Code is copied!
Define a class called with the following specifications:
Class name: Eshop
Member variables:
String name: name of the item purchased
double price: Price of the item purchased
Member methods:
void accept(): Accept the name and the price of the item using the methods of Scanner
class.
void calculate(): To calculate the net amount to be paid by a customer, based on the
following criteria:
void display(): To display the name of the item and the net amount to be paid.
Write the main method to create an object and call the above methods.
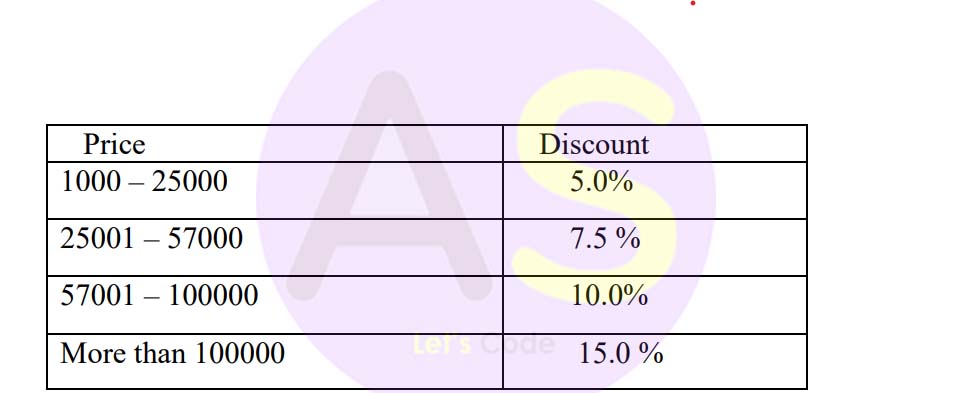
Solution:
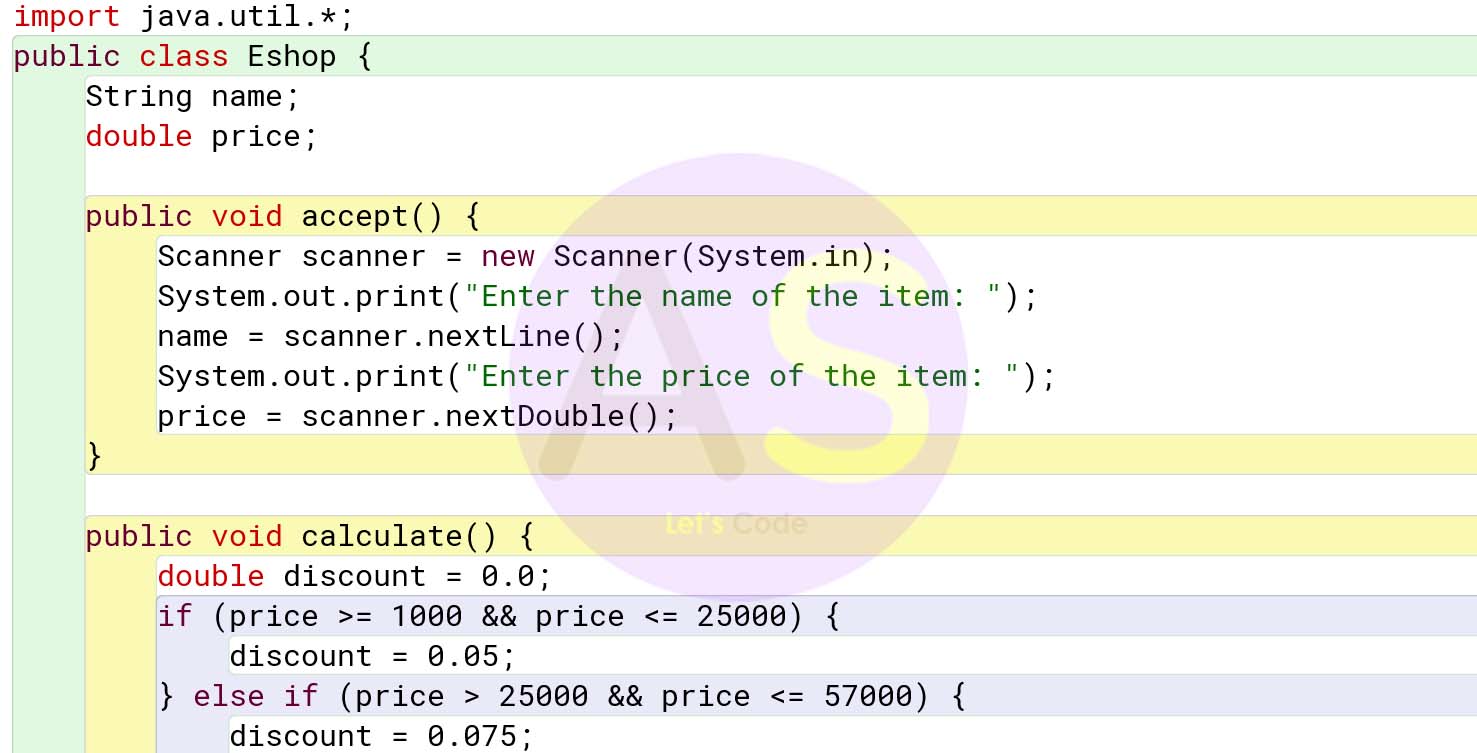
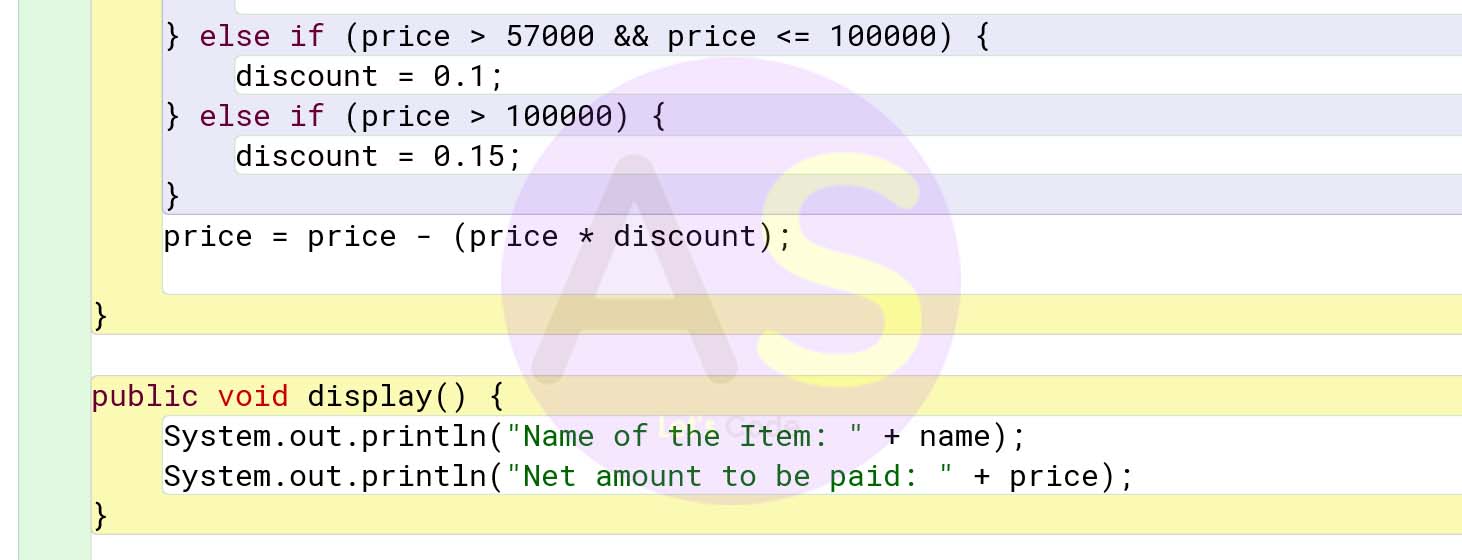
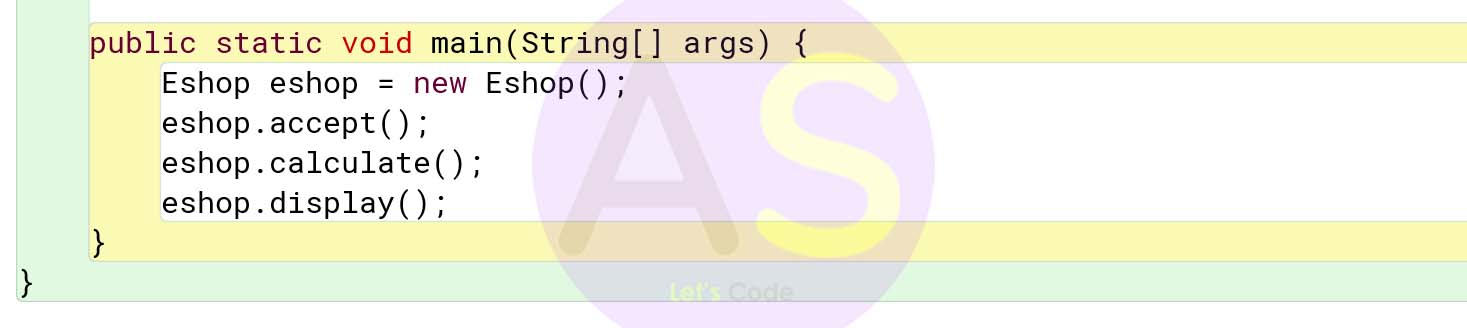
import java.util.*;
public class Eshop {
String name;
double price;
public void accept() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the name of the item: ");
name = scanner.nextLine();
System.out.print("Enter the price of the item: ");
price = scanner.nextDouble();
}
public void calculate() {
double discount = 0.0;
if (price >= 1000 && price <= 25000) {
discount = 0.05;
} else if (price > 25000 && price <= 57000) {
discount = 0.075;
} else if (price > 57000 && price <= 100000) {
discount = 0.1;
} else if (price > 100000) {
discount = 0.15;
}
price = price - (price * discount);
}
public void display() {
System.out.println("Name of the Item: " + name);
System.out.println("Net amount to be paid: " + price);
}
public static void main(String[] args) {
Eshop eshop = new Eshop();
eshop.accept();
eshop.calculate();
eshop.display();
}
}