Code is copied!
Define a class to accept 10 integers and arrange them in descending order using bubble sort. Print the original array and the sorted array.
Solution:
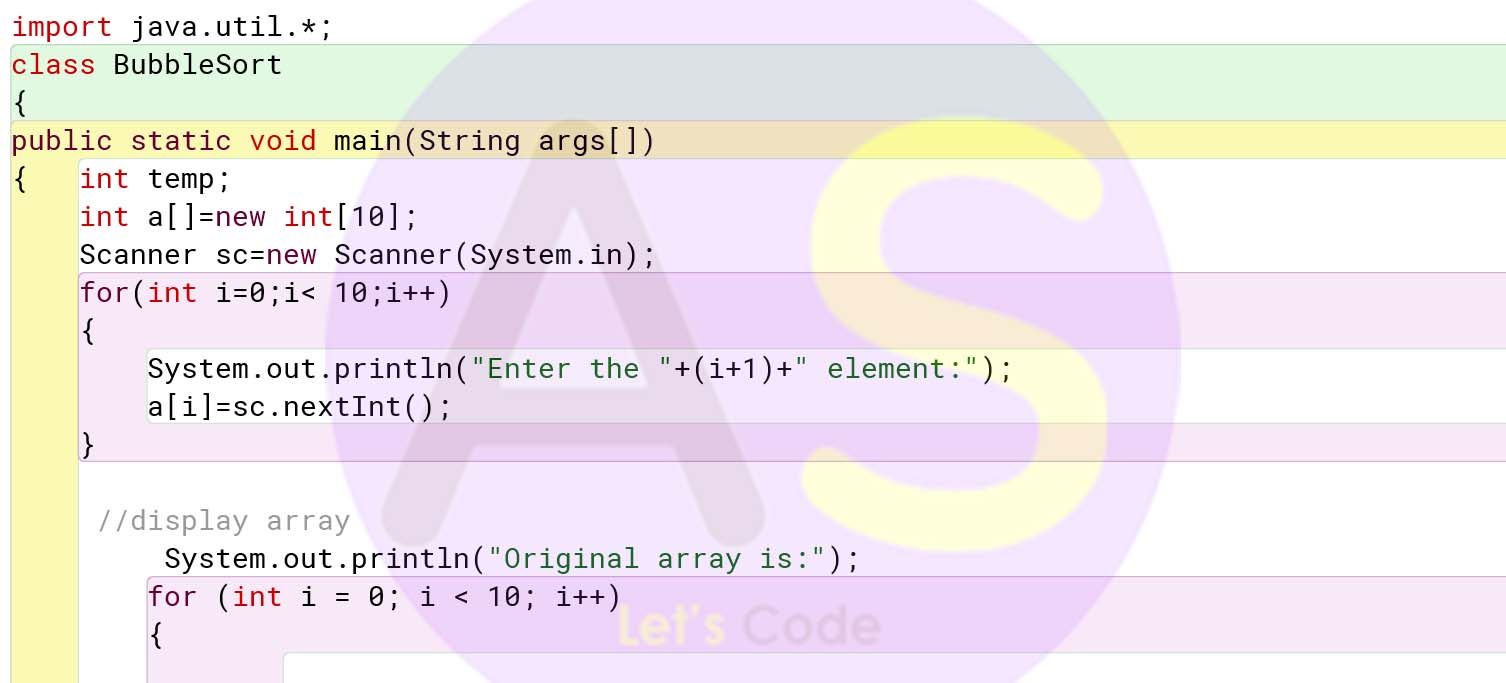
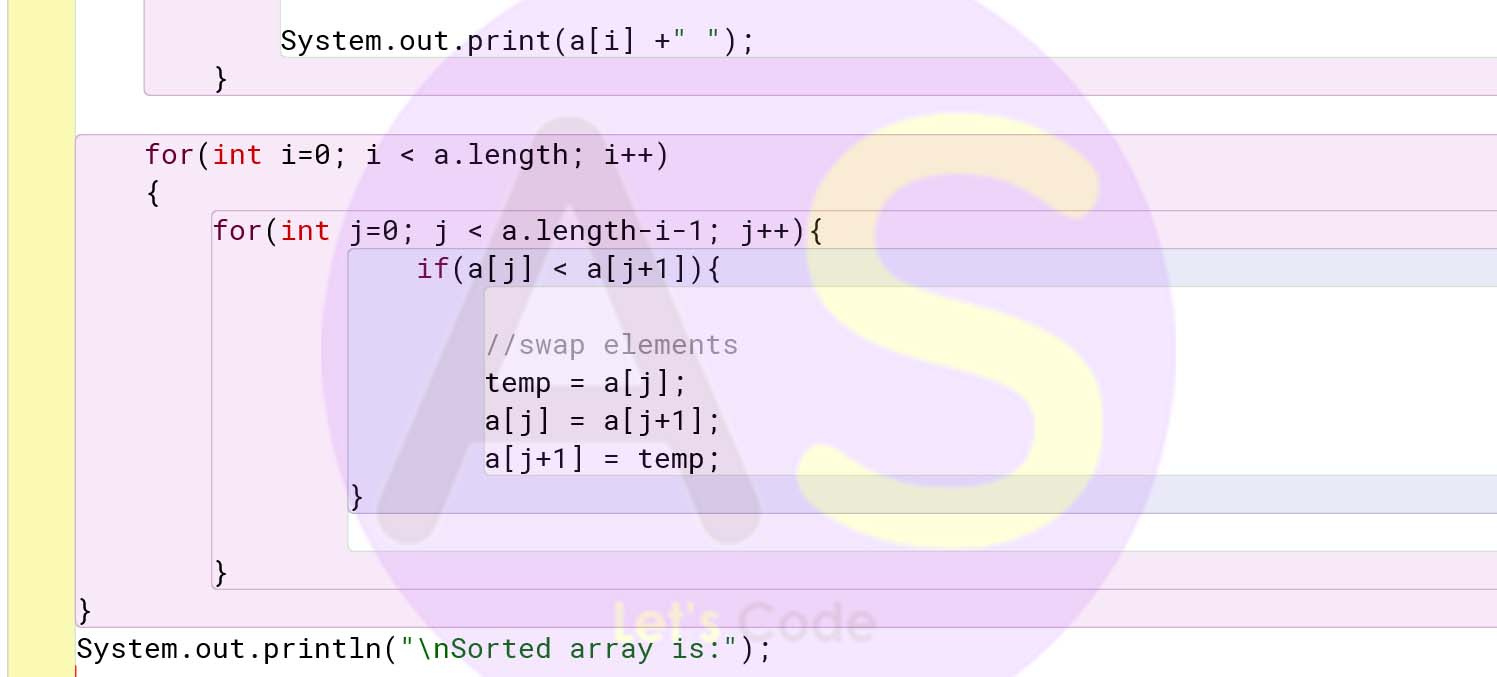

import java.util.*;
class BubbleSort
{
public static void main(String args[])
{ int temp;
int a[]=new int[10];
Scanner sc=new Scanner(System.in);
for(int i=0;i< 10;i++)
{
System.out.println("Enter the "+(i+1)+" element:");
a[i]=sc.nextInt();
}
//display array
System.out.println("Original array is:");
for (int i = 0; i < 10; i++)
{
System.out.print(a[i] +" ");
}
for(int i=0; i < a.length; i++)
{
for(int j=0; j < a.length-i-1; j++){
if(a[j] < a[j+1]){
//swap elements
temp = a[j];
a[j] = a[j+1];
a[j+1] = temp;
}
}
}
System.out.println("
Sorted array is:");
for (int i=0;i< 10;i++)
{
System.out.print(a[i] +" ");
}
}
}