Code is copied!
Define a class to overload the function print as follows:
void print(): to print the following format
1 1 1 1
2 2 2 2
3 3 3 3
4 4 4 4
5 5 5 5
void print(int n): To check whether the number is a lead number. A
lead number is the one whose sum of even digits are
equal to sum of odd digits.
e.g. 3669 odd digits sum = 3 + 9 = 12
even digits sum =6+ 6 = 12
3669 is a lead number.
Solution:
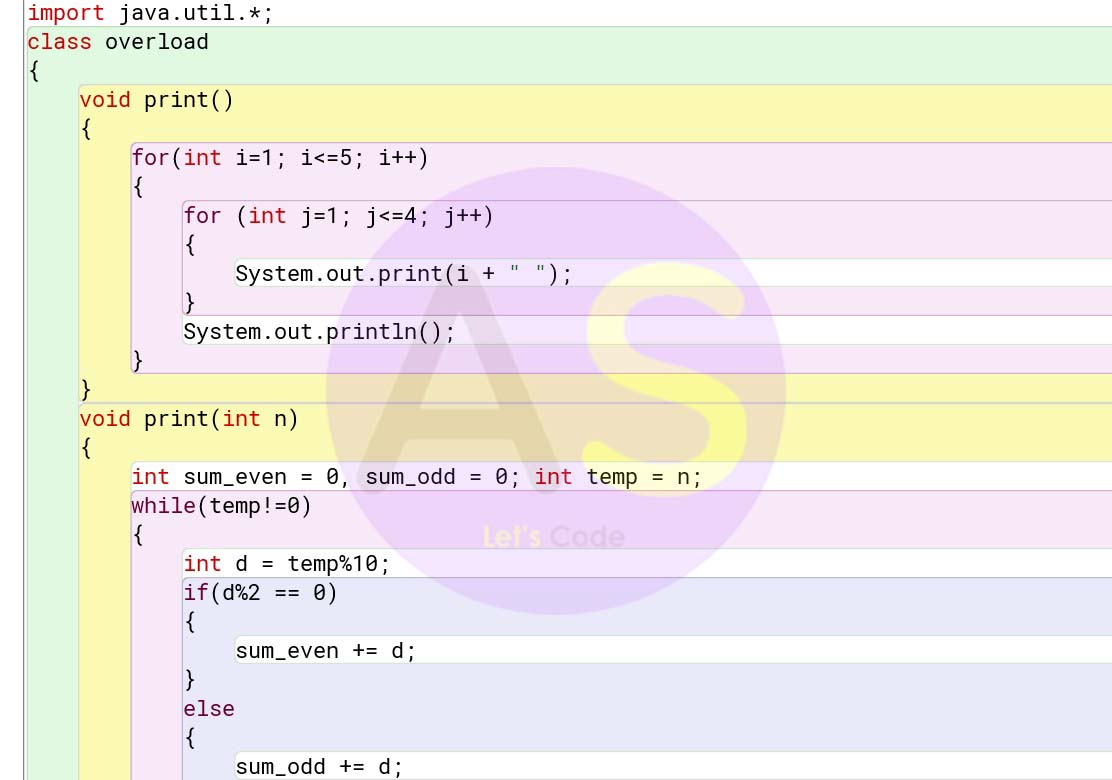
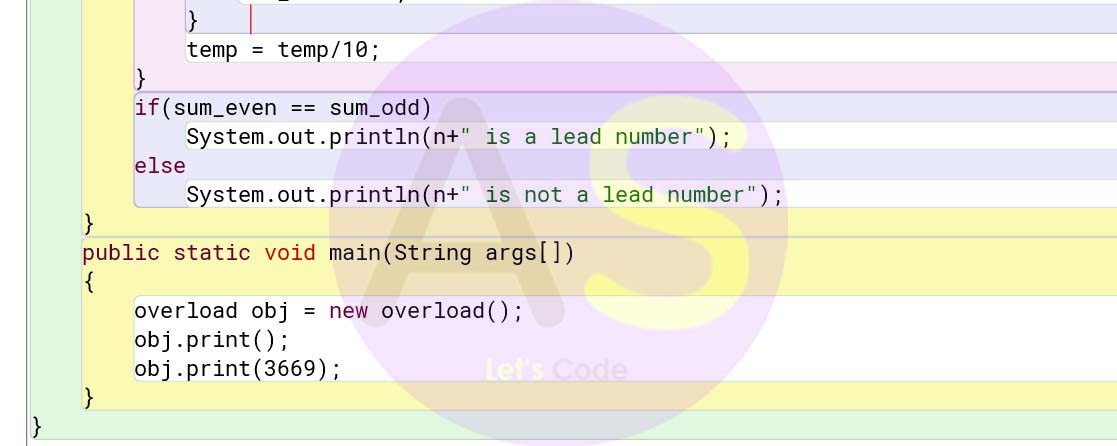
import java.util.*;
class overload
{
void print()
{
for(int i=1; i<=5; i++)
{
for (int j=1; j<=4; j++)
{
System.out.print(i + " ");
}
System.out.println();
}
}
void print(int n)
{
int sum_even = 0, sum_odd = 0; int temp = n;
while(temp!=0)
{
int d = temp%10;
if(d%2 == 0)
{
sum_even += d;
}
else
{
sum_odd += d;
}
temp = temp/10;
}
if(sum_even == sum_odd)
System.out.println(n+" is a lead number");
else
System.out.println(n+" is not a lead number");
}
public static void main(String args[])
{
overload obj = new overload();
obj.print();
obj.print(3669);
}
}