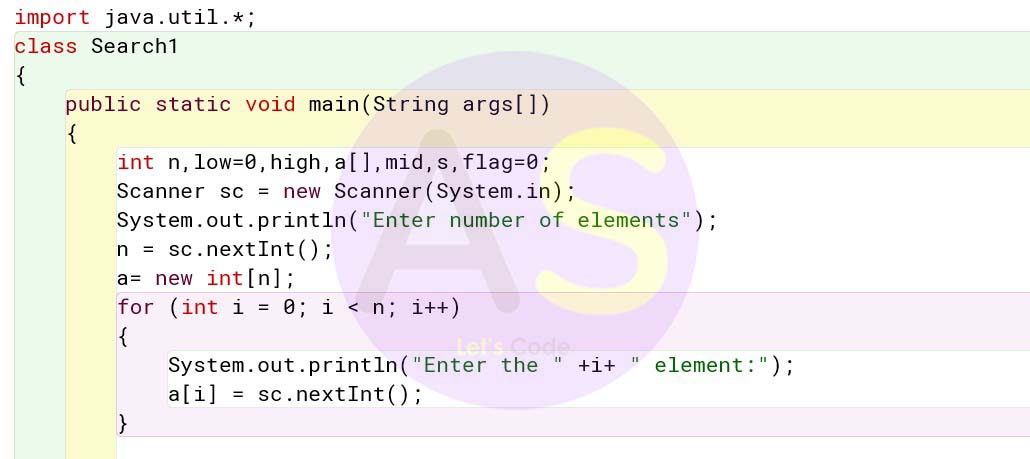
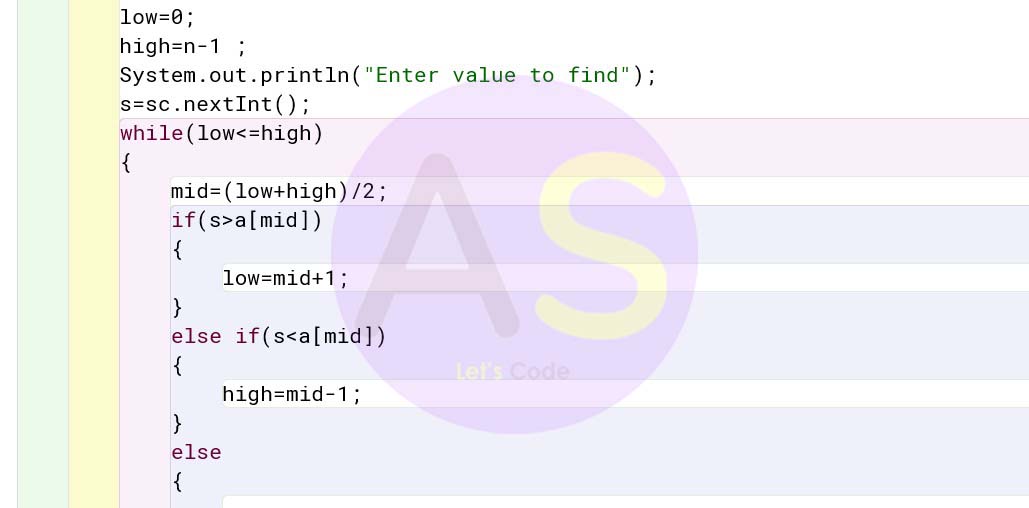
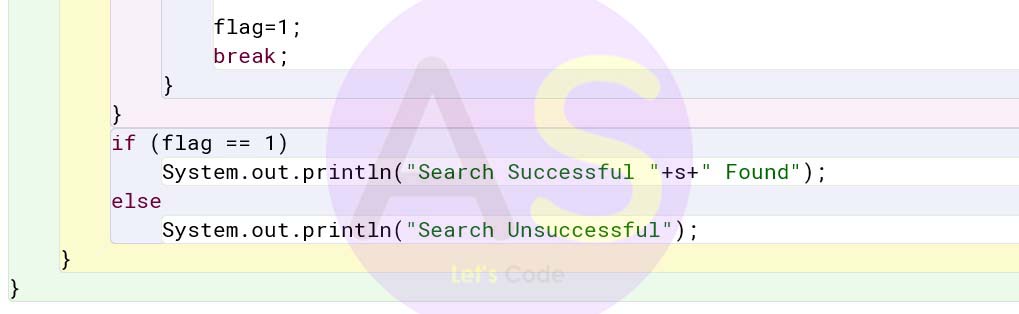
import java.util.*; class Search1 { public static void main(String args[]) { int n,low=0,high,a[],mid,s,flag=0; Scanner sc = new Scanner(System.in); System.out.println("Enter number of elements"); n = sc.nextInt(); a= new int[n]; for (int i = 0; i < n; i++) { System.out.println("Enter the " +i+ " element:"); a[i] = sc.nextInt(); } low=0; high=n-1 ; System.out.println("Enter value to find"); s=sc.nextInt(); while(low<=high) { mid=(low+high)/2; if(s>a[mid]) { low=mid+1; } else if(s< a[mid]) { high=mid-1; } else { flag=1; break; } } if (flag == 1) System.out.println("Search Successful "+s+" Found"); else System.out.println("Search Unsuccessful"); } }
Question 6
Write a program to input a sentence and convert it into uppercase and display each word in a separate line.
Example: Input : India is my country
Output : INDIA
IS
MY
COUNTRY
Solution:
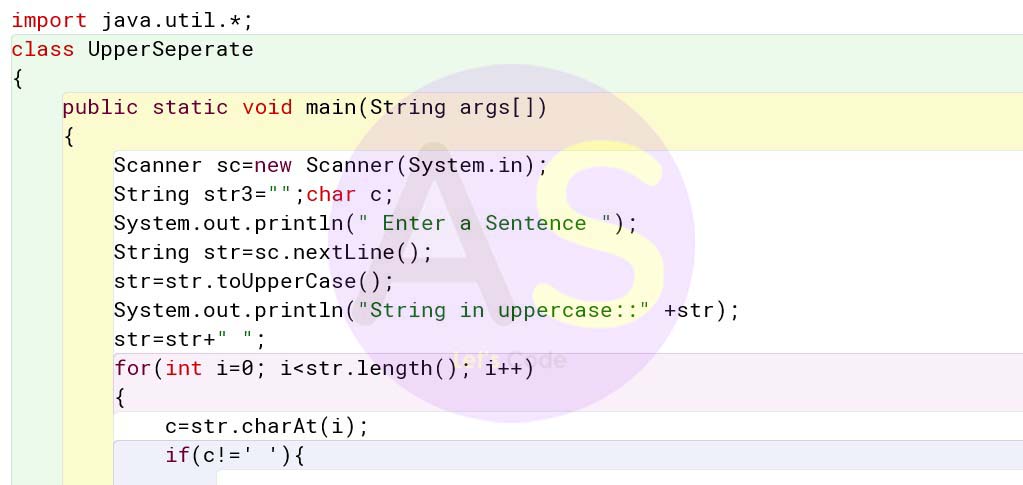
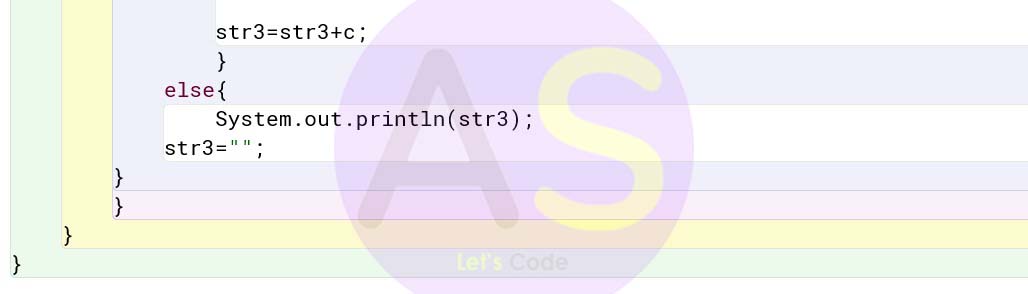
import java.util.*; class UpperSeperate { public static void main(String args[]) { Scanner sc=new Scanner(System.in); String str3="";char c; System.out.println(" Enter a Sentence "); String str=sc.nextLine(); str=str.toUpperCase(); System.out.println("String in uppercase::" +str); str=str+" "; for(int i=0; i< str.length(); i++) { c=str.charAt(i); if(c!=' '){ str3=str3+c; } else{ System.out.println(str3); str3=""; } } } }
Question 7
Design a class to overload a method Number( ) as follows:
(i) void Number (int num , int d) - To count and display the frequency of a digit in a number.
Example:
num = 2565685
d = 5
Frequency of digit 5 = 3
(ii) void Number (int n1) - To find and display the sum of even digits of a number.
Example:
n1 = 29865
Sum of even digits = 16
Write a main method to create an object and invoke the above methods
Solution:
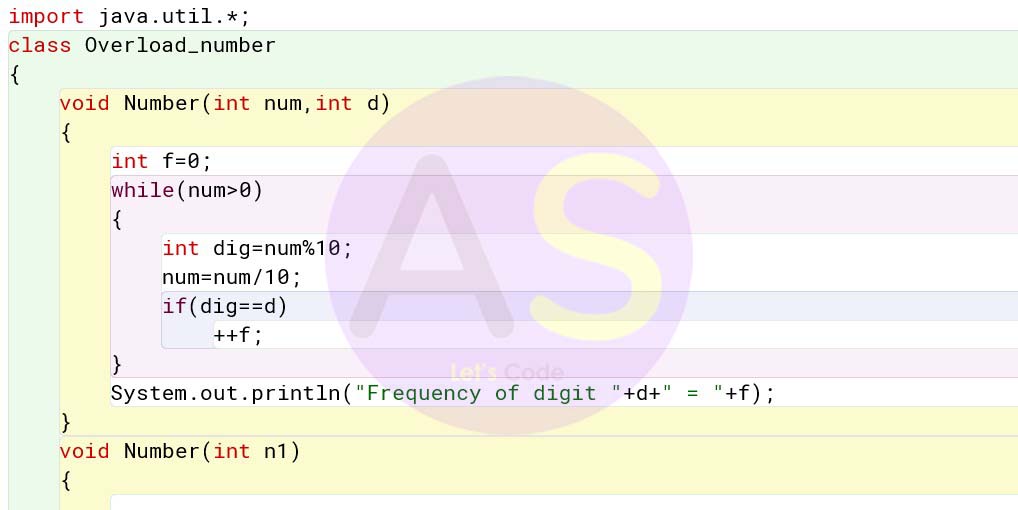
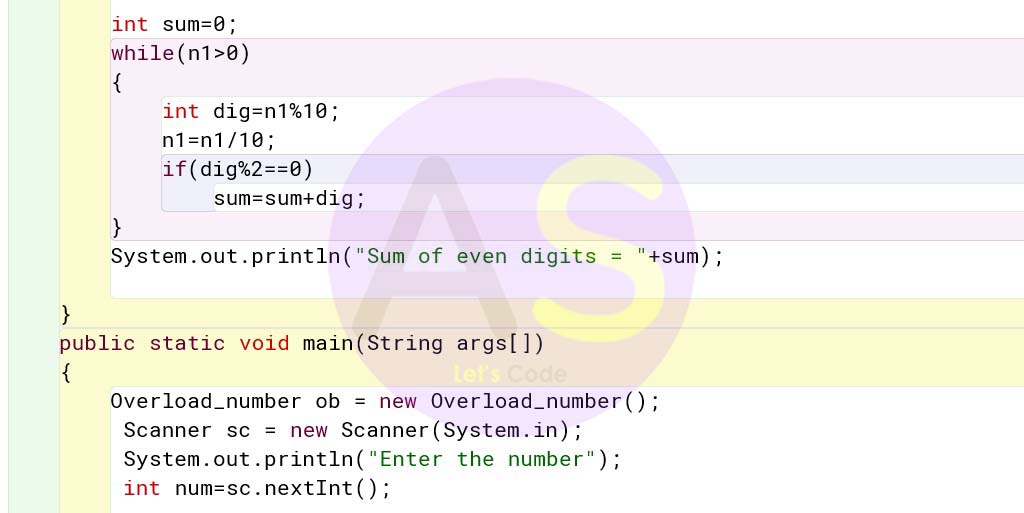
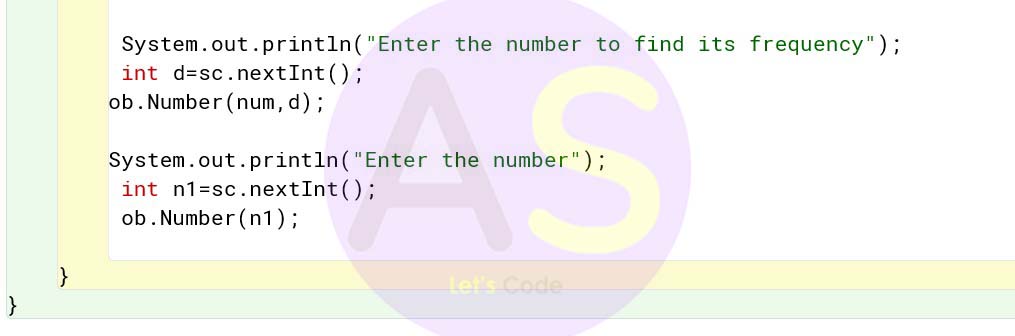
import java.util.*; class Overload_number { void Number(int num,int d) { int f=0; while(num>0) { int dig=num%10; num=num/10; if(dig==d) ++f; } System.out.println("Frequency of digit "+d+" = "+f); } void Number(int n1) { int sum=0; while(n1>0) { int dig=n1%10; n1=n1/10; if(dig%2==0) sum=sum+dig; } System.out.println("Sum of even digits = "+sum); } public static void main(String args[]) { Overload_number ob = new Overload_number(); Scanner sc = new Scanner(System.in); System.out.println("Enter the number"); int num=sc.nextInt(); System.out.println("Enter the number to find its frequency"); int d=sc.nextInt(); ob.Number(num,d); System.out.println("Enter the number"); int n1=sc.nextInt(); ob.Number(n1); } }
Question 8
Write a menu driven program to perform the following operations as per user’s choice:
(i) To print the value of c=a2 +2ab, where a varies from 1.0 to 20.0 with increment
of 2.0 and b=3.0 is a constant.
(ii) To display the following pattern using for loop:
A
AB
ABC
ABCD
ABCDE
Display proper message for an invalid choice.
Solution:
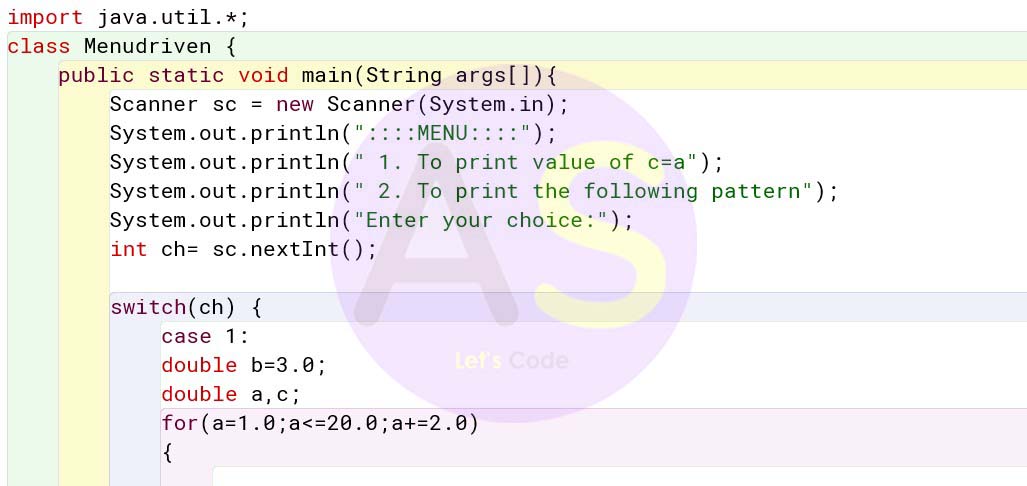
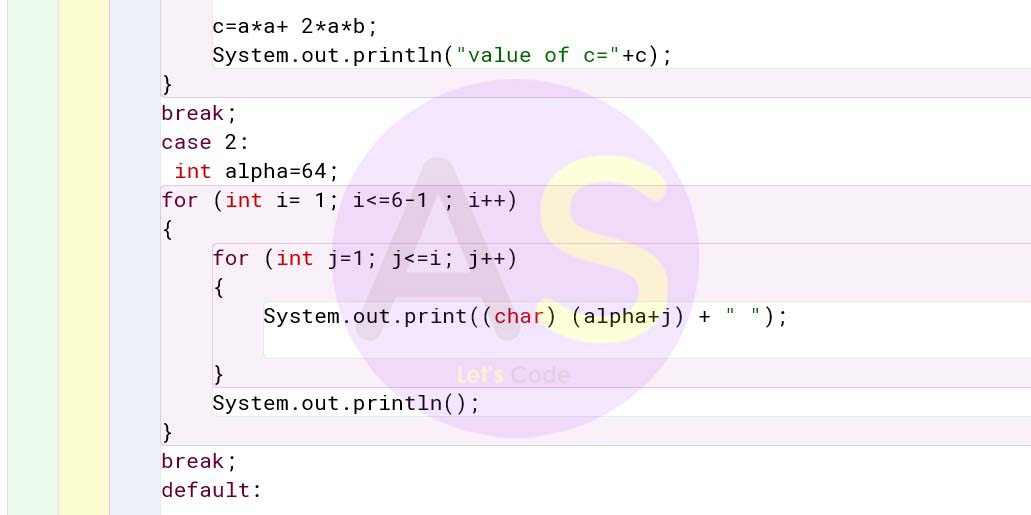

import java.util.*; class Menudriven { public static void main(String args[]){ Scanner sc = new Scanner(System.in); System.out.println("::::MENU::::"); System.out.println(" 1. To print value of c=a"); System.out.println(" 2. To print the following pattern"); System.out.println("Enter your choice:"); int ch= sc.nextInt(); switch(ch) { case 1: double b=3.0; double a,c; for(a=1.0;a<=20.0;a+=2.0) { c=a*a+ 2*a*b; System.out.println("value of c="+c); } break; case 2: int alpha=64; for (int i= 1; i<=6-1 ; i++) { for (int j=1; j<=i; j++) { System.out.print((char) (alpha+j) + " "); } System.out.println(); } break; default: System.out.println("Invalid Input"); break; } } }
Question 9
Write a program to input and store integer elements in a double dimensional array of size 3 x 3 and find the sum of elements in the left diagonal.
Example:
1 3 5
4 6 8
9 2 4
Output: Sum of the left diagonal elements = (1 + 6 +4) = 11
Solution:
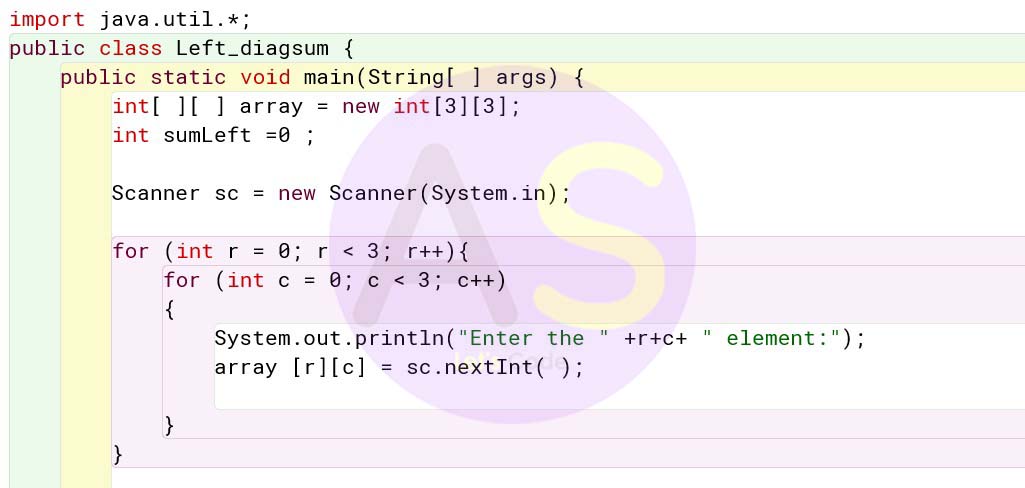
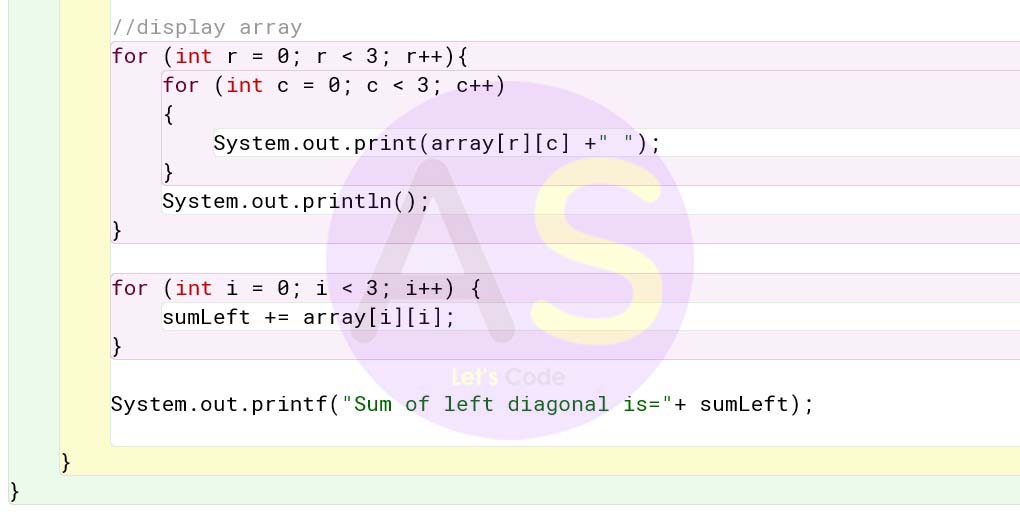
import java.util.*; public class Left_diagsum { public static void main(String[ ] args) { int[ ][ ] array = new int[3][3]; int sumLeft =0 ; Scanner sc = new Scanner(System.in); for (int r = 0; r < 3; r++){ for (int c = 0; c < 3; c++) { System.out.println("Enter the " +r+c+ " element:"); array [r][c] = sc.nextInt( ); } } //display array for (int r = 0; r < 3; r++){ for (int c = 0; c < 3; c++) { System.out.print(array[r][c] +" "); } System.out.println(); } for (int i = 0; i < 3; i++) { sumLeft += array[i][i]; } System.out.printf("Sum of left diagonal is="+ sumLeft); } }