Code is copied!
Define a class to accept values in integer array of size 10. Find sum of one digit
number and sum of two digit numbers entered. Display them separately...
Example:
Input: a[] = {2, 12, 4, 9, 18, 25, 3, 32, 20, 1}
Output:
Sum of one digit numbers :2 + 4 + 9 + 3 + 1 = 19
Sum of two digit numbers : 12 + 18 + 25 + 32 + 20 = 107
Solution:
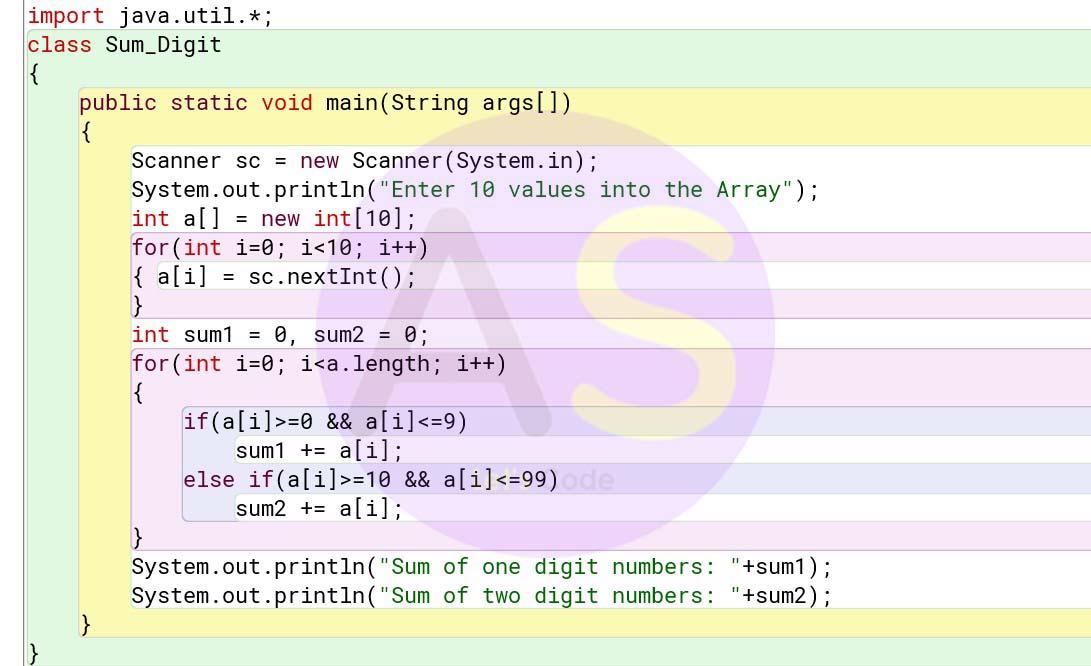
import java.util.*;
class Sum_Digit
{
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.println("Enter 10 values into the Array");
int a[] = new int[10];
for(int i=0; i< 10; i++)
{ a[i] = sc.nextInt();
}
int sum1 = 0, sum2 = 0;
for(int i=0; i< a.length; i++)
{
if(a[i]>=0 && a[i]<=9)
sum1 += a[i];
else if(a[i]>=10 && a[i]<=99)
sum2 += a[i];
}
System.out.println("Sum of one digit numbers: "+sum1);
System.out.println("Sum of two digit numbers: "+sum2);
}
}