Code is copied!
A super class Circle has been defined to calculate the area of a circle. Define a subclass Volume
to calculate the volume of a cylinder.
The details of the members of both the classes are given below:
Class name : Circle
Data members/instance variables:
radius : to store the radius in decimals
area : to store the area of a circle
Methods / Member functions:
Circle( ... ) : parameterized constructor to assign values to the data members
void cal_area() : calculates the area of a circle (πr2)
void display( ) : to display the area of the circle
Class name :Volume
Data members/instance variables:
height : to store the height of the cylinder in decimals
volume : to store the volume of the cylinder in decimals
Methods / Member functions:
Volume( ... ) : parameterized constructor to assign values to the
data members of both the classes
double calculate( ) : to calculate and return the volume of the cylinder
using the formula (πr2h) where, r is the radius and
h is the height
void display( ) : to display the area of a circle and volume of a
cylinder
Assume that the super class Circle has been defined. Using the concept of inheritance,
specify the class Volume giving the details of the constructor(...), double calculate( ) and
void display( ).
The super class, main function and algorithm need NOT be written.
Solution:
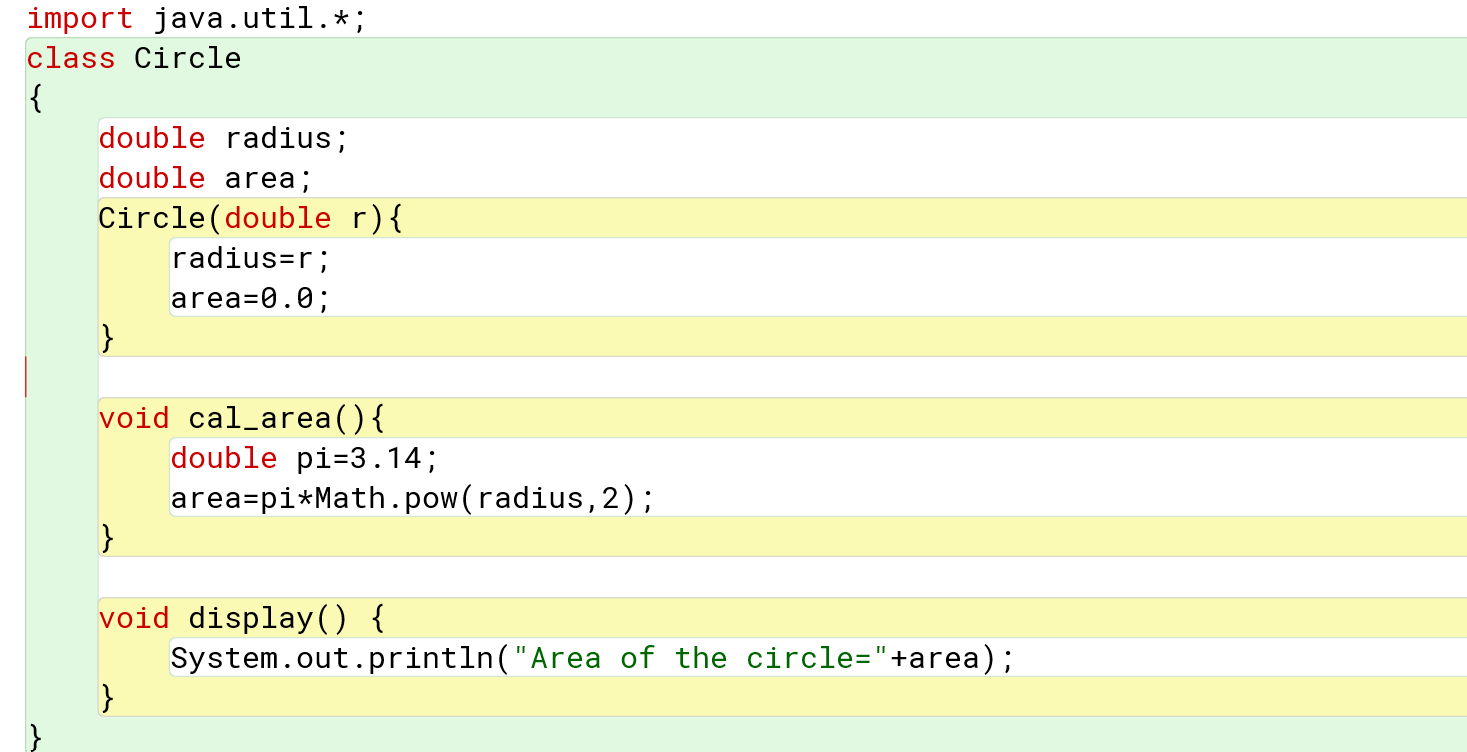
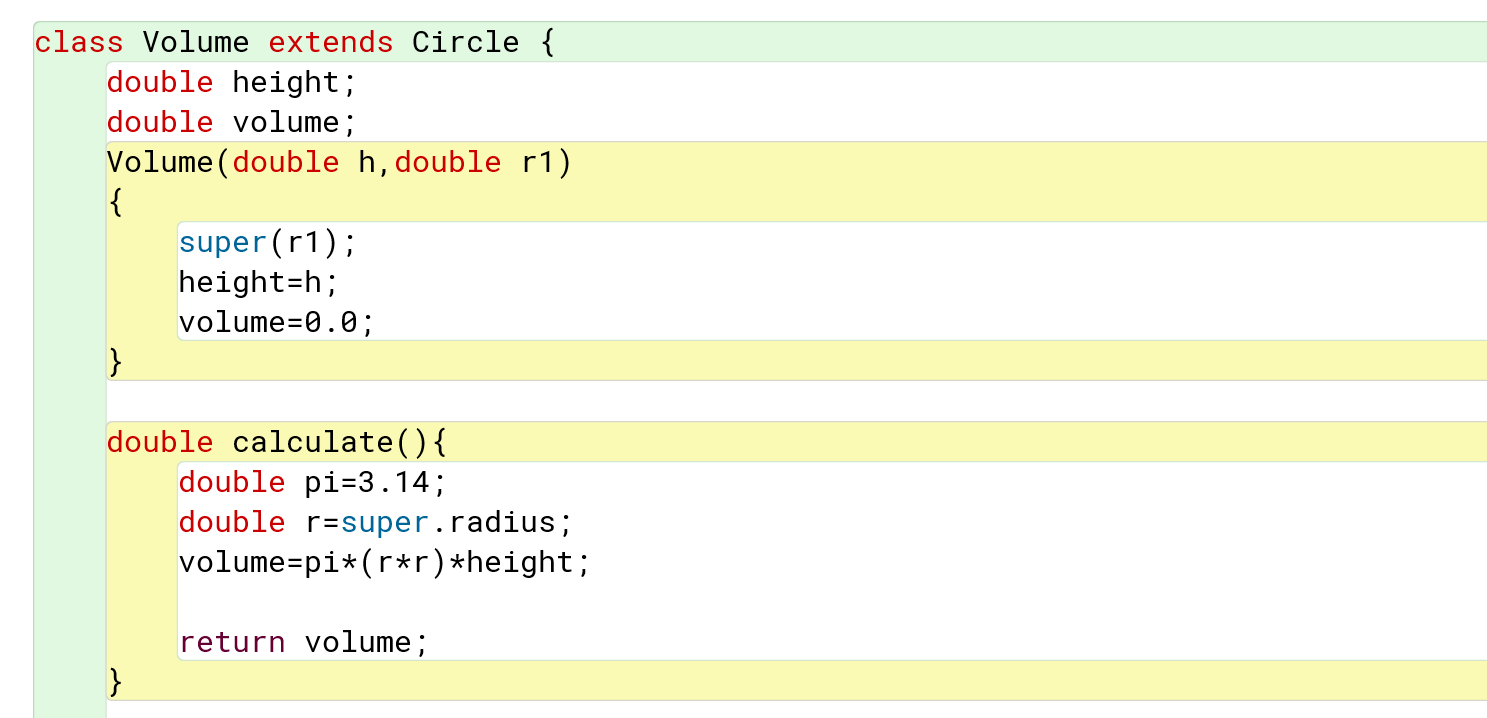

import java.util.*;
class Circle
{
double radius;
double area;
Circle(double r){
radius=r;
area=0.0;
}
void cal_area(){
double pi=3.14;
area=pi*Math.pow(radius,2);
}
void display() {
System.out.println("Area of the circle="+area);
}
}
class Volume extends Circle {
double height;
double volume;
Volume(double h,double r1)
{
super(r1);
height=h;
volume=0.0;
}
double calculate(){
double pi=3.14;
double r=super.radius;
volume=pi*(r*r)*height;
return volume;
}
void display()
{
super.display();
System.out.println("Volume of the cylinder"+volume);
}
}