Code is copied!
A double ended queue is a linear data structure which enables the user to add and remove integers from either ends i.e., from front or rear. The details of the class deQueue are given below:
Class name: deQueue
Data members/ instance variables:
Qrr[]:array to hold integer elements
lim: maximum capacity of the dequeue
front: to point the index of the front end
rear:to point the index of the rear end
Methods/Member functions:
deQueue(int l):constructor to initialise lim= 1, front = 0 and rear = 0
void addFront(int v):to add integers in the dequeue at the front end if possible, otherwise display the message "OVERFLOW FROM FRONT"
void addRear(int v):to add integers in the dequeue at the rear end if possible, otherwise display the message "OVERFLOW FROM REAR"
int popFront():removes and returns the integers from the front end of the dequeue if any, else returns -999
int popRear(): removes and returns the integers from the rear end of the dequeue if any, else returns -999
void show():displays the elements of the dequeue
Specify the class deQueue giving details of the functions void addFront(int) and int popFront(). Assume that the other functions have been defined. The main() function and algorithm need NOT be written.
Solution:
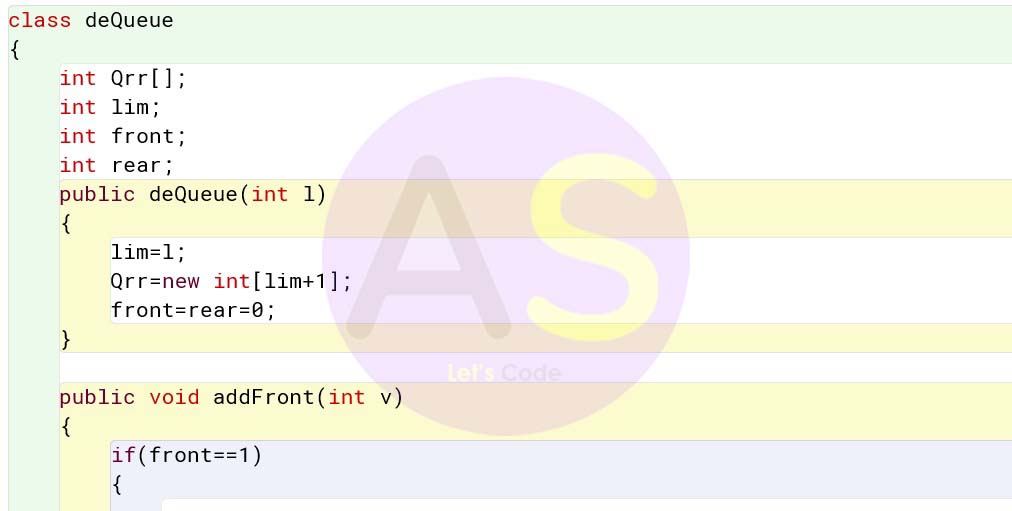
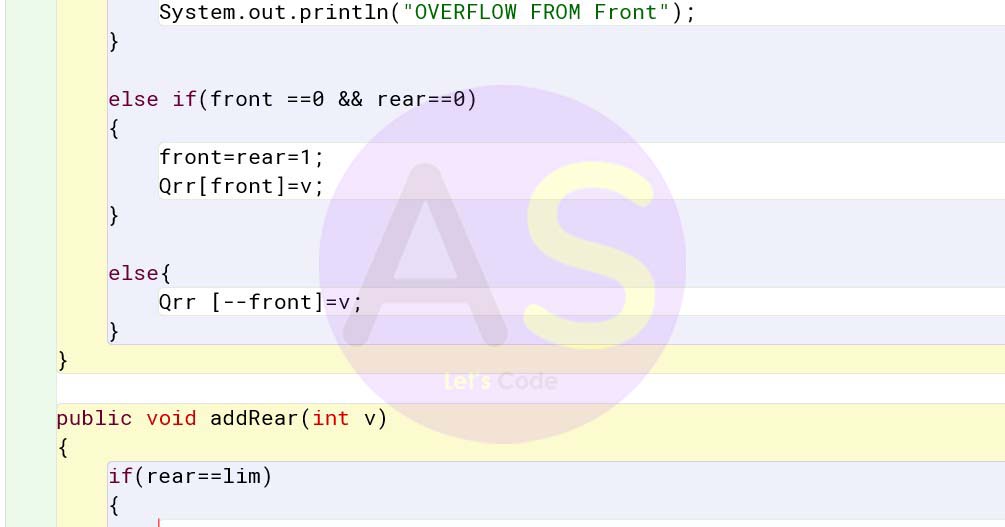
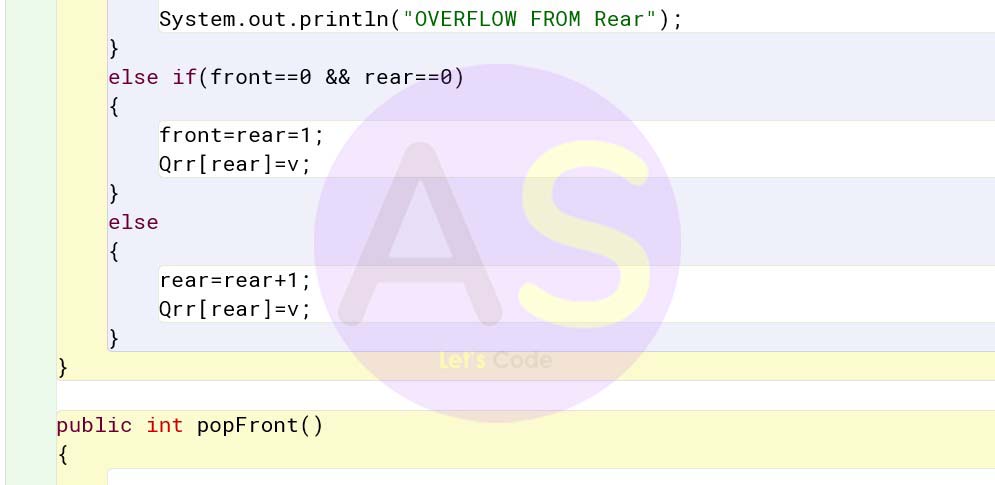
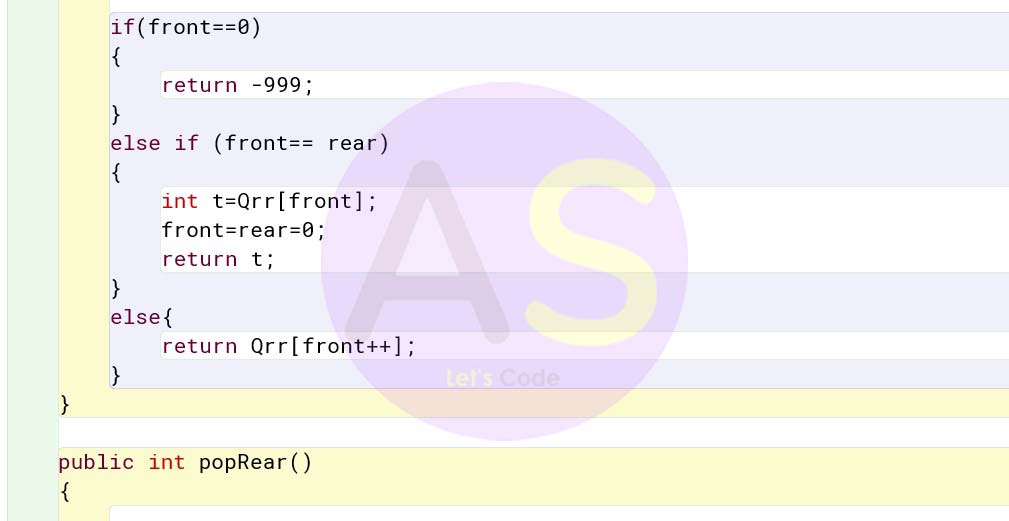
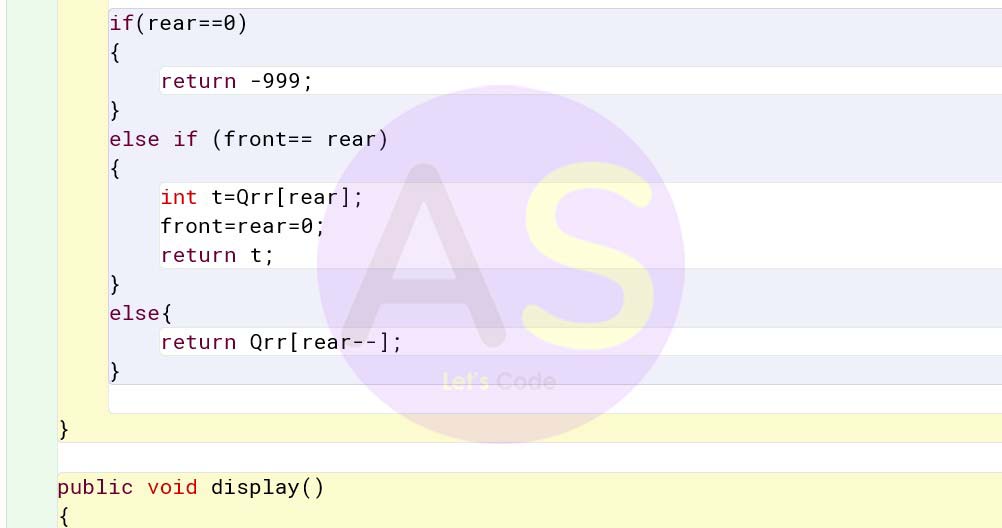
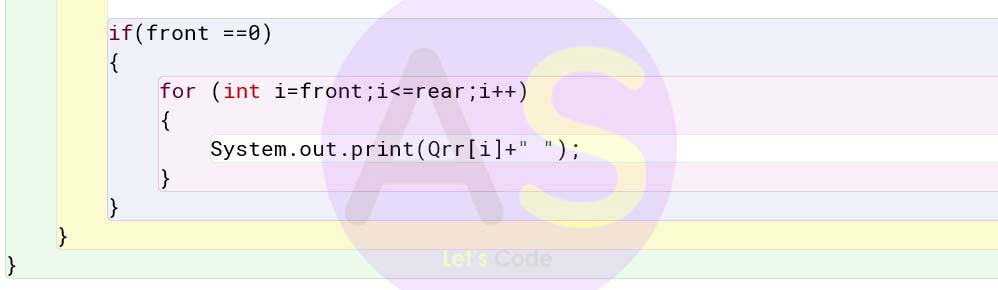
class deQueue
{
int Qrr[];
int lim;
int front;
int rear;
public deQueue(int l)
{
lim=l;
Qrr=new int[lim+1];
front=rear=0;
}
public void addFront(int v)
{
if(front==1)
{
System.out.println("OVERFLOW FROM Front");
}
else if(front ==0 && rear==0)
{
front=rear=1;
Qrr[front]=v;
}
else{
Qrr [--front]=v;
}
}
public void addRear(int v)
{
if(rear==lim)
{
System.out.println("OVERFLOW FROM Rear");
}
else if(front==0 && rear==0)
{
front=rear=1;
Qrr[rear]=v;
}
else
{
rear=rear+1;
Qrr[rear]=v;
}
}
public int popFront()
{
if(front==0)
{
return -999;
}
else if (front== rear)
{
int t=Qrr[front];
front=rear=0;
return t;
}
else{
return Qrr[front++];
}
}
public int popRear()
{
if(rear==0)
{
return -999;
}
else if (front== rear)
{
int t=Qrr[rear];
front=rear=0;
return t;
}
else{
return Qrr[rear--];
}
}
public void display()
{
if(front ==0)
{
for (int i=front;i<=rear;i++)
{
System.out.print(Qrr[i]+" ");
}
}
}
}