Code is copied!
A class Trans is defined to find the transpose of a square matrix. A transpose of a matrix is obtained by interchanging the elements of the rows and columns.
Example: If size of the matrix = 3, then
Some of the members of the class are given below:
Class name: Trans
Data members/instance variables:
arr[][]:to store integers in the matrix
m:integer to store the size of the matrix
Methods/Member functions:
Trans(int mm): parameterised constructor to initialise the data member m = mm
void fillarray():to enter integer elements in the matrix to create the transpose of the given matrix
void transpose():to create the transpose of the given matrix
void display():displays the original matrix and the transposed matrix by invoking the method transpose()
Specify the class Trans giving details of the constructor(), void fillarray(), void transpose() and void display(). Define a main() function to create an object and call the functions accordingly to enable the task.
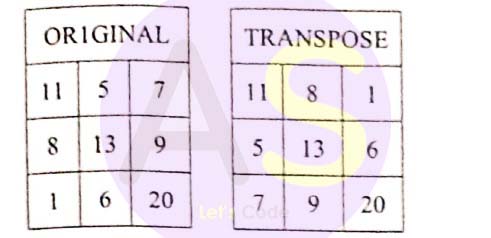
Solution:
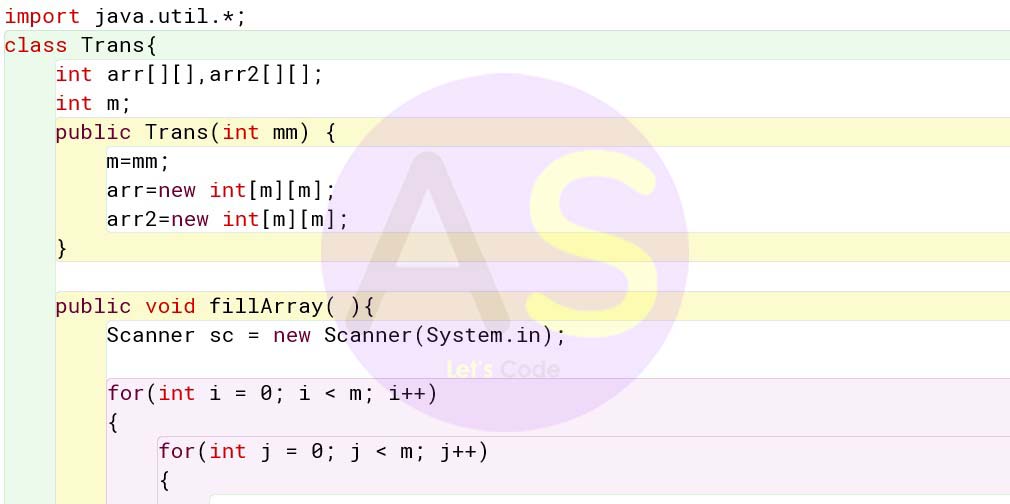
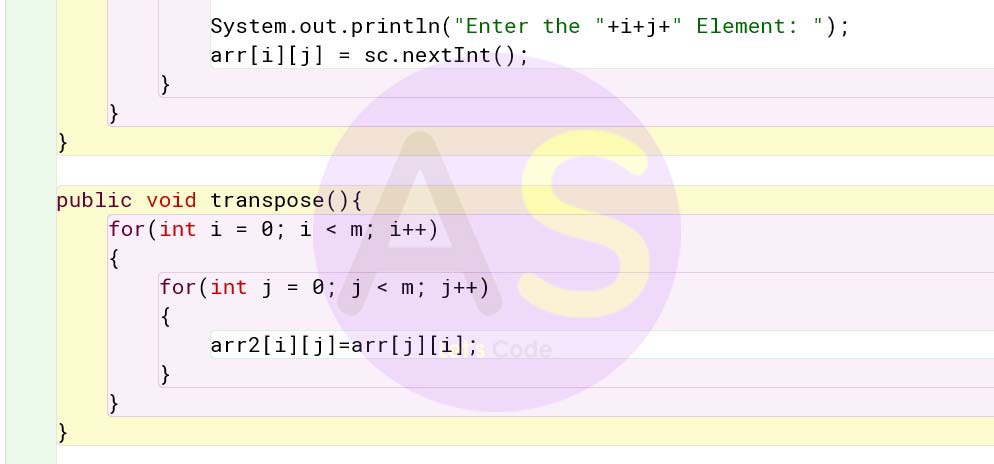
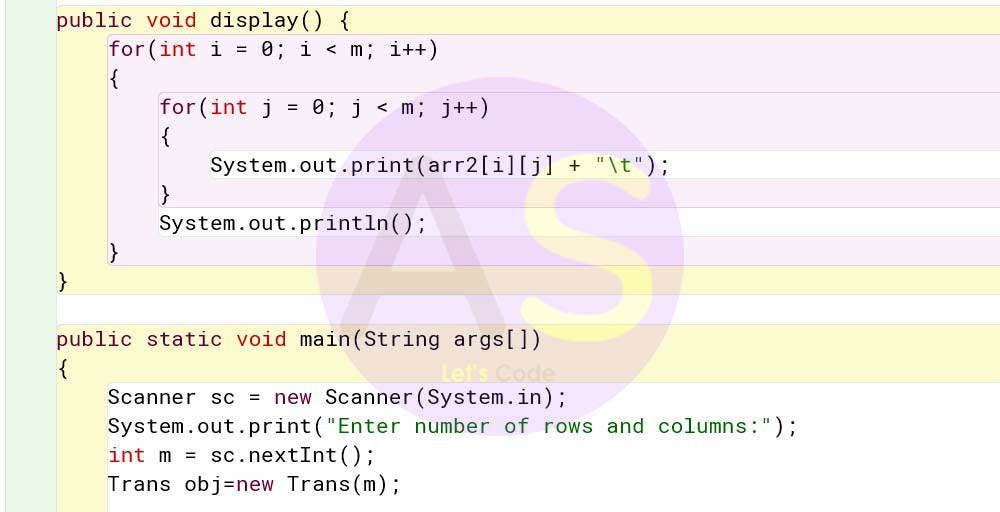
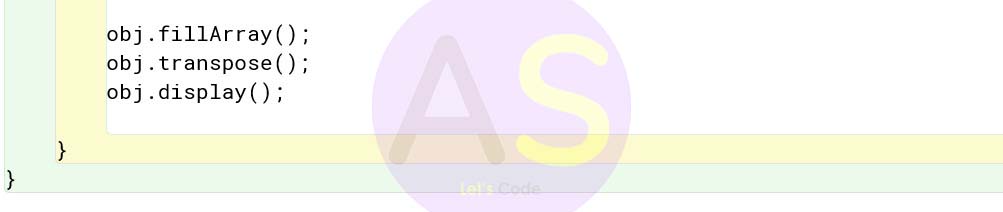
import java.util.*;
class Trans{
int arr[][],arr2[][];
int m;
public Trans(int mm) {
m=mm;
arr=new int[m][m];
arr2=new int[m][m];
}
public void fillArray( ){
Scanner sc = new Scanner(System.in);
for(int i = 0; i < m; i++)
{
for(int j = 0; j < m; j++)
{
System.out.println("Enter the "+i+j+" Element: ");
arr[i][j] = sc.nextInt();
}
}
}
public void transpose(){
for(int i = 0; i < m; i++)
{
for(int j = 0; j < m; j++)
{
arr2[i][j]=arr[j][i];
}
}
}
public void display() {
for(int i = 0; i < m; i++)
{
for(int j = 0; j < m; j++)
{
System.out.print(arr2[i][j] + " ");
}
System.out.println();
}
}
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.print("Enter number of rows and columns:");
int m = sc.nextInt();
Trans obj=new Trans(m);
obj.fillArray();
obj.transpose();
obj.display();
}
}