Code is copied!
Define a class with the following specifications:
Class name: employee
Member variables:
eno — employee number
ename — name of the employee
age — age of the employee
basic — basic salary
[Declare the variables using appropriate data types]
Member methods:
void accept() — accept the details using scanner class
void calculate ()— to calculate the net salary as per the given specifications:
net = basic + hra + da — pf
hra = 18.5% of basic
da = 17.45% of basic
pf = 8.10% of basic
if the age of the employee is above 50 he/she gets an additional allowance of Rs.5000.
void print() — to print the details as per the following format
eno ename age basic net
void main() — to create an object of the class and invoke the methods
Solution:
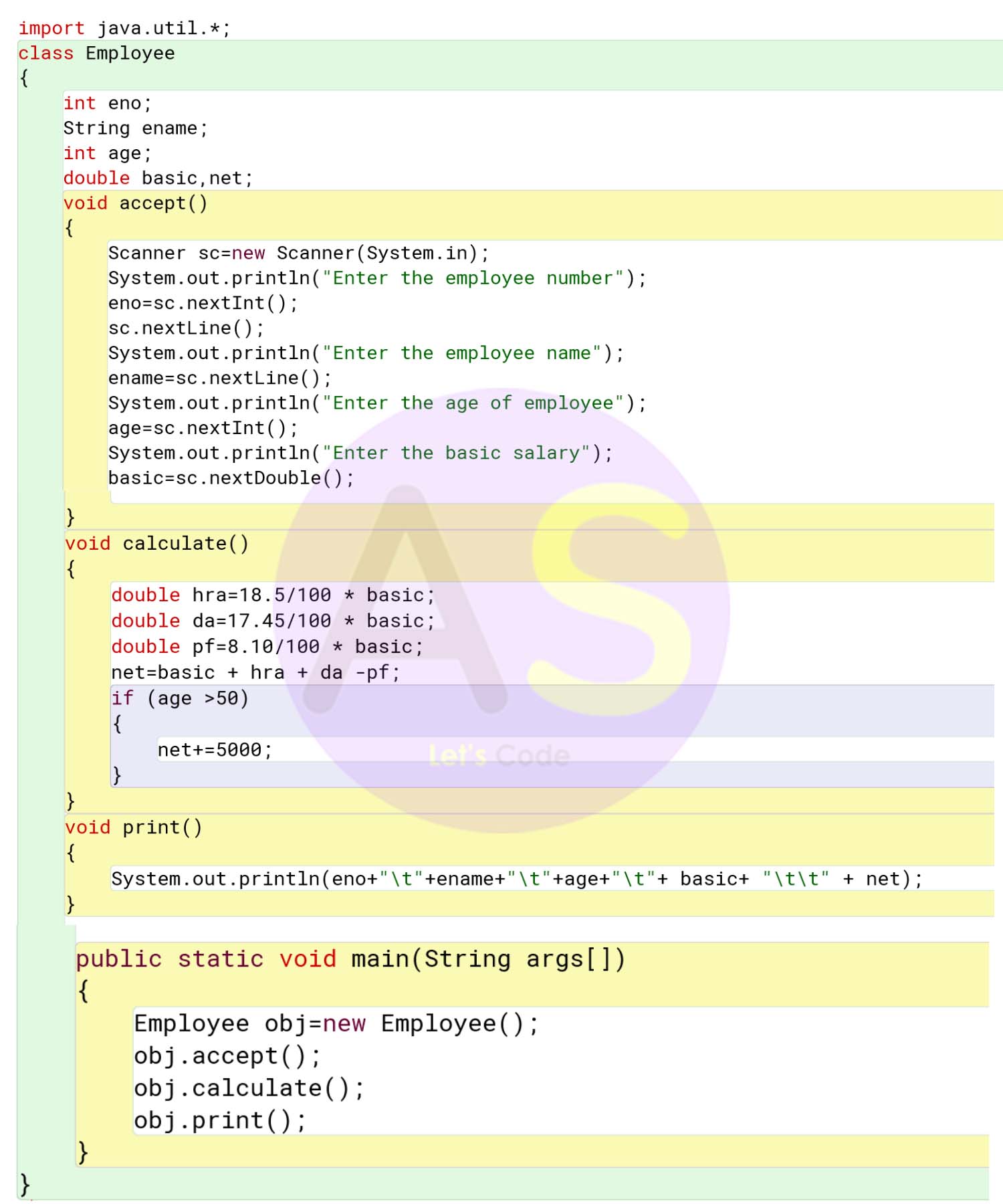
import java.util.*;
class Employee
{
int eno;
String ename;
int age;
double basic,net;
void accept()
{
Scanner sc=new Scanner(System.in);
System.out.println("Enter the employee number");
eno=sc.nextInt();
sc.nextLine();
System.out.println("Enter the employee name");
ename=sc.nextLine();
System.out.println("Enter the age of employee");
age=sc.nextInt();
System.out.println("Enter the basic salary");
basic=sc.nextDouble();
}
void calculate()
{
double hra=18.5/100 * basic;
double da=17.45/100 * basic;
double pf=8.10/100 * basic;
net=basic + hra + da -pf;
if (age >50)
{
net+=5000;
}
}
void print()
{
System.out.println(eno+" "+ename+" "+age+" "+ basic+ " " + net);
}
public static void main(String args[])
{
Employee obj=new Employee();
obj.accept();
obj.calculate();
obj.print();
}
}