Code is copied!
Design a class NumDude to check if a given number is a Dudeney number or not. (A Dudeney number is a positive integer that is a perfect cube, such that the sum of its digits is equal to the cube root of the number.)
Example: 5832 = (5+8+3+2)=(18)³ = 5832
Some of the members of the class are given below:
Class name : NumDude
Data member/instance variable:
num:to store a positive integer number
Methods/Member functions:
NumDude():default constructor to initialise the data member with legal initial value
void input():to accept a positive integer number
int sumDigits(int x):returns the sum of the digits of number 'x' using recursive technique
void isDude():checks whether the given number is a Dudeney number by invoking the function sumDigits() and displays the result with an appropriate message
Specify the class NumDude giving details of the constructor(), void input(), int sumDigits(int) and void isDude(). Define a main() function to create an object and call the functions accordingly to enable the task.
Solution:
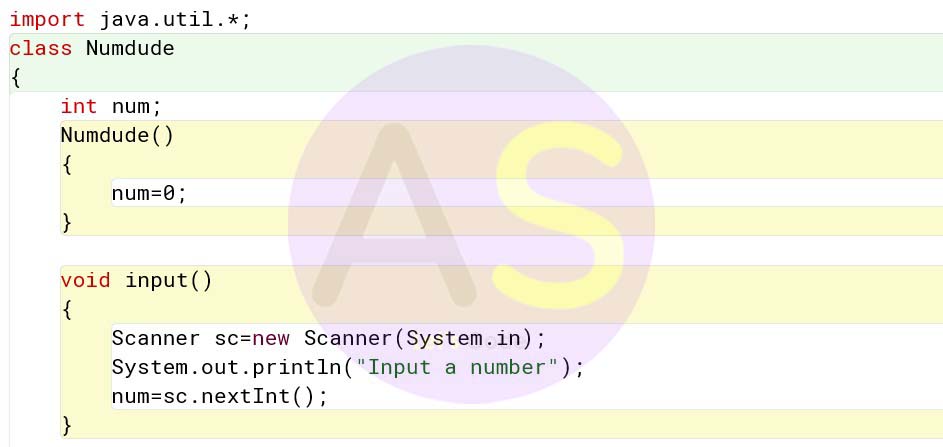
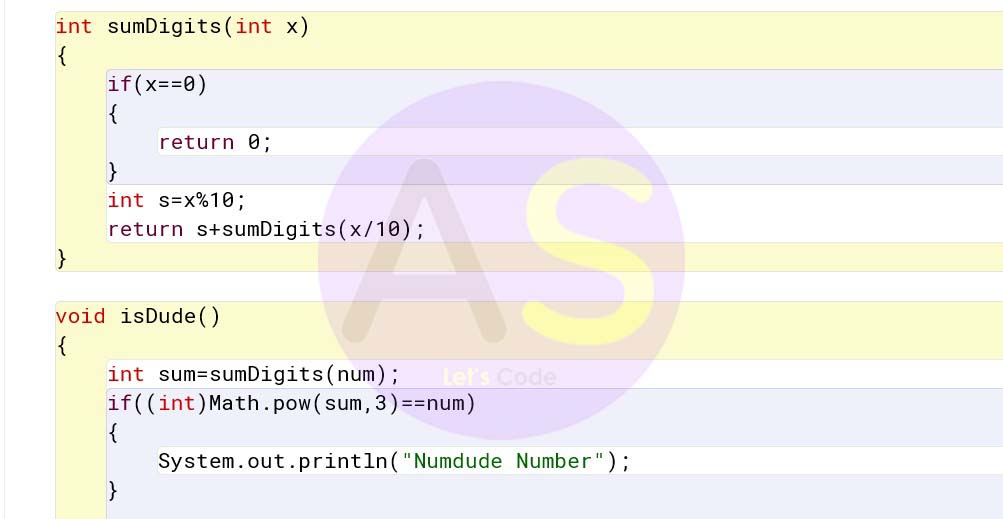
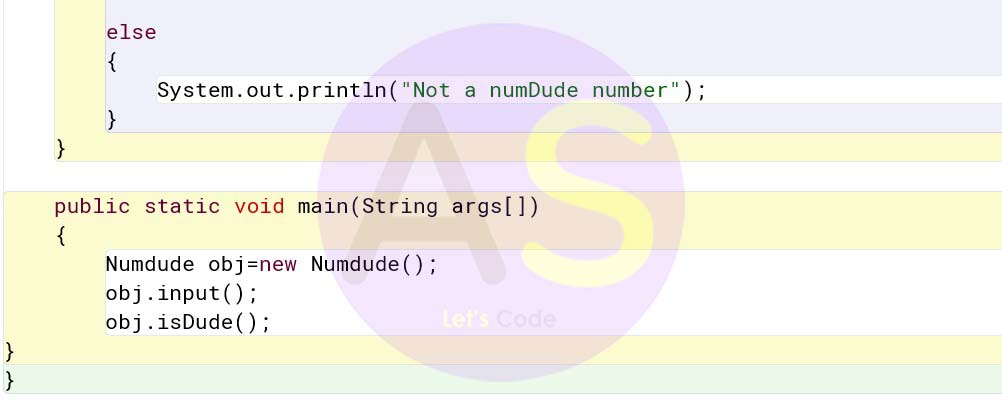
import java.util.*;
class Numdude
{
int num;
Numdude()
{
num=0;
}
void input()
{
Scanner sc=new Scanner(System.in);
System.out.println("Input a number");
num=sc.nextInt();
}
int sumDigits(int x)
{
if(x==0)
{
return 0;
}
int s=x%10;
return s+sumDigits(x/10);
}
void isDude()
{
int sum=sumDigits(num);
if((int)Math.pow(sum,3)==num)
{
System.out.println("Numdude Number");
}
else
{
System.out.println("Not a numDude number");
}
}
public static void main(String args[])
{
Numdude obj=new Numdude();
obj.input();
obj.isDude();
}
}